❔ Call base method in multiple inheritance
Example of what i have:
When i call C#Foo() i get "Hello World!" but i only want to call the base method of A so my console result would be "Hello !"
I hope you guys can understand what i want.
How can i doing this
35 Replies
You can't do that
Okay
What you basically want is something like
base.base.Foo()
, right?
Unfortunately it's not possibleYeah, i mean something like that
Maybe with reflection ?
It's possible with reflection, but at this point the question would be why do that?
the only thing that comes to mind would be to extract it as protected non virtual method to call it later in C
I want to override OnClick() from WPF Button which is already overrwriten in a framework i use, but i have to use the button because its the NavigationViewItem from WPF UI
Sorry i cant do that because i have no access to the framework
You need to pass as a parent
OnClick? Why do you want to override it?
I dont want to use the WPF UI navigation, i want to use my own, because wpf ui dont support multiple pages of the same type with there same instance
I guess at this point your only solution is reflection, or extending WPF without the additional framework on top of it
Works perfectly
that still invokes it virtually
What does that mean ?
Aaron
REPL Result: Success
Console Output
Quoted by
<@270457259065081856> from #void-spam (click here)
Compile: 499.563ms | Execution: 96.666ms | React with ❌ to remove this embed.
it still calls the version in the derived class
But it works
I think this is because i use the type of ButtonBase and NavigationViewItem extends from it.
I did the same thing there
I used A as the type for reflection, which B derives from
How can i use MODiX ?
$eval
To compile C# code in Discord, use
!eval
Example:
Please don't try breaking the REPL service. Also, remember to do so in #bot-spam!Kleidukos
REPL Result: Success
Compile: 722.838ms | Execution: 98.919ms | React with ❌ to remove this embed.
Oh, i forgot the result...
This won't even work, you need
BindingFlags.Public
as well and as antiwraith said it's still a virtual call
I believe you'd need to get a function pointer to it
typeof(A).GetMethod("Foo").MethodHandle.GetFunctionPointer();
something like that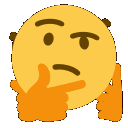
Need the public bindingflag
BindingFlags.Public
oh I added Instance again
lmao
Aaron
REPL Result: Success
Console Output
Compile: 718.116ms | Execution: 87.091ms | React with ❌ to remove this embed.
yeah there
Are we trying to output "!" ?
trying to get Hello
In my app it works, why not here
I dont understand why it works in my project (Not the example)
Here a screen from my code
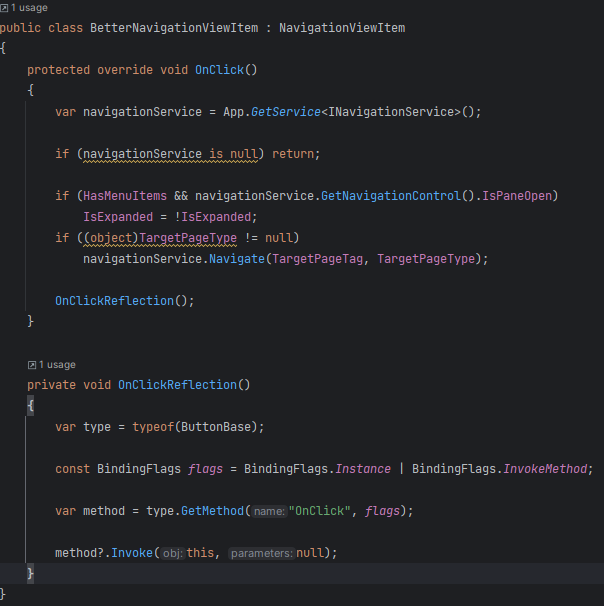
And i checked, method is not null
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.