Display eloquent data in custom widget
How can I display User::count in a custom widget thats in the Dashboard? I cant seem to get it right, as I get Constant expression contains invalid operations error:
23 Replies
That's right, you can't initialize class properties with function calls, you can do it in the
mount()
method instead
And then what about the blade file?
You'll need to modify the built-in view I think:
Great! My only issue now is that polling doesnt work. Is there any reason for that?
So, I have a widget on the Dashboard and I want to display the number of users
And when I create a new user, the widget doesnt update
Yeah, I don't think the base
Widget
class has pollingYou may be able to add
wire:poll
directly in your template instead :
https://livewire.laravel.com/docs/pollingLaravel
Polling | Laravel
A full-stack framework for Laravel that takes the pain out of building dynamic UIs.
Oh but then I think you'll have issues with
mount()
, it runs only once when the page is initialized
Try boot()
instead if you run into any issuesI dont get any errors
There is just no polling
Yes, that's because you are using the base
Widget
class. It doesn't have polling like the stats widgets.I tried with Stats Widget, but nothing
still no polling
Please check the link I shared 👆
You can add polling yourself with a Livewire directive
I did, but then I have an issue with render() in filament
Declaration of App\Filament\App\Widgets\UsersCount::render() must be compatible with Filament\Widgets\Widget::render(): Illuminate\Contracts\View\View
Sorry but the solution is in the error message
Your
render()
method must match the one in Widget
(the return value)I see, all I had to do was add
: View
It works now. Thank you! Will check the extended classes carefully next time
Solution:
No worries, it trips me up too when I'm using vscode... (phpstorm has a feature that does it for you automatically)
Uhhh, which one 😅
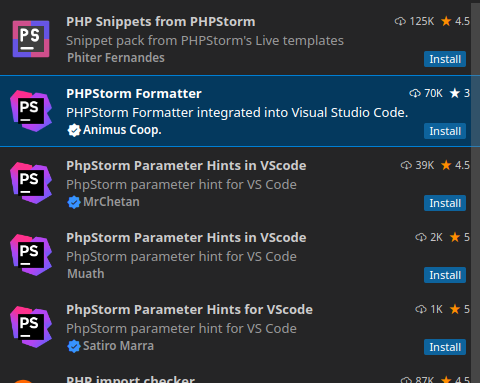
I assume the first one with the most downloads?
oooo its an IDE
Somehow all JetBrain IDE's look identical
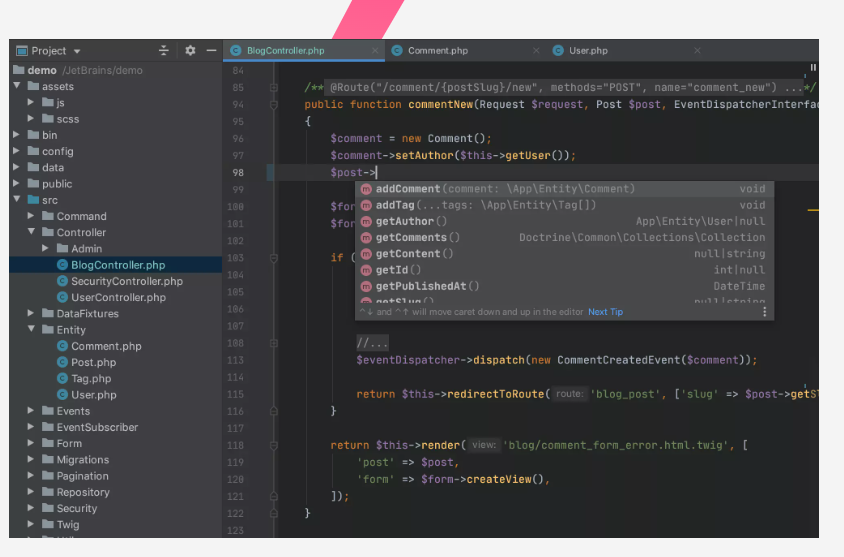
They work really well, but they just look ugly :/
Yeah I think they all use the same UI