❔ EF Core: Owned property with converter expression cannot be converted
Hey guys, we're having this issue.
ShoppingCart is an owned entity and Items is a List of objects, which has a specified converters.
No idea what could be causing this.
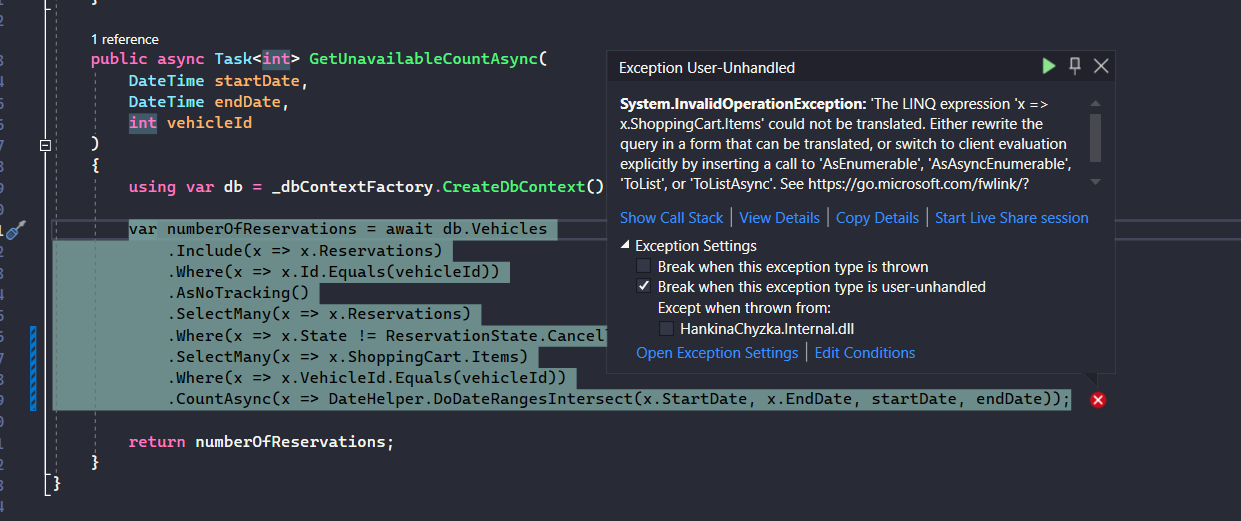
11 Replies
It isn't able to convert
x => DoDateRangesIntersect(...
to SQL
If you can make a
You can try making an
and pass it like
then it might translate it to SQL
what is the content of DoDateRangesIntersect?
are you familiar with what an Expression is? It's sort of one level higher than code.
A normal Func<> would be like
This becomes like a method you can call, it's almost the same as
an Expression however, is kind of code before it's turned into code.
this variable is of type expression which means you can call .Compile()
to get the Func
but more importantly, you can inspect it.
If you inspect this, it will say, this expression is a lambda expression with a variable and a body
when you inspect the body, it will say it's a return expression which returns the value of a binary expression, the binary expression is made up of x
, the multiply operation and 2
.
Using this power, you can write "code" which Entity Framework will inspect. It recognizes that if you have a binary expression with multiply, it can turn that into SQL
So only if you turn DoDateRangesIntersect
from a "normal function" into an expression, can Entity Framework inspect what the expression is made of, and translate that to SQL
another example, the
this secretly is creating an expression, even though it looks like code.
EF will see, a .Where
and will inspect whether it can turn the expression into SQL.
It encounters an EqualsExpression
which it knows it can turn into
Then it sees the left side is a MemberExpression
which accesses the VehicleId
property from x
. It knows it can translate that like
and the right side is a VariableExpression
and it knows it can do that by introducing a SQL parameter
it's kind of magic, but really coolUnrelated to the problem, but do you have example of how something like that is actually inspected and parsed like that? Expressions is one of those things I never wrapped my head around.
This is kind of an example from the EF code itself
https://github.com/dotnet/efcore/blob/main/src/EFCore.SqlServer/Query/Internal/SqlServerQuerySqlGenerator.cs#L115
It inspects a custom made SelectExpression, and adds to the SQL query depending on the structure of the expression
GitHub
efcore/src/EFCore.SqlServer/Query/Internal/SqlServerQuerySqlGenerat...
EF Core is a modern object-database mapper for .NET. It supports LINQ queries, change tracking, updates, and schema migrations. - dotnet/efcore
Notably
@rese7948 we can help turning your method into an expression :) if you want to share it
@tvde1 thanks for the effort provided into your response 🙏 , but I'm familiar with expressions. Don't mind sharing the contents of the method (will do in the next message), but from what I understand, EF Core already converts some expressions as lambda expressions that are then translated into SQL as you've mentioned and I've already used this function in an EF Core query with no issues before.
Additionally, if that were the issue, wouldn't it point to that part as the problematic one instead of simply the x => x.ShoppingCart.Items lambda?
as you can see, they're all functions, but they're essentially just simple expressions
and as I mentioned, they did work in the past with EF Core queries just fine
How far do you get with
You might need to inline
IsWithinRange
I'll try it out once I'm home from work, but why would this be an issue if the error states it's elsewhere?
oh that's true, the ShoppingCart.Items is the issue. What kind of property is that?
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Sorry for the lack of response, I had my plate full. Putting the main issue of this thread aside for a second, I actually did find out the IsWithinRange function is actually not translatable by EF Core for some reason? Even though when I simply extract it's code, it seems to work just fine.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.