❔ ConfigureAwait(false) (IntelliCode Suggestion)
Can someone help me to understand why IntelliCode is suggesting ConfigureAwait(false) at line 16
await Dns.GetHostEntryAsync(ipAddress);
?
Would this really result in an advantage?
18 Replies
It would increase performance, but unless you know exactly what it is doing, i would nod recommend it.
It wouldn’t increase performance. It would change the synchronization context that the callback uses.
Yes, but to change the synchronisation context takes a bit of time, so not doing it increases performance
If you actually have one, which is only really the case in gui applications
I guess your right
The suggestion has little to do with performance. It's a best practice to always use ConfigureAwait(false) if you are writing code in a library that does not need to capture the syncrhonization context. It avoids the possibility of deadlocks if some consumer of your code decides to call .Result or .Wait (which is usually a poor practice, but it happens in the real world all the time.)
I would disagree, microsoft themself say that the reason is performance and deadlock related: https://devblogs.microsoft.com/dotnet/configureawait-faq/
Stephen Toub - MSFT
.NET Blog
ConfigureAwait FAQ - .NET Blog
.NET added async/await to the languages and libraries over seven years ago. In that time, it’s caught on like wildfire, not only across the .NET ecosystem, but also being replicated in a myriad of other languages and frameworks. It’s also seen a ton of improvements in .NET,
I'm familiar with that post and I agree with everything in it. Just that performance is not the primary reason to use ConfigureAwait(false)...
Ok, i see
I wonder if I have a benchmark for this...
Depends 100% on what the synchronisation context actually does.
Ah yes, I do
GitHub
MiscBenchmarks/ConfigureAwait/README.md at main · Treit/MiscBenchma...
Contribute to Treit/MiscBenchmarks development by creating an account on GitHub.
This is a console app, it doesnt even have a synchronisation context, or am im I missing something
It has the default synchronization context (which is the thread pool)
It's entirely possible that it's a noop for the default task scheduler
I never checked
Although the benchmark numbers do seem to show that ConfigureAwait(true) has some small overhead
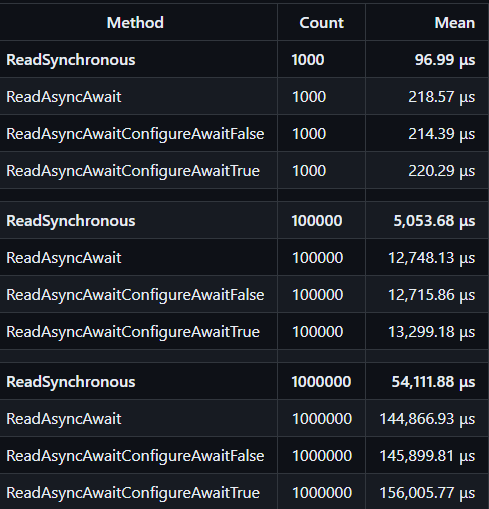
Congratulations @kocha you have lead me down a complete rabbit hole:
https://discord.com/channels/143867839282020352/143867839282020352/1133887368131264532
mtreit
I have a two line file. Literally these bytes:
If I feed this file to the above code, what does it print out?
Quoted by
<@406536255426396160> from #chat (click here)
React with ❌ to remove this embed.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.