❔ Class with Func<> and class initializers
If I define a class with members to be passed to known create type functions how do I set the Func<> member to the known function?
For example,
Thank you in advance.
50 Replies
Besides public fields, the code seems fine
What is the
PROBLEM HERE?
?Thank you for the reponse. Let me look at it. . .
Sorry, around this expression the compiler is compainong about :
Right at the comma at the end of "one",
Probably because of the missing
new Known
or at least a new
C# is not JavascriptAnd I just tried adding that too
To create a new
Known
it would either need
a) a constructor
b) properties instead of fieldsIt was complaining so I removed it
Adding constructor now . .
would work, if you used properties instead of fields
would work, if you had a constructor
tried too earlier, let me go back to it.
The first method didn't work because you have public fields
Instead of public properties
(
new() {...}
)Angius
REPL Result: Success
Result: <>f__AnonymousType0#1<int>
Compile: 482.180ms | Execution: 115.355ms | React with ❌ to remove this embed.
No need for
()
That didn't create a
Foo
thoAh, huh
You're right
Fixed
With new { . . . } now the compiler complains about using null for the return type member:
Show code
Which makes sense given that is literally an c# interface. I need to replace it with the baseclass. . .
True,
null
cannot implement an interface
But, also, that field is not nullable eithershould be able to be null just fine
what definitely won't work is
new IRet
Angius
REPL Result: Success
Result: Bar
Compile: 481.026ms | Execution: 52.361ms | React with ❌ to remove this embed.
It seems to work, yeah, you're right
In that case something else is wrong
But since we don't have the updated code... the answer is ¯\_(ツ)_/¯
Cannot assign '<null>' to anonymous type property
seems pretty clear to meero
REPL Result: Failure
Exception: CompilationErrorException
Compile: 687.224ms | Execution: 0.000ms | React with ❌ to remove this embed.
typor 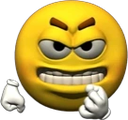
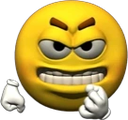
Cannot create an instance of the abstract type or interface 'IRet'
That's a different messagei mean yeah
i'm referring to this
Which... is a different error
An abstract class didn't help. But if I understood correctly the interface should work too?
Just show us your updated code already
There is a lot of it. And, more importantly, sensitive. It is painstaking to recreate in Discord,
Then we're wading in the dark
"Doctor, it hurts"
"Show me where?"
"Me hurty"
"Okay, but what hurts?"
"Tea didn't help"
"???"
If there's nothing to work with, well, let's hope Ero and Thinker can help you, because I'm out
it's almost difficult to believe there can possibly be any sensitive data where there isn't even any working code
Does this work?
i don't know, does it?
You can't instantiate an interface
Added the ?
But that didn't help. ? means nullable?
It does, yes
IRet iret = new(param1, param2);
You can't instantiate an interfaceWould the construtor help with (trying now):
No
Instantiating a concrete class would
You cannot instantiate an interface
You cannot instantiate an abstract class
You can only ever instantiate a concrete class
As in new Known(. . .)?
As in
I am getting two. . .
Changine frm { to )
Are you initializing a
Known
with
again...?That was my initial setup . . . since added new Known { . . . } now trying new Know( . . . ) and adding the constructor
will work ONLY if you write your code properly and turn those public fields into public properties
Can do that. Let me try now. . .
For reference, $structure
For C# versions older than 10, see
$StructureOld
Making changes (a few) be right back . . . thank you all for the help.
Thank you. The compile and build worked. I need to test it now. Using as properties did the trick. And I am still using an interface as the base.
Continued use and improvements of what I worked on is successful. Thank you all.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.