ReferenceError: Cannot access 'addon' before initialization
This is one of the files in ./schema/
When I run
npx drizzle-kit generate:sqlite
I get the error:
And I don't understand why since the schema doesn't have any self references22 Replies
Any good way of debugging this yet?
The error mentions a file
.../schema/addon.ts
What's in that file?it's simple
problem probably is here
because you use a alias try change it into
relative path
___
sorry for my English but i try learn it
Same issue here. Can somebody from drizzle team please comment? Appears to be a circular reference problem that breaks queries and drizzle-kit push, etc. I'm working around the issue by putting all my schema into the same file, but this is less than ideal, naturally. I'd create a repro but I've already lost my entire evening to this (but will do so if needed. just lmk). thx!
Filed issue here ... https://github.com/drizzle-team/drizzle-orm/issues/1308
GitHub
[BUG]: Relations circular reference breaks query and drizzle-kit pu...
What version of drizzle-orm are you using? 0.28.6 What version of drizzle-kit are you using? 0.19.13 Describe the Bug Query relations involving multiple tables (i.e., .schema files) where circular ...
Again, for anybody running into this issue, adding all my schema and relations into single file resolved the issue for me.
This issue is due to circular circular dependencies. For me I had an enum which was imported into one file and then another, so I just extracted it to its own file which cleared it up
A repro repo will come a long way. It's very likely an issue on your imports and an actual circular dependency
I'll work on a repro. I previously (before filing ticket) double and triple checked for an circ deps on my end and the only ones I could find were the ones that the drizzle schema and relatiions declarations require me to introduce.
Here is a repo repro'ing the issue -- https://github.com/rolanday/drizzle-circ-dep
It's a solid-start project, but not important. Just run the package.json
db:push
script and you'll see output below. I didn't bother working up a query because I've observed that whenever the push command fails so will the runtime query.
GitHub
GitHub - rolanday/drizzle-circ-dep
Contribute to rolanday/drizzle-circ-dep development by creating an account on GitHub.
Sounds good, I'll check it out if I have some time today
Thank you @Angelelz . Btw, the repro does indeed have circ deps, but these are artifacts of expressing relations between schema in multiple files. Very possible I'm going about it the wrong way, but not seeing any other way short of expressing expressing all in a single file (my current workaround).
Alright let me take a look
You definitely have circular dependencies
The solution it's pretty simple
I'm all ears
Just remove the relations from the user schema file, and create a new file just for the relations
I tried that, but then the relations don't seem to get picked up when I build query
i'll try again, but is there any special convention I need to follow? Or idea is I should be able to express relations in sidecar files along side my schema (just like what you have ^^).
Btw, ty for taking time to look into this. I'll go ahead and give another try breaking out the relations into separate file(s), but wasn't working for me last night.
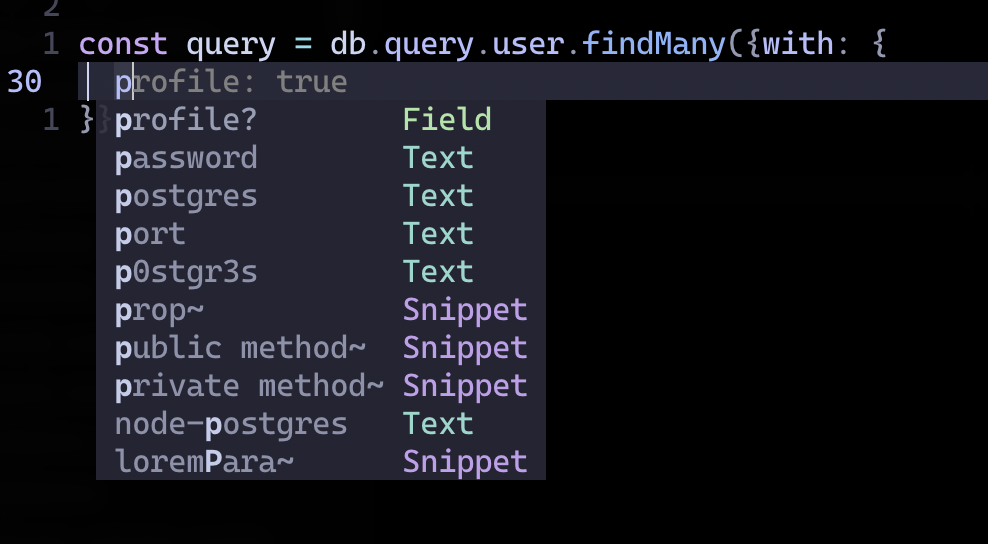
You create an index.ts file that looks like this:
This is what your db file should look like:
And it will infer properly
I pushed all my changes to a fork: https://github.com/Angelelz/drizzle-circ-dep
GitHub
GitHub - Angelelz/drizzle-circ-dep: correction repo
correction repo. Contribute to Angelelz/drizzle-circ-dep development by creating an account on GitHub.
I'll take a look now. ty for this!
Hi @Angelelz , got to the bottom of the issue with your help. When I created my relations to support query (after creating my table schemas), I didn't go back and add
relations.schema.ts
to the list of barrel exports. (Like I mentioned, I originally had them factored out this way.) This in-turn lead me to incorrectly believe I needed to keep the relations in same file as user.schema
, which admittedly made no sense to me, but it worked -- from perspective of being able to type out the query in VS -- in effect sending me down the wrong path.
Apologies for eating up your time on this, and I much appreciate! Hopefully helps somebody down the road who runs into similar.
Thanks again!! You rock.Wanted to confirm the above advice worked. Thank you @hotshoe for the further questions, really saved me a lot of time. And thanks to Angelelz for answering!
It helped me to solve it