ā (solved) dot net cannot send post request to my endpoint
Hey guys, need some help understanding why my dotnet app is not working:
I have such js frontend that sends a post request to my 'localhost:8080/controller'
I have such coontroller in my dotnet app:
69 Replies
here is my program.cs file:
Your url is wrong
in js file?
Your controller sits at
/login
YesI thought:
[Route("[controller]")]
means /controllerNo, that would be
[Route("controller")]
The brackets format the class namegotcha
well, changed it and still doesn't work
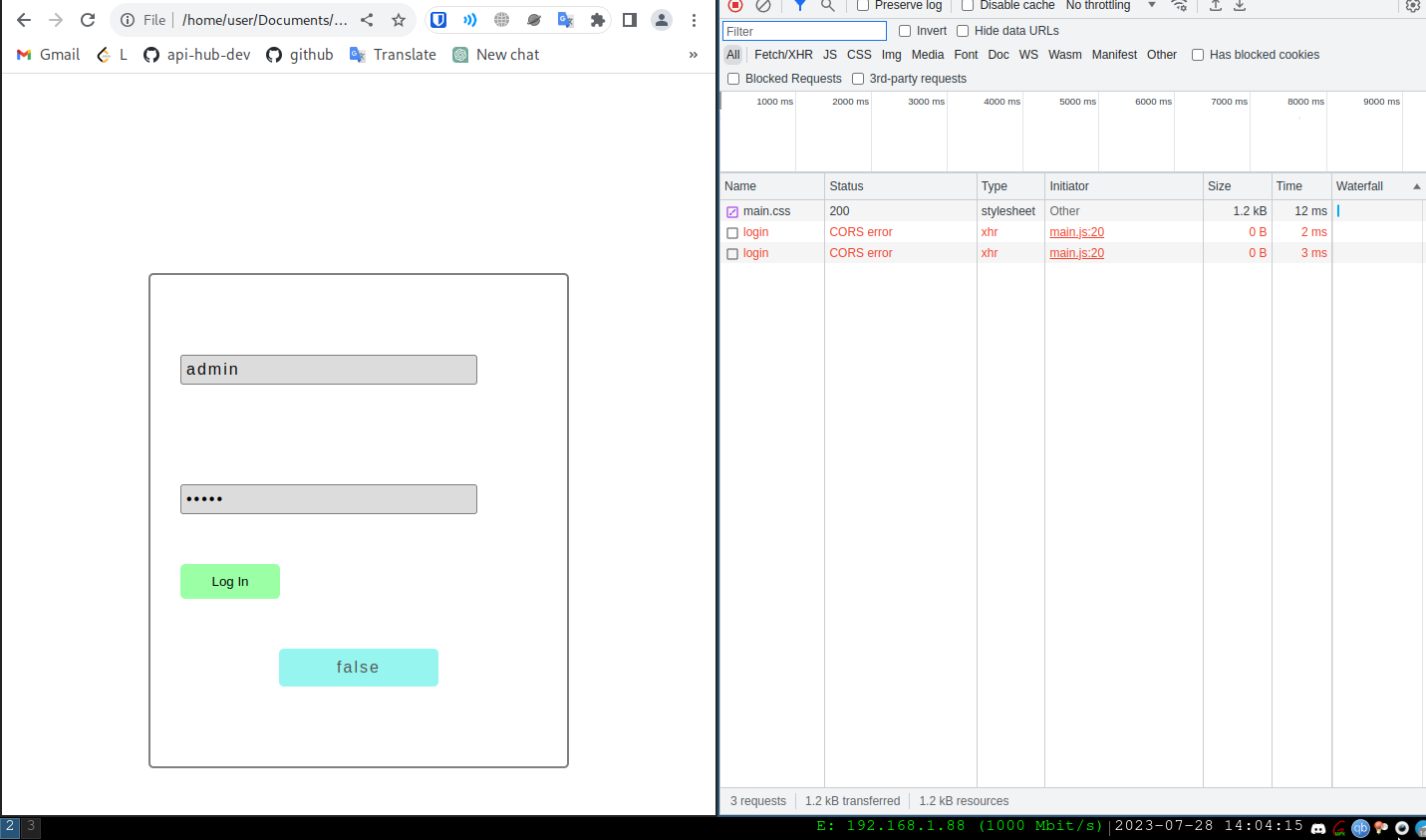
let URL = 'localhost:8080/login';
not sure you can issue requests like that from a
file://
use live-server or similar to run your frontend
so it uses the http protocol
you're getting a CORS error because your frontend and backend have different origins atm
so you'll also need to disable CORS in .NEThmm
well it appears to be working if I send it by curl

well yes, since CURL uses the http protocol š
unlike
file://
also, CURL issues a raw http request. its not a browser, so there is no CORS going on.
CORS is a client-side thing built into browsers to prevent redirect attacksso what's the fastest way to "host" my html file?
are you using VS Code?
yup
Name: Live Server
Id: ritwickdey.LiveServer
Description: Launch a development local Server with live reload feature for static & dynamic pages
Version: 5.7.9
Publisher: Ritwick Dey
VS Marketplace Link: https://marketplace.visualstudio.com/items?itemName=ritwickdey.LiveServer
Live Server - Visual Studio Marketplace
Extension for Visual Studio Code - Launch a development local Server with live reload feature for static & dynamic pages
basically I have this
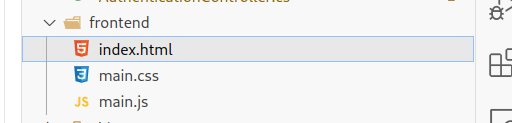
this
get this extension
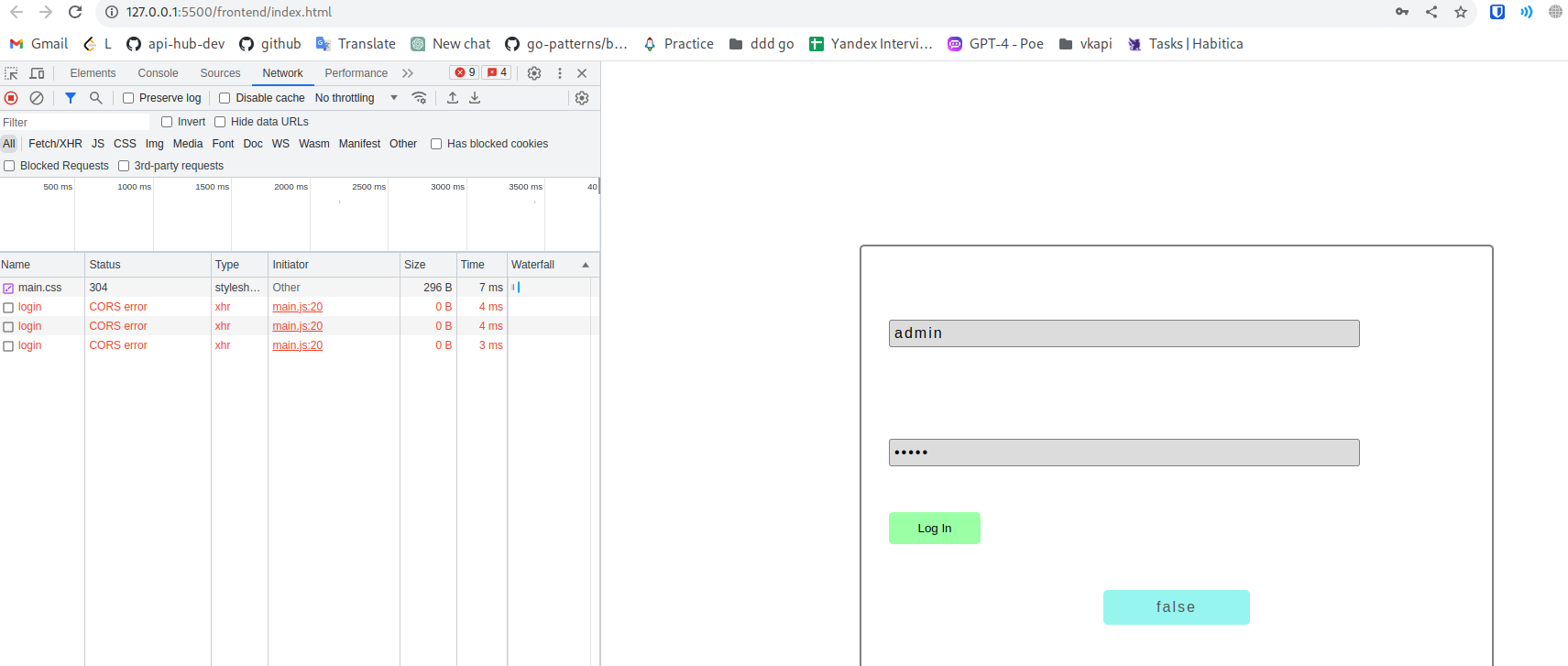
hosted at 127.0.0.1/5500
good
now, fix the CORS issue in your program.cs
I've never used c# before in my life
this will "disable" cors
by allowing everything
well
I put it like that
still getting cors errors
well yes
it needs to go before any other
app.Use
middleware order matterslooks better.
just right after
app
declaration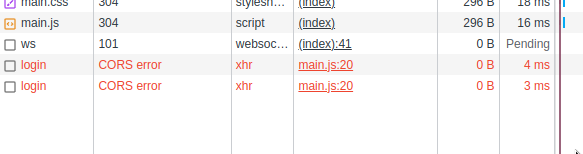
just sent a post request via curl:
logged this
thats a different middleware, probably not related.
okay, lets re-order some middleware and add another
I'm ready
what parts of code should I delete to put this
like this?
uhm you removed all the middleware after cors, including your controller mapping
true
dont do that :p
re add everything after cors, except HttpsRedirection
looks better
give it a spin
still nope :(
hang on, creating a new project to test
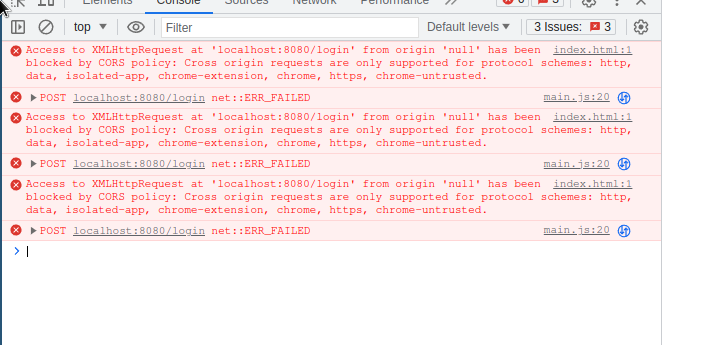
by the way
yeah, CORS errors.
but we already knew that
can you pass me your html?
sure
Please don't upload any potentially harmful files @AlexeyS, your message has been removed
maybe something is wrong with my controller:
nah thats not it
the cors is the issue for sure, but I cant seem to reproduce for some reason
well yeah maybe something wrong with my pc after all idk
I got it working when I swapped to
fetch
instead of using XmlRequesthmm
unfortunately I cannot change any front end code
only backend
okay.
thats my program.cs
it works, no CORS errors
trying
using .net 7 btw
me too
hmm wait
still :(

read the top error
ah I know the issue
your URL
let URL = "https://localhost:7242/login";
you didnt specify a protocl in your URLyess
it works
thanks man
stupid error
thats what we get for not reading the error message properly
š
yup and it changes here to true
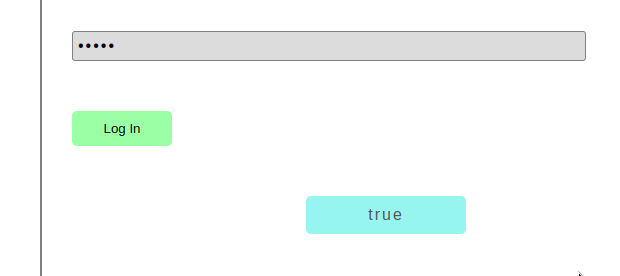
yeah
thanks for help man, really appreciate it
I would highly suggest using
fetch
and async instead of XmlHttpRequest thouyeah but the code is not mine, that was a, let's say an "exercise"
right
like, how much nicer is that š
decided to take a part in hackaton and they haven't even mentioned anywhere that you would need to write code in c#
yeah looks way better
Was about to say, why would anybody use
XmlHttpRequest
nowadays lol$close
Use the
/close
command to mark a forum thread as answered