DT
Drizzle Teamfermentfan
Mocking Drizzle instance
Hey there, I am currently trying to find a good way to mock our drizzle instance for our unit test suite, but the nature of the usage of it makes it kinda hard. Is there somebody who is running a code base with a mocked drizzle?
fermentfan•289d ago
@bloberenober @Andrew Sherman Any idea? 😦
Andrii Sherman•269d ago
Sorry for a late response, finally have free time to answer all questions in Discord
https://discord.com/channels/1043890932593987624/1101108758127591435/1139240309939777719
I'll answer this question anyway
We don't have mocks for drizzle. How do you want to use it?
And why do you need to mock database for tests at all?
andrevandal•213d ago
hey @Andrew Sherman I have the same scenario here, I'm testing my repositories and I'm creating an unit test and I need to mock it hehe
bloberenober•212d ago
why not use something like sinon to stub/mock specific methods on the db?
or if you are using vitest it has its own mocking API I think
there's nothing specific to Drizzle that can't be mocked like anything else
andrevandal•212d ago
I've tried to mock using vitest but I'm facing some errors
have you already use vitest to mock drizzle?
jakeleventhal•129d ago
Then add this to your jest config:
And here is a sample test
jakeleventhal•129d ago
youre welcome
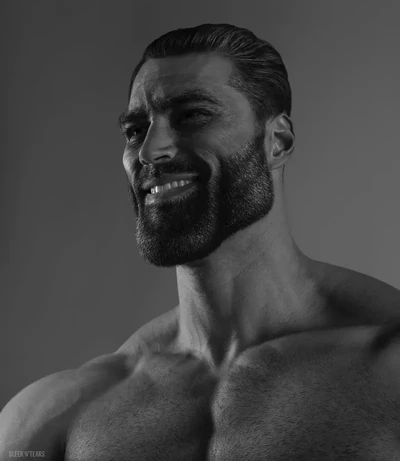
collinbxx•121d ago
@jakeleventhal How can I mock with select, insert and update?
jakeleventhal•121d ago
what i eneded up doing is basically using very few of those drizzle mock tests
IMO, any test like that is kind of weak. its much better to either do an integration test or dont make those checks entirely
but if you really wanted to you can just extend the return types of each of those funcs
collinbxx•121d ago
I have a User creation function like this that uses the returning() function. I want to mock test it but don't know what to pass in below.
KHRM•28d ago
did you ever figure this out?
it gives me issues when im not using the query method

The official Discord for all Drizzle related projects, such as Drizzle ORM, Drizzle Kit, Drizzle Studio and more!
7.4KMembers
View on DiscordWant results from more Discord servers?
More PostsHow to run a Cleanup ScriptI saw someone provide a nice script to empty the database, here's a version of it:
```typescript
aAdd conditions on relationshipsIs it possible to add conditions when declaring relationships?
In my example, I have books that havhow is remove nested relation name "collection". Used many-to-many relationship.request:
await db.query.products.findMany({
with: {
collections: {
I tried date format in the where clause it showing me an error.this is the query im converting
SELECT *, (SELECT COUNT(*) from `daily_check` WHERE (`daily_checks`groupby multiple thingsthe SQL query I want to convert ends with: ```GROUP BY
u.user_id,
e.ExerciseID;``` I see no whUnwrap the return type of a Subquery, native jsonAgg support or RQ Join Names?Hi There,
I am currently investigating Drizzle ORM and seeing if I can bend it to do everything I Unform methods for result accessIs there a set of uniform methods for accessing the results of a query? Could be good to have somethCannot query DB table get `COALESCE types smallint and text cannot be matched`When i run the following query
```javascript
let tc = await db.query.ATable.findFirst({
where: Cannot Access Primary Key on TableHello, I am trying to access the primary key named `id` on the following table:
```ts
export const Typescript build fails, pointing to internal types.My typescript build fails with 4 errors, all of them pointing to internal type declarations. Is theronConflictDoNothing does not exist on planetscale clienti am getting an "Property onConflictDoNothing does not exist on type" on the following script....
`Are relational queries supported on mysql?I'm new to this ORM and I'm having trouble getting relational queries to work.
Normal db.select()Is there a way to write a query that orders and filters based on an array of possible options?I'm trying to do some server-side filtering and sorting for a table, and I ended up writing this forcannot set alias for composite primary key, getting (errno 1059) (sqlstate 42000) errorsMy table has the following schema ->
```ts
export const emergencyContactPhoneNumber = mysqlTable(
Help with query writingHi, I'm hoping someone will help me with this query. I have the following query in one of my serviceMySql NOW() in DrizzleHi! I might be struggling because this is hard to search for, but I'm wondering if it's possible to Postgres: install plugin during migrationSo, I'm using Drizzle with Postgres and am using uuid for the id fields.
For that, i need to run theIntrospect failing no pg_hba.conf entry for hostI'm trying to introspect an existing postgres db. It's hosted on heroku and I'm facing `no pg_hba.coIs there a way I can use relational types?I'm currently trying to make a helper function that essentially wraps a database call, but I can't sDifference in using unique() on the column definition vs the index?What is the different between using something like
`name: varchar('name', { length: 256 }).unique(