Nested joins / where on relation
Hey guys! I have a question regarding filtering a query. Basically, I need to filter the query by
order
relation - order.date
is the field I want to filter by. However, I've noticed that filtering by relations is not yet supported. How do I write the query with joins? I can't figure out how to created nested joins (subOrders -> order
and subOrders -> product -> kitchens -> kitchen
etc.)
This is my query:
46 Replies
I tried with something like
Jesus Christ That's a block
Give me a sec
Wait what? filtering by relations should work, no?
Yeah, it definitely does...
It does? I want to be able to do something that works like that:
of course this thing does not work ^ and I don't know how to implement it
also, there's no
where
on the with.order
object - it's a parent-child relation in one-to-many, not the reversePreviously with RQB you could filter by a relation one level deep.
If I’m not mistaken, this feature was removed in the latest update - to help with typescript performance.
AFAIK this is achievable with the regular query builder, I suggest you search for other similar questions here.
Thanks for help!
could you send me a link to the thread? I cannot find it...
Also, if I would be able to filter with the regular qb - how to create nested joins? As you can see I have to have a lot of layers of relations and I can't find an example using the same with the .select() api
Well tbh I almost never touched the regular qb for select ops.
As for the thread, search for comments posted by me you’ll find it in no time.
ok thanks 🙂
You can definitely filter within relations in 28.1 (Which I think is latest)
@peterpkp123 From the docs:
It’s outdated.
See here https://discord.com/channels/1043890932593987624/1052239146699210863/1137795951491289110
I have used it today tho, it definitely works...
It works in a very wierd way - if the
with
object contains many children it works, if it's a parent in one-to-many relation it does notJust verified. it works
Well, if it's a one-to-one the parent has an "assigned" relation so I can see why it wouldn't
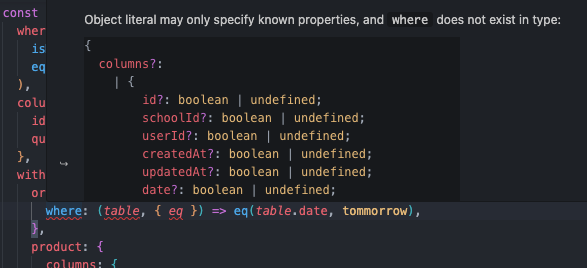
it's a one-to-many though
not one-to-one
Yeah, but you wrote the
or
incorrectlyit's
order
, just covered by the error message hahaOh kekw
Could you show me the code without the error message?
Here you go, the entire block once again:
Ok that’s weird. Are you sure you’re on the latest version? Maybe I misinterpreted the release note 🤷♂️
Really weird that that errors out for you, as this code is currently working for me with no problems:
Oh, can you even add
order
where you did? Could you try doing order: true
and see if it still yells at you?
I think it's potentially a schema/relations issueshouldnt
be
you need the correct table name
this works, and your example also works for me - sent and received are arrays and not single objects
im filtering by a nested relation too, in 0.28.1
nah it also fails, it's just a function arg
if the relation is an entity not entity[] it does not have filters i think
i think the notes are on about the top level
where
using a related table column maybe?My
package.json
:
So, are you sure you're using a one-to-many?yep i'm sure, it works without the filtering as expected
So what's this about single objects, I'm kinda confused
When you use
order: true
, does it return an object or an array of objects?
Because if it's an array it shouldn't be a problem...
Could you maybe show me the relation setup?an object and that's the problem
cause it looks like you can filter by arrays but not objects
Yeah
Because a "where" goes over shit, and when you only have one object there's not much to go over.
Just add a line checking it after you get everything from the db\
like technically it works, i have it like that, but it means i basically have to download much more stuff from the db than i would normally do
even on my test dev db i'm getting 20 entities instead of4
for now it's not THAT important but would be nice to have some sort of filtering
Wait what, it seems like order is its own thing no?
Like, products and shit aren't in order so it shouldn't matter that much
i have orders and suborders, basically meaning that one suborder - one type of product
order is a group of suborders for a selected day and i want to get only suborders that would be part of an order for JUST the next day
so yeah, it means a lot actually
actually i can do it in a really stupid way thinking about it
So I guess what I'm ordering is why this would be structured this way? You could 100% Just query for orders where the date is the next day and then just go from there
i can make a query to orders table instead and merge the array afterwards
Like, why are you querying from
suborders
when you could query from orders
yeah
just realised lmao
Yeah, that other way is just... wrong
i just need suborders as the result but i can do it in code
Well, that's a different issue
Also, the initial way would mean if you had 2 orders for the next day you'd only get 1 at most....
Like, there's a good reason why
where
doesn't work from the one side. There's just always a better way...well i had it in prisma written exactly like that and it worked... maybe something weird under the hood tho
not sure
thanks for help though!
Definitely something weird under the hood! Since
suborder.order
could only refer to one order
, in theory you should only be querying that one for the day
Anytime!This is my Prisma code if you're interested 😉
@yuval59 there’s something off with your setup. Drizzle has indeed deprecated this feature.
You’re welcome to see for yourself:
https://codesandbox.io/p/sandbox/beautiful-tharp-8z4s98?file=%2Flib%2Fdrizzle.ts%3A14%2C4
beautiful-tharp-8z4s98
CodeSandbox brings instant cloud development environments that keep you in flow.
.
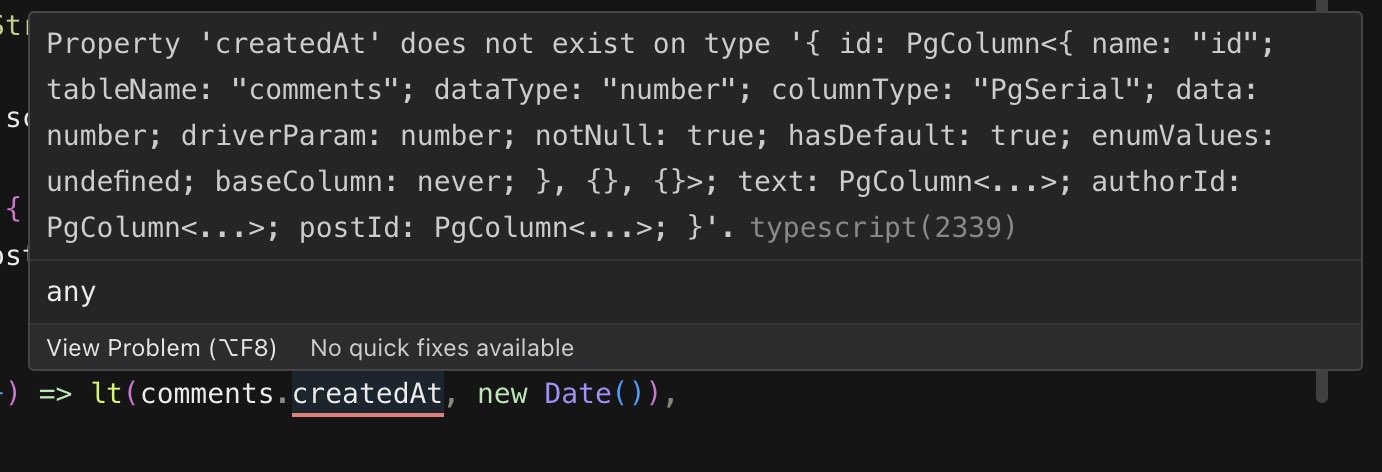
@mendy.l odd, i am able to do this in 0.28.1
this 100% works
with mysql that is
The change states this code won't work
its the top level "where" using a related table column value that shouldn't work, nothing about "where" clauses in the nested table joins