Test ASP.API controller with xUnit: can't assert Type
Hello 👋
I hope my question is not too dumb but I m stuck on the testing of my API controller. (It is my first test project)
Me controller looks exactly like this :
And i am trying to test the first case : Recipes.Any() = false, in this test :
But it doesnt work. Both my IsType<NotFoundResult>(result) and Equal(controller.NotFound(), result) fail. How could i test this please? 😁
51 Replies
Oh yes maybe i should say that i made a "test" recipe service in Tools.RecipeService, looking like this :
Test fails but they look the same to me 🥲
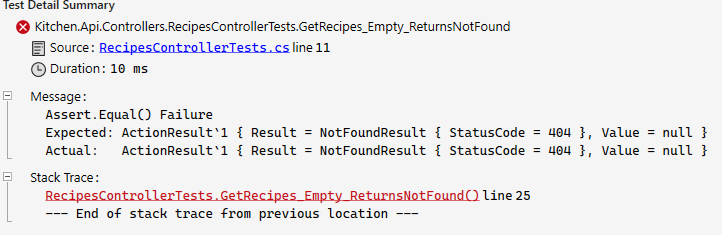
Ok sorry but I found the answer after being stuck for hours, it was as simple as :
I need to take the .Result of it 🤣
Or better yet,
await
itWhat do you think i should await ? I dont understand
The thing you're trying to
.Result
I think I'm blind I don't see a .Result
"ActionResult" does not contain a definition for GetAwaiter it says
var result = response.Result
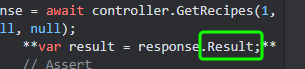
Ah,
response
is not a Task
?i tried to put it in bold with two ** but it didnt work 🤣
It's just a completely unrelated type that has a
.Result
property?it is an action result
If so, carry on
ok ok :p thank you !
I'm totally blind; I don't see that
Here 😁
oh okay
NIT: I hate testing controllers this way
how would you do it ?
WebApplicationFactory and call the endpoint
https://learn.microsoft.com/en-us/aspnet/core/test/integration-tests?view=aspnetcore-7.0
I personally will only unit test things that provide a quantifiable advantage
The tests you're writing just make code harder to change and add negligible value
I had doubt that unit testing my api was really useful, for real
I thought about integration test but it seems a step harder
Maybe it will be more useful than unit testing
I see
so, by quantifiable, let's say you wrote conversions for dry ingredients from metric to imperial and vice versa
that's worth testing
Yes i have none of that haha
I get that; but if you did
lets say that you had a recipe doubler as well
so more ingredients might change time sorta thing
and you have a really smart way to do that
totally unit testable
Ok thank you a lot i see better now
controller? meh; who cares; just call it like an entry point to your application same as a user would
it can be harder
May i ask you, when you do integration tests, do you test on the real DB ?
I was thinking of giving test data to my test project, using copy of my Seed class that seeds my DB with entity framework
yesnt
WebApplicationFactory has some tools to swap things out; it's worth some research
so swap out the db for sqlite or something ( there are known compatability issues for some edge cases )
Ok thank you @Ruskell McCheese I will make integration tests, honnestly thats what i wanted to do at start 🤣 But i was kinda afraid the step was too big since i didnt make any test project yet
but in a pipeline for instance; I might spin up a db in docker and seed with data and test against that
docker ❤️ i need to try this too
that s a really good opportunity here
some folks go for various "cobra" metrics; and it's a separate issue
i m HYPED for real
cobra ~= perverse incentive
so like, peeps will say, you need 90% code coverage; now you're in perverse incentive land
meaning you'll do a lot of silly things to get there that aren't valuable just to get there
( not quite a perverse incentive, but )
oh so 90% is already too much if i understand well ? and you can use Cobra to get to the 90% ?
The cobra bit, is that back in day in India, they started paying bounties on cobra snake heads, so now, perversely I'm going to just raise snakes and kill them for the bounty
so at some point someone will say, "hey this is a bad idea we're just creating more snakes"
and stop paying bounties
so I'll let all my snakes go
so now we have a larger snake problem
oh ok i see
i will keep that in mind while doing my tests
so using unit tests to get to high code coverage, now code is very ossified and hard to change
testing the most important, where the bugs are likely to be existing, right ?
ossified == turning to stone
yes its like in french, os = bone
ok nice
This is the exciting part about something like web application factory; when you hit your controller, you're just going to also test everything in that stack
it looks like a lot of fun, i can learn docker and sql lite and the tests at the same time, 3 birds with one stone 😮
with some creativity you can create tons of scenarios from a single test... Theory supports other types of "inputs" more than just inline data
and suddenly you have hundreds of tests
for scenarios that actually make sense
i was thinking that my unit tests of api endpoint was kind of useless 🤣
https://www.thomasbogholm.net/2021/12/01/xunit-using-theorydata-with-theory/
they can be valuable, but that will come with experience
Yes i guess, but not with endpoints like mine i think
Thank you for all the resources and info
hey no problem
NB: this is a highly opinionated area
results may vary
I see, but i know it will help me learn new things so it will be helpful to make tests that way, even if not every one agrees
plus, its kind of what i wanted to do at start haha 😅
Just dropping in to mention that if you are interested in using Docker to test database related stuff
Testcontainers
is a really cool package to check out
makes creating and cleaning up Docker images real easy
🙂I will check this out, thank you 😉