Specular/Normalmap with custom renderer
Hey, I wanted to ask something! This is my custom code for rendering OBJ meshes which works, with rethinking voxels it also recieves shadows, AO etc.
I wanted to ask, is it possible to also bind the specular and normal maps? Can the shader work with that and use it (for example for screen space reflections and normal maps)? :)
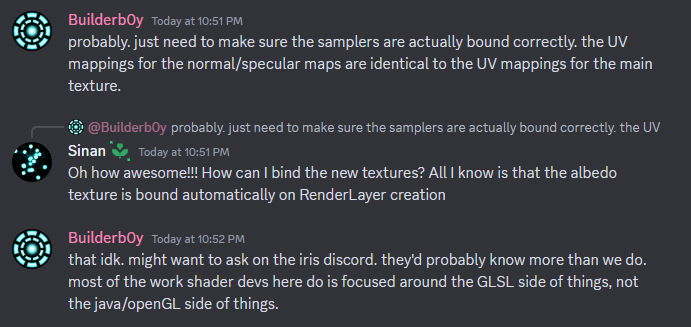
560 Replies
@IMS Hello, given your bio I thought I might ping you for this :)
Is it possible to bind the specular and normal maps alongside my rendering, aka do the shaderpack shaders have access to that?
sorry, didn't get to this
No problem, thank you for answering
The way this is supposed to work is you use AbstractTexture's, and then you can just have _s and _n variants of those textures
and Iris should just know how to use them
May I ask in which sense? In my code above I do not render like normal blocks or items
so first try adding textures/re45_s.png
any sense, it's caught at texture binding
not at blocks or entities
Yes, I have the _s and _n with new Identifier
Oh okay, I never heard about AbstractTextures, I am scrolling through the docs but I am unsure on how to use them
Nvm!
So basically:
I should do this before calling draw?
no
don't do anything with _s or _n
pretend they don't exist, just have them
Iris will grab them for you
if you're registering them, also don't do that
Oh uhm, I should just have them as files?
yep
Woah
I'm gonna give it a shot
Hmm that did not seem to work, I will provide you with my code:
First off I think this is what you meant?
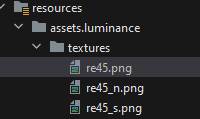
yep
This is my render code:
This is all I do basically
what is NewRenderLayer.getNewLayer?
And this is the renderlayer:
hm, ok
that should be making a simpletexture...
just to be sure; you're using a shader that actually uses pbr and you've tested it with packs?
Yes, I am using rethinking voxels and no, I have not tested it with packs actually
i don't believe rethinking voxels has pbr?
try it's non-voxel version, Complementary reimagined
Omg now that you say it
I had to switch PBR support to SEUSPBR
Omg I think its working
HOW BEAUTIFUL
I just need to convert the yellow normalmap to the purple standard and I can show it here
👍 yeah, try moving to labPBR if you can
Alright! Oh yes, I just read labPBR even has dielectric/metal support :o
yeah, quick note about that
some shaders support that, some don't
for example reimagined/rethinking voxels don't last I checked?
Oh okay! Still, that's so freaking awesome that Iris automatically loads that! I LOVE IRIS
no problem!
Hm, when I change from Integrated PBR+ to SEUSPBR or labsPBR, the whole lighting changes drastically. However, I really liked the Integrated PBR look...
Integrated
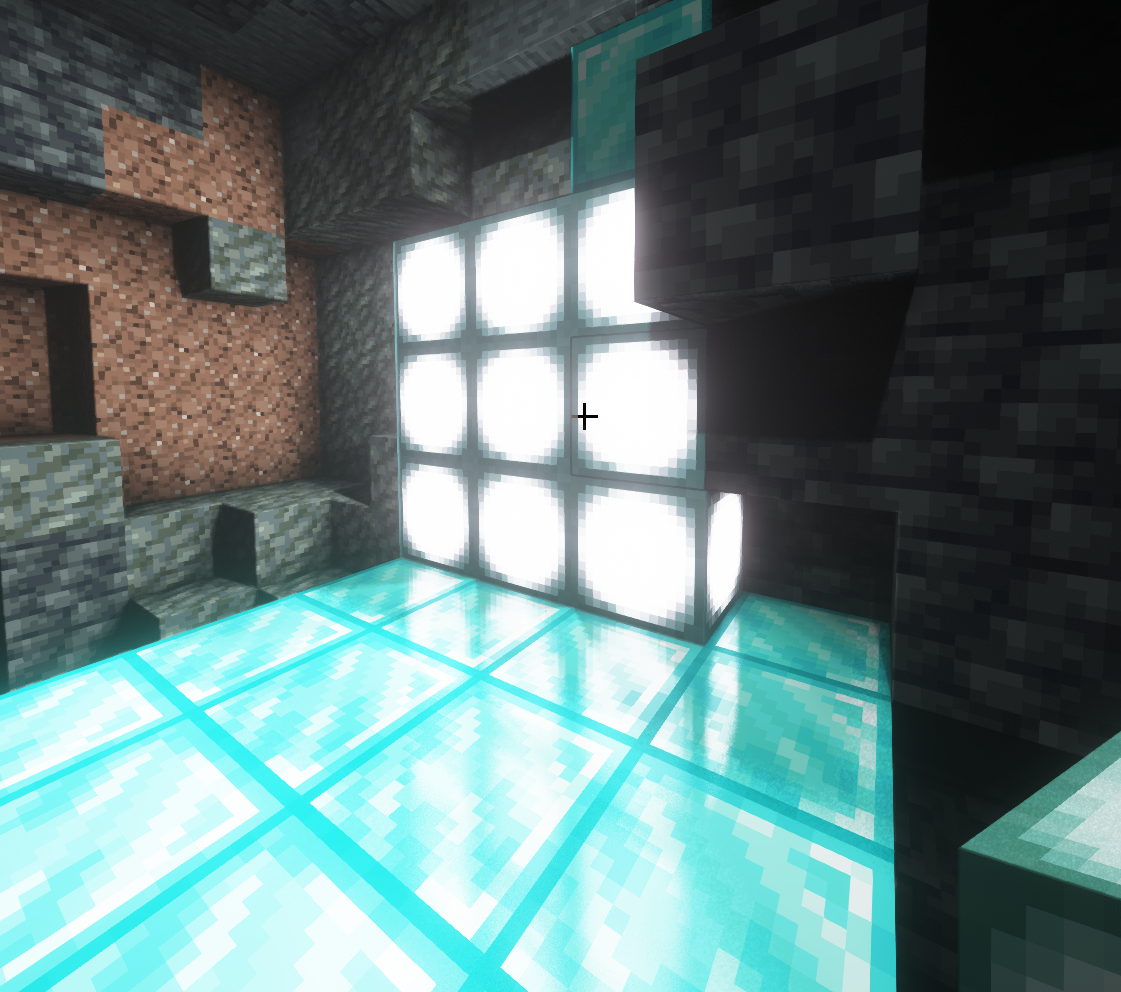
Integrated PBR does not allow any PBR textures
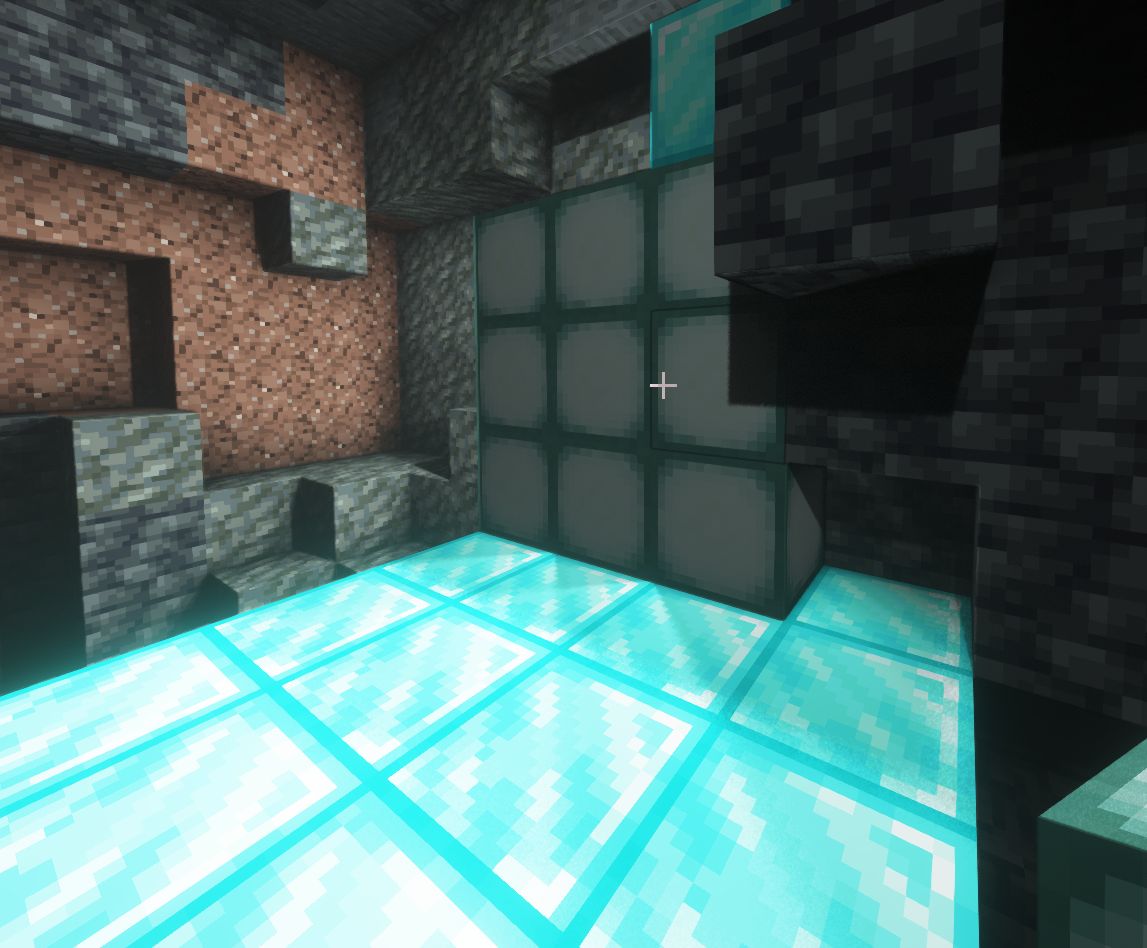
SEUSPBR/labPBR
it's PBR hardcoded to the shader
that doesn't look right... what pack is it?
oh wait you're on rethinking voxels
yeah seuspbr is broken there
Ah okay I see... Is there a way to get the hardcoded properties to a resourcepack?
try Complementary Reimagined
Yes
no
https://cdn.modrinth.com/data/HVnmMxH1/versions/RYvsFGd2/ComplementaryReimagined_r2.2.1.zip
use this one instead
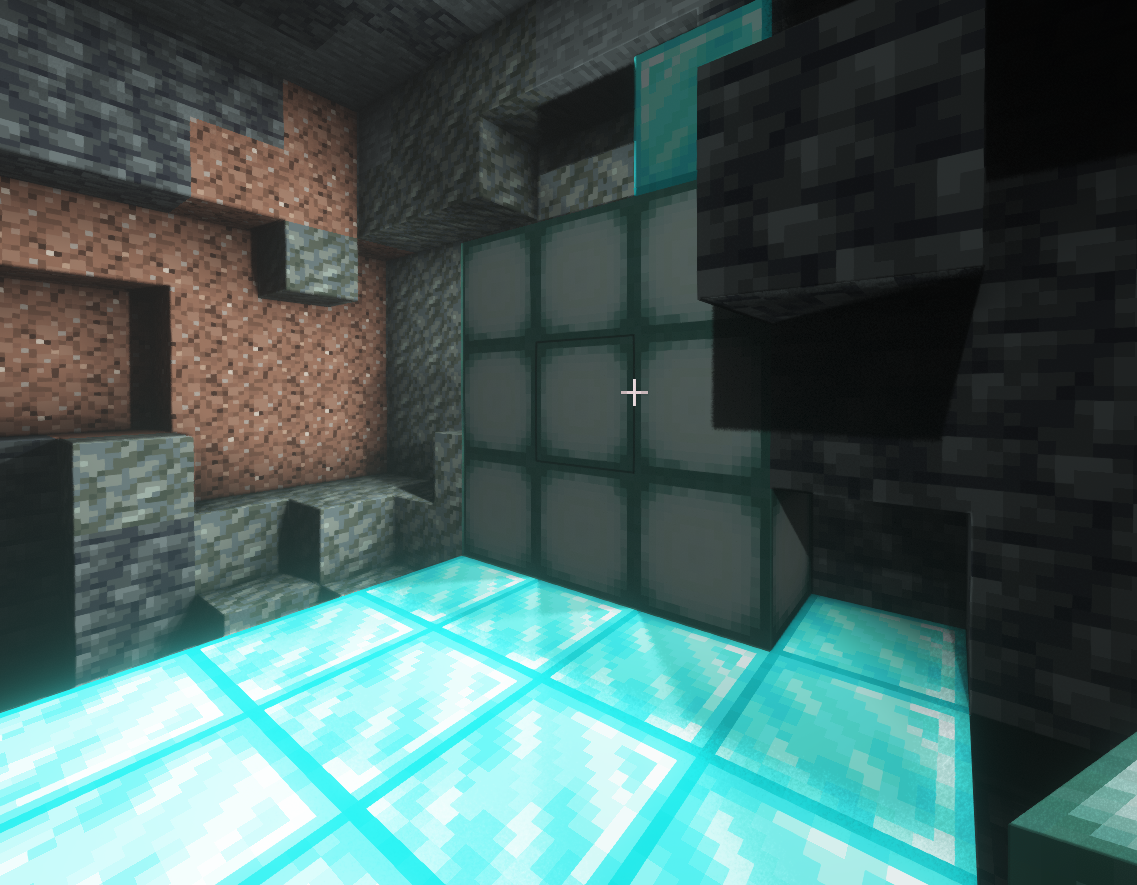
But this is labPBR :(
I really love rethinking voxels and would super like to stick with it
it's a bug in rethinking voxels
I will however try the other pack too
Rethinking Voxels is an edit of this other pack
this is the original
But without voxelization or how can I understand that?
it's without the ray traced shadows and lights, yeah
same look though
Ah that's unfortunate, I really fell in love with RV for exactly that
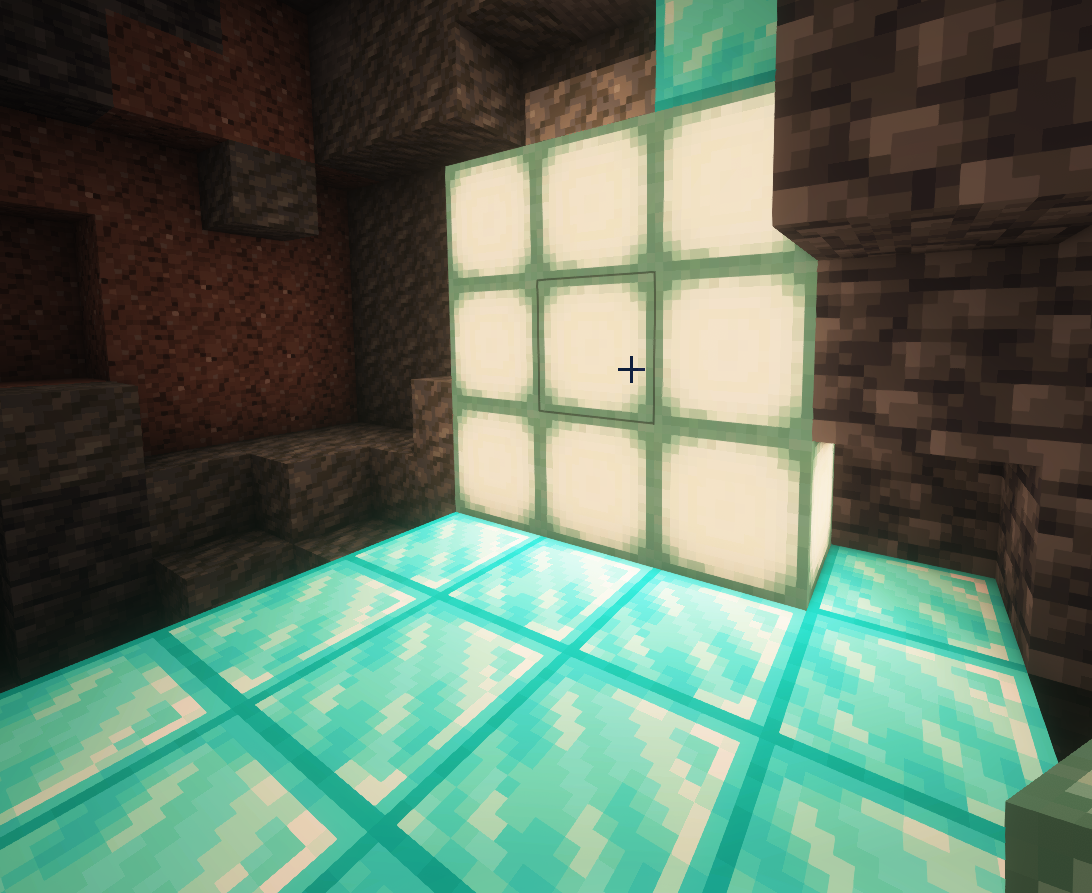
Okay, this is complementary with seuspbr and labpbr, looks the same, I guess because now it is relying on the non existent maps right?
yep, now add this
May I ask is there a way to get the hardcoded properties into a resource pack?
yes, one second
well partially
Ah thanks!
Hm, this does not look right
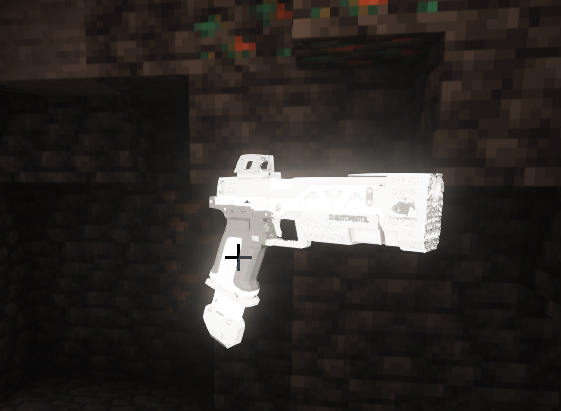
specular map is set to completely white and the normalmap looks like this:
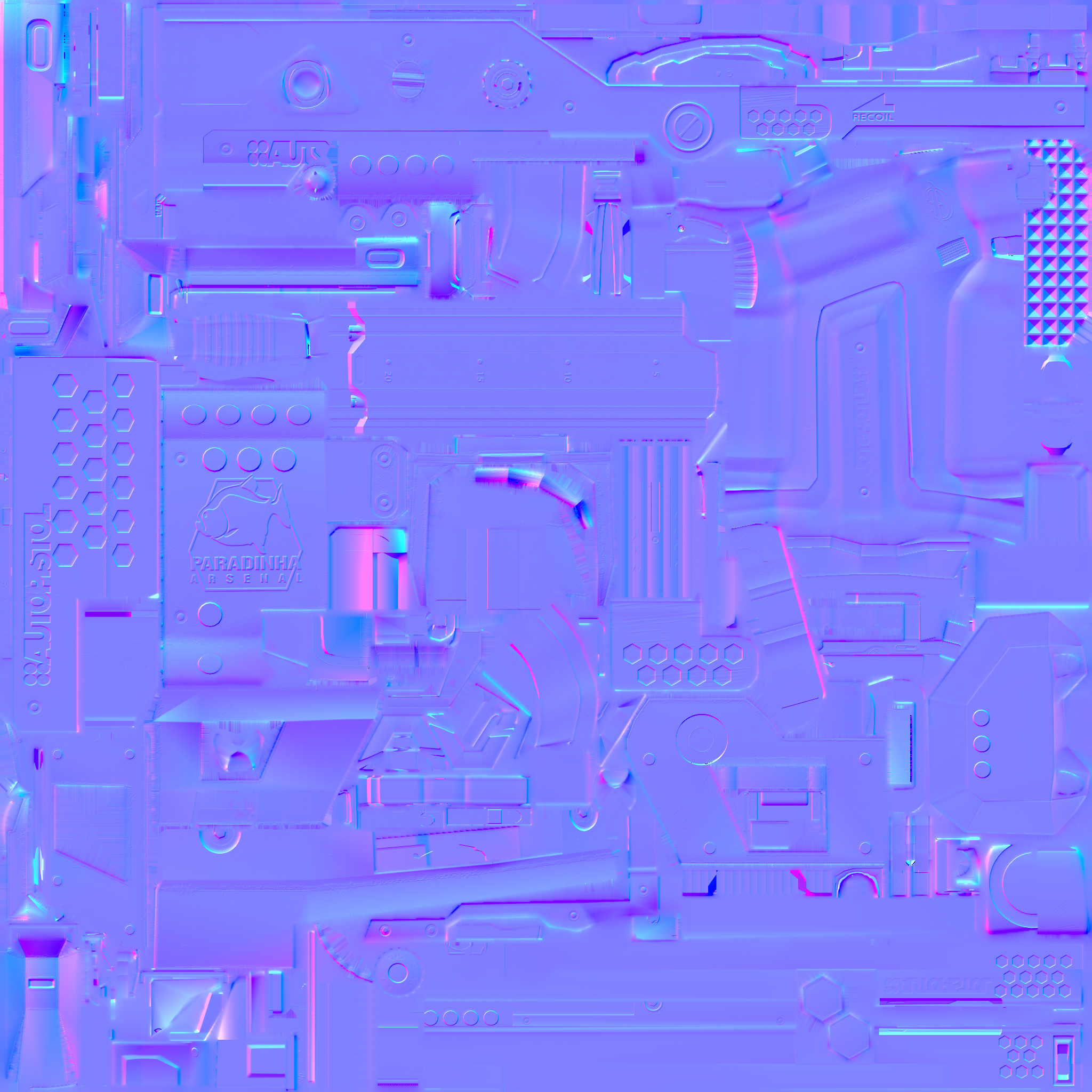
specular map should be black
white means you want everything hyper bright
I wanted to see highly reflective
then you need to properly change that iirc
I will now build the maps according to labPBR standard, brb
it's Vanilla Normals Renewed, a pack that recreates that same look iPBR gives to some extent
Thank you! Regarding the RV bug, do you know what is happening there? And is it just having trouble rendering emissive meshes or is it something more complex?
RV has never been able to fully do PBR to my knowledge, lemme see
Thanks for looking! 😊 I mean, isn't integrated PBR PBR? It looks hella good
what version of RV are you on?
The latest github master branch
I cloned it
Integrated PBR is PBR, but all the PBR is done in code
and not in textures
it's probably a rethinking voxels bug
May I ask the difference? For me (who doesn't know anything) it seems as if coding a PBR shader and then hardcoding the surface properties doesnt seem too far off of loading according textures
you get more fine grained control
there's no limit as to what pixel you're on or what the options are
Okay, with labPBR I get some pretty damn awesome results 😍
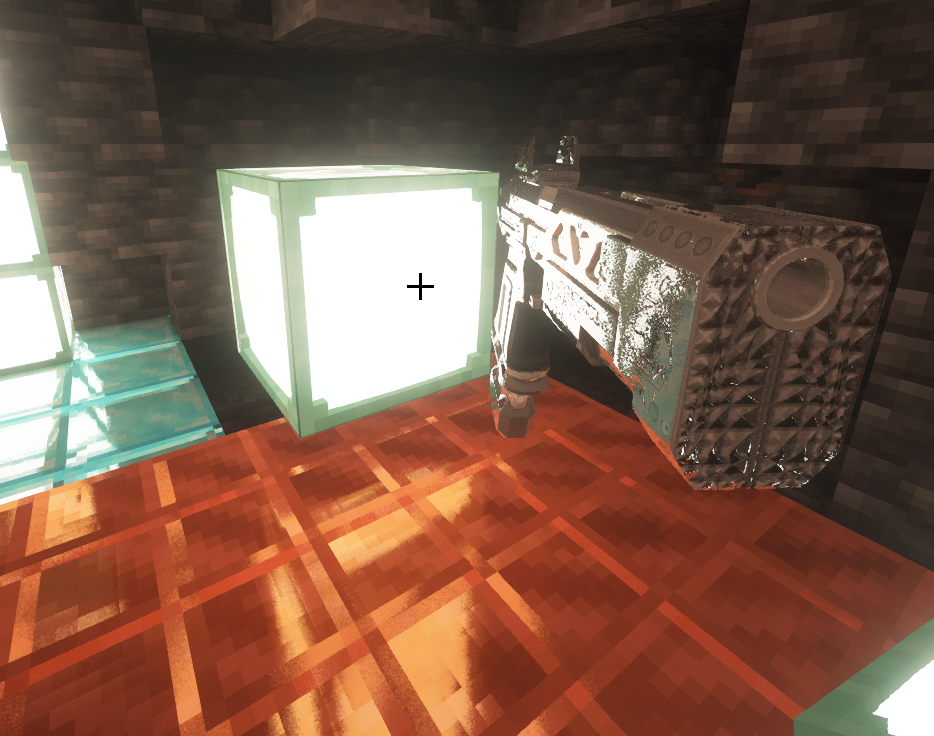
This is with a completely white specular map again
LabPBR Material Standard
This is a standard covering the material data storage using only two textures in resource packs, as well as the decoding done by shader packs. They can use this data for physically based rendering (PBR), but be aware that normal and specular textures themselves are not directly related to PBR in any way.
But labPBR suggests that a green channel with 255 is metal, however my object looks like a glossy dielectric
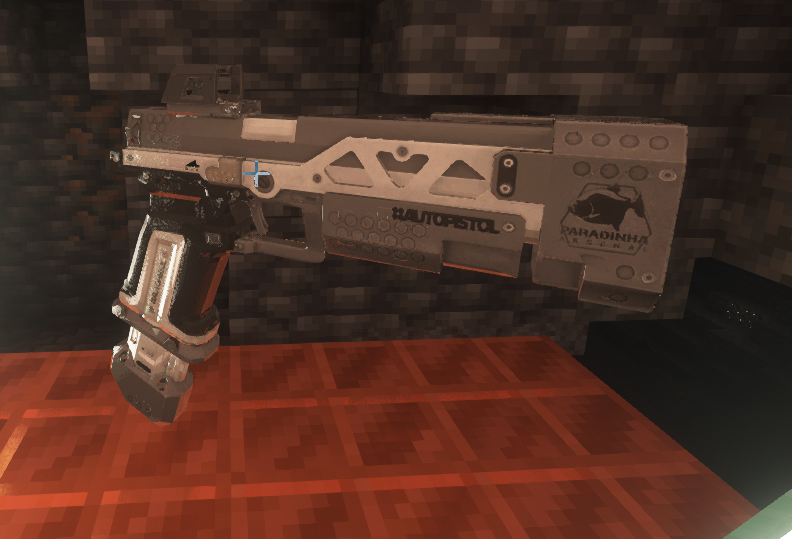
yep, use Kappa shaders to test those
Also hold up, RV works fine with labPBR, it was just that the default pack did not make the sea lanterns emissive
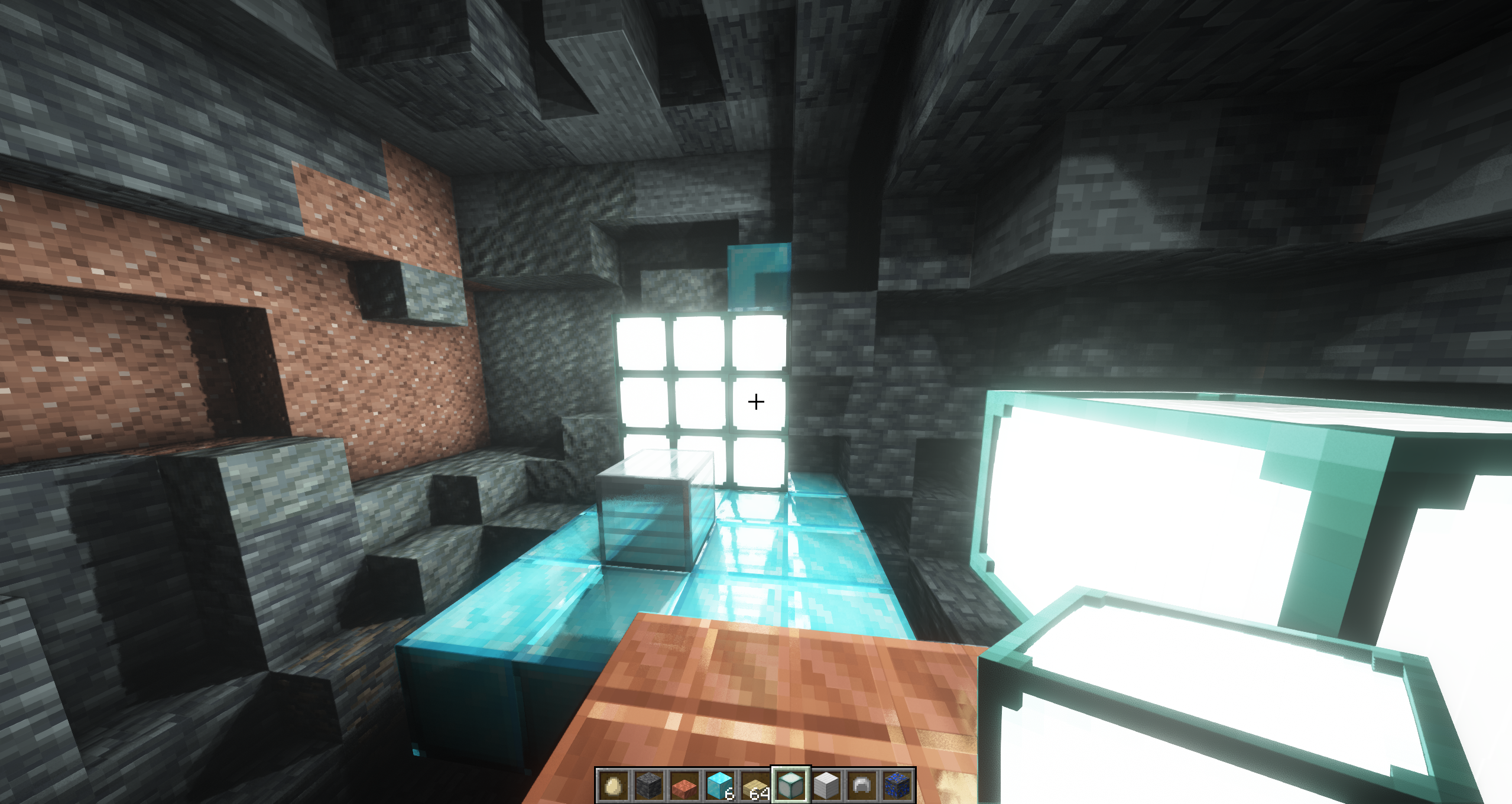
oh nice
weird
😍
I LOVE IT
okay this looks way more metallic I guess :Flushed_AE:
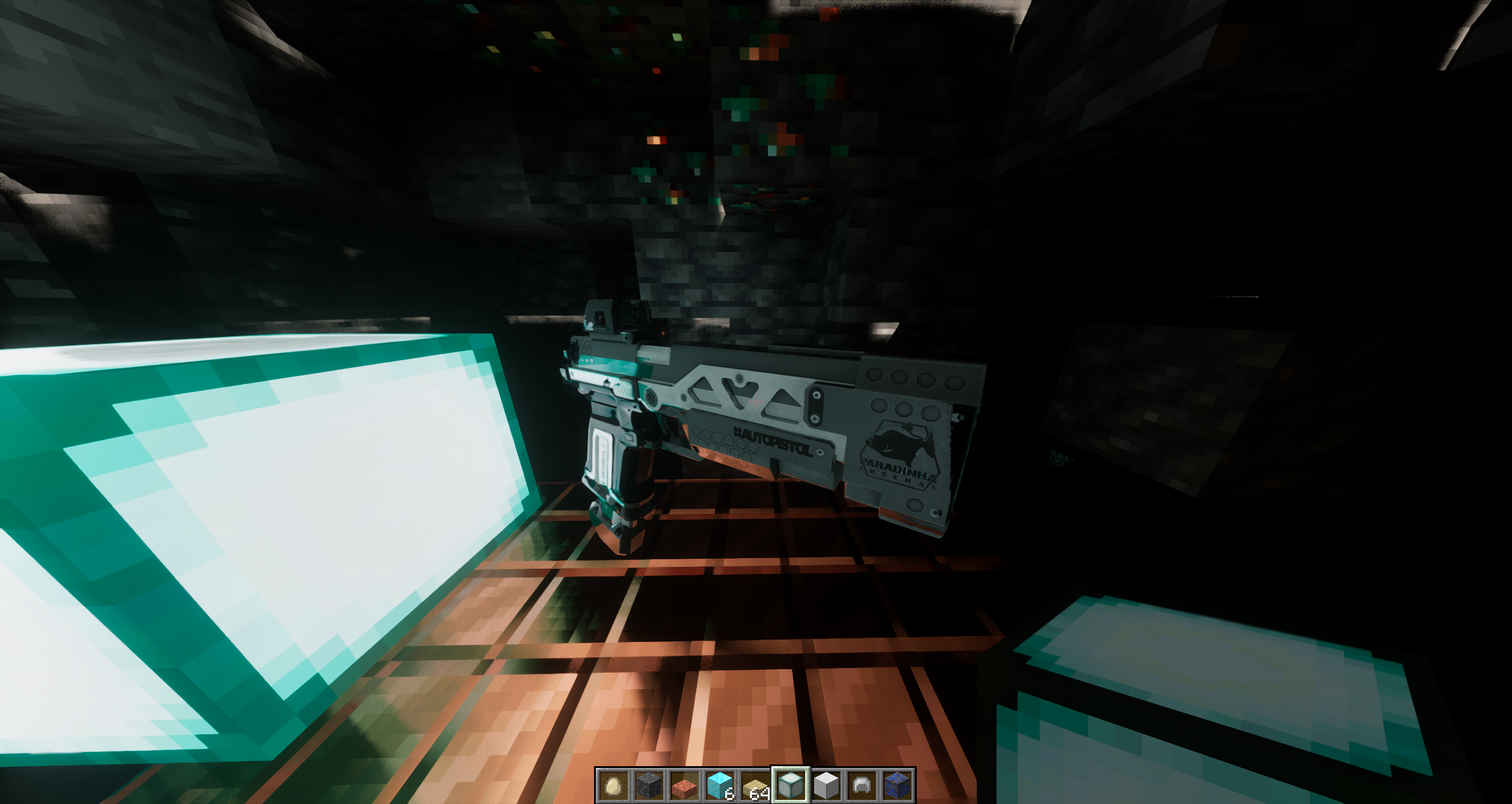
that's really nice
Thats SO NICE
So you are saying rethinking voxels does not support metallics atm?
complementary reimagined, the original, doesn't
iirc it's not planned
because it's too complex
Ah that's such a bummer
Before I thank you a million for everything, I have two final minor questions :)
This is Kappa's shaders:
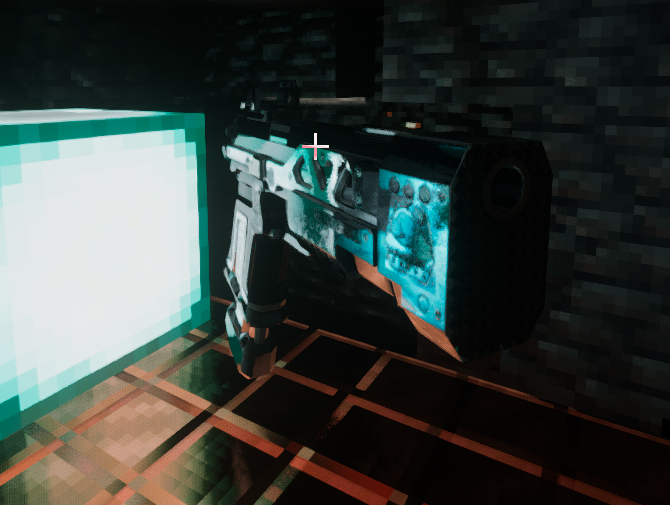
And this is rethinking voxels:
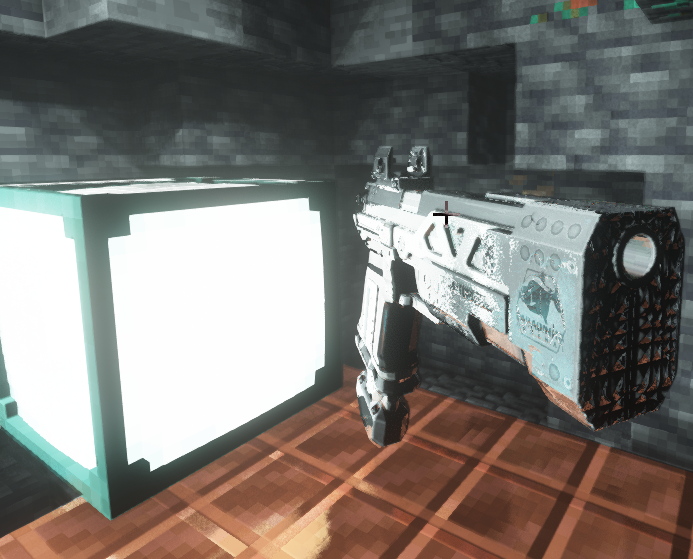
Is it just me or does Kappa seem to correctly map the normal map and rethinking voxels somehow fails?
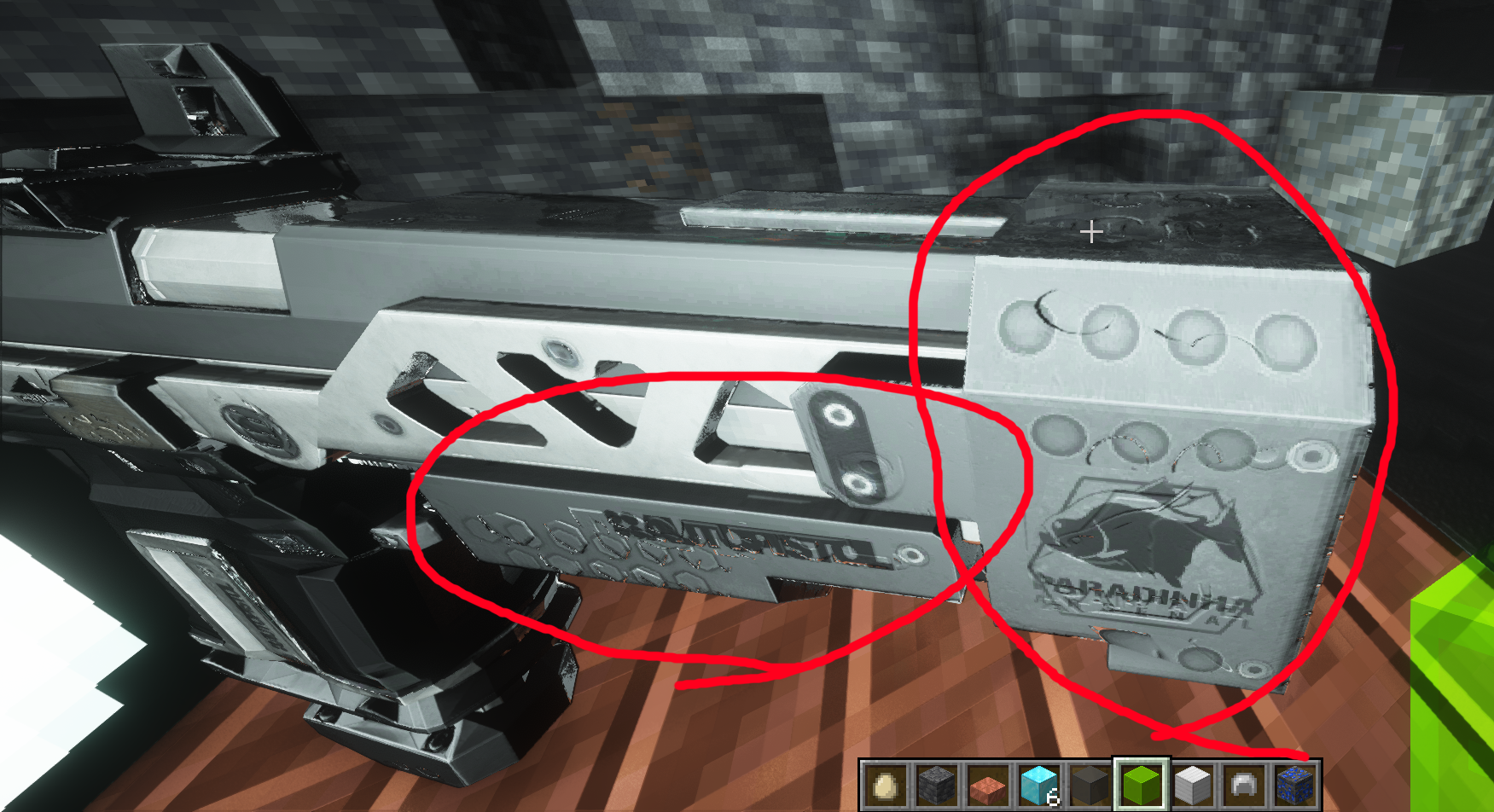
rethinking voxels: there is some UV mapping gone wrong, let me see if I can get the same result in Kappa
rethinking voxels doesn't have it as advanced
so i wouldn't doubt it
Yup, kappa is rendering it correctly
Hm, in general you mean? So their UV map code for normal maps is off but for the albedo texture not?
yeah
does it also happen on complementary reimagined?
if so, report it there
if not, report it to rethinking voxels
Huh, that should be easy to fix though right?
maybe, maybe not
Yeah it does actually
I will do a GitHub issue (or is there a better way?)
Ah great, thank you :)
Okay great, then my second question
How can I enable smooth shading for my built mesh? (I don't need smoothing groups, double vertices at sharp faces will suffice I think, the model comes with that)
you'd probably need to edit the mesh
unless you mean with shaders it's not smoothed
Actually now that you say it
Let me try one more mesh
But Kappa seems to be the all round winner, it seems to shade the mesh perfectly smooth
Again seems to be a Complementary 'issue'
kappa is one of the only shaders with 100% labPBR support, yeah
Arc is a very close second, if you want to look into that
No actually it also doesn't
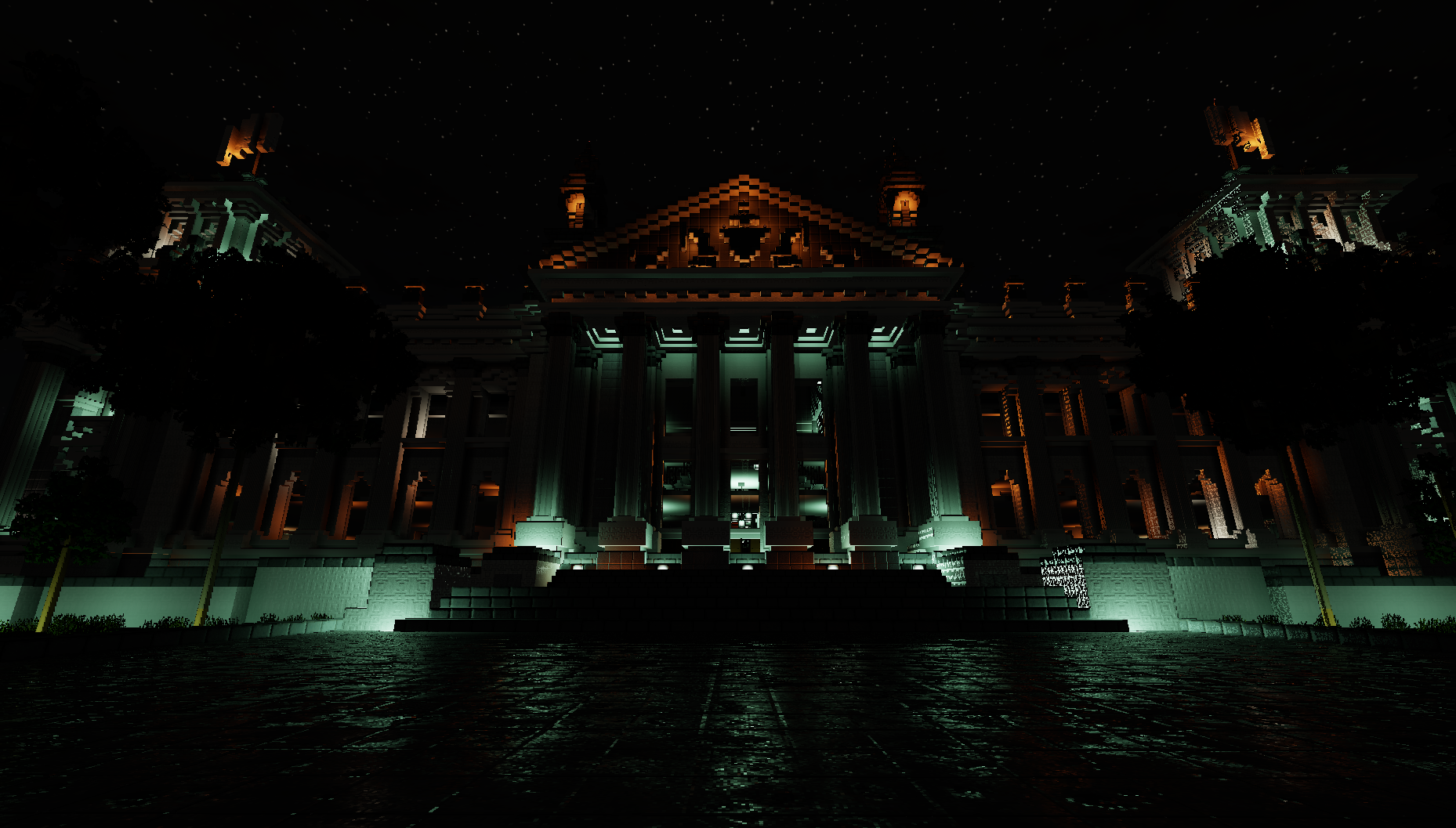
:o OMG what resource pack is this?
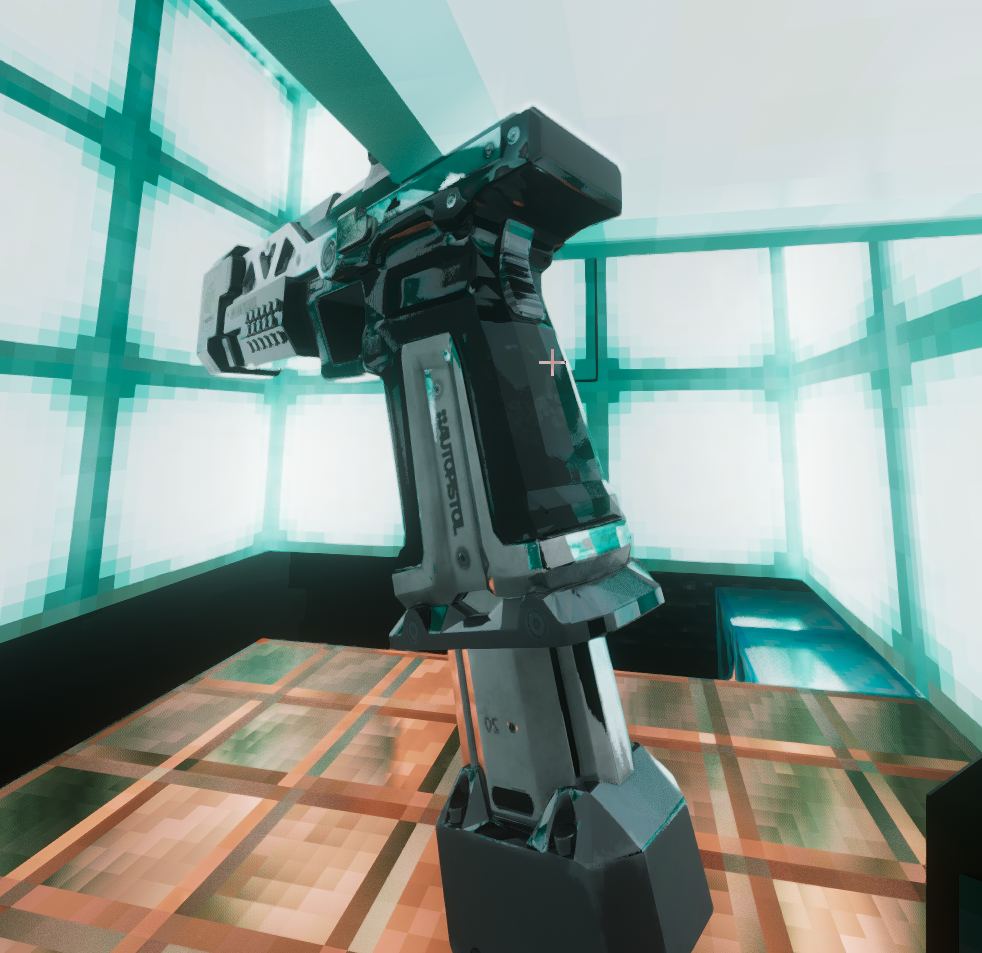
then the mesh is probably bad
It isn't
hm
Probably my OBJ loading though
Perhaps? Idk
Or the renderlayer
Which makes total sense to me, you wouldn't want to render smooth block edges
As in you also don't want to have double vertices with blocks etc
sadly i wouldn't know much about that
if you want to look more into it renderdoc is your friend
it can show you all GL state during the render
Wait woah is that a debug tool?
The mesh in question in blender:
yep, it's amazing
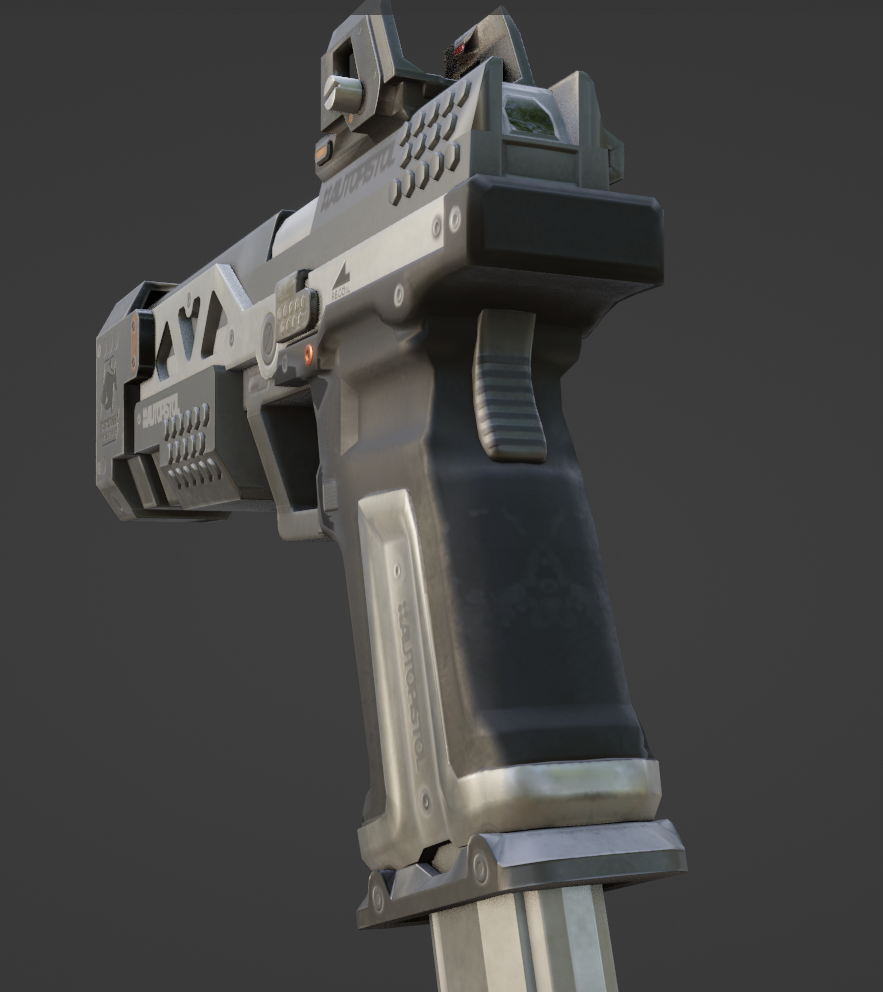
with renderdoc you can literally scroll through how the game renders, and see every part
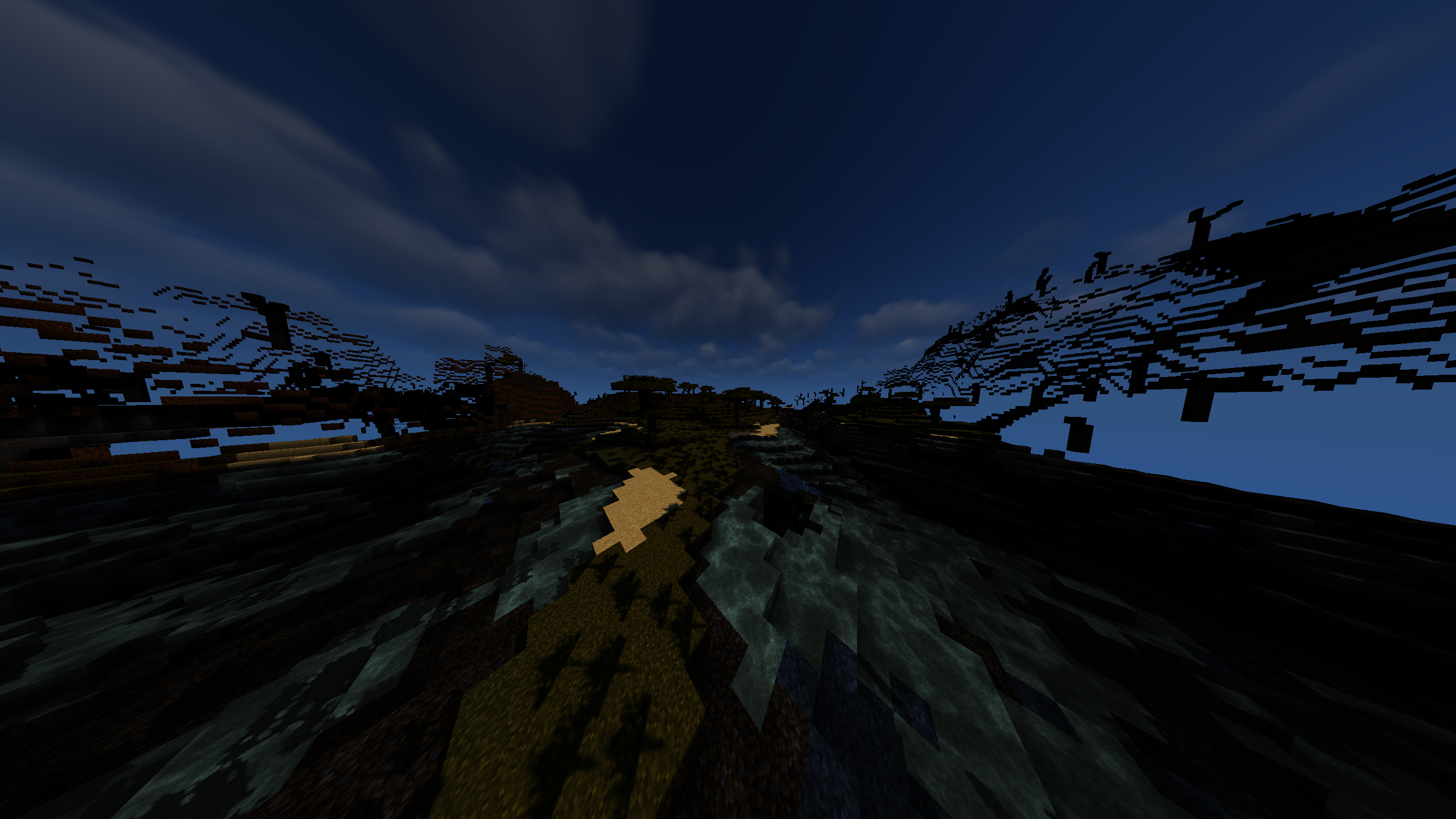
as well as what the GPU is doing and/or thinking at that moment
though it's quite a chore to set up sadly
oh my... I'm installing it rn
yeah not sure then
I bet it's some single GL or shader flag or something
you'll need to grab the launch arguments for the game, which is... annoying, to say the least
maybe?
But just to be sure, the buffer builder has no way or order to enable smooth shading, the interpolation is all on the shader right?
one quick check
how big is the texture
1k
ok, hm...
Nvm 2k, sorry
I mixed something up
it's fine, still that should work?
nvm
I do not load the roughness, both my maps are 2k
In which sense do you mean that?
i'm just looking into comp reimagined's settings
i'm honestly not sure what would cause such a bug
Ah you mean the UV thing, that's so nice of you!
oh no i meant the shading
i can look into uv's too, but i wouldn't know much
Ah huh, smooth shading is not dependent on normal map, it's a shading feature
Or am I misunderstanding you right now?
i meant i was looking into shading before looking into uv's
I am sorry, regarding which problem exactly? This one?
nah, this one
You know what
I average the normals out when baking my mesh (average of each3 vertices)
I will try removing that
Nope
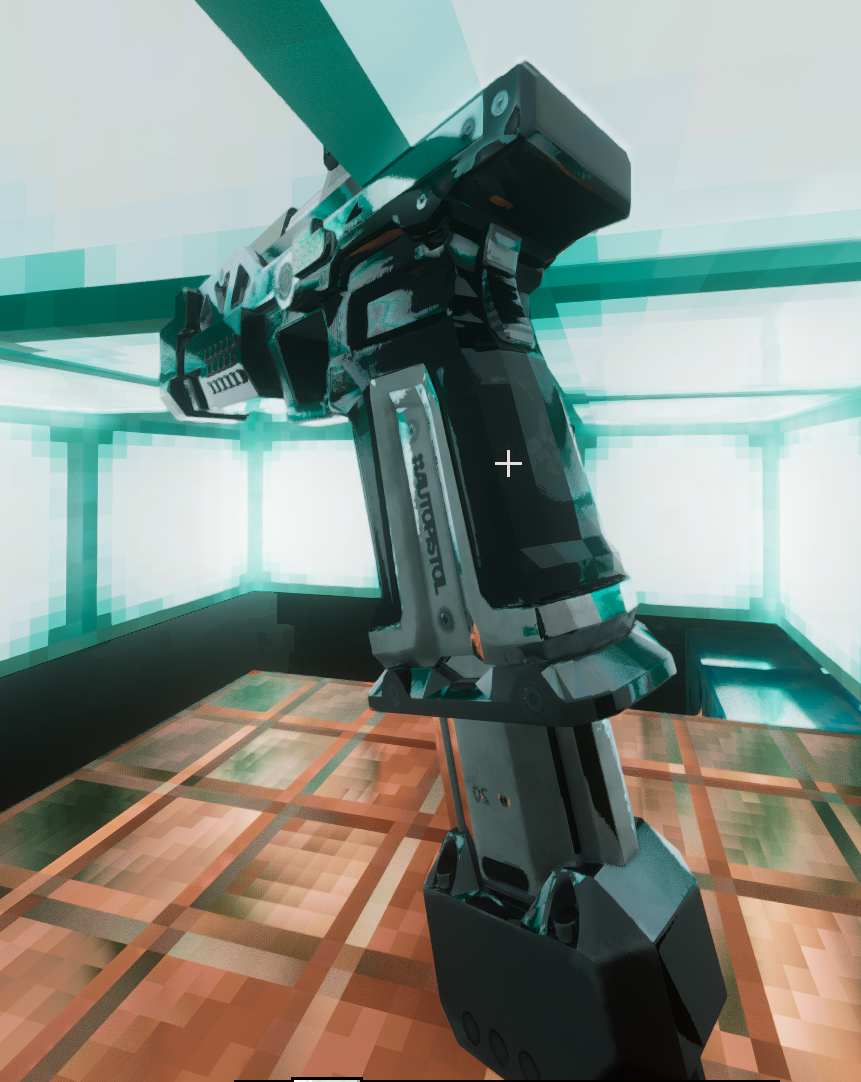
Still that
Then if I had to guess it’s a shader bug
since iris really doesn’t influence those things
Hm, maybe I exported it wrong... Let me look into that
Nope. I tried all different export settings, but when reimporting into blender it works and in minecraft it doesn't.
Did you find anything in the shading? If I am not mistaken they just need to lerp between the vertex normal values and that's it
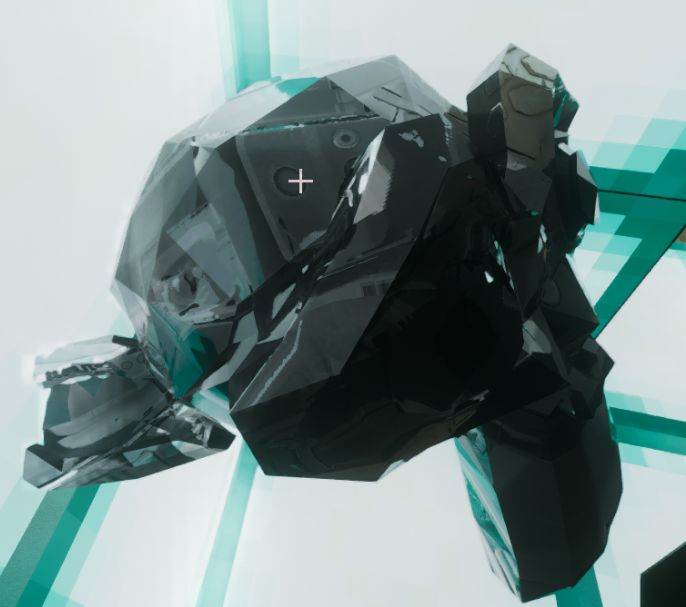
I guess my OBJ parser works fine...
sadly, no
didn't find anything
Okay so they do interpolate nicely there?
however, Create has a custom OBJ loader i've helped with if you're interested in that
no
i just don't know why
Hm, then the shader doesn't support smooth shading at all right?
it should
which is what confuses me
Wait, should the vanilla shader also do it?
Because it doesn't for me
what do you mean?
smooth shading doesn't work even in vanilla?
Okay I didn't know that
no, i thought that's what you were saying?
i was asking a question lol
Oh lol, I was just asking if vanilla does by default, that would result in my mesh being built incorrectly
Speaking of:
well, you can check
just press K and see if it's still shaded badly
Yes please, thank you very much :) I already wrote mine, could you please take a look over it?
yup I did, its not smooth either
sadly, i haven't really worked on it in a while
ok that's very important
one sec
That's the whole parser
Looks fine ig, worked to the point that you saw above
And here is baking the mesh and uploading it to the GPU:
i think you're just running into the "no smooth lighting" vanilla issue, yeah
which can't be fixed really
Ah okay, thought that I might check against vanilla if it did support smooth
@IMS I contacted the complementary lead, we found the UV issue :)
Disabling parallax occlusion mapping fixes the normal map
nice
:))
Okay, so may I ask did you take a quick glance at that code? Imo it looks correct, nothing much can be done wrong with supplying normals
I would really like to fix smooth shading... I understand it the way that no GL flag changes smooth shading but actually the per vertex normal? Idk
Hey, I finally solved the smooth shading!!!
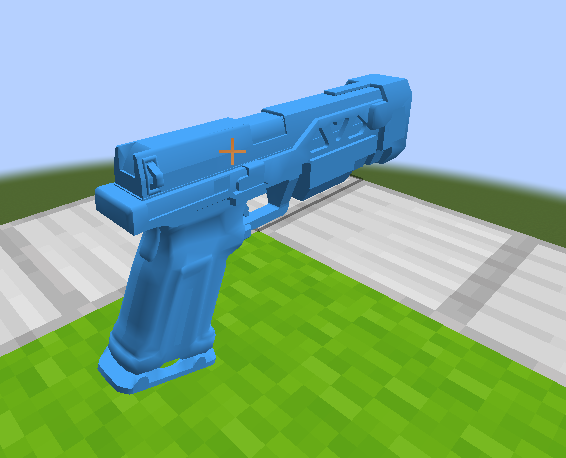
@IMS Hi! :) However, I found a super weird bug, even with shaders
When I look at this mesh from here, its fine
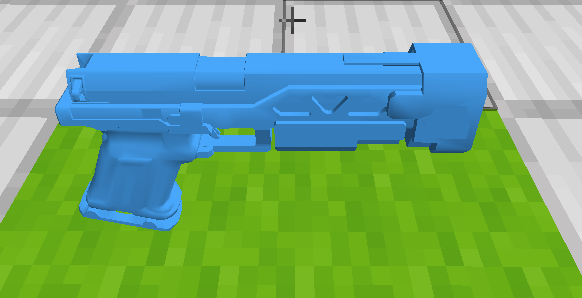
However the more I look from the top, the darker it gets
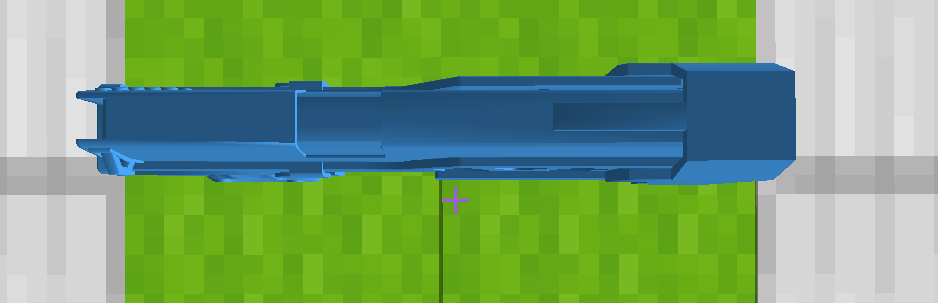
The following is a sequence of decreasing camera angle.
It seems as if the camera angle is the only thing changing lighting, NOT THE VIEWING ANGLE
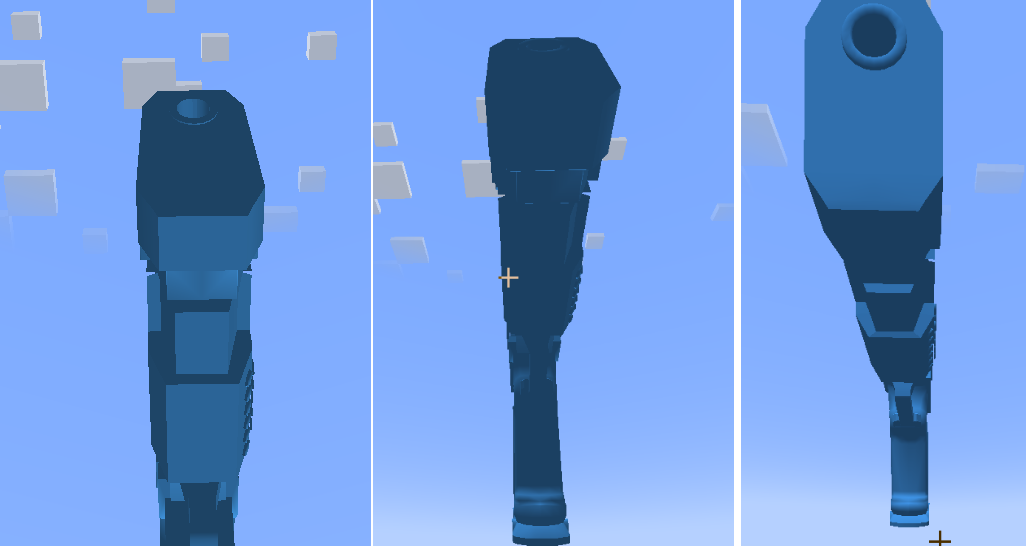
Note that in the middle, it almost looks flat, not shaded at all
With BSL the angles are different, though the same behaviour
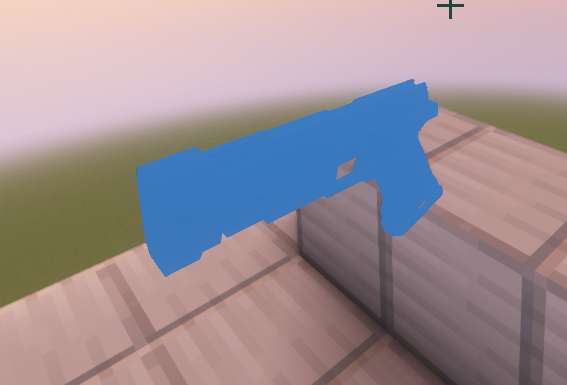
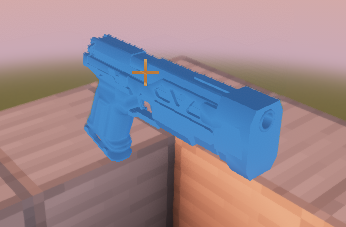
With Kappa shaders, it's flat from all angles
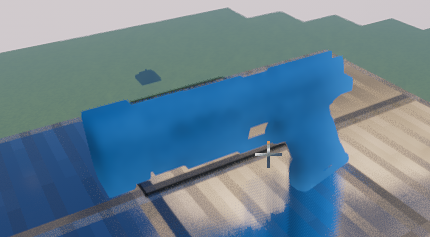
Do you know what's going on here?
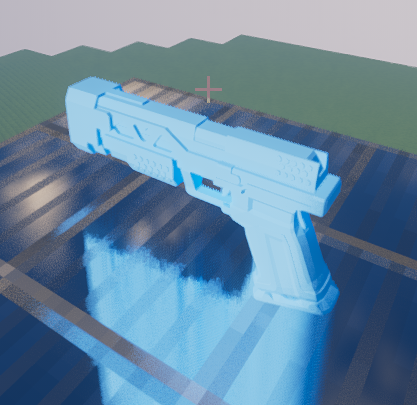
This is kappa when exporting the mesh as flat shaded
i assume it's related to normals
normals are in view space
(there is no normal map btw)
Hm, do you have an idea why in Kappa for example it's completely unshaded ("flat") when exporting as a smooth mesh?
Do these mesh normals look right to you? So, is my mesh built correctly?
Between smooth and flat, my code stays the same, only the exported values are different, does that mean my mesh building code is correct?
I want to exclude mesh building factors, maybe it's where I subscribe my rendering event or something
Ok, so the whole mesh acts as a flat mirror
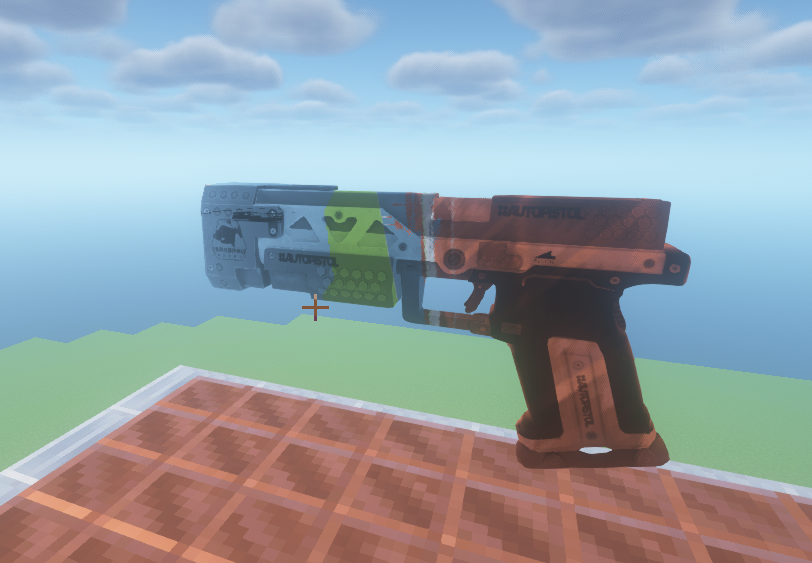
it's kinda rotated by 90 degrees etc
I guess my mesh building code is definitely doing something sketchy with exported shaded meshes?
it seems as if even the normalmaps have been overwritten... What could the cause of that whole smooth shading be?
(normal map normally makes spikes, which worked with flat shading)
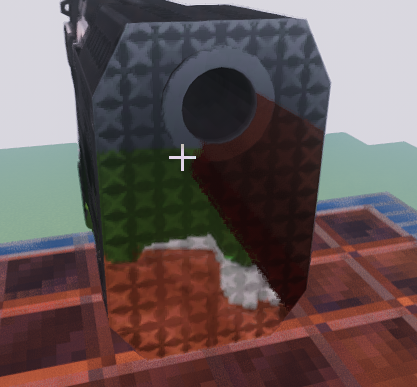
nvm, also happens on flat exported meshes when they are metallic
I also noticed super weird behaviour, some smooth exported meshes are rendered flat, some are not (same mesh but exporting twice whilst no process is accessing the file)
I will rework my parser again...
Hm, I am really unsure
When I export as obj, it's always flat
When I export as obj(legacy) it looks like this with rethinking voxels:
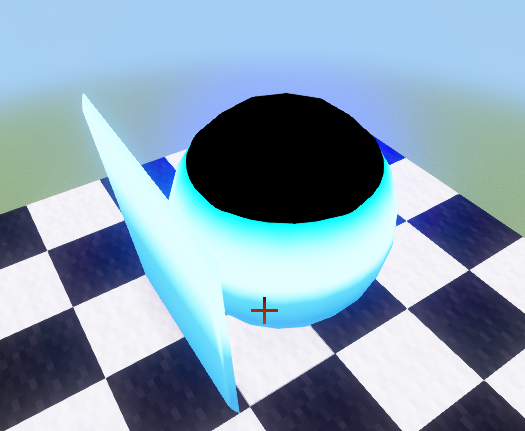
(should be a normal only color diffuse map, nothing more)
different angle
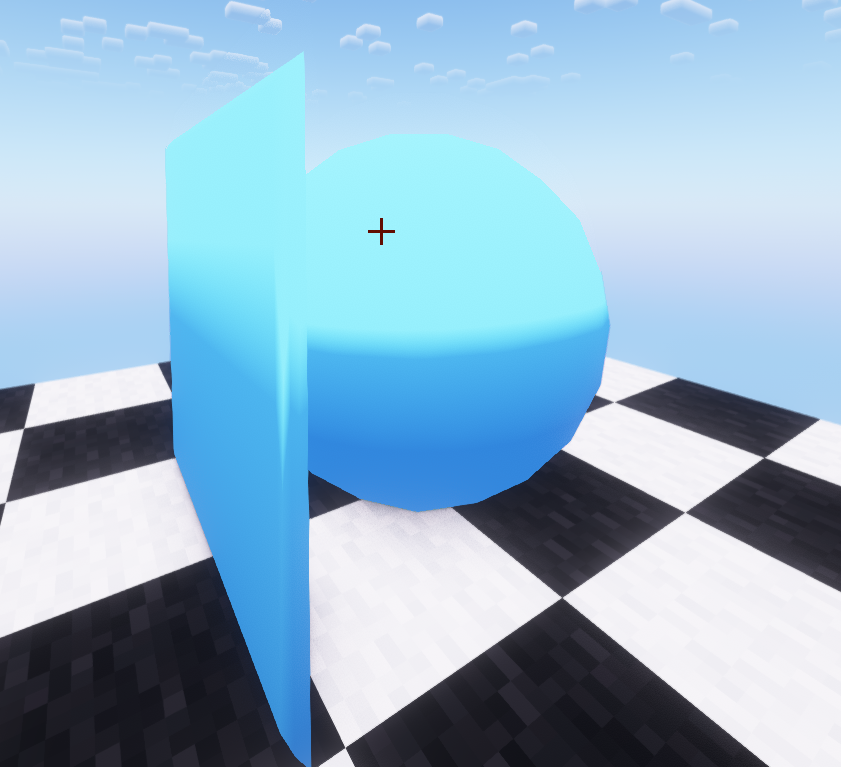
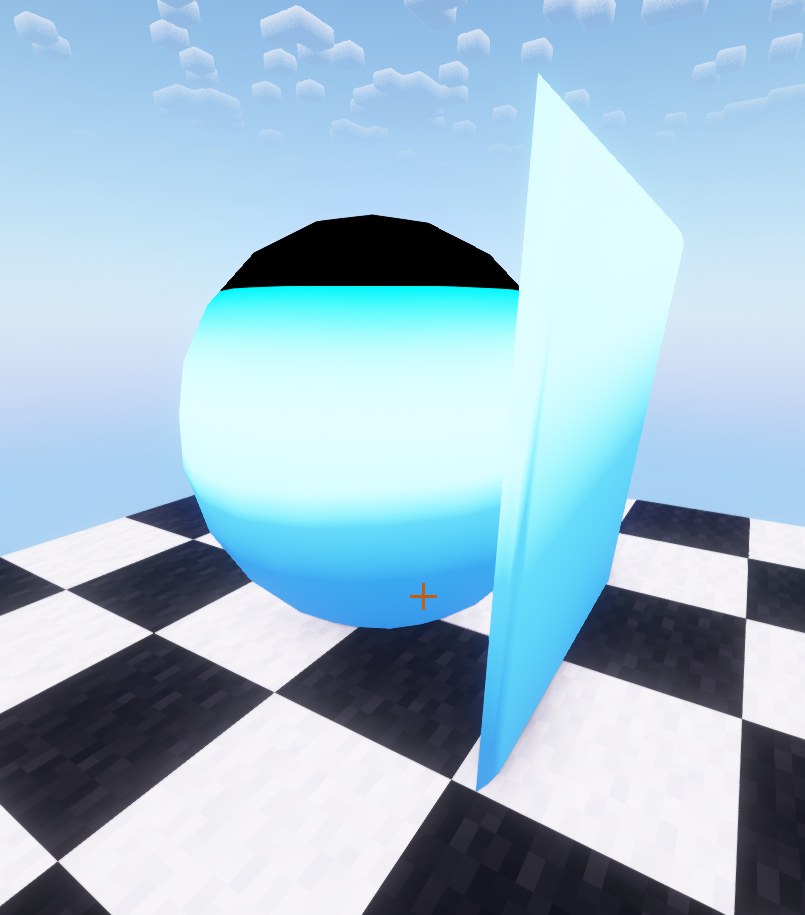
different angle again
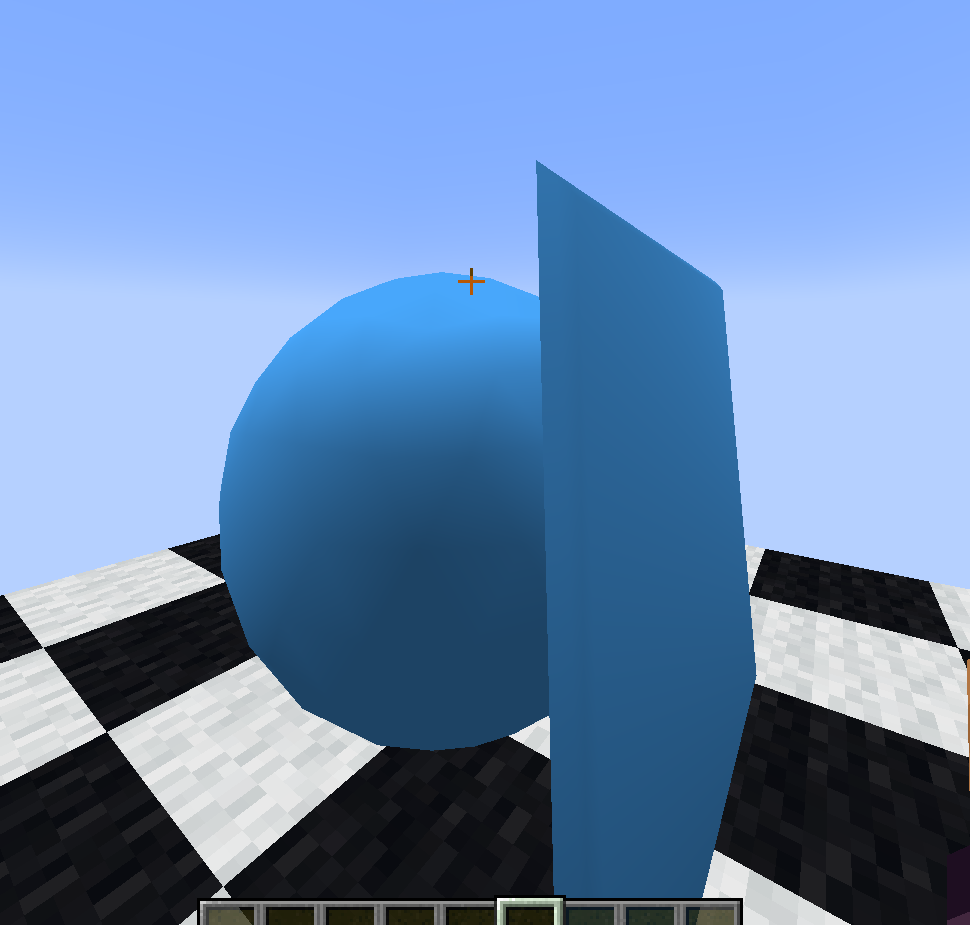
this is vanilla (dark areas change with me moving around, def not how it's supposed to look)
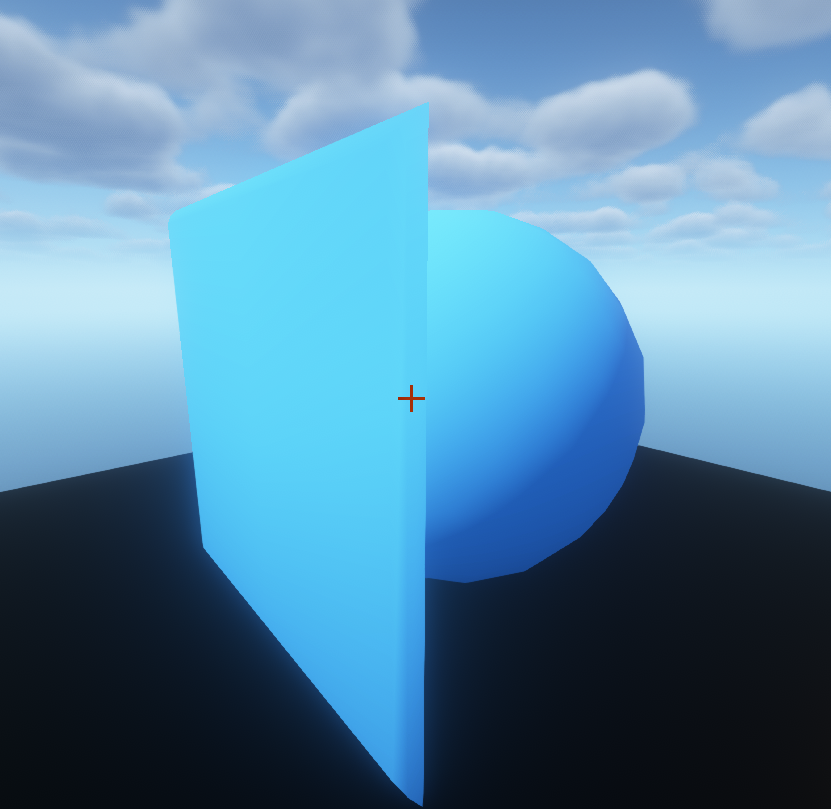
BSL is the only shader apparently rendering it like its supposed to (I am super confused)
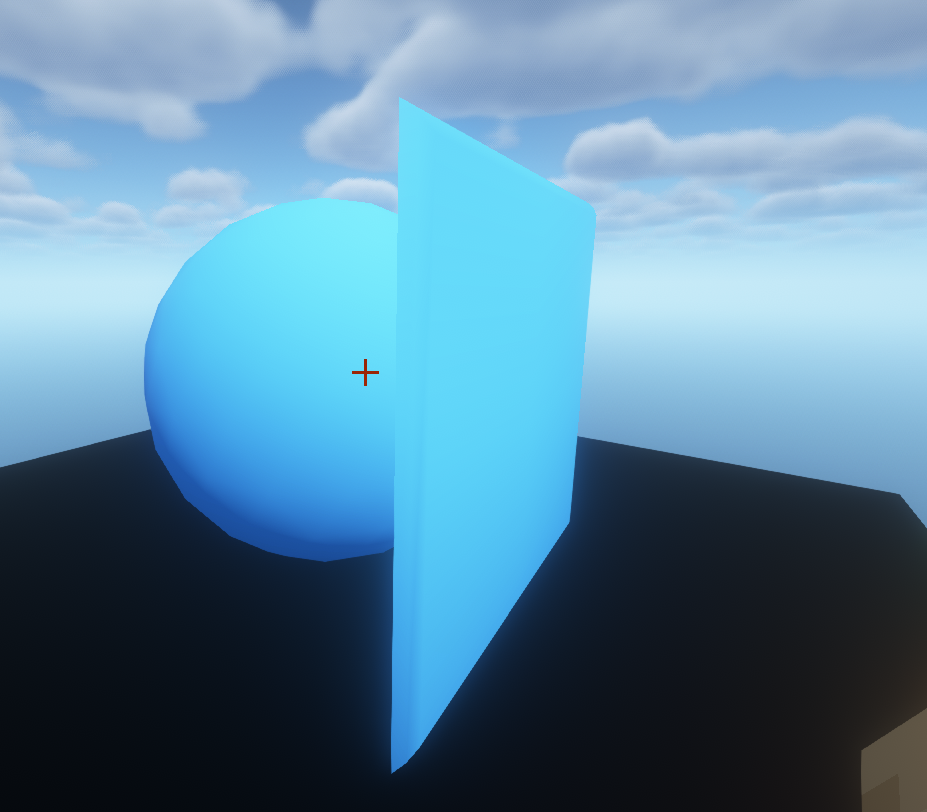
OK I definitely need your help hedge hog. I just found out: when loading a color map only, it is being shaded smoothly.
When I load a color and specular map, the mesh is being rendered completely flat. When loading color, specular and normal, it has the werid effect being shown in my pistol screenshots
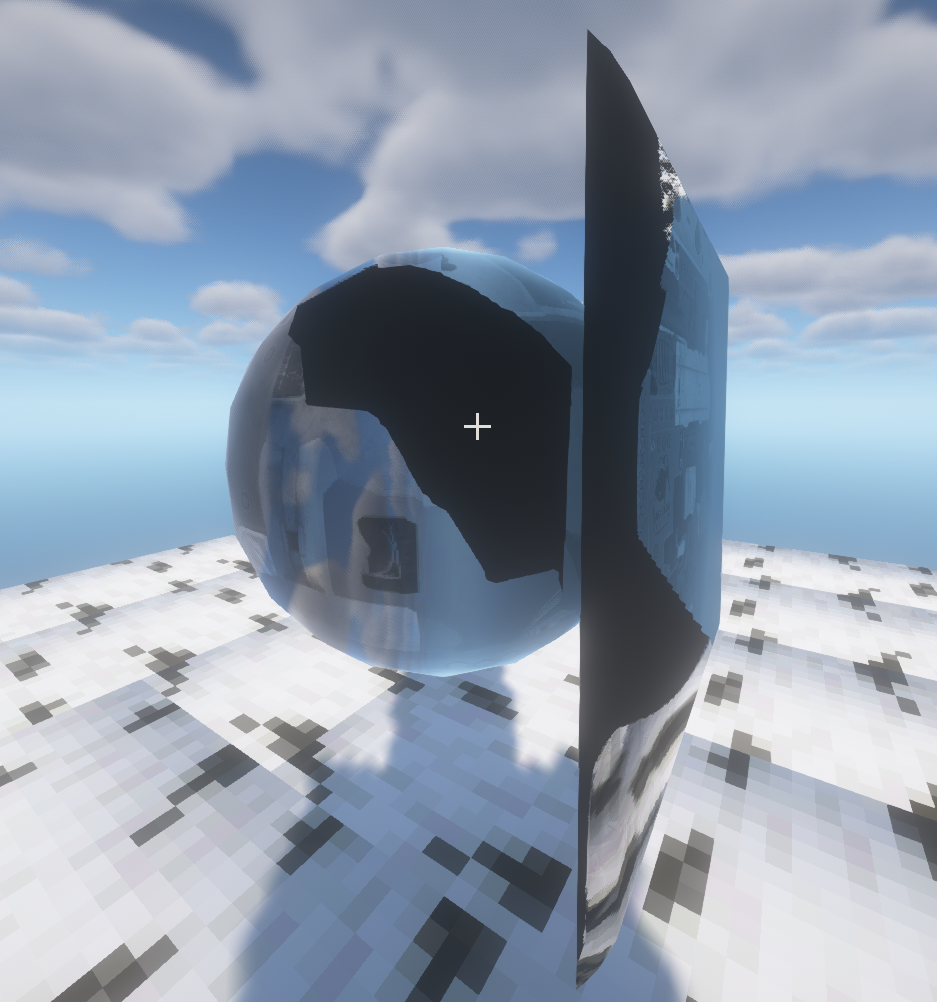
(clouds are 90 degree rotated)
that seems like almost certainly a shader bug
But it's consistent across all shaders I have installed
the only thing I can think of is if we're somehow screwing up the tangents
we do automatic tangent calculation based on normal + uv
here, download supertester from https://github.com/sp614x/supertester
GitHub
GitHub - sp614x/SuperTester: Super tester shader pack
Super tester shader pack. Contribute to sp614x/SuperTester development by creating an account on GitHub.
apply it as a shader, and then set GBUFFER_DEBUG in the shader settings to at_tangent
see if it looks right
Yes thanks I am doing that rn
🙏 :)
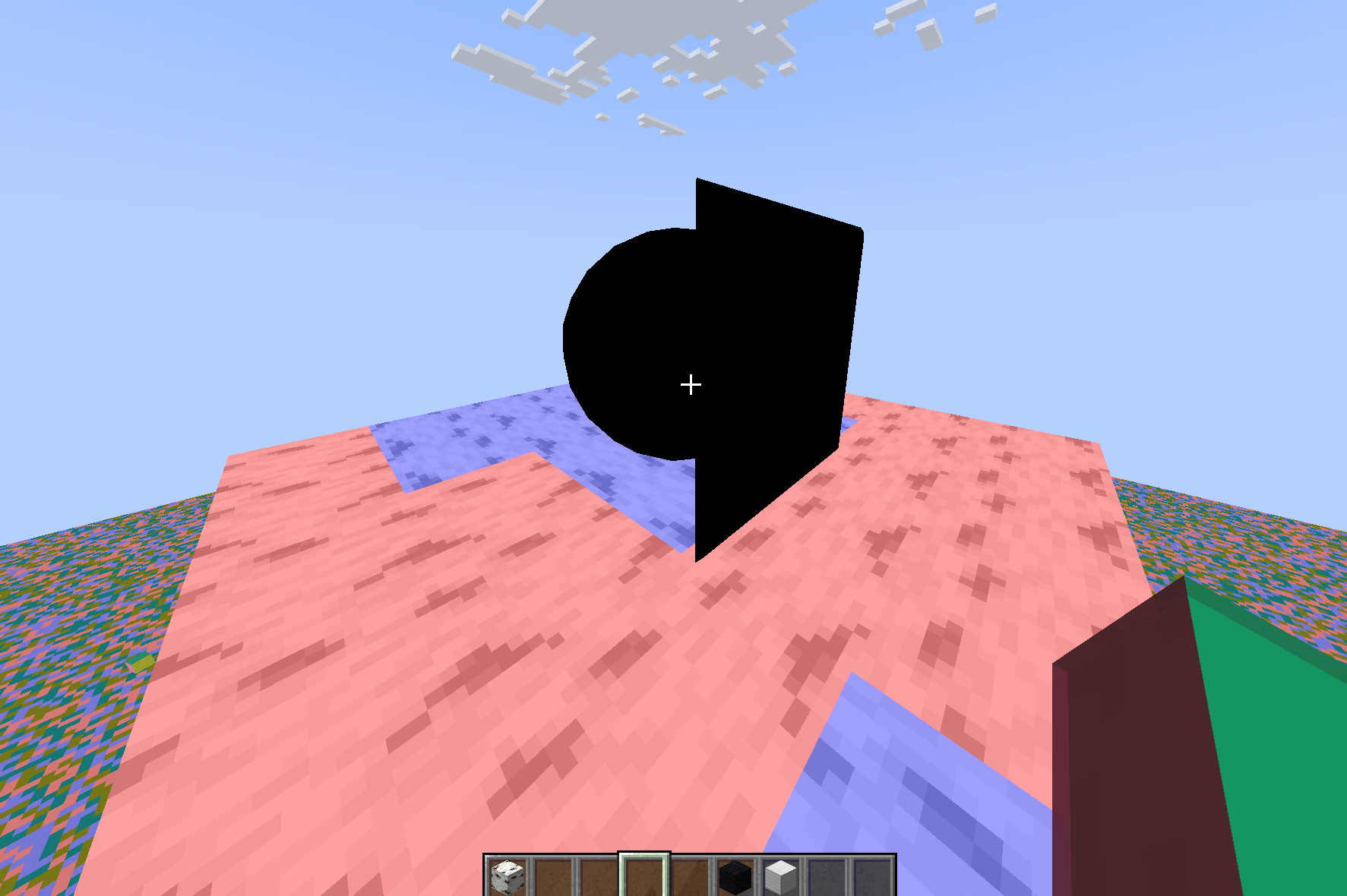
Color, Specular, Normal -> Black from all sides
Let me check for all texture combinations (except normal map only, should I also set that up)?
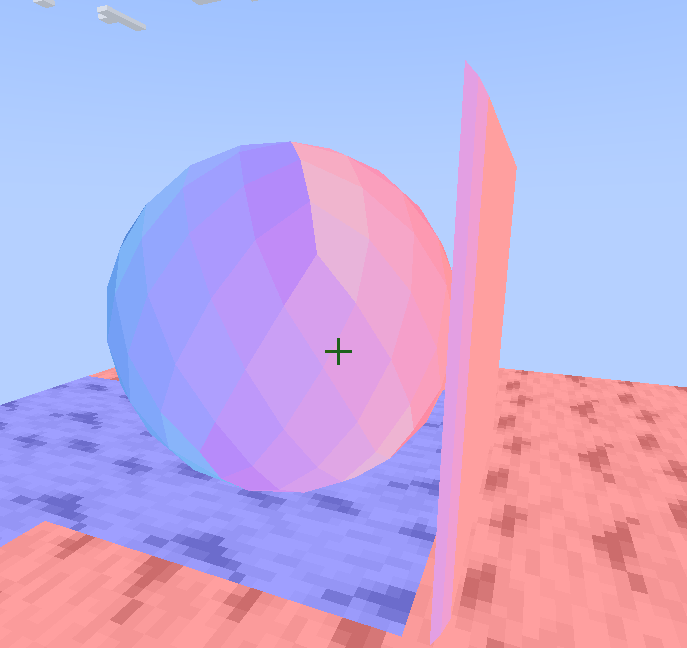
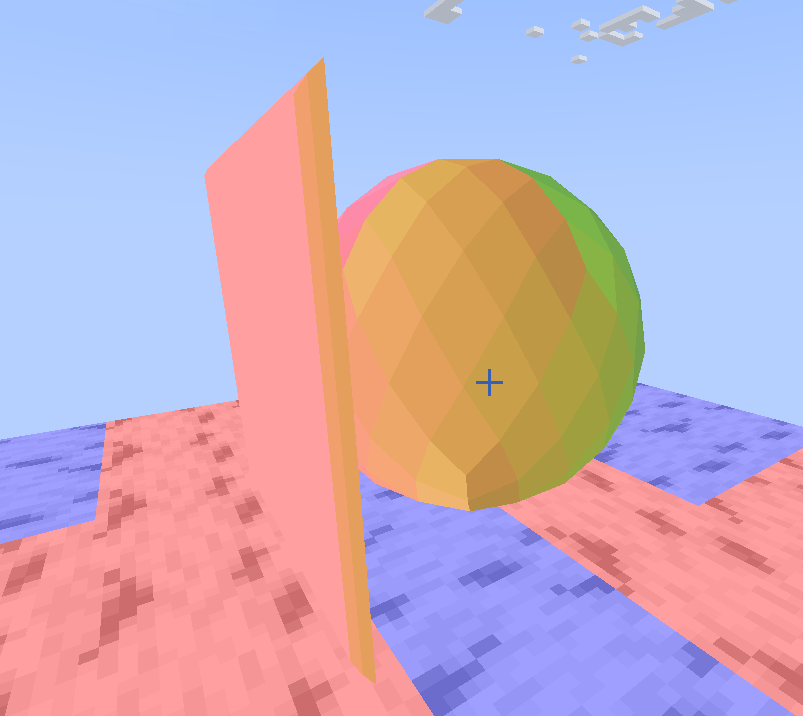
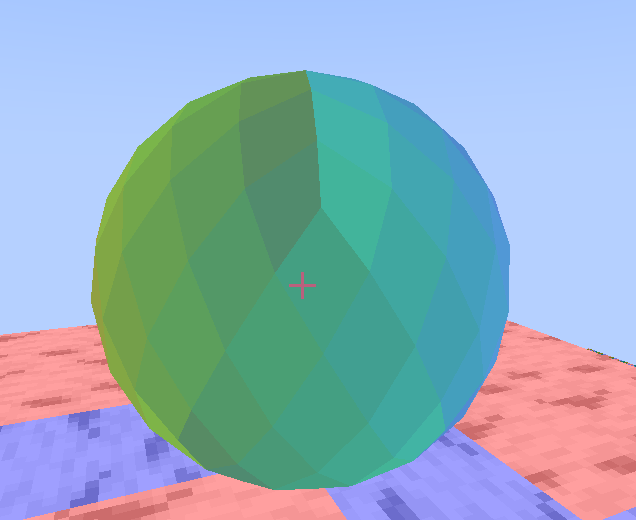
^ Color and specular only
ok, that's not good...
wait a second
lemme scroll up to your code again lol
I will send you the newest one!
I did a complete rework
hm, your render layer is corect
(its better readable and actually does make smooth meshes somehow)
the only reason tangent should be null is if your code is somehow skipping it
which it isn't afaik
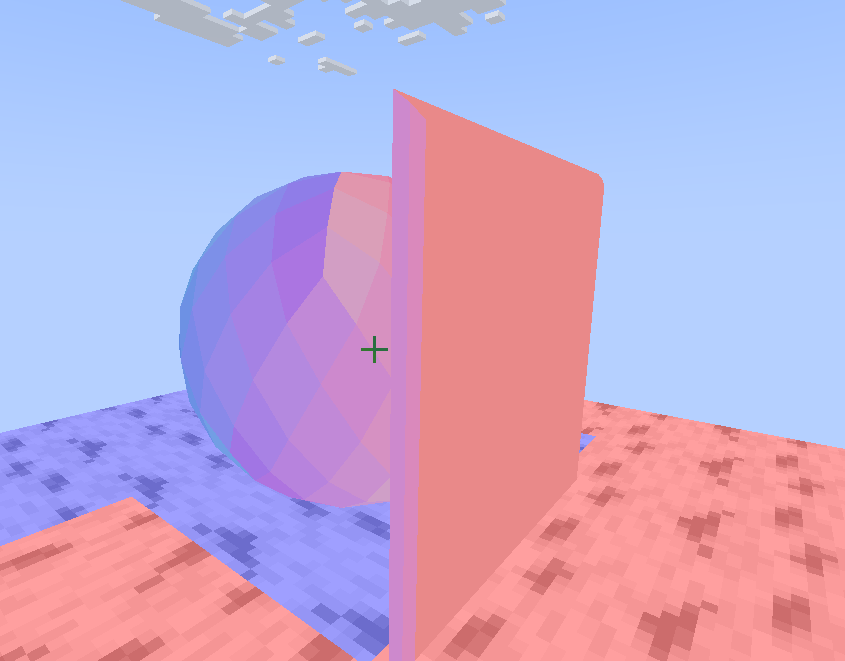
Tangents are the same for color only and color+specular
do they look correct?
so it's color+normal+specular that's broken only?
I can not tell. Should I export flat only? Should they be identical? (I am doing that rn)
do the colors match up with the world
well actually nvm
does each side have it's own color
it should look kinda like a color sphere
Well then it's probably wrong, if you look here it looks off
I provided 360 screenshots, do you need more angles?
no, i'm just confused
i don't know how you could be breaking tangent calculations
It's booting up, I am testing against flat exported mesh
do normals look right?
and i mean in the shader
I will provide my code in a bit
switch gbuffer_debug to glx_normals
Let me test, one sec
Wait please, I just found out that exporting obj vs obj(legacy) makes it flat and smooth respecitvely
I am sorry for the delay, I can't hotswap, need to reboot mc when changing models and I can only test the normals when the mesh is smooth in vanilla too which it isnt rn
HUH?
Now with color only its also black with tangent
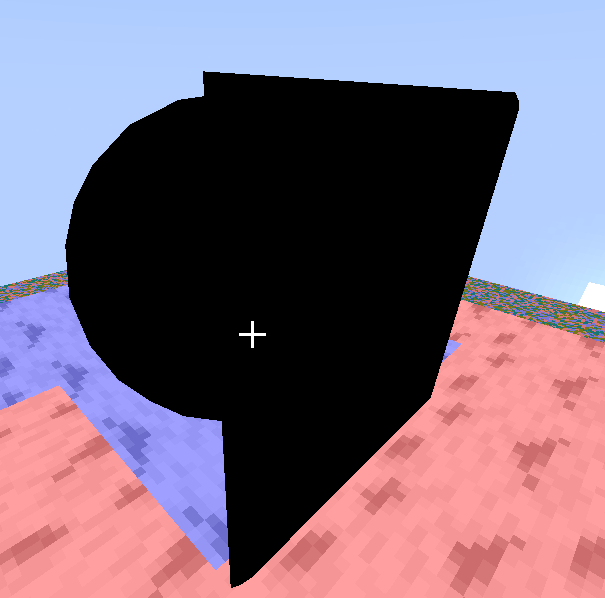
this is color only texture
Before it was not like that, I presumably didn't change anything
I will give you normals in a second, just need to confirm that the mesh behind the normals is 'correct', at least coherent
??? after restarting with another color only texture the tangents are back and it's completely flat [EDITED]
I am so sorry for all the messages but I believe verbosity is key to strange problems like this
Absolute, consistent findings:
Booting into world with vanilla shaders loaded + color only texture
->
smooth shading in vanilla (still looking slightly weird with weird dark patches)
black tangents
correct shading in bsl (only color, idk about reflections)
incorrect shading with kappa and rethinking voxels
Booting with vanilla shaders loaded + color and specular texture
->
smooth shading in vanilla (still looking slightly weird with weird dark patches)
black tangents
incorrect reflections (90 degree angle etc)
Booting with vanilla shaders loaded + color, specular and normal texture
->
same findings
AHA
Booting with BSL loaded initially
->
Renders mesh flat in every shader including vanilla??? Therefore giving it tangent colors now
how???
that doesn't make sense
😭
IDK, booting with BSL loaded makes it flat with every possible texture
So, to conclude, it was NOT a texture issue, it was an issue with which shader I boot minecraft.
Keep in mind, BSL messes up reflections, but diffuse seems to shade correct! (every other shader though messes up even diffuse only)
Here are the normals (for vanilla loaded, black tangents):
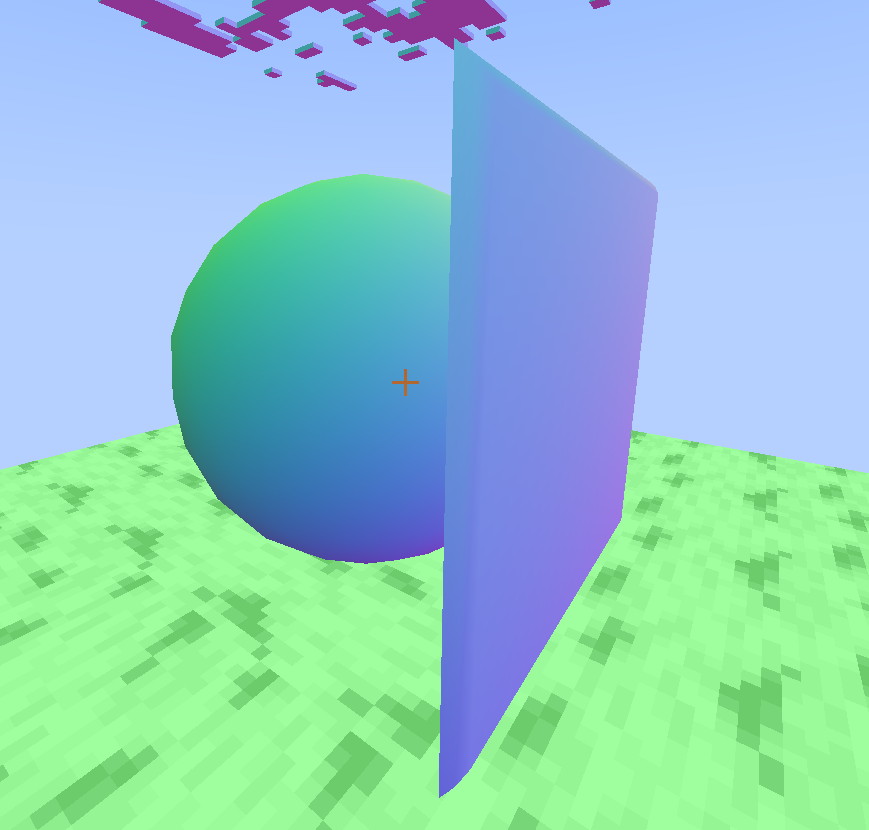
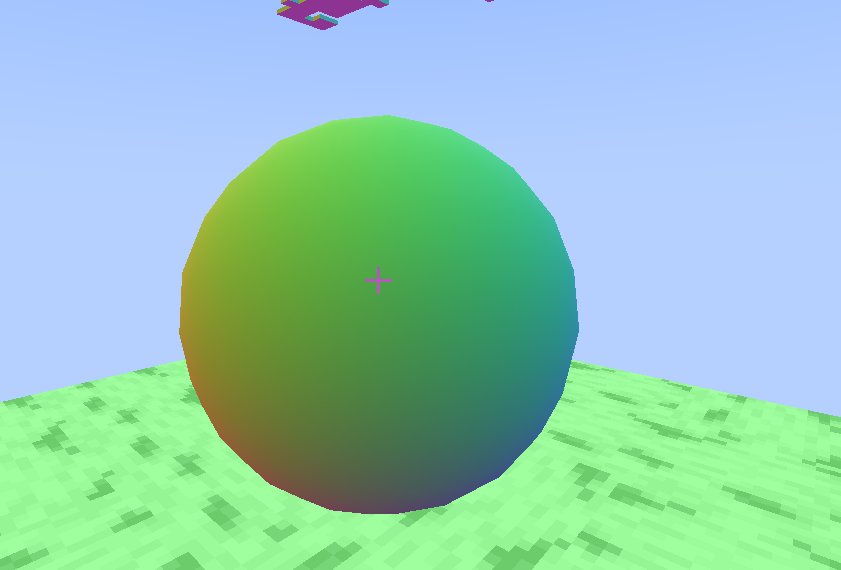
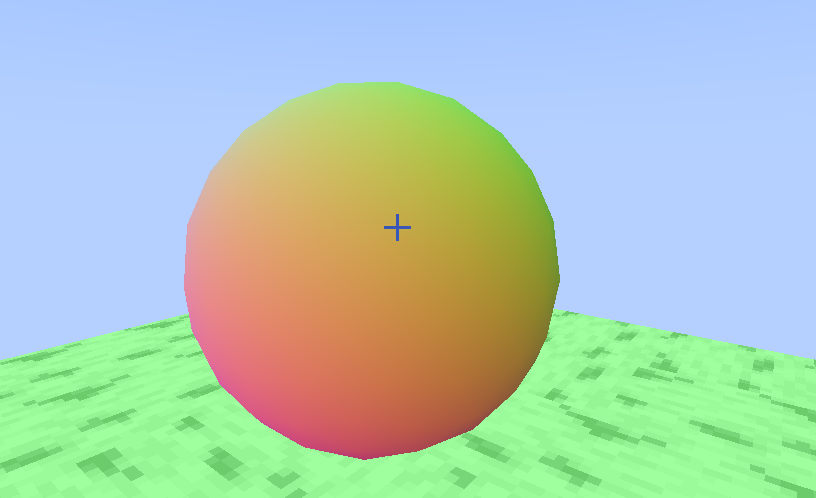
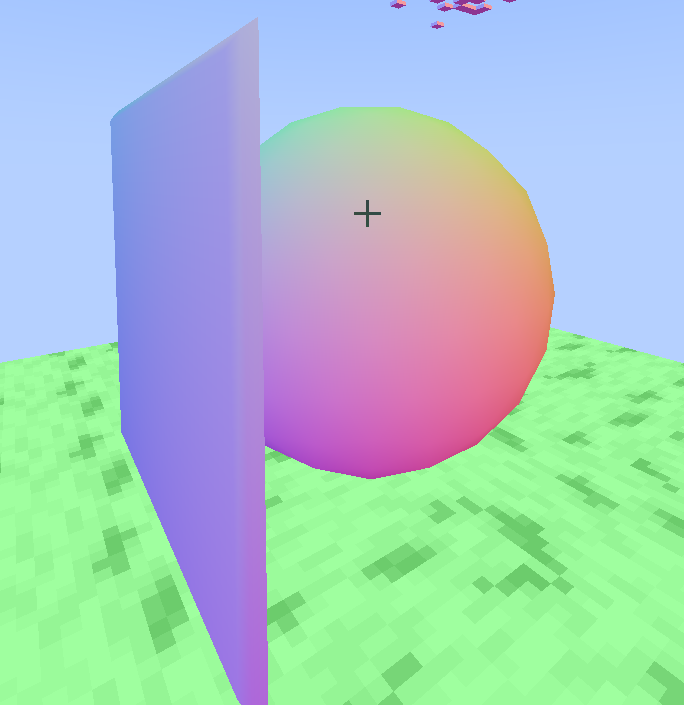
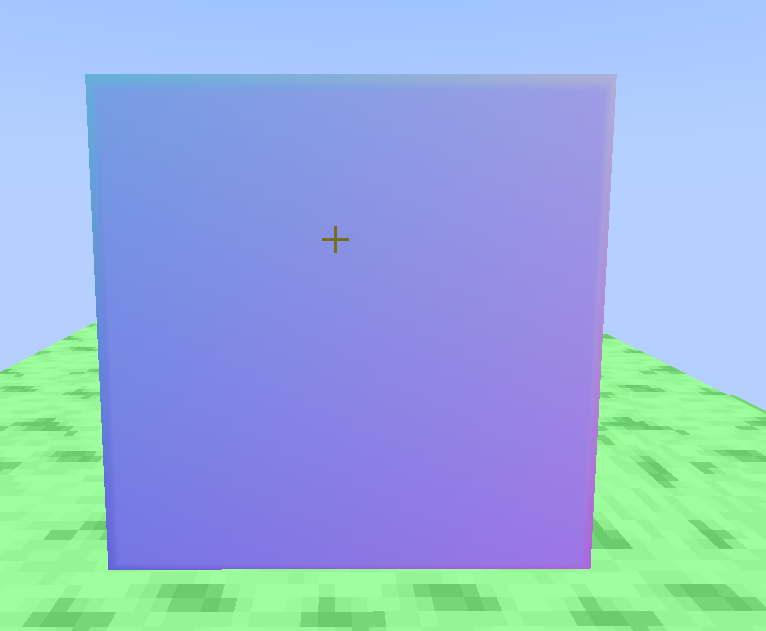
What was I talking, that was default interpolation behaviour, yes, the normals are correct, at least when I boot the game in vanilla shaders.
The tangents seem to be the problem:
Rendering code:
That's my whole rendering code. Ignore the .get(newLight) part, I was too dumb to change vertex lights after building the buffer, so I just prebaked many possible block and skylight combinations and put them in a hashmap like an ape.
So, I booted into vanilla, THEN loaded BSL (or Kappa or even the supertester) and then baked the mesh. The mesh builds flat.
wait
This must be Iris, when any shader is loaded
are you baking the mesh once
and then rerendering it?
that must be why
Iris assumes you will be doing per-frame meshing
as MC itself does
Yes. Here is the baking code:
yeah... that's a critical problem
Basically it:
- makes a new BufferBuilder, begins it
- takes all faces of the mesh and builds it with the bufferBuilder
- ends the bufferBuilder, creates a VBO (which uploads it to GPU) and yeah
the problem comes from the fact that with shaders off, Iris doesn't produce the extra info
like tangents
because it's a waste
Per frame meshing is super inefficient for static meshes
and if you force it to produce that extra info
vanilla will crash
i don't think you need to do it per frame
but per-reload somehow
when a shader is changed
Hm, does that make sense?
I think I don't get your point
After I have long loaded any shader, I then shift (which bakes the mesh) and it's still flat
So basically all shaders are already loaded, then I bake, then it's flat
If I build in vanilla at any points it's smooth (correct I guess) but Iris doesn't provide tangents
yeah, i don't know why that would happen...
Hm
So, the whole bufferBuilder has nothing to do with shaders nor GPUs, so that's excluded
That's one of the 'variables' changing when a shader is loaded
it does
iris replaces major portions of it with shaders on
that's where all of the tricks take place
Well up until uploading the BufferBuilder is completely CPU side right?
yep
but Iris does a bunch of checks
Oh
So that must be it
it's where tangents are added to the mix
Damn
you have to somehow make it remesh on a shader reload
Just out of curiosity, what are the tangents for exactly? Does vanilla have them?
pbr
and no
it's a critical part of PBR lighting models
Okay... Now it makes sense to me, the testing results above
I will take a look into iris' source code
👍
Before I do (so that I prime my knowledge, understand more) what does Iris do with BufferBuilders?
you're looking for net.coderbot.iris.mixin.vertices
❤️
that's where all the bufferbuilder stuff happens
when you call endVertex for the third time, it goes through the whole triangle, computes a bunch of info, and adds backward
to give the triangles info about it's own vertices
Which branch should I look into?
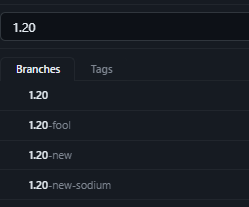
1.20-new
endVertex doesn't seem to exist in 1.20
Thank you
we use mojmap names
it's next in yarn
Oh I see, thanks!
https://linkie.shedaniel.dev/mappings?namespace=mojang&version=1.20.1&search=field_44912&translateAs=yarn
use this to translate from mojmap to yarn
Ah that's awesome 😍
And sorry for my rusty mixin understanding, but is that endVertex completely intercepted, so everything is being replaced by iris?
Yeah
yep
you're looking for
computeTangent
though prob
since normal is right, isn't it?Actually, it's just that
Normals are correct in vanilla
When shaders are loaded, normals are flat all of the sudden
ok, yeah
Because of this
Omg it seems as if I just need to remove that line
What a blessing
Tangents should be rebuilt automatically
yep... but the problem is that's kinda important
for other cases
Hm, for what exactly?
I think we somehow need to add a flag that says to Iris "yes, these normals are right"
Minecraft's normals are completely and utterly wrong
Iris has to recreate them
But, Minecraft rarely uses tris right?
it does the same thing for quads
look below
just a different function
I know, but what if we just removed the tris recalc?
Then MC shall be good
that might work, but there's a chance if a mod uses those normals it'll break
I guess it's fine, though
I'll remove itg
Thank you so much 😍
Actually
Can I do a PR please :3
@IMS
Only if it's okaty
sure, but we usually apply fixes to the 1.18.2 branch first
Yayyy
Shall I do a PR to that too?
sure
❤️
What do you prefer, if I completely remove the line or if I comment it out and add a comment saying that for some mods with broken normals they need to recalculate their normals themselves?
comment it plz
Sure!
Ok I did the PRs :)
i meant to do one to 1.18.2 lol
don't worry, i'll fix it
Wait its also loading
oh
I did both, just needed to copy the text over :)
Oh, it was immediately closed?
i merged the 1.18.2 one and closed the 1.20 one
i'll do 1.20 when I update 1.20 to 1.18.2's code
Ah I see now, great, thank you!
And thank you for letting me do the PR :)
Regarding the 1.20 one (and I wanted to know that anyways for other mods), is there an easy way to host my mods from github repos?
Or do I have to compile my branch to a jar?
not really
but once your mod is on modrinth, modrinth itself acts as a maven
for all of your releases
Oh modrinth is so damn nice
Other than that, can I somehow modImplement my local repo files?
to iris?
or to another mod
To any for that matter
iris doesn't use gradle so it's quite different
it uses a custom system made for minecraft
Oh I see, so I guess I will compile the branch manually
probably best, yeah
Woah, sounds complicated
And regarding my own other mods?
I'd like to have API access without compiling to JARs
modImplementation(files("filename.jar"))
put filename.jar in your mod source folder
Oh great thanks! It must be compiled right?
yep
you can compile iris using one command
java -jar brachyura-bootstrap-0.jar build
Great, thank you a lot!
I will compile and see if the tangents work :D
no problem!
1.20 isn't updated yet
give me a few minutes lol
i'm still on 1.19.4
Oh okay sure, thanks a lot :D
should be up in a few minutes, watch #iris-updates
for 1.20 to show up
Okay! :blush_AE:
@Sinan up now, pull 1.20-new
actually i'll just send you a jar
That's so nice of you 😍
Instantly! Thank you so much!
it takes like 15 minutes for a new user to build iris
due to the amount of downloads
I'm so excited ^^
Okayy, so not quite what we expected
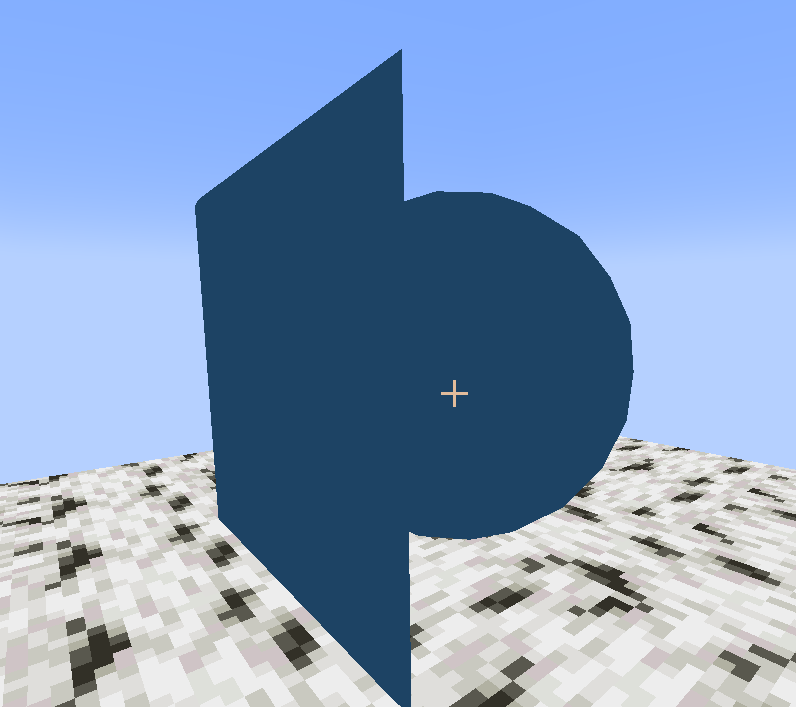
This is vanilla
Although the tangents are now there!
that's really weird
BSL looks the same
maybe with iris no longer there to supply normals it doesn't know what to do
Oh ofc!
?
The mixin completely replaces the function right? I believe the normals might not even get saved from the beginning?
lemme check
Ok sure me too! (idk what I am doing lol)
no, normals get saved
nevermind, your fix did nothing
because of a mistake in my area of the code
pain
Oh lmao
May I ask what it was?
the line you commented out was to give normals to a vector
however, iris didn't know you commented that out
so it was just giving empty data and nuking whatever existed before
Lol
How does Iris decide that on it's own? Is that the custom system?
yep, see the code right below
it packs the normals into an integer, regardless of the fact you commented the thing that gives the normals
so it was just packing 0, and replacing the right values with 0
Oh wait, it was completely my fault, now I see it
xD
try this one
Interesting?
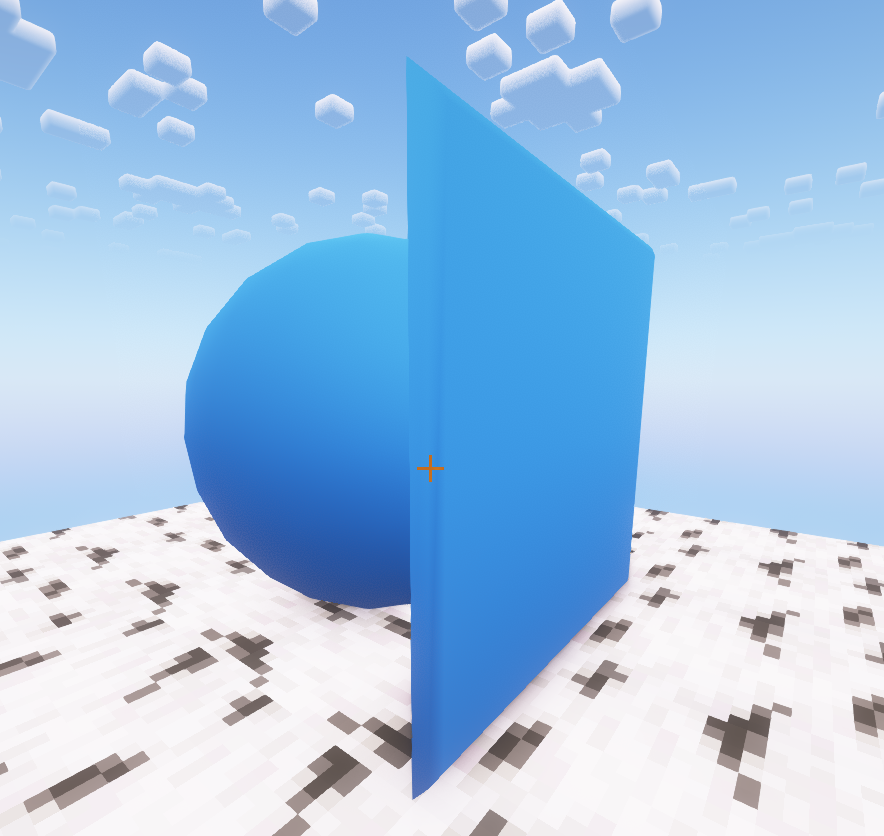
Rethinking voxels
(BSL also does a great job)
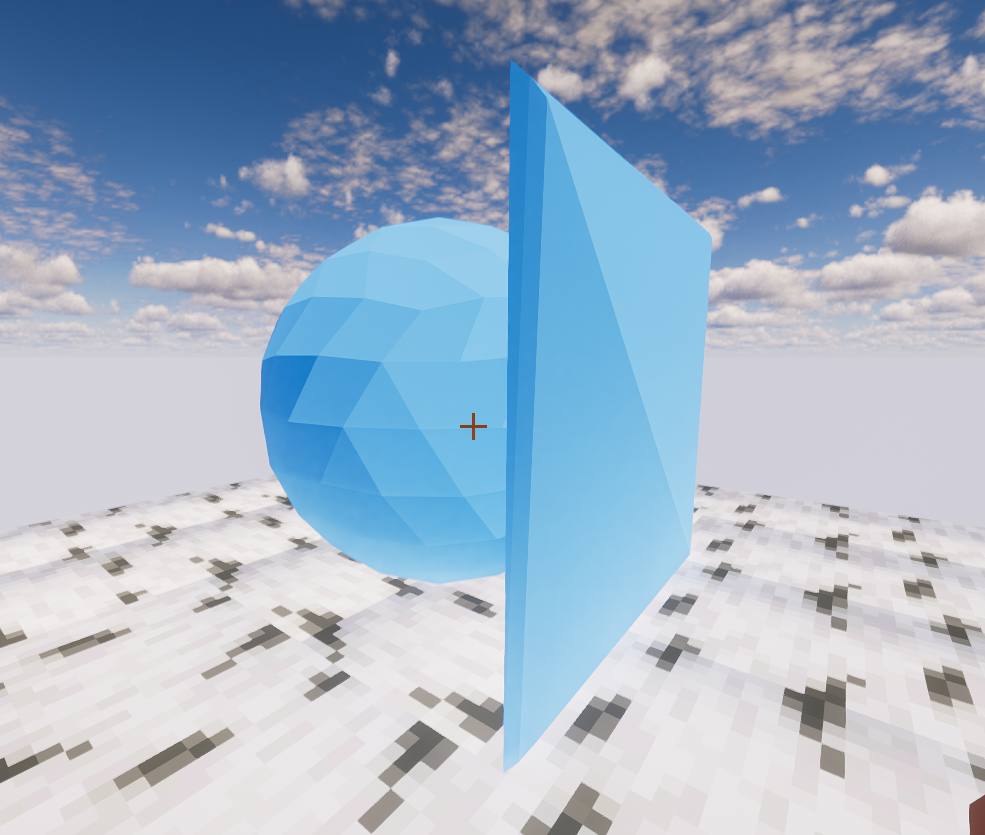
Kappa
Despite that, let me try to load metallics
So basically the Tangents are still calculated for a flat normal
lemme see
Maybe Kappa relies only on tangents
hm...
Whcihc makes sense cause kappas shading was the only one that was completely flat in the beginning
i can make some code quick to make the tangents work with your normals
That would be awesome!
I can also give it a shot but you will be 1000x faster and 1000x more accurate
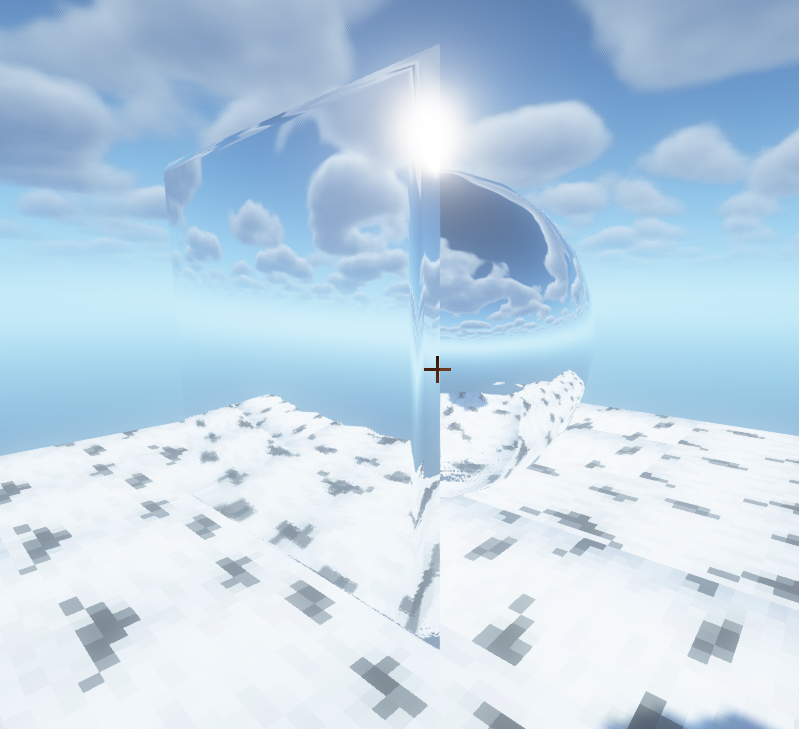
Juup, BSL does excellent with the new normals :D
IT'S SO BEAUTIFUL
try this
(i know it's the same file name; trust me it's different :ioa:)
Hahahaha
Hm, still the same flat tangents
Can I please have a look into your new codesnippet? Just to also learn from that
So it's one tangent being passed
basically instead of computing a normal, i steal it from whatever was put in the original bufferbuilder
yes
not per-vertex
per triangle
I believe we need 3 per-vertex
Or do we? Idk
Otherwise the shader can't interpolate
you probably do, honestly...
that's pretty expensive
i can probably do that just for the triangle code path though
Yes that would be awesome 😍
Thanks :))
It's still flat
Both in kappa and supertester
then it's probably a flaw with the tangent calculation itself
which i am way too dumb to understand
GitHub
Iris/src/main/java/net/coderbot/iris/vertices/NormalHelper.java at ...
(WIP) A modern shaders mod for Minecraft intended to be compatible with existing OptiFine shader packs - IrisShaders/Iris
ok
@Sinan
i found out it is impossible to calculate a spherical tangent using the normal tangent calculation
you must use a separate equation which minecraft doesn't have
so... it's not really possible
kappa probably needs to just find a way to make it smooth, i can send you the discord of the kappa dev if you want
(though they're not too active)
Yes please! I was looking for it but didn't find it, nor github
How is that?
the normal tangent calculation relies on the concept that a triangle can have it's tangent calculated using the 3 points + the UV's
when it's a sphere, it doesn't understand that it curves
and that falls apart somehow idk
What separate equation is needed?
i honestly don't know
i'm just going off a forum thread
Can you please share it?
Khronos Forums
Sphere tangents
Hi, i hope is the right section. I need to know how to compute sphere tangents. For the normals i saw i can approximate them with vertices coordinates. But for tangents i don’t find nothing. I did this: Vec3s triangle = shape.triangle.at(k); Vec3f vertex1 = shape.vertex.at(triangle[0]); Vec3f vertex2 = shape.vertex.at(triangle[1]); V...
I am trying to investigate, it must be possible somehow
Thank you very much!
Just give me a second, I am trying to understand
I see, basically one is getting the triange in 2D space first (in UV texture space, that's the difference, just to get the relative triangle as vectors)
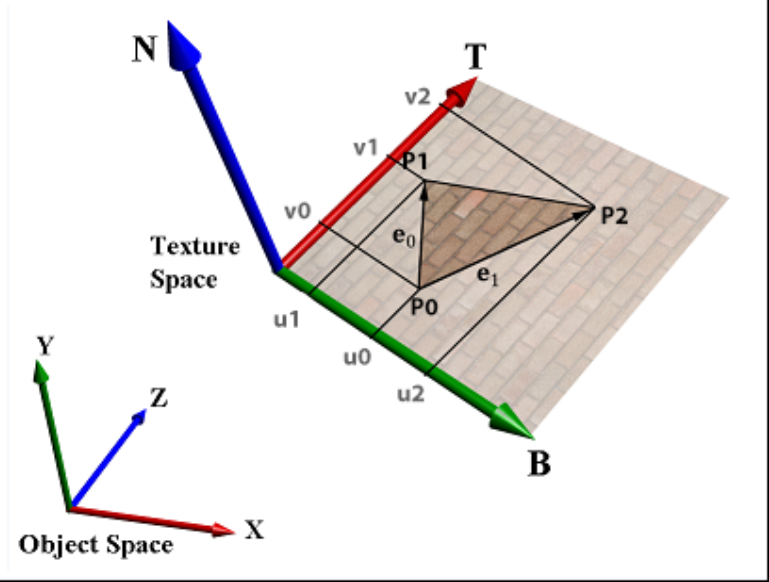
Suzuka Site|Tangent Space
In this post, I focus on reviewing the Lighting Models.
https://gamedev.stackexchange.com/questions/68612/how-to-compute-tangent-and-bitangent-vectors
This is by far the best explanation I found
Game Development Stack Exchange
How to compute tangent and bitangent vectors
I have a texture loaded in three.js, then passed to the shaders. In the vertex shader I compute the normal, and I save into a variable the uv vector.
<script id="vertexShader" type="x-shader/x-
<script id="vertexShader" type="x-shader/x-
I am trying to work out the iris calculations
But just to be sure, could you please code a check if the tangents computed are even the same?
Something like, if the tangents are roughly equal, print a log so that I can see it
Well, only if you want, if you trust the debugshader to interpolate tangents then the check is not needed
The iris calculations are a direct copy of learnopengl
Which explains the exact calculation in their normal mapping page
LearnOpenGL - Normal Mapping
Learn OpenGL . com provides good and clear modern 3.3+ OpenGL tutorials with clear examples. A great resource to learn modern OpenGL aimed at beginners.
I believe I have an intuition on how to implement per vertex tangent space
Let me work this through mathematically (and let me grab a water lol)
currently eating dinner, no need to rush
Ah great! Here it's 5 am lmao
So, since I wrote this (excluding eating lol), I had the same idea but just thought more about it to be 100% sure that it's stable and correct, I believe it is.
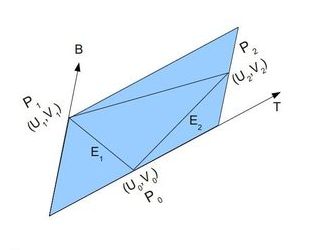
If you take a look at this, the blue plane is so to speak the tangent space. E1 and E2 are the geometric triangle.
The tangent space is used to map height maps etc to 3D space via a transformation matrix
What the Iris code does it is relies on the information that the normal vector is the geometric normal, which it is not for smooth meshes
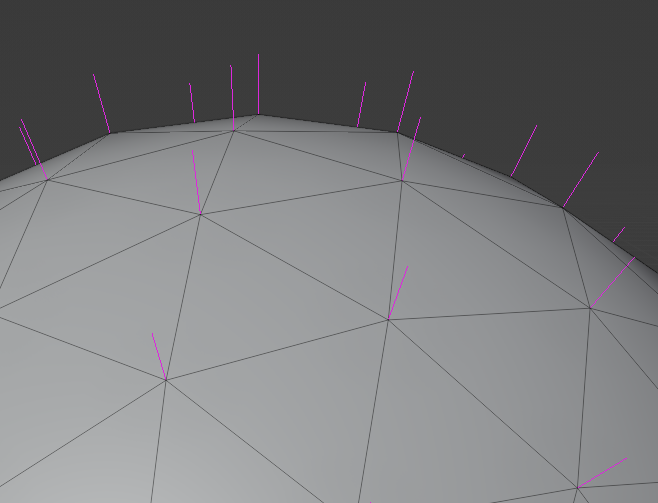
This is from blender how smooth normals look. You can see that for mulitple vertices in one spot, they point in the same direction, making the transition between edges smooth. All normals inbetween are being lerped by the shader
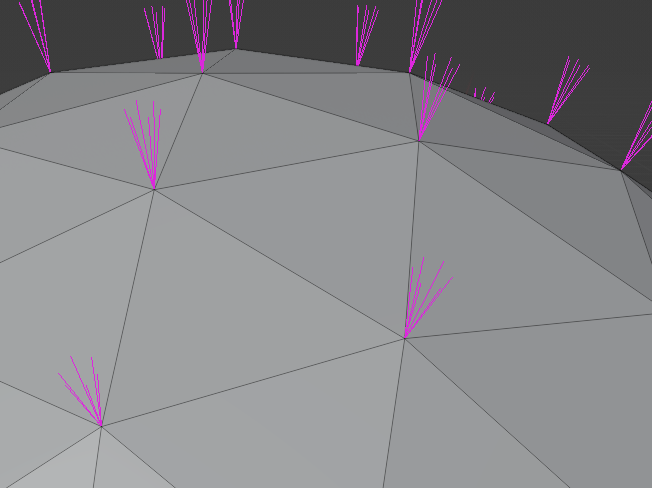
This is for flat shading, each vertex normal is different despite sharing the same spot, they point in the direction of the geometric triangle
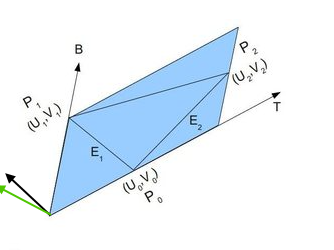
The black arrow is the geometric normal, the green arrow is our smoothly shaded vertex normal
Now, the Iris code builds the T and B vectors to specifically be inside the vectorspace that is being spanned by the geometric triangle (given it uses the poligons)
To adapt the Iris code to the smooth shading needs, I project the vertices to be inside my new vectorspan, spanned by the perpendicular to my smooth normal
(Hold up I am making a visualization)
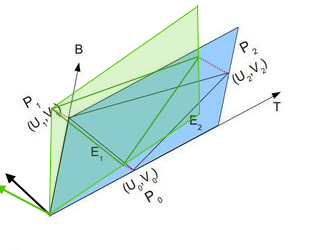
So, all we are doing is taking the points P0 P1 and P2 (vertices) and slide them along the normal vector direction until we hit the green plane, which is spun by our smooth normal vector!
This is easily done by a dot product and a recriprocal squareroot and some translations
Basically, we do project the point vector onto the normal vector, take the difference of the projected vector and the normal vector and then offset the polygon by that amount
And I (why I was holding back with this before) wondered if this may stretch the tangents in a way that would offset the UVs not as wanted. But no, it does not, it should work perfectly fine, because we do that for every vertex! The shader is lerping between those point tangent spaces, so in the end, point to point, the tangent spaces and UVs will easily line up and result in an accurate tangent space computation for smooth meshes.
This is pseudo code:
And this is the actual change in computeTangent, let's hope I did not make an error:
(hold up I will write it somewhat efficient)
why is it pseudocode? That looks valid
I do not wanna rely on Vector3f, will do everything component wise
fair
Probably still not as efficient as last one, so I’ll leave it for triangles specifically
Actually it's 18 multiplications and 9 subtractions more
No more than that, should be as efficient, but yes Id also say only for triangles
That should be it
Let's hope it works, it's 6 AM and I didn't have lots of sleep last night either, so let's see haha
time to see
Efficiency wise, if d0, d1 and d2 are basically 0, we can skip the whole offset part
:nervousIntensifies:
i'm replacing all usages for the test
Which test usages?
i mean i'm replacing terrain and all
Ah right!
Blazing fast
How do you do that
building takes less than 5 seconds on our toolchain
lol
still, you got like 50 words a sec lol
OK SO
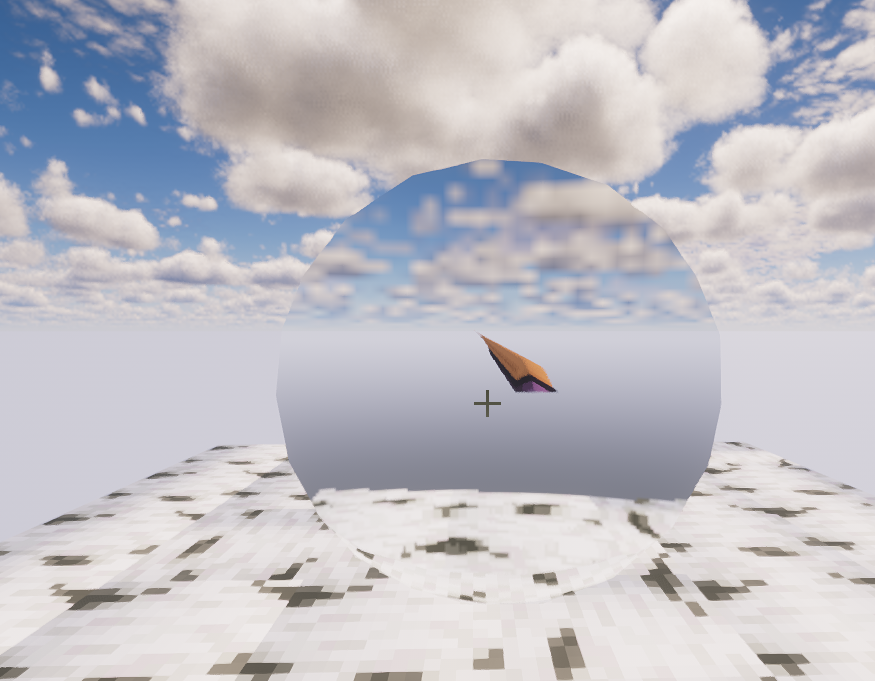
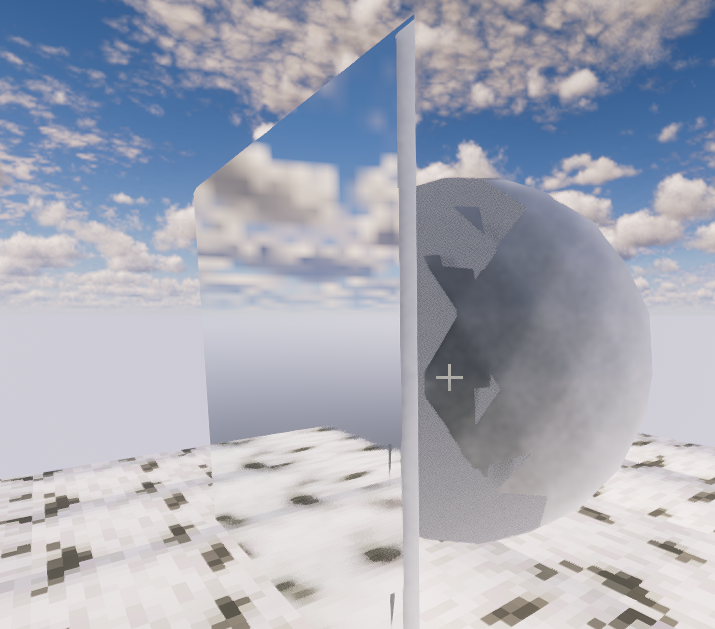
ALMOST
This is Kappa btw
Let me check the other shaders
Ok, interestingly the supertester still shows flat tangents, that doesn't make a whole lot of sense, but well
wait
should i have put in the change that makes the tangents per vertex
i reverted it
Yes please
calculateTangent per vertex and keep the smooth normals
👍
@Sinan
Huh, that's very interesting
wait
i think i got the code wrong
it's grabbing the same normal 3 times instead of grabbing the 3 normals
Ah alright :D
Was that the same behaviour in the beginning?
yes
i think
maybe
i don't know
Let's see :)
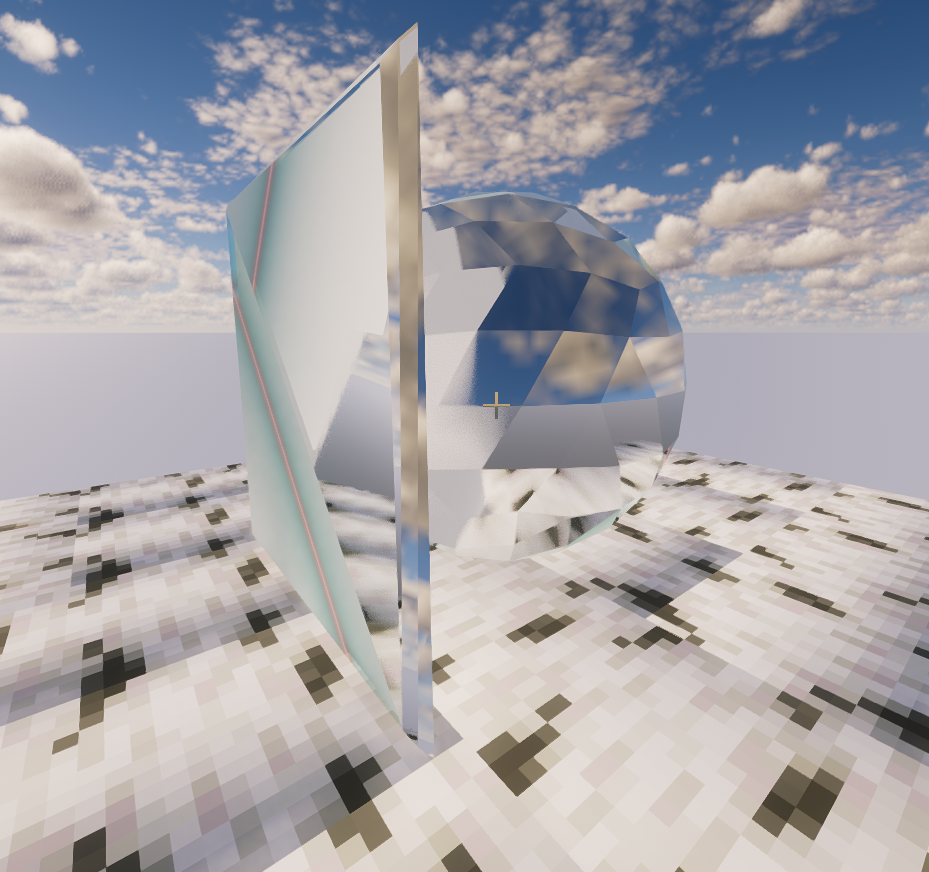
Hmm
good enough™️
xD
how does the flat mirror look
Idk why it's still flat
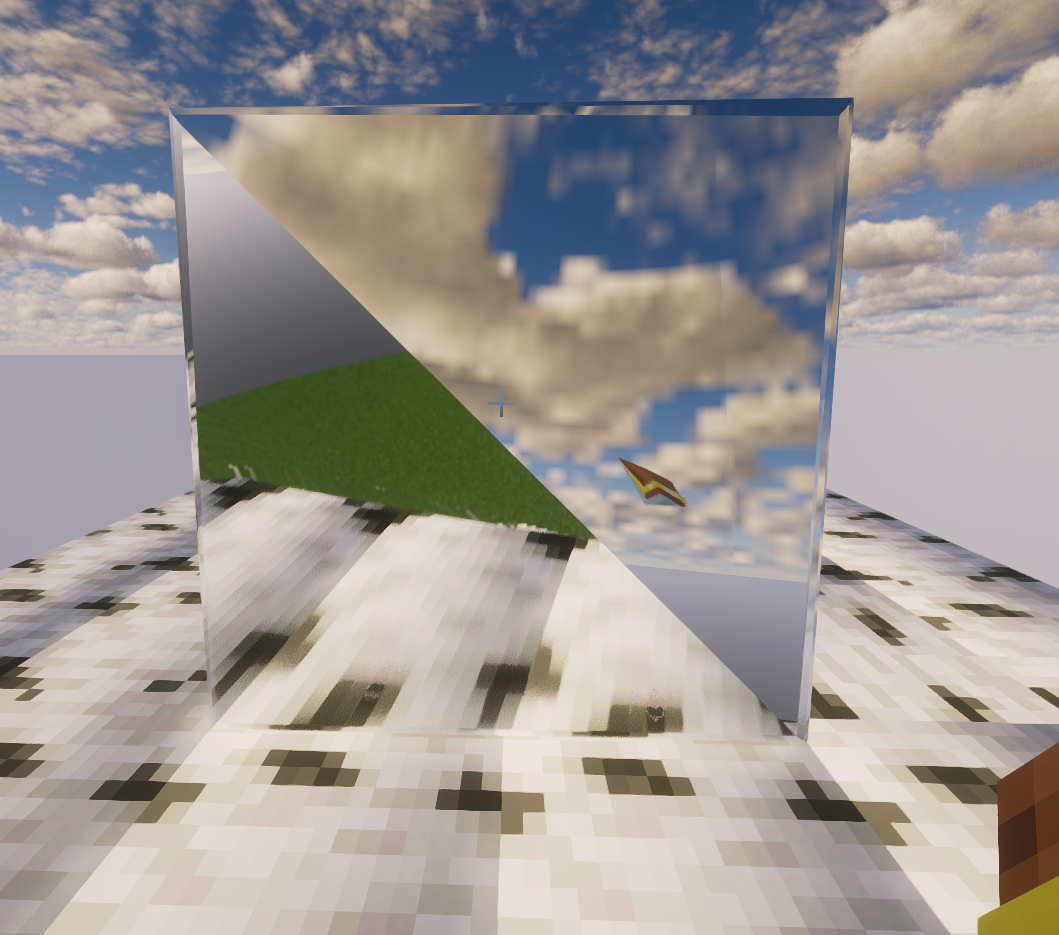
It looks like it's still only fetching the tangent from one vertex
Let me try something
Is that the latest code I got?
yep
packedNormal = buffer.getInt(nextElementByte - normalOffset - stride * vertex);I dont understand much about that (what is stride) but it seems to be fine
and the addition to computeTangent
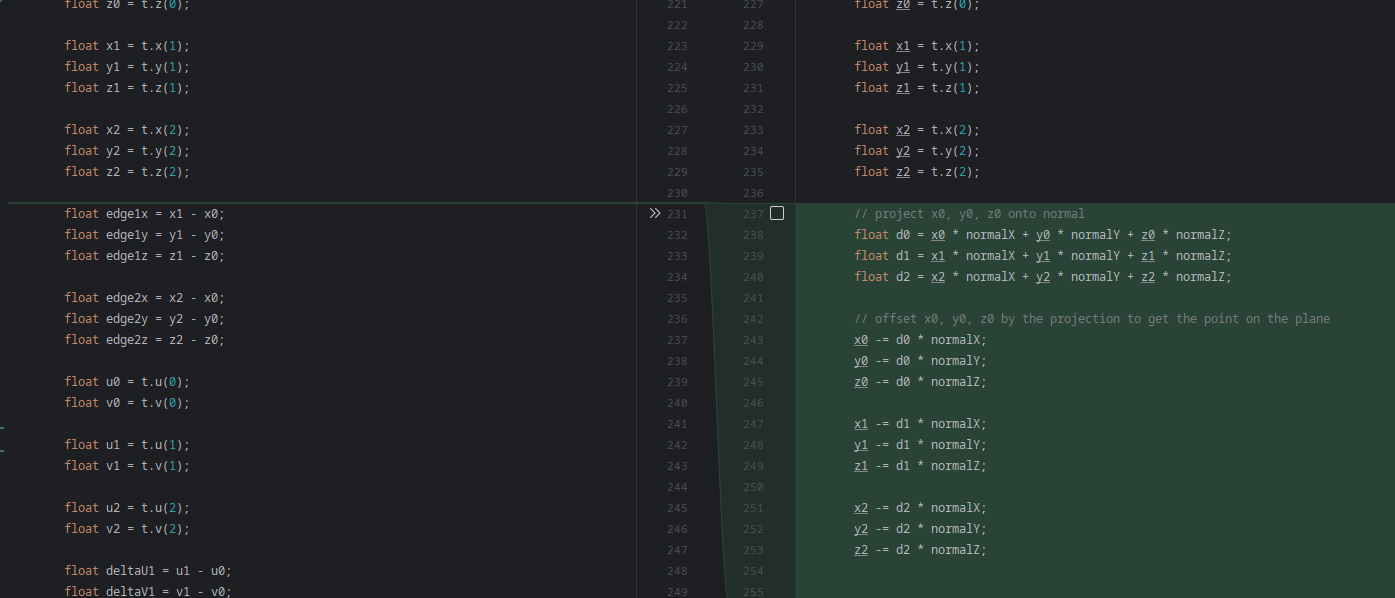
the size of a vertex
so it's basically displacing by the amount of bytes in a vertex
Ah okay
Argh idk why it's doing that, being flat
I just loaded an old iris and it looks exactly the same
i think it's up to rre (the creator of kappa) to try to smooth out tangents prob
Which leads me to believe, does kappa not interpolate tangent?
maybe not?
i know there were similar problems at some point
lemme go through the backlog
Yeah, but why is supertester not working
But take a look at this
if you mean across triangles no
Supertester:
you cannot interpolate across triangles, that's not possible
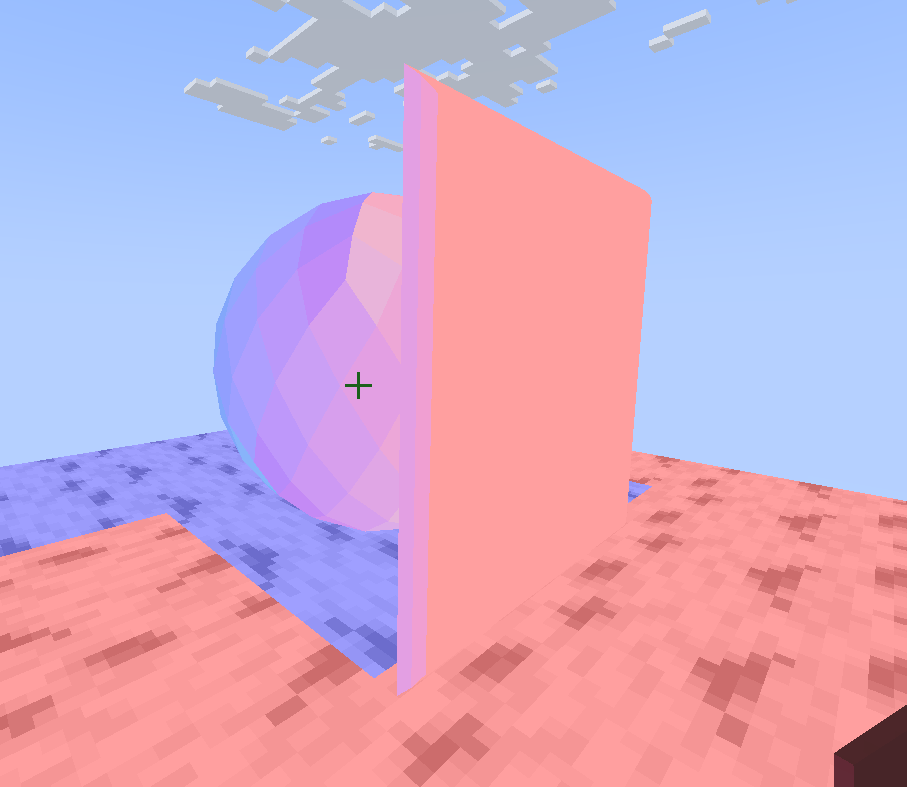
Flat mirror is at least flat
yeah, with the flat mirror i'm pretty sure something's wrong in kappa
with the sphere the tangents are pretty obviously pixelated
This is BSL (before the iris tangent recalculation, BSL ignores tangents alltogether)
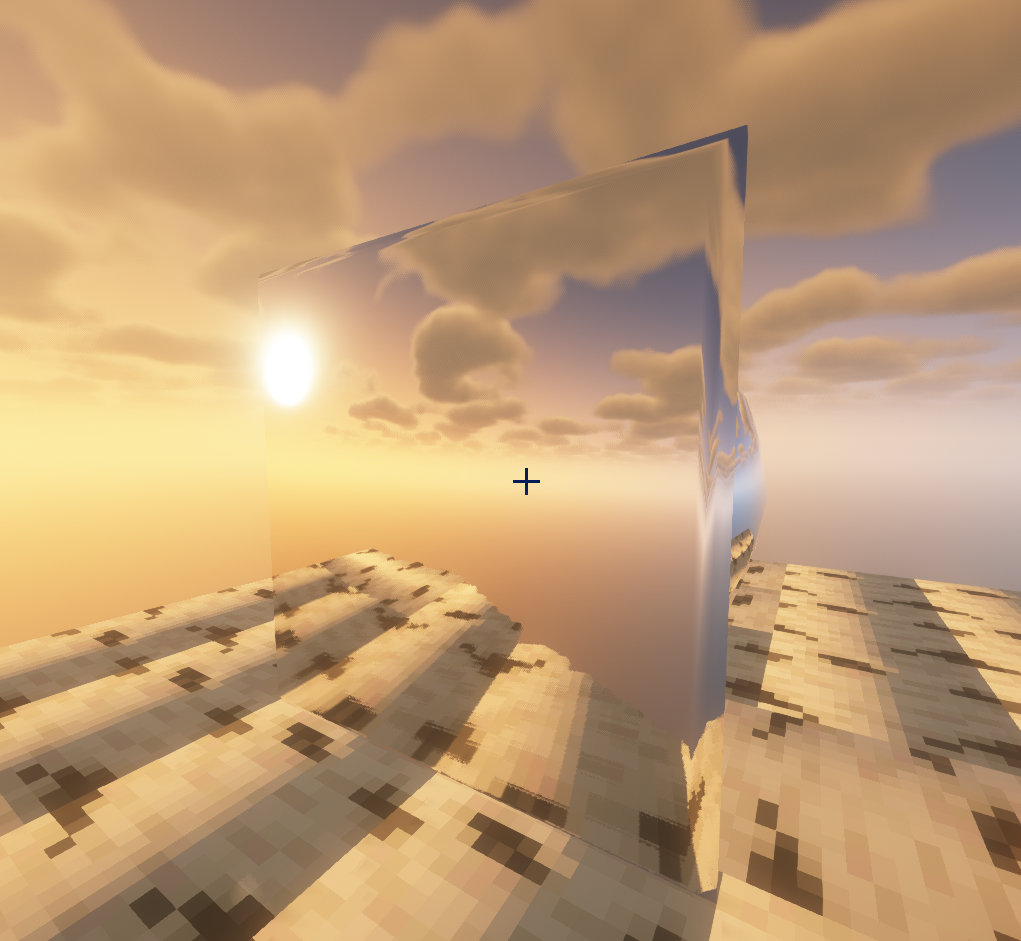
bsl can't ignore tangents, though?
time to grab bsl and see the code
Ah Im sorry, I mean at least it doesn't base it's normalmapping offof it
Yayyy
but that's... a critical part of normal mapping
you get extremely scuffed normal mapping without it
Can we test setting the tangent to a fixed value?
Some random vector that's the same for all vertices?
ok yeah tangent is definitely used in BSL
sure
what value?
1,0,0
Or something
w value?
(if you know what that does :ioa:)
it's just that BSL constructs everything into a TBN matrix
oh sry also 0
before messign with it
But it uses the T for it right?
the
B
is T cross N
iircYes, but why does BSL work
I guess we will see with a fixed tangent value
i don't know
@RRe36 can probably look at this in the morning, idk
(bsl has a perfect mirror made out of triangles, but kappa has problems with shading seemingly due to interpolation, see photos above)
the normals are smooth shaded but the tangents are flat shaded due to being calculated on the fly
Apparently, Kappa does not care about smooth per-vertex normals and perhaps bases it's own normal (not normal map, just displayed normals) on the tangent vector space rather than on interpolating between the vertex normals, other shaders like BSL or Complementary do also take the tangent into account but somehow are able to smoothly interpolate between normals despite wrong per-vertex tangent spaces
wait
i think i remember some option for this in shader settings
go to terrain and flip on vertex attribute fix
see if that does anything
i think it was for entities only though
Is that the new fixed value tangent? Somehow in the shaders it looks exactly the same, only in supertester it shows me the fixed val
I am using the entity solid and entity vertex provider
I tried the vertex attribute fix, when I toggle it on, it makes the mesh render flat
if supertester shows it it's the real value
Yes, supertester shows the real value, Kappa looks exactly the same with the fixed tangent
But BSL breaks
that's a problem, but it's likely not the problem here
but it's probably related in some form
the tl;dr is long ago (3 years ago) optifine was notorious for providing just completely screwed normal and tangent values on entities
so shaderpacks made fixes in the form of calculating their own
I didn't know what to do, should I switch to solid in genereal? Can I then expect rethinking voxel shadows etc?
some shaderpacks just kept it that way ever since, even when it was fixed
i don't mean it's a problem with your renderer
I totally see...
it's just a historical problem with entities and normal mapping that shaders worked around
and now the workaround breaks things
i can't say if that's what's happening here
Just for the record
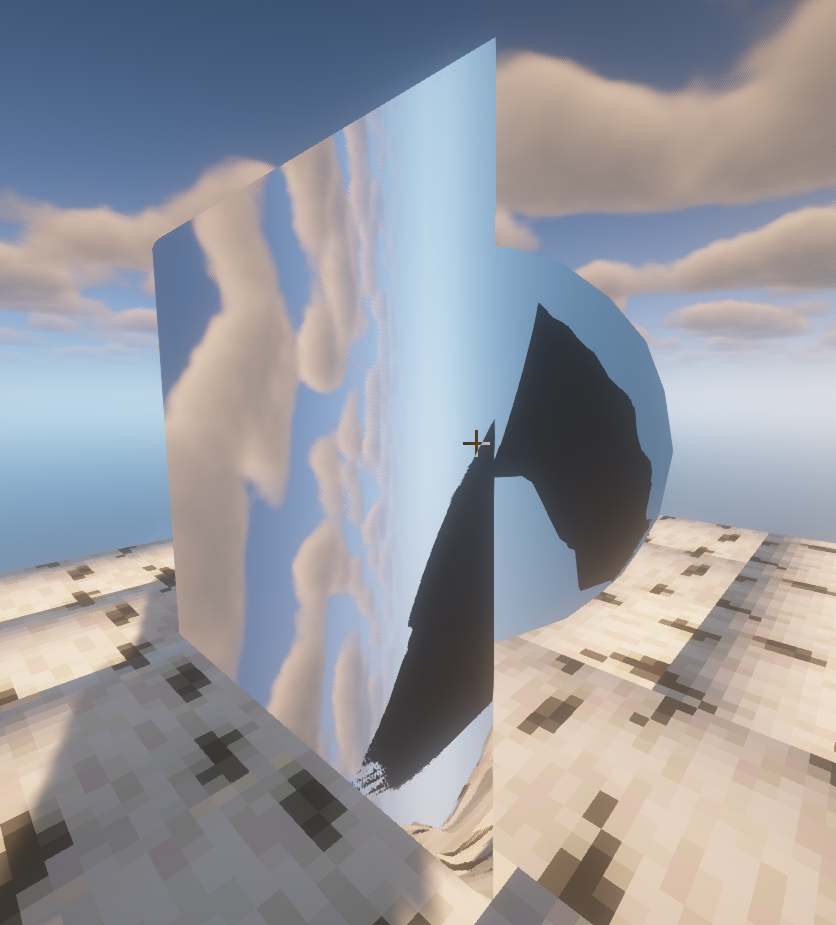
BSL with fixed tangent space
yep, that's tangents being screwed
exactly what i'd expect
if kappa is not as screwed that indicates it's making it's own TBN
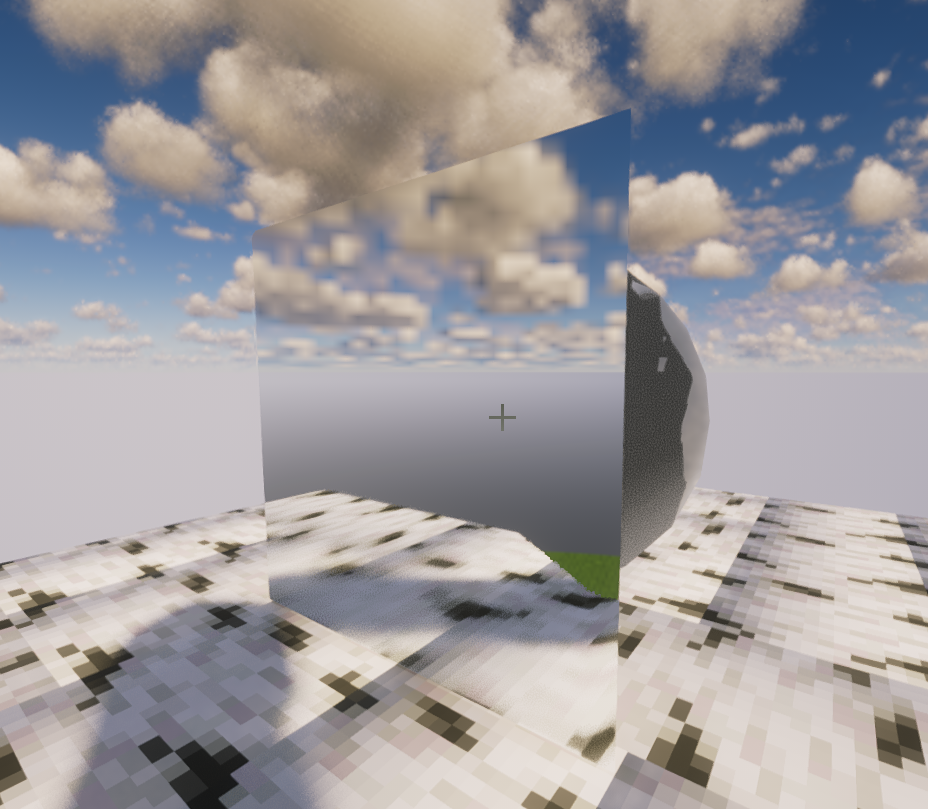
Kappa with fixed tangent space
Notice that now that it's facing in one world space axis (I suppose), the mirror is flat and from the other side the sphere works (as a flat mirror)
For completeness sake:
i found the code in kappa that makes it's own tbn
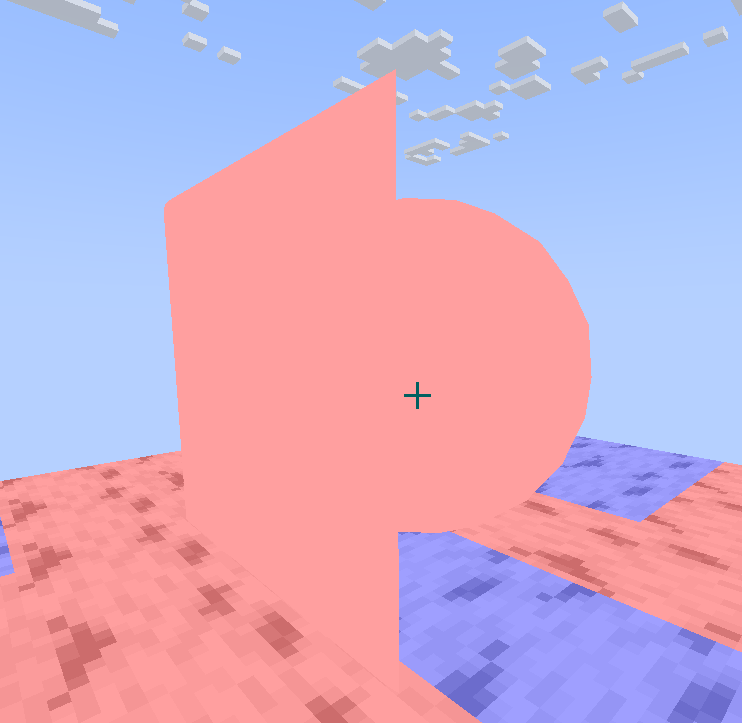
(same from all angles)
this is the code in kappa
but it should be off unless
tbnFix
is on
which is activated by vertex attribute fixThat makes sense, rendering everything flat
Can you please test something?
sure
I wanna make sure that even supertester interpolates the tangent space
supertester does the bare minimum
debug = tangent * 0.5 + 0.5;
where tangent = normalize(at_tangent.xyz)
interpolated across the triangle
Could you please set the tangent space matrix to be a randomized
Oh really, hm
normalmatrix?
that will probably screw with much more than you want
including the game visual itself
By sending random tangent spaces per vertex?
the normalmatrix is the model view matrix inverted and transposed
For simplicity, randomly decide between 1,0,0,0 and 0,1,0,0
it's used in calculations outside normals
ok
No that's not what I meant
you want every other vertex or completely random?
I meant the tangent space output to be nrormalized
completely random
But 1,0,0,0 and 0,1,0,0 should at least give some info
These are very bad tangent space values, as in they will project everything into 1d space, but meh it's about seing if interpolation works
this should do it
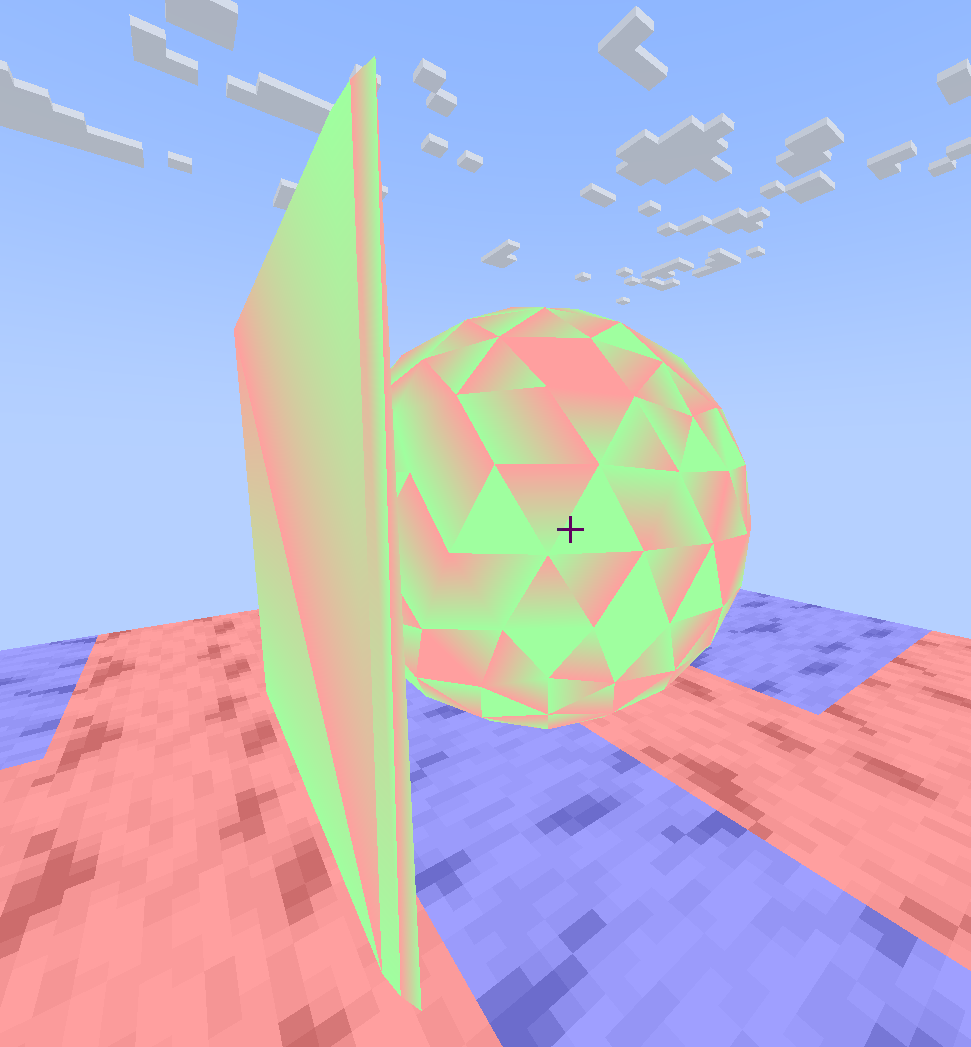
Yuuup, interpolation works
This is the old nonfixed tangent space code
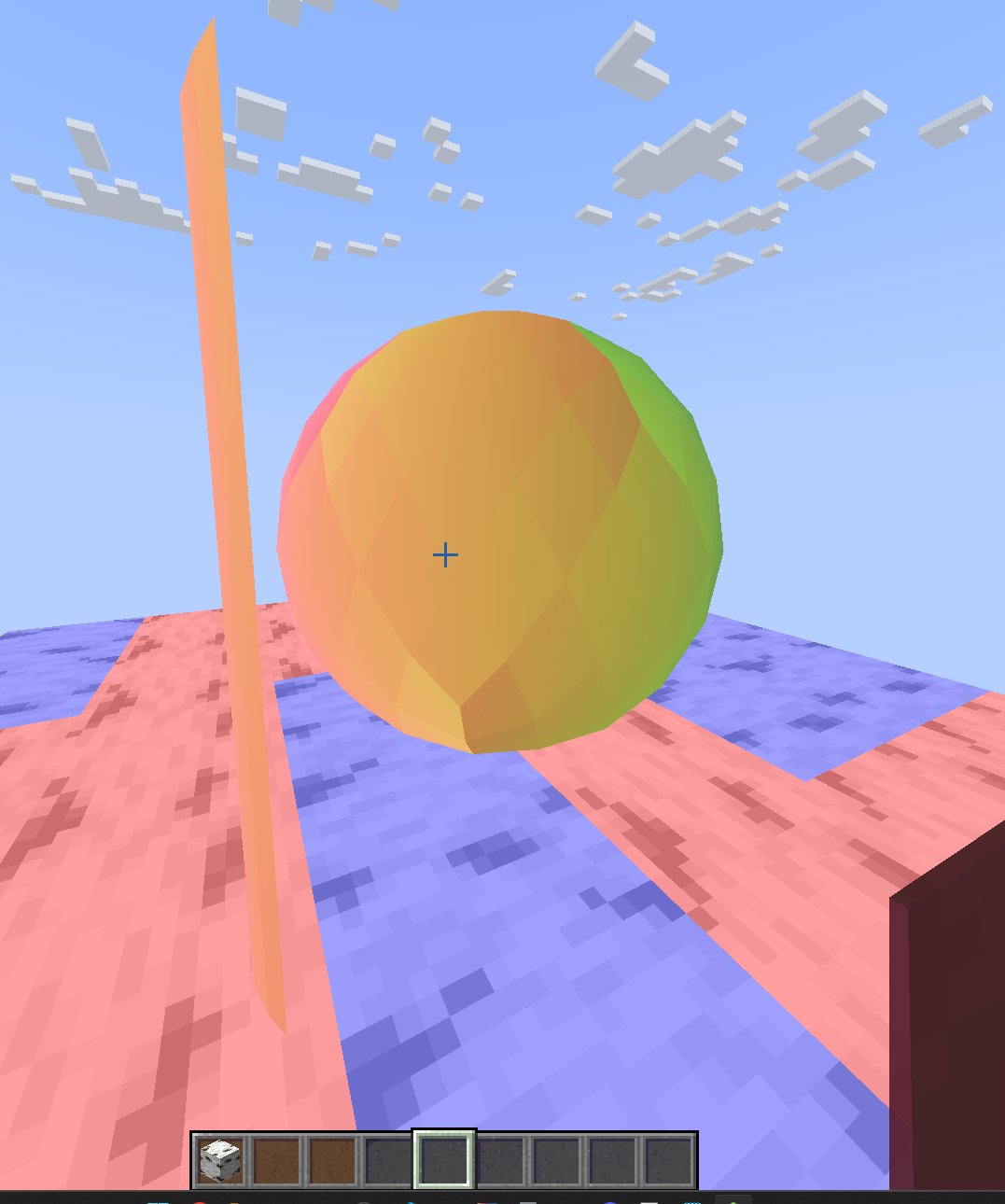
It does do interpolation
Which could explain why BSL etc is seemingly working
going to sleep soon, tell me if you have something else for me to try
I am out of ideas on what we could test on the Iris side...
I wish you a good night and thank you for your awesome help!
no problem! i can help more tomorrow if needed
(I hope you disabled notifications before going to bed)
I think the tangent fix we did works beautifully, the picture below shows flat tangents, compare them to the tangents I sent above and it looks correctly interpolated
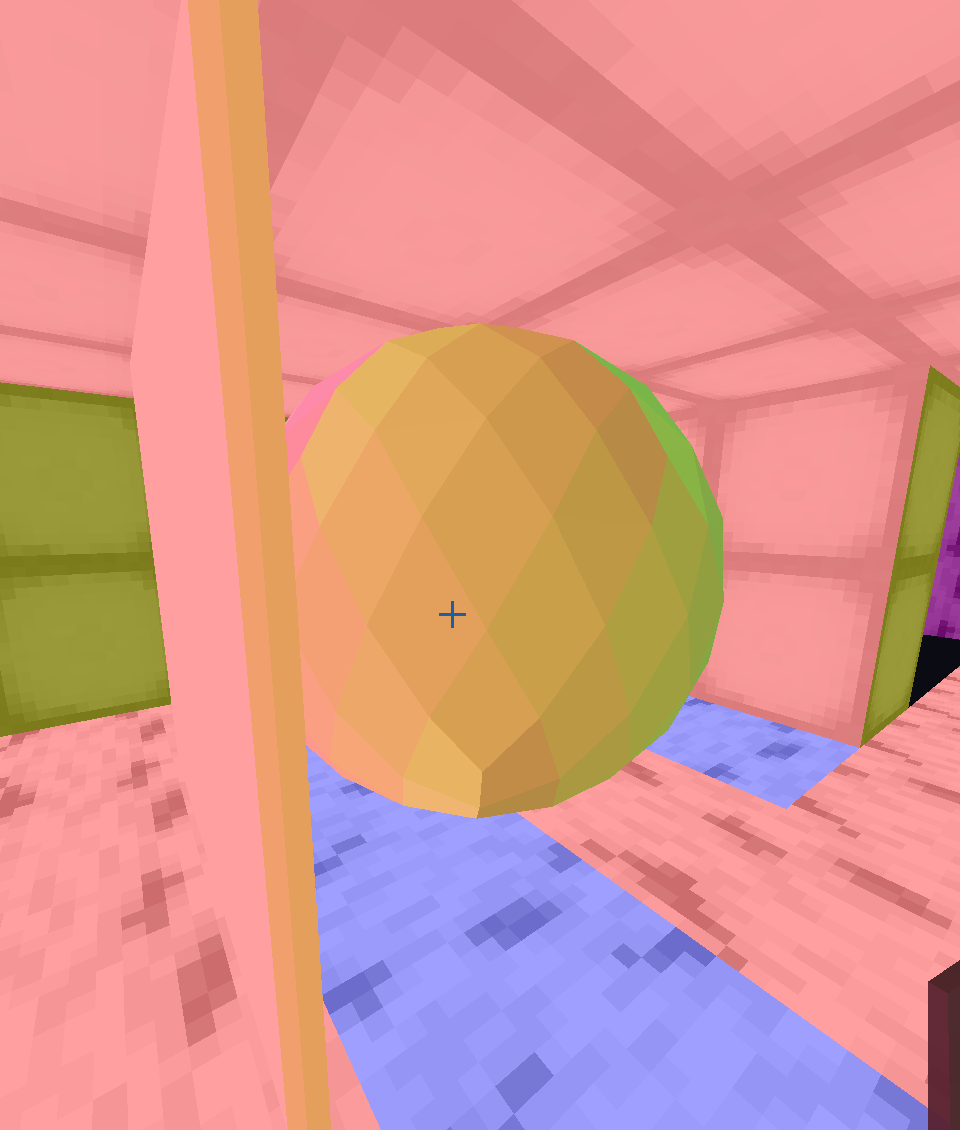
Which explains why the other shaders work flawlessly
@RRe36 Hey, I just worked with hedge hog on implementing per vertex tangent space, I modified it by simply projecting the vertices on the smooth normal plane instead of the geometric normal, which supposedly works
BSL and Complementary shaders now support smooth mesh shading:
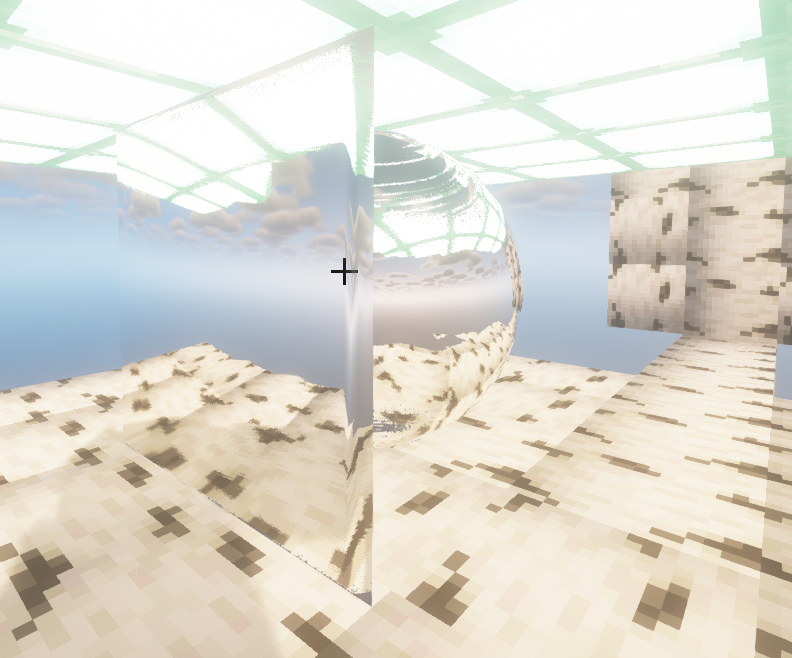
But Kappa (which I totally LOVE, I have never seen a shader like it, true metallics, reflection probes and just all post processing effects one would ever want with the highes quality, JUST A DREAM COME TRUE) does this:
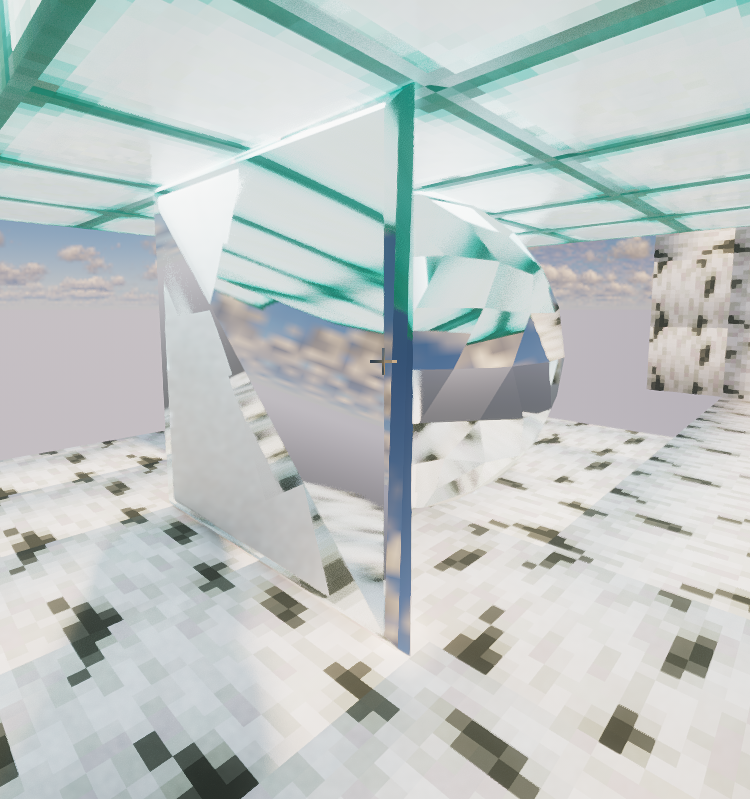
Perhaps Kappa is only using the tangent space of one vertex and using that for all 3 vertices? That would explain the "broken" mirror
@IMS wouldn't it be wise to ask for a forum channel in slabs for developing shaders for iris?
@IMS Hey! :) I wanted to ask, what did you do to the packing normals to 0, did you just remove the line to make smooth normals work? I am preparing the tangent space per-vertex PR (I wrote it with an argument overload boolean so that only on tris this gets executed, only in the right place the boolean overload gets called. This way it's maximum efficiency, it's only 19 mults and 8 additions overall only for tris.)
what do you mean by doing the packing normals to 0?
to get the packed normal
buffer.getInt(nextElementByte - normalOffset - stride * vertex)
to unpack it
packedNormal = NormalHelper.packNormal(normal, 0.0f);
The code calls this after the comment out of the tri normal recalculation
yeah, I had to change that
put that in the quad only code
Ok I would place that where the line I sent was for tris only right?
and where the tri normal recalculation took place, do this
packedNormal = buffer.getInt(nextElementByte - normalOffset - stride * vertex);
Okay nice sure :)
I am so glad that it all worked out beautifully! I am excited to know where the Issue with Kappa lies, after solving that, Iris has full support for custom smooth meshes! :blush_AE:
Does that look fine to you?
Oh and also, does Iris have an API call event for when the shaders are done being reloaded?
sadly, no
no, since
normal
isn't computed by packedNormal
so you need to unpack it using my unpack functionAh of course!
Now okay?
that works
though it'll certainly not be fast lol
this actually doesn't really matter because Sodium codepaths don't use BufferBuilder and use a faster alternative
In what sense?
this is per-quad
anything you do gets multiplied hundreds of thousands as much
so everything is expensive
You mean
packedNormal = NormalHelper.packNormal(normal, 0.0f);
? It was like this before, just for all quads and tris, right?
https://github.com/IrisShaders/Iris/blob/168599f79a6877ee27c296f22fba126612940a09/src/main/java/net/coderbot/iris/mixin/vertices/MixinBufferBuilder.java#L253it's probably fine honestly
don't worry
replace normal = new Vector3f with normal.set
because fun fact; creating a
new
object is horrendously expensive
which is why it's always avoided in hot pathsAh okay!
looks ok
I don't quite get, why would that be expensive and per quad?
per-primitive i mean
not per-quad
so triangle, quad, whatever you're using
I still don't get what I introduced that did more operations per primitive
basically, everything you do in BufferBuilder needs to be aggressively optimized otherwise your performance dies
the unpack functions are more operations
but like i said, it's unavoidable
so it's fine
Oh okay, but that's just for tris now right?
only if
if()
wasn't expensive too :Waaa:
but yeah it's fineOhhh, you mean the vertexAmount == 3 in the computeTangent
Hold up
just make a new computeTangentSmooth
Yeah I wanted to do that
and use that in the if vertexAmount == 3
:)
Okay!
Wait, I think this is missing from my code
Let me try to write something efficient with as less ifs as possible
Is a new for loop introducing any overhead?
I would do a per-vertex for loop inside the if(vertexAmount == 3), storing the tangents in an array (and fill the array with all same quad data otherwise)
Why not just move the last for loop into the if statement?
and duplicate it
Oh sure!
Does this look fast? Lol
You can remove the putInt for packed normal on tris
because it’ll always be identical
otherwise yeah, it looks good
What do you mean?
Doesn't this then remove the smooth normals?
you’re doing buffer.putInt on packedNormal
but packedNormal is retrieved from buffer.getInt
Ohhh, I see!
Shall I remove the line or comment it out?
Remove it since it’s in the quad path
Ok great, I did the PR!
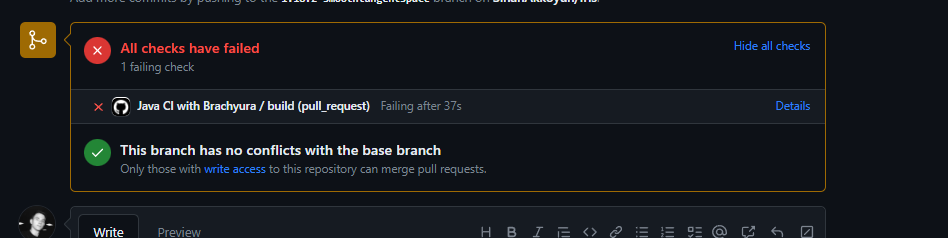
Wait I did something wrong
Oh these were not in the code...
@IMS Should I add the functions to MixinBufferBuilder or use
normalX = Norm3b.unpackX(normal);
normalY = Norm3b.unpackY(normal);
normalZ = Norm3b.unpackZ(normal);
These?
import me.jellysquid.mods.sodium.client.util.Norm3b;
Don’t use those
They’re from sodium, so if you launch the game without sodium it’ll crash
Ah right, I am dumb
ill add em to normalhelper
Sorry, now the checks check out :flooshed:
Committed it
Okay, the whole custom mesh rendering journey is almost over
There is just one thing to do, I discovered UV mapping issues, but I think it's closely related to the shaders ways of using the tangent space
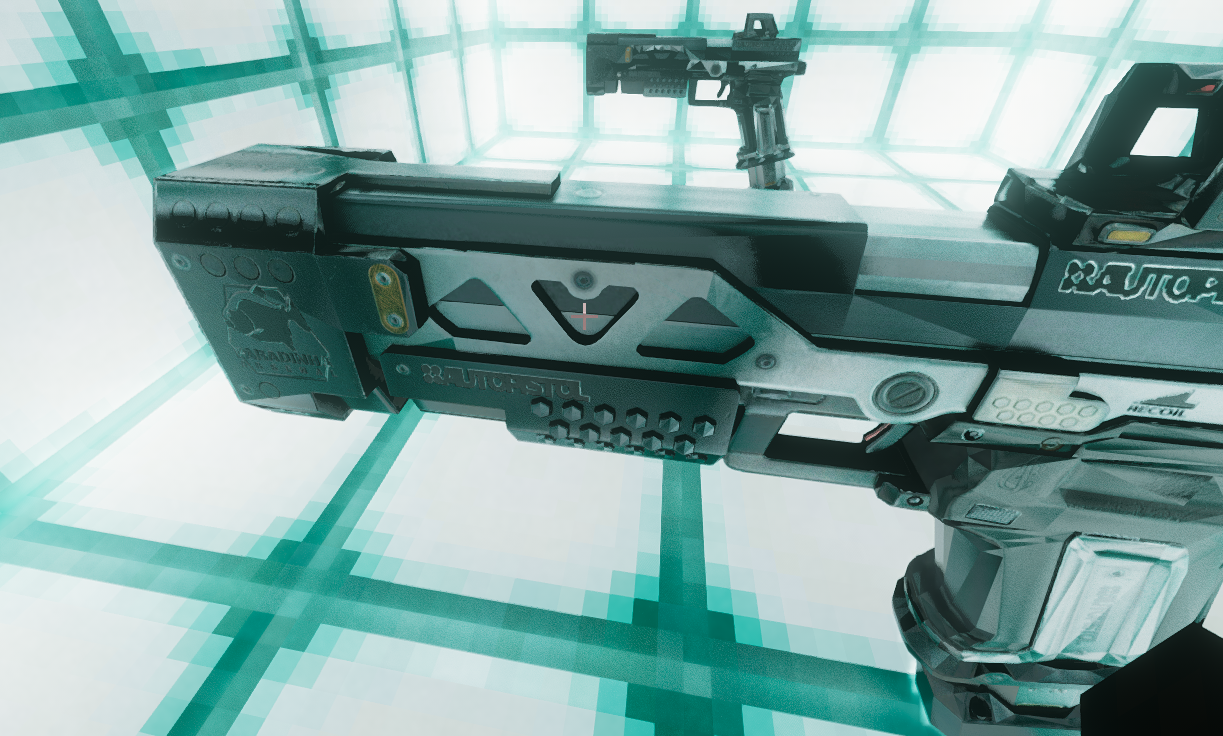
In this screenshot with Kappa (so, don't mind the flat rendering despite the mesh being flat), the specular map maps perfectly fine
(hold up, I need to construct a new texture)
However, in BSL the specular mapping is all over the place
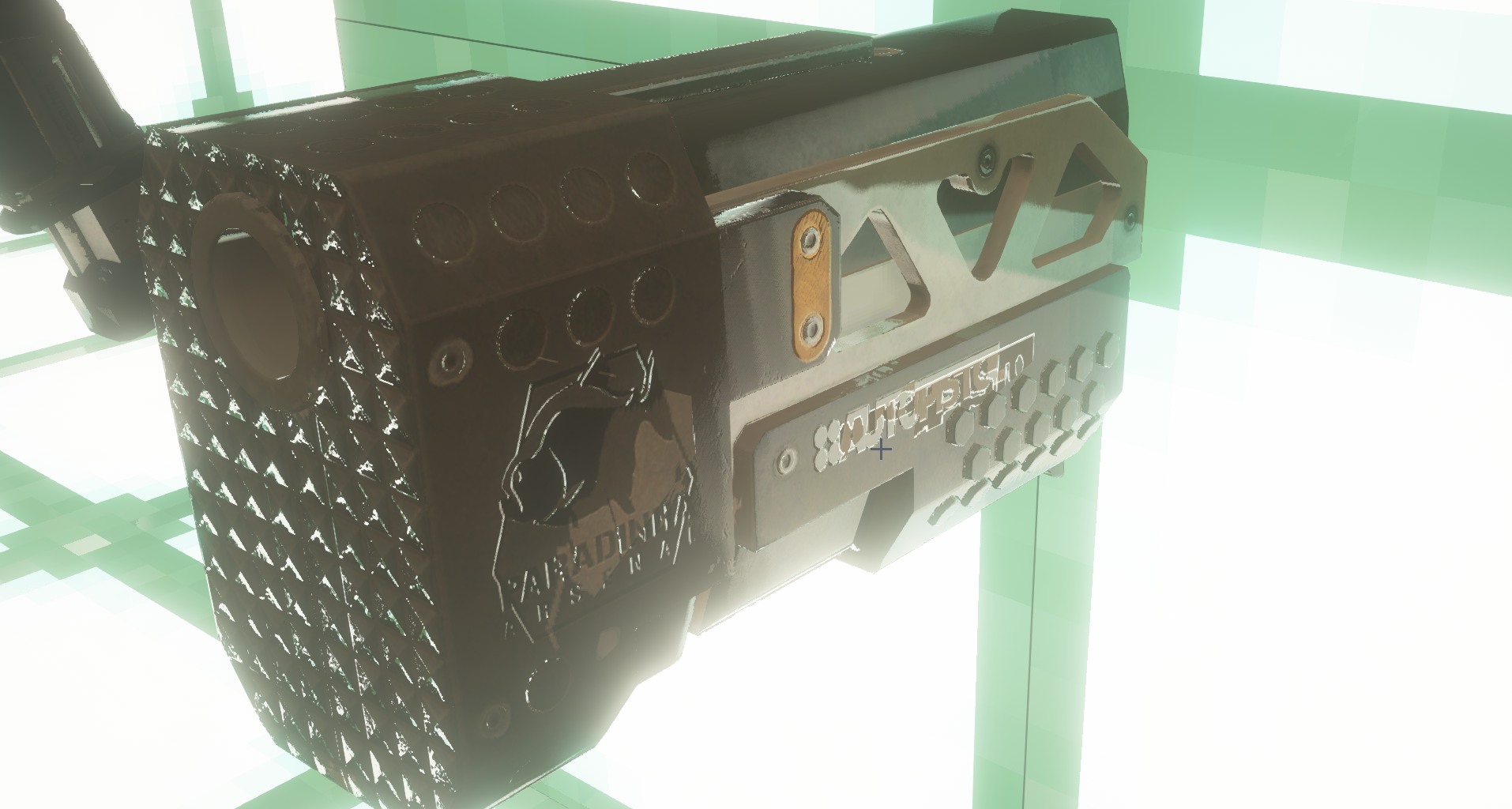
That's the whole issue. Now here is some side info:
When I enable parallax occlusion mapping, every shader messes up all of my UVs. Take a look:
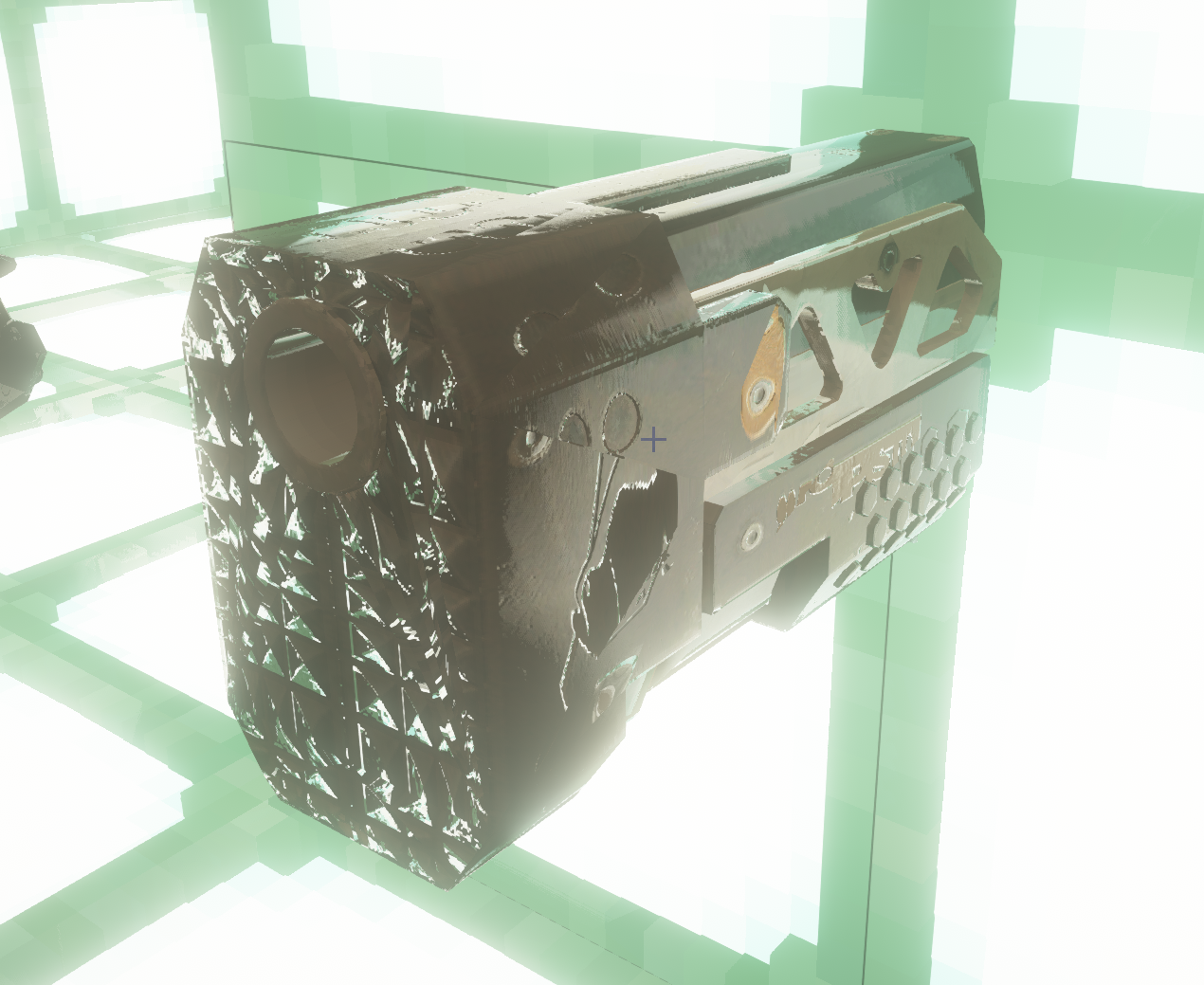
With
Parallax occlusion mapping
all (c, s, n) textures get displaced in the same pattern as only the s texture without parallax occlusion in BSL only:agony:
Id' say if we fix the POM issue in general, the specular map will fix itself in BSL
:Sob_AE:
i don't know what that could possibly be
if the tangents are right
I sadly can not enable parallax occlusion mapping in supertester
i rewrote a lot of your PR and merged it
https://github.com/IrisShaders/Iris/commit/9b04558f60538e5523031bf4696f38d7a8587441
(it now uses a dedicated NormI8 class to handle normal packs)
Oh awesome, thank you! :)
I wanted to ask, is there an API or event that I can register to rebake my meshes on switching from shadern on vs off?
I also wanted to ask, which of those shaders gets used when I draw with the ENTITY_SOLID_PROGRAM aka getRenderTypeEntitySolidProgram()
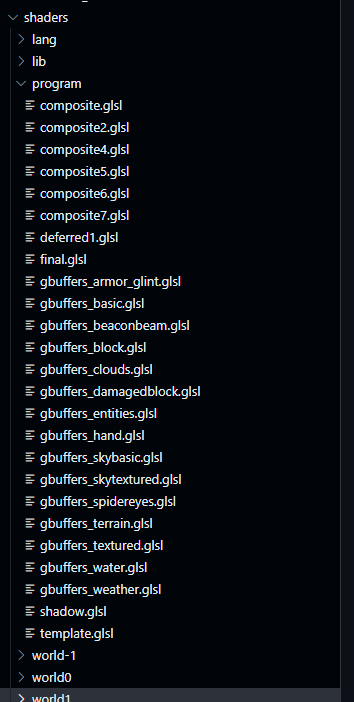
Is it the gbuffers_entity.glsl?
I just wanna know to look into the code and POM implementations
Yep
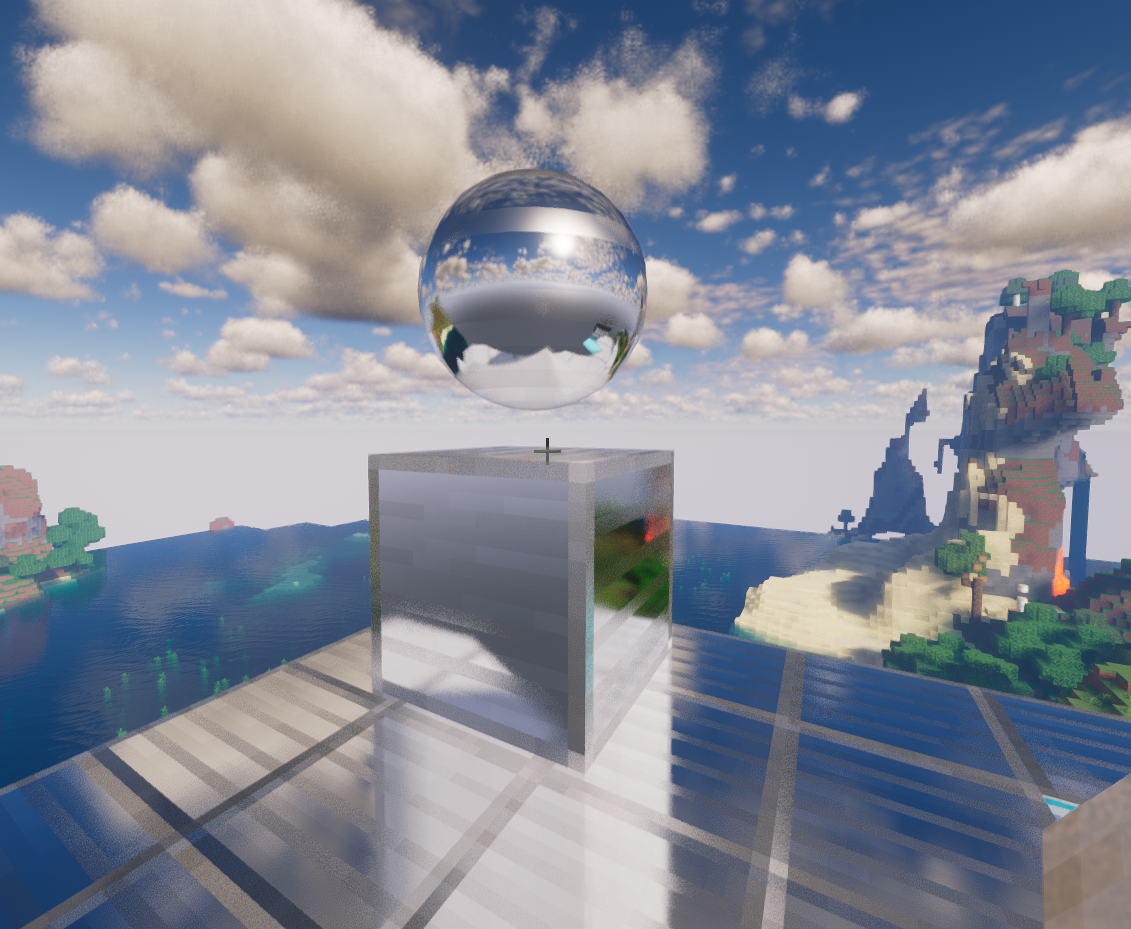
Yay I modded Kappa :D
was a simple removal of
flat
for normal and tbnThis is epic, I was looking for something like this for ages.