Help me not to give up on t3.
I've been trying to implement optimistic updates on my app but i'm getting a lot of errors.
I started by following the react query docs and tried implementing it like this
but i keep getting this error
then @brendonovich adviced i used trpc helper wrapper functions instead of the react query queryClient directly.
i then did this with the trpc helper functions
but i keep getting this error
i really need help because im junior but dont want to give up on trpc yet. if anybody needs my repo link, i can provide it please.
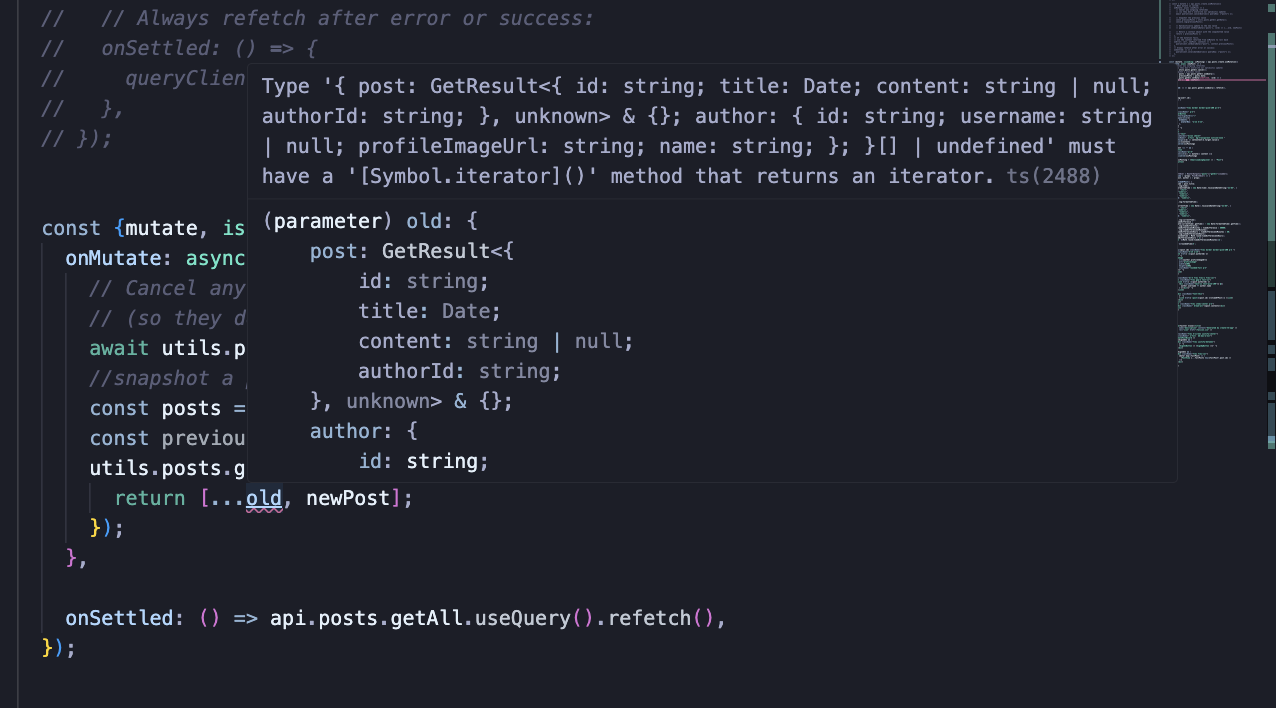
2 Replies
Why would you manually set data to cache?
In your place, I would have waited until mutation is successful, then:
await utils.posts.getAll.invalidate()
this is causing a weird layout shift so i decided on optimistic updates