ā Async Method with current code.
I am having a hell of a brain fart, probably my damn ASD again.
Can someone help me fix this?
Thanks
57 Replies
DownloadCoverArtAsync(cover, archivedFolderPath);
await this call
private static string GetFilePath(StringBuilder builder, TrackViewModel track, string downloadPath, FileFormat fileFormat)
make this method async Task<string>
And await
it wherever you call it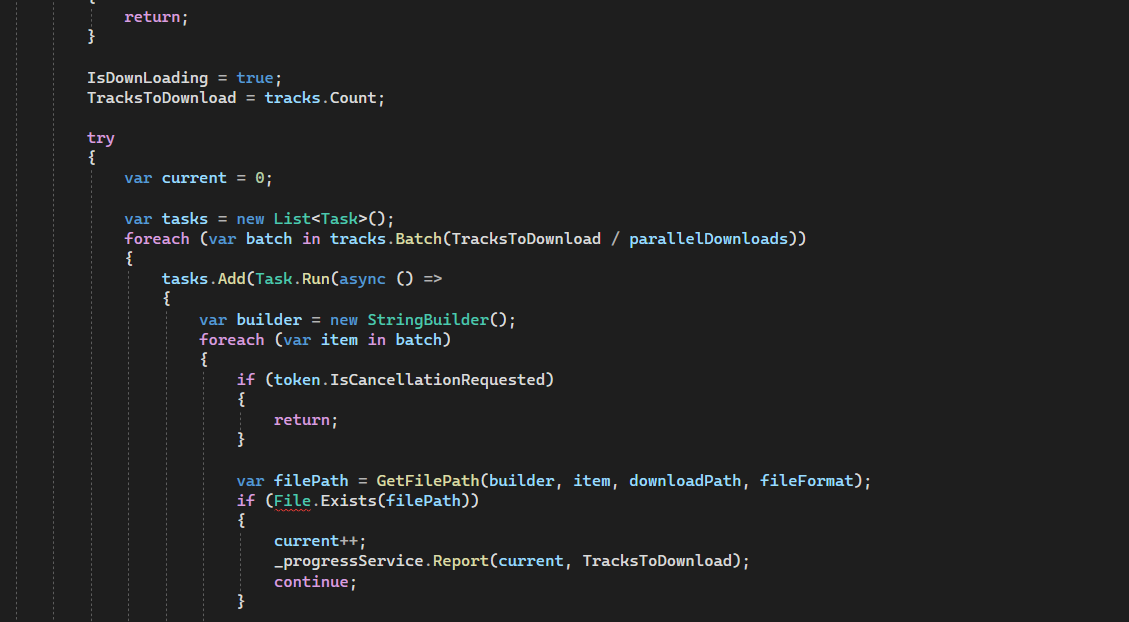
that broke something else now š¦
CS0104 'File' is an ambiguous reference between 'System.Net.WebRequestMethods.File' and 'System.IO.File' S
Remove the reference to
System.Net.Web
or whatever it is that brings this class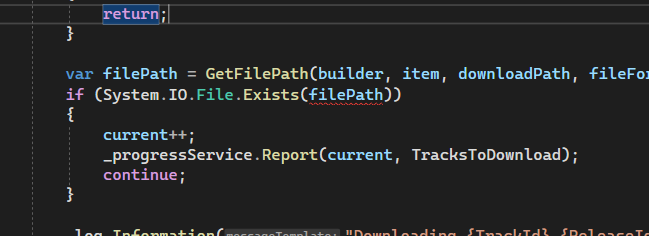
grrrrrr
OK
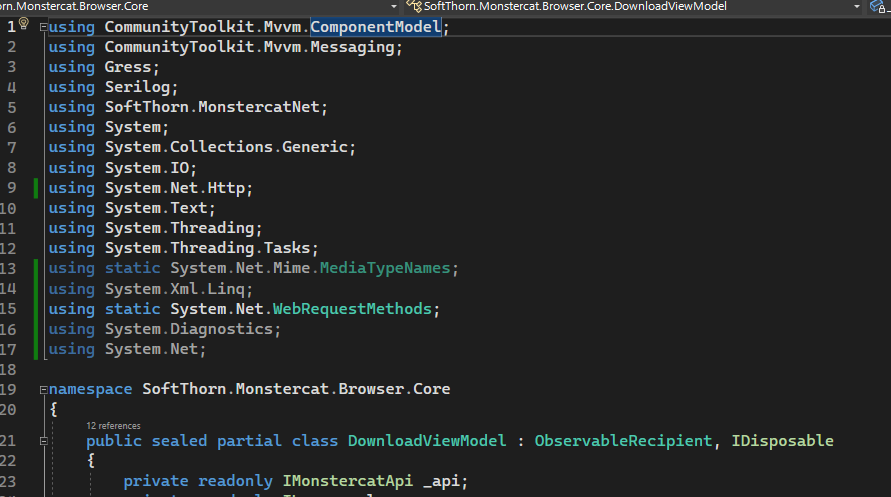
nothing
oh, i see it
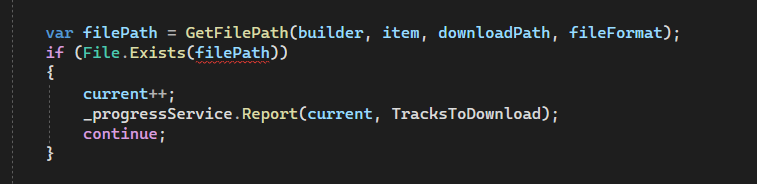
nope
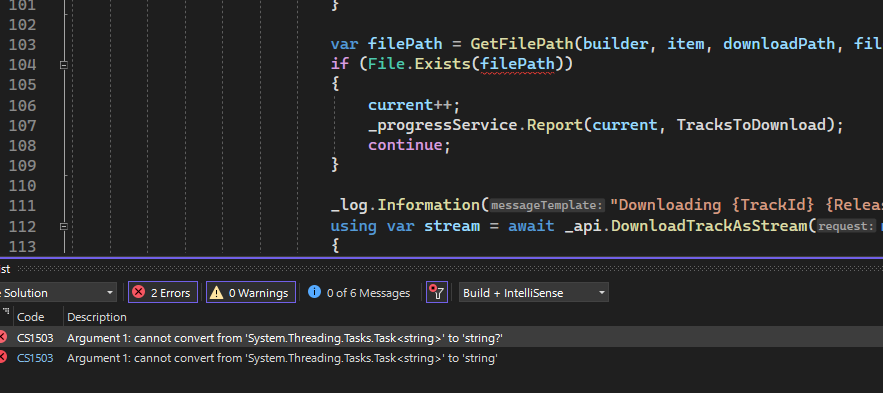
There you have it
all because of the damn Task<string>
Well, just
await
it
If a method is async, await it
await GetFilePath(...)

for fuck sake
ALL I WANTED WAS A DAMN IMAGE
:*(
What's wrong now?
see the squiggly?
Yes, but I don't see the error message
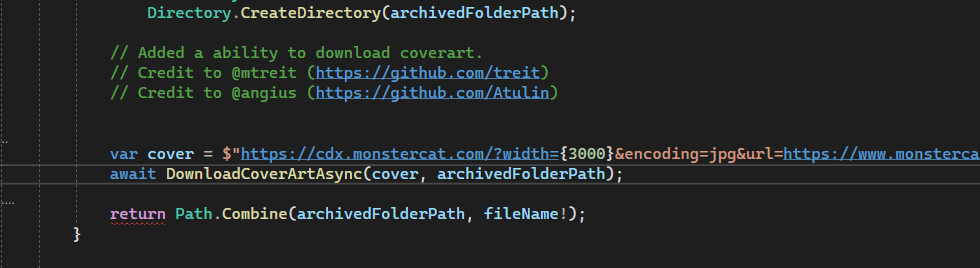
THIS IS FUCKED
it was compiling fine before all of this
Do you want help or do you just need to vent?
If it's the former, provide error messages
If it's the latter, let me know so I can go do some other things
sorry


this
Well, your method returns a
Task
it seems
void
-> Task
T
-> Task<T>
i will repost code
since we changed 100 things
Right now the method is akin to
void
, but you're trying to return a string
from it
latest
It is exactly as I said

yes i NEED the string in order to do the file saving
So... return a
Task<string>
Not a Task
oh
so put return Task<string> Path.Combine(archivedFolderPath, fileName!); ?
No
if I do Task<string> it breaks the entire app
Change the return type of this method
this is not m,y app
From
Task
to Task<string>
ok
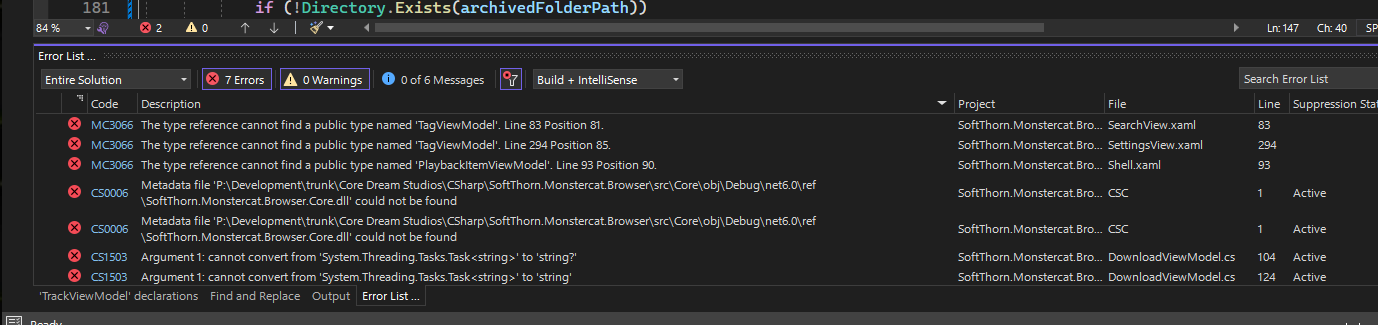
ok well im just gonna remove the ability for cover art
im not even getting paid
š¦
everything is broken when I use Task<string>?
Well, the last two errors are obvious at least
Wherever you call this method, you don't await it
Asynchronous code is "infectious"
i dont even need it awaited/async
its ONE image
Once something is
async
, the entire calling chain needs to be async
all the way down to Main
im not downloading wikipedia here
Just use synchronous versions of those methods then ĀÆ\_(ć)_/ĀÆ
HttpClient
should have a synchronous GetStream
methodnope, ilooked
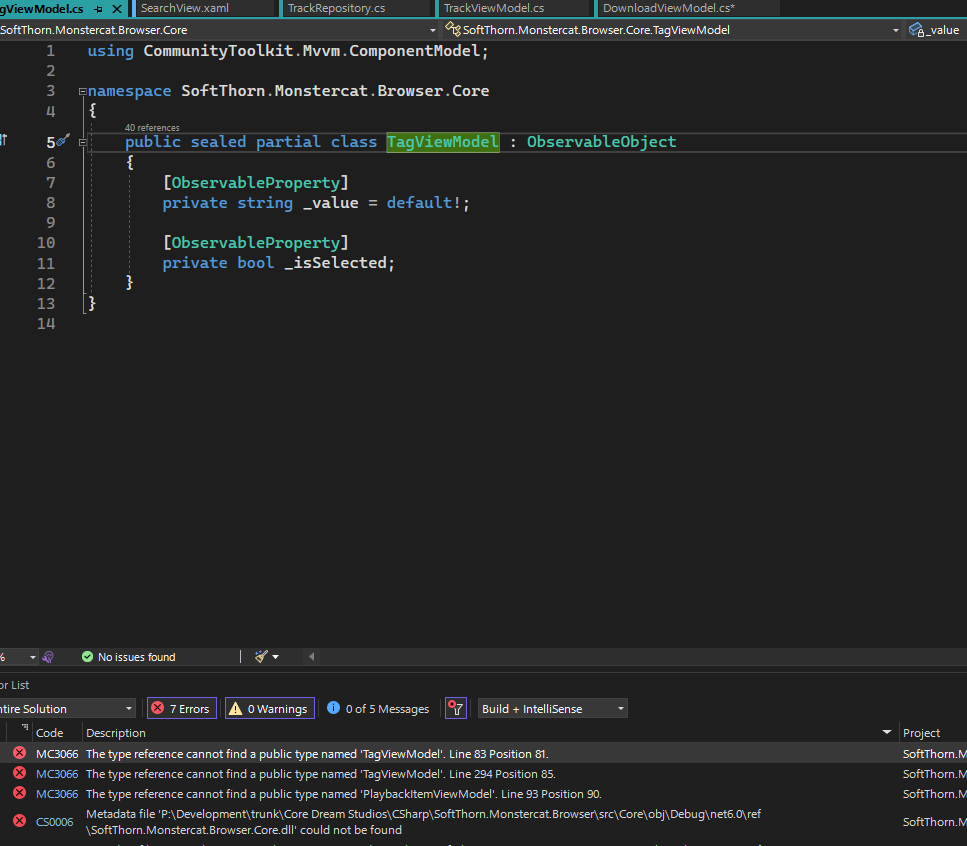
somehow that goddamn code broke this too
and i dont know mvvm so im not touching this
im just removing cover download
they can add their own code
its call gitHUB for a reason
You want a synchronous version of downloading a file with HttpClient?
yes š¦
i appreciate it
Here you go
oh
didn't know you could use HttpRequestMessage
gonna add that for homework
I need some air, letting my BP control my emotions heh
I appreciate the non async version though
if it was my project, id do it that way but this was someone elses
hug @ZZZZZZZZZZZZZZZZZZZZZZZZZ and @mtreit
i feel better now, took some water too heh
i need to relax and try to google some more too
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.