✅ Cannot figure out what I am doing wrong.
I am currently taking classes for C#, and im kind of throwing things around with casts and things, in this I am not allowed to use an array to finish the assignment, could maybe put me in the right direction without giving me the correct awnser.
The comments to the side are just reminders for myself to go off of(so don't worry about them if they are wrong.)
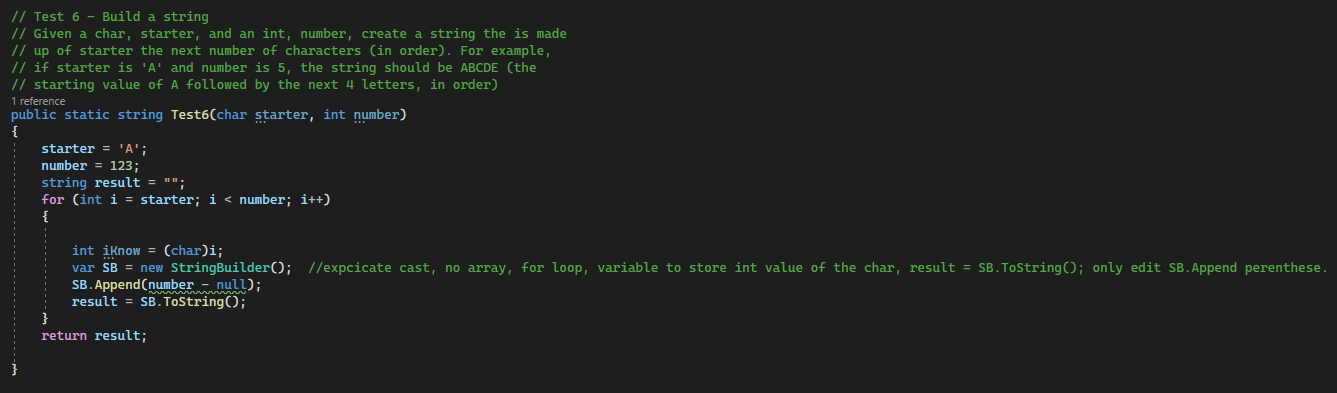
82 Replies
Why are you trying to subtract
null
from number
...?
And you should have only one StringBuilder
Before the loopOkay first off, you can return the StringBuilder.ToString() directly ya know :)
oh idk actually i got a little annoyed so i through something in there, i knew that wouldn't work
You don’t need a result string
ahh okay
alright,ill try that
If you want to turn a number to a letter, you probably want to be adding to
'A'
insteadAngius
REPL Result: Success
Result: <>f__AnonymousType0#1<char, char, char>
Compile: 403.041ms | Execution: 65.320ms | React with ❌ to remove this embed.
Hmmm, no. How you would go about this is like this. Each character has some numerical equivalent (I think Unicode or ASCII I forgor)
If you’re given a starter char
were woudl I apply this exactly? in the new SB?
What you would need to do is get its equivalent number in whatever system, then you add one to said number and add the char cast(can you do this?) to string builder in a loop
In the loop
How do I use the bot thingy, im not sure if something like (char)90 would work
That's how you generate consecutive characters
!e [code here]
Thanks
yes but like new would throw a sythax no?
It was just for demonstration sake
since new does not exist yet, so im asking how to build that.
To show you how math on chars works
Moods
REPL Result: Success
Console Output
Compile: 588.636ms | Execution: 26.996ms | React with ❌ to remove this embed.
ahh okay
Okay yeah that’s how I’d go about it
Get the number from the starter, increment, char cast and append to your stringbuilder in a loop and return it
okay I tried that, but it was throughing an error for me fo some reason saying I can not convert a char to an int.
ill try it again, maybe i spelled it wrong
Tbf, I converted an int to a char, and im surprised that even worked…
Why?
Chars are integers
Like, literally
Ehh I wasn’t too certain I’ve tried it out before but I wasn’t 100% it’d work
waiti i just noticed that says a, how does that work? is that because 97 = a and visual studio just knows that or something?
Moods
REPL Result: Failure
Exception: CompilationErrorException
Compile: 539.812ms | Execution: 0.000ms | React with ❌ to remove this embed.
cause a is not to anything, im lost on that.
My quotes got 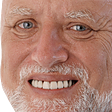
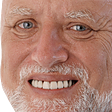
lol
It's because of how char is implemented in C#
A char is a wrapper around
int
, basically
It's a number the character has on the ASCII tablealright, so char variable = cast of char to its number... hmm
Ok I confirmed 97 is the ascii code for ‘a’
Angius
REPL Result: Success
Result: string