❔ ASP.NET always validates invalid JWT
I have a custom configuration for Asp.net where the JWT Token is stored in an http-only cookie, because of this there is 1 method in specific that is always returning 200 no matter if there is no actual JWT attached
57 Replies
When a different jwt token requests data of a diferent id it returns an empty array but 200
are you sure it returns 200 even with no JWT? shouldn't be possible 🤔 . I assume you still had a JWT stored somewhere that authenticated you, is that possible?
How about using expiration on the JWT? accept JWT only for 24 hours or smth and include check for expiration date in your policy maybe
Yes, let me show you
not sure how you would show this to me. What exactly does
options.Save = true
mean? where does it save the JWT?so its fetched from a cookie which is currently set
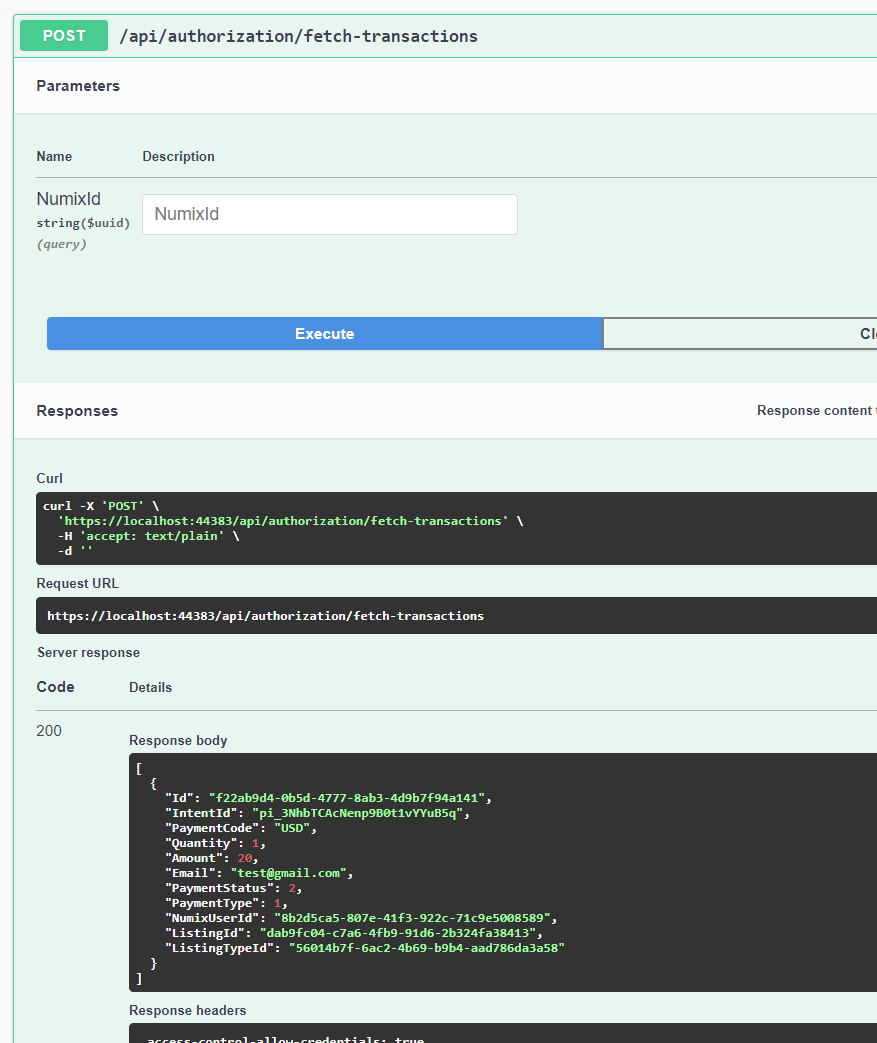
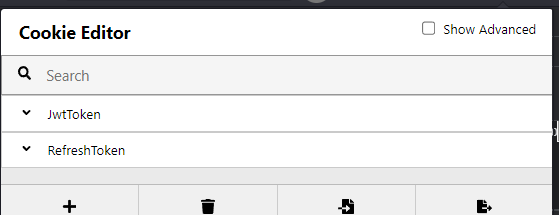
also sure just a sec
I remove the cookie / log out and seems to still be allowing it
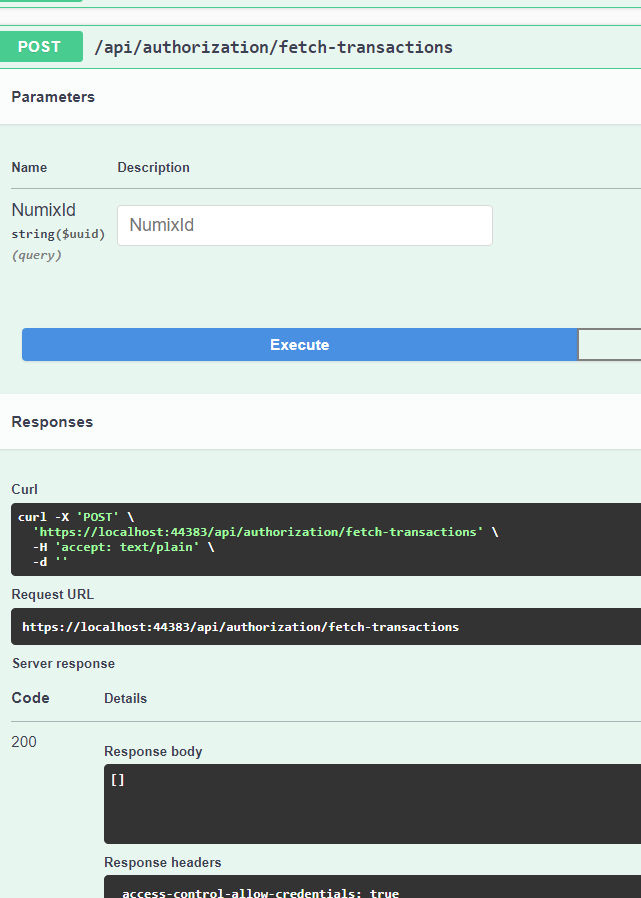
it doesnt really allowt o fetch other ids but
Possibly like you said this

let me check
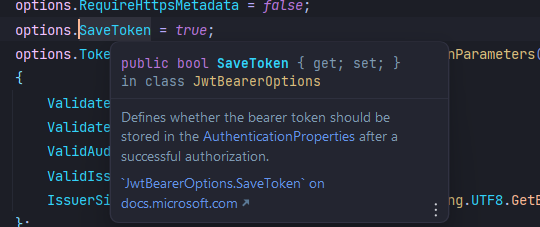
I guess a JWT might have been saved there from your last auth
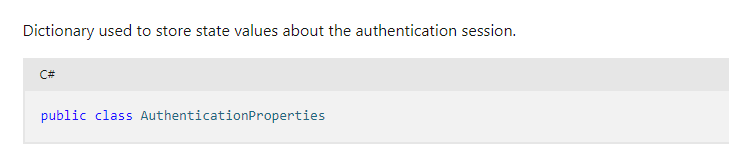
just a sec cuz i gotta remeber the passoword
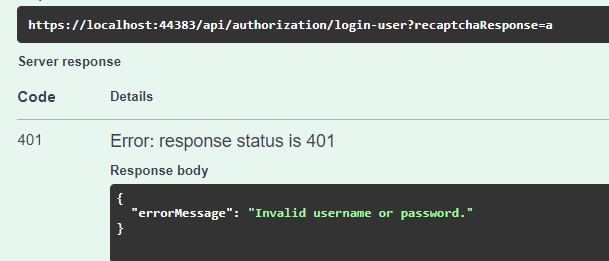
no still no
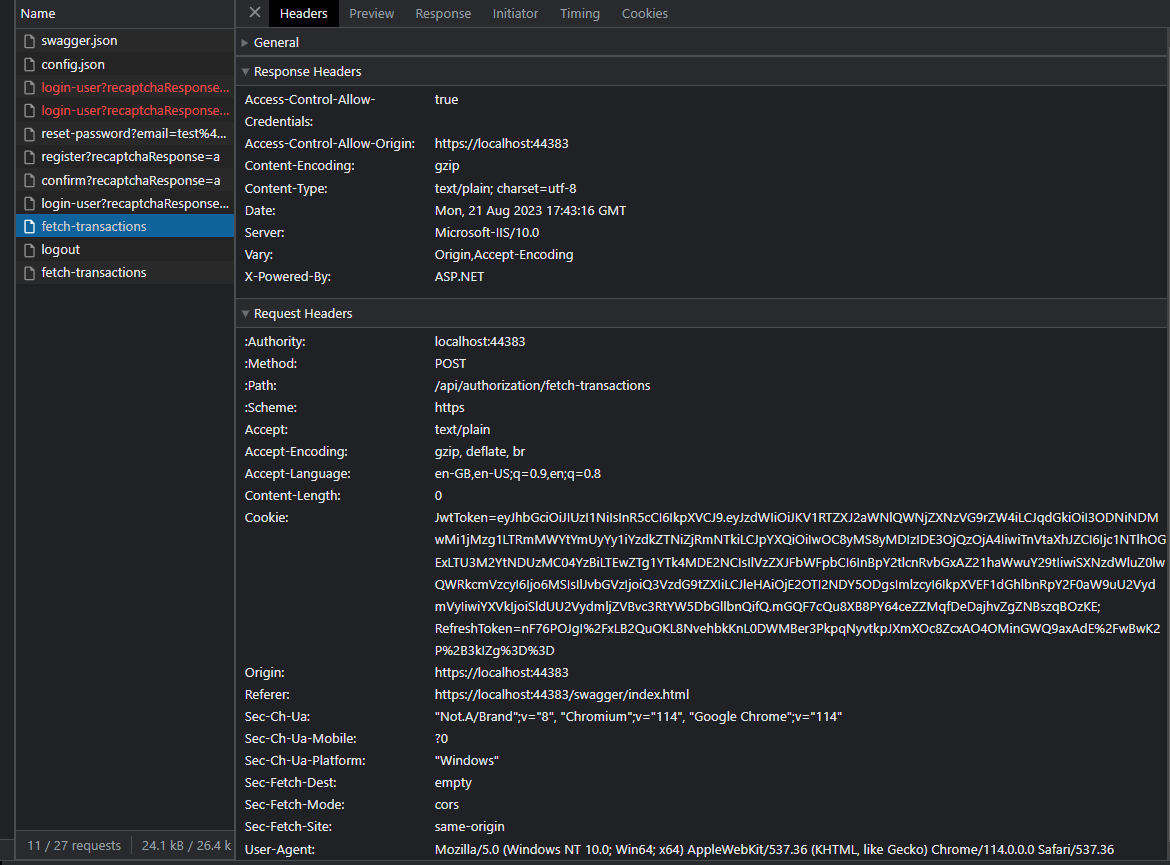
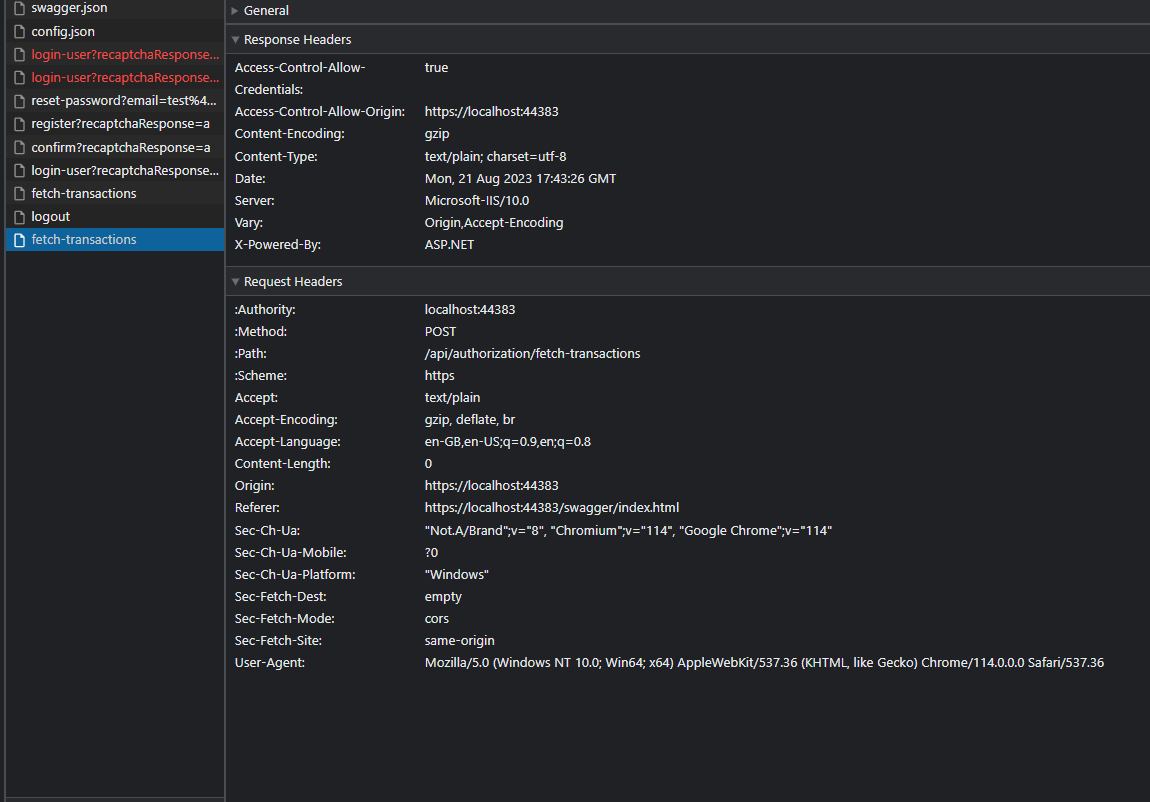
both are 200s
Not sure if this will help us find out but when you go in browser into dev tools where you use swagger onto Application, can you find a JWT token in cookies there? Not sure what name the cookie would have, any cookie value that starts with "ey..."?
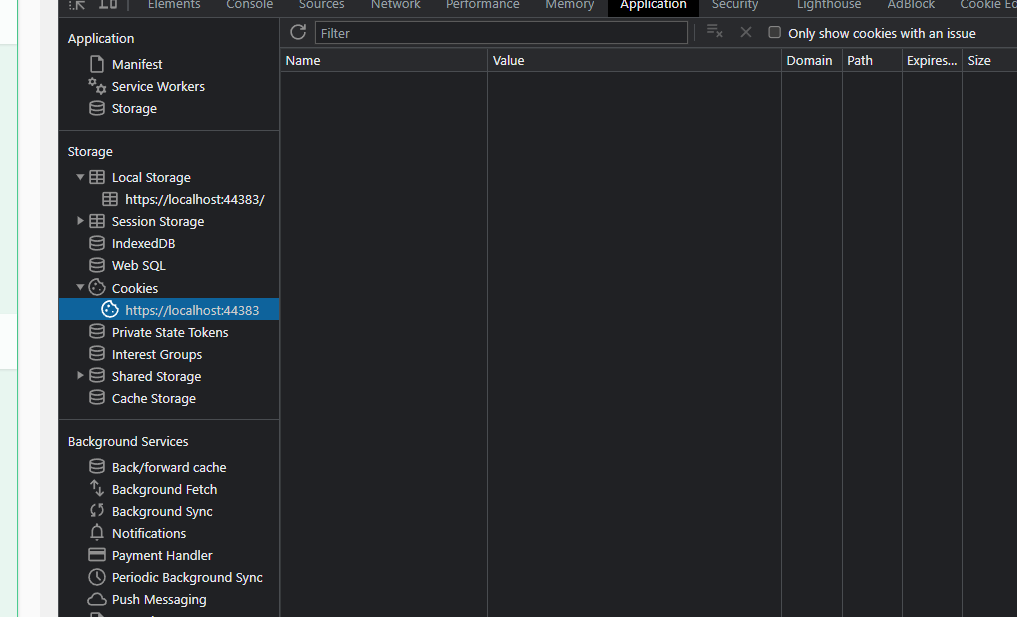
im thinking could it be because of this
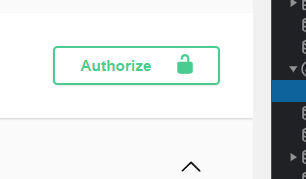
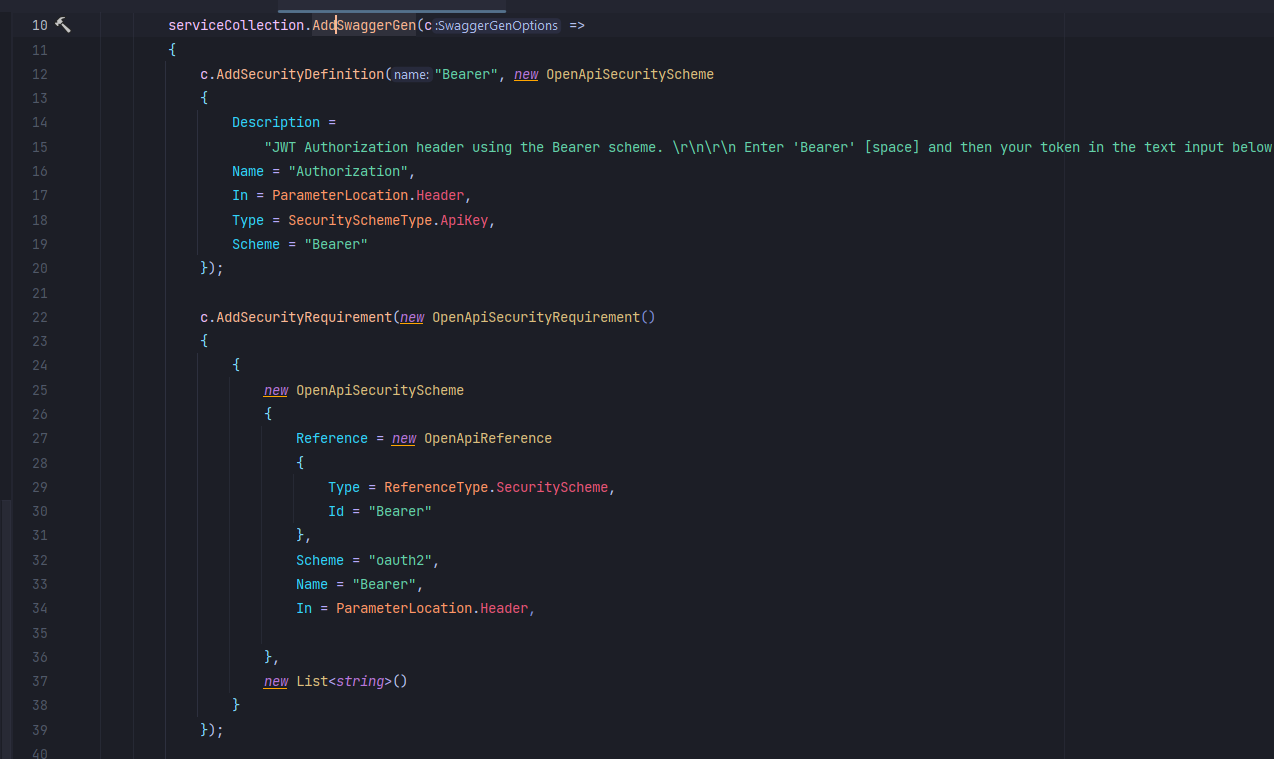
i mean its old and wont work now anyway but
im thinking now it could be smthn to do with one of my middlewares
but i dont think thats the case
weird because it seems like its skipping over auth completly for this specific method
this seems to add the Token to request in Swagger I would guess
this request here contains a token
this one does not
yes thats before log out
yes
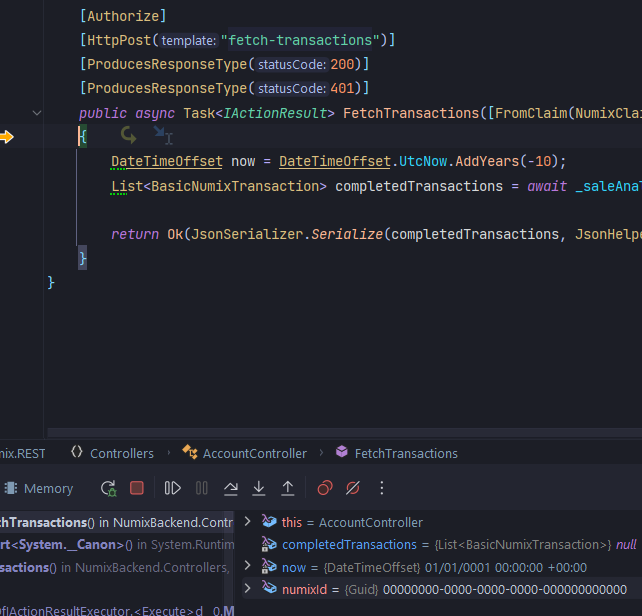
for some reason its skipping authorization
have you tried adding the name of your auth policy on the attribute?
it should apply it anyway but I'm not sure
that auth policy breaks everything and puts everything on 403 so i dont use it anymore
then the one you'd like to be using
thats the thing there isnt any policy
in the middleware you have shown to me there was 🤷♂️
let me check
that could be why
oh this

its not used anywhere i just removed it
yep
okay
seems like a bug within asp but im not sure
very unlikely
more likely that you and me are both being 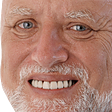
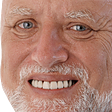
probably
Do you have
AllowAnonymous
on your controller? @S-IERRAyes
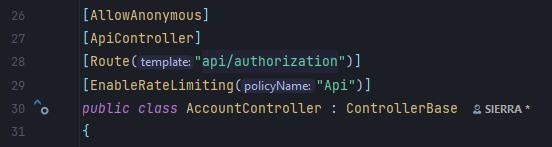
but shouldnt authorise on the method its self over write this?
No, it's the other way around afaik
I ll try unlinking that allow anonymous on all other methods
Inlining
You don't need to inline it
and remove it from the class
As long as the controller doesn't have
Authorize
it will allow Anonymouse accsess by defaultim thinking for some reason allow anounymous is over writing that access controller below which wouldnt make sense but it could be
ok that was indeed the case
i assume this is a ASP.NET bug
possibly someone from asp.net team could investigate?
I don't think it's a bug tbh
i mean allow anon on class shouldnt over write authorize on method
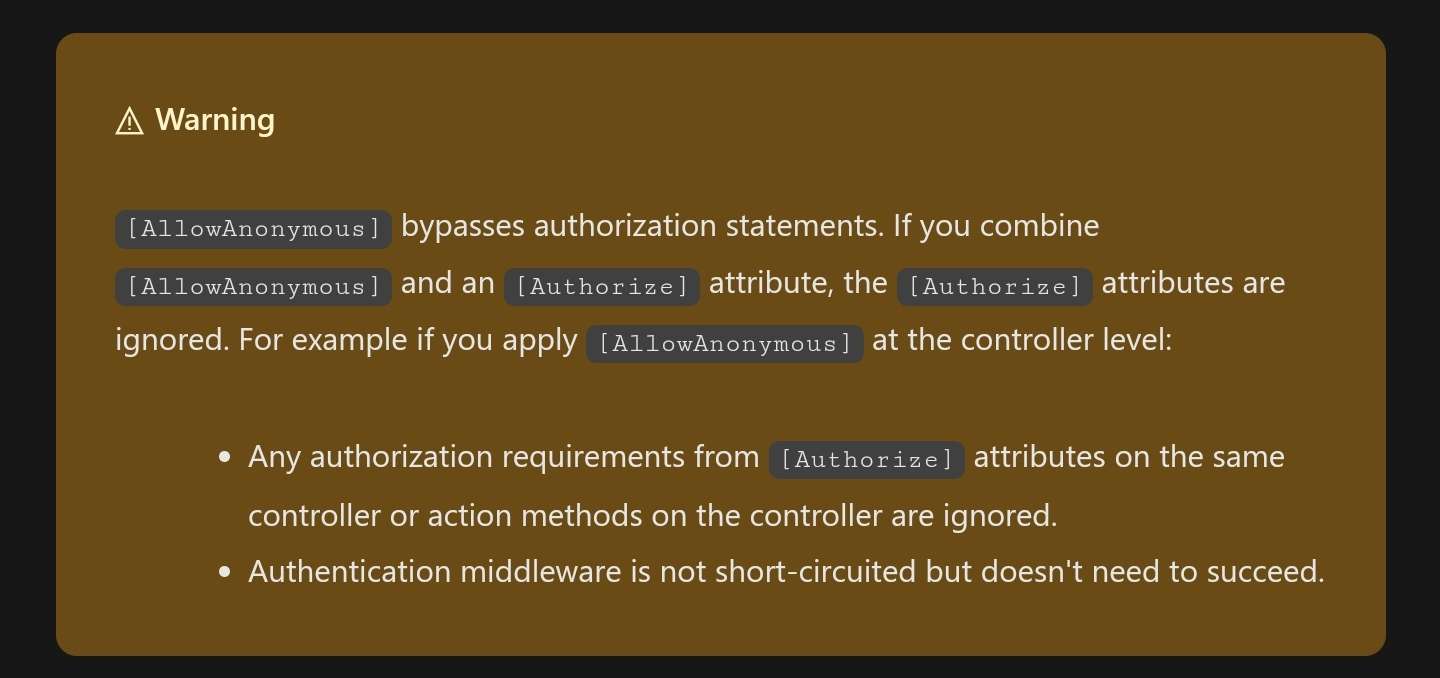
^
oh shit
alright thanks thats good to know
It seems a more likely scenario you'd have Authorize on your controller and AllowAnonymous on a specific method
yes i see now
thanks
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.