❔ Problem while adding same article with different quantity on 2 different tables
I'm using EF Core and WPF.
Once I add same article with different quantity on 2 different tables,quantity is replaced on all tables.
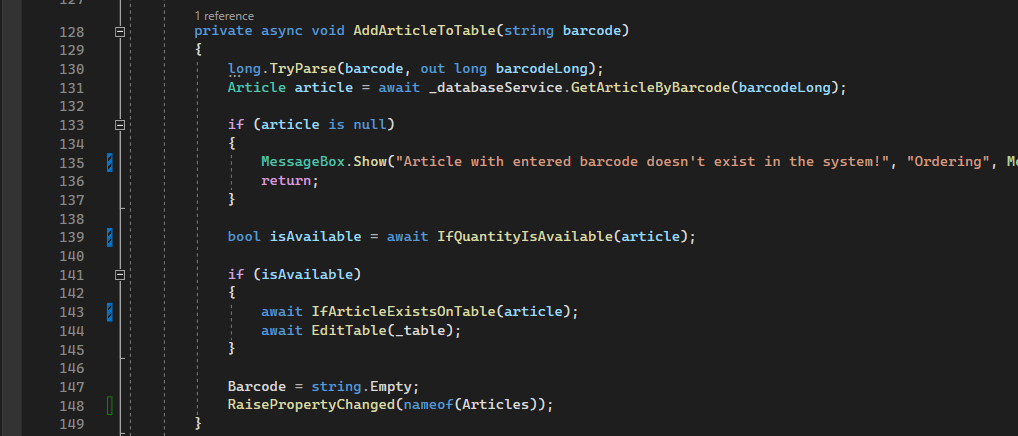
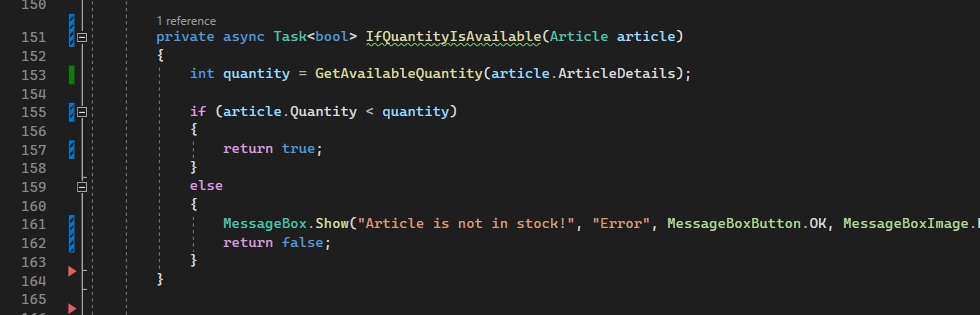
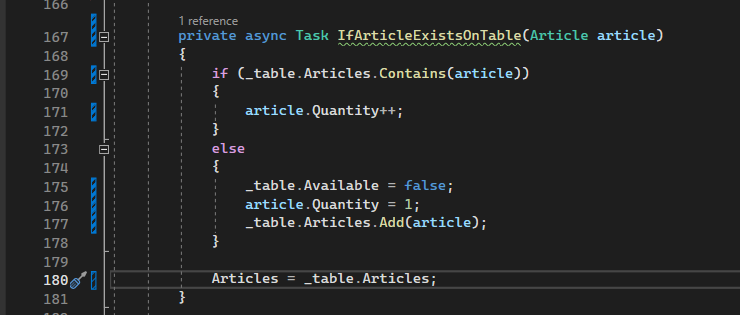
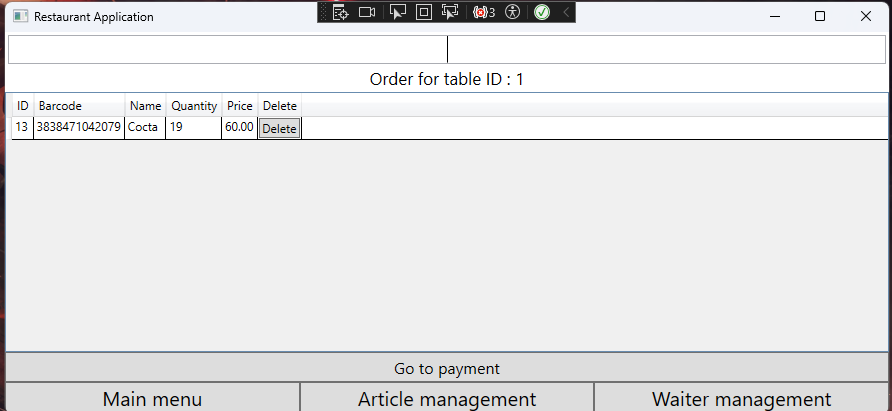
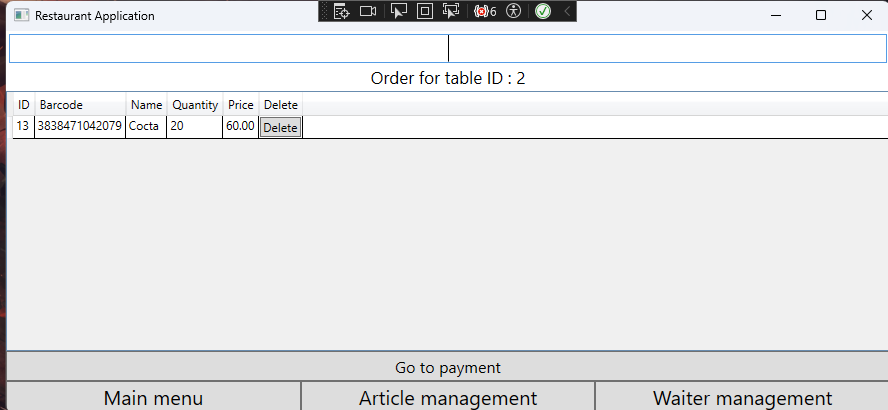
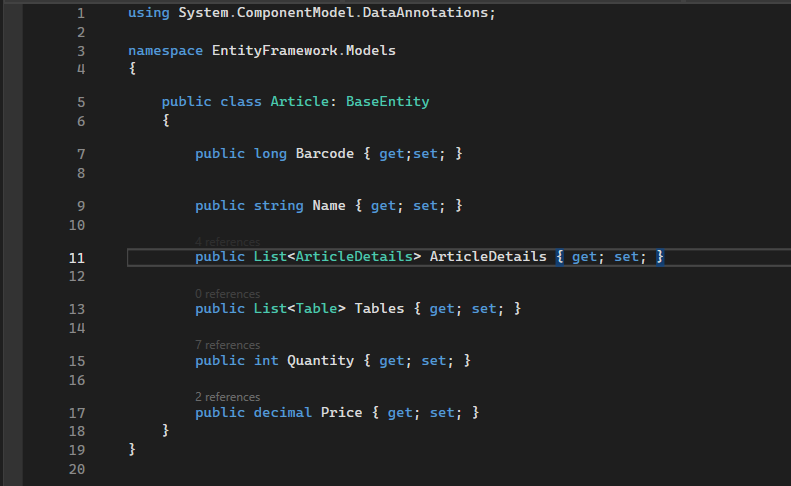
58 Replies
Make sure it's not actually the same item, as in, the same reference
The change tracker does keep track of it
so what would be the best way to solve it
Should I disable change tracker?
First, what's the relationship supposed to be?
many to many
Many to many between article and table?
articles can be on multiple tables
and tables can have multiple articles
And, I assume, the quantity is the quantity of a given article in a given table?
That's right
For example,
Table1
can have 10 apples, and Table2
24 apples?Right
Well, in that case, place the quantity not on the article, but on the join table
Im not sure if I get you
Many-to-many requires a join table
Ohh wait
EF can hide it, but it does exist
And you can tap into it
I understand
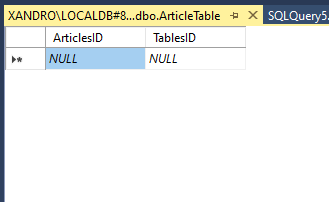
Or you can set it up explicitly
Yep
You mean to add here quantity right?
Exactly
I actually thought about that
but someone said it's not possible on stackoverflow
you can't add custom columns in join table
Of course you can
I was really thinking that would be the best solution until I found someone said that and I gived up on that idea.
And on your
Article
you can have a List<Table>
and a List<TableArticles>
And vice versaI will have to create new model called TableArticles?
Yep
Or you can create a
DbSet<TableArticles>
and just tap into it directlyOkay pal I will try to do what you said and I really hope it will work 😄
I will fill you in
I just need couple of minutes
I have something like that set up in my project, so I can dig for that code if need be to show you an example
I'll be back in an hour or so
Okay one more thing
quantity from Article should be removed right?
yep
Okay

And If I don't have anymore article.Quantity how else should I call it?
Can I do it like this? Do I have to create method to save this table or EF will do this for me since this is many to many
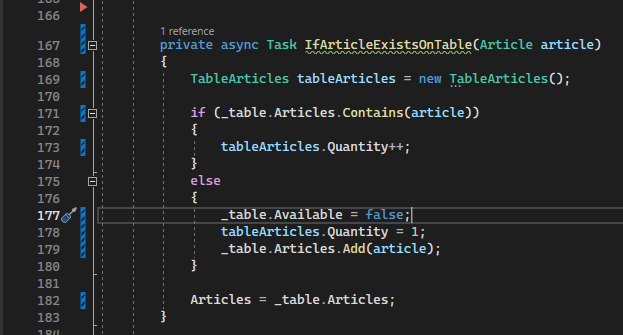
Trusting stack overflow was your first mistake😛
Ahahahahahha
Where should I learn new stuff then?
Stack Overflow has lots of good answers and lots of garbage answers and if you don't have a lot of experience it's hard to know which is which. This Discord is a good resource to leverage, as evidenced by this discussion.
Thank you
After creating new model will i still have articleTable join table?
Or it will be replaced with new one
Yeah, it will still exist in the db
Just make sure it's named the same and EF should pick it up
So wait I will than have join table and one more?
No
You'll only have one table
You might need to provide a default value for the
Count
though, since the data that exists in the database right now doesn't have that
After that, in the next migration, you'll be able to just remove the default value thoughHow I will access to quantity?
I cant do article.quantity anymore right?
You'll need to know the article and the table first, yes
And add those values to my new model right?
And create crud for that model
Then you can do
or
or
Okay and I should have crud options right?
For that model of course
If you need them, yes
One more think
This is called junction table right?
Yep
Okay thank you so much for all of these informations
Or a "join table", or a "pivot table" I even seen used once or twice
All the best for you
Anytime 

I can't create Crud for ArticleTable because I didn't do DBset
Also I can't do article.ArticleTable
It doesnt exist
And If you do this
_context.TableArticles
It means you added it to DbSet<>? RightMake it exist
Yes
Ok, I made it work with EF Core
Is there any way to make it "easier"
someone told me I could use dictionary<int,List<Article>>
and save tableId in int and all articles from table to that list in dictionary
Sure, you can select the results into a dictionary
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.