❔ ✅ Using sound in C#
Could someone help me with adding a sound to my program. I'm trying to make a very basic timer which asks the user for a timer and once the time is set, it plays a sound
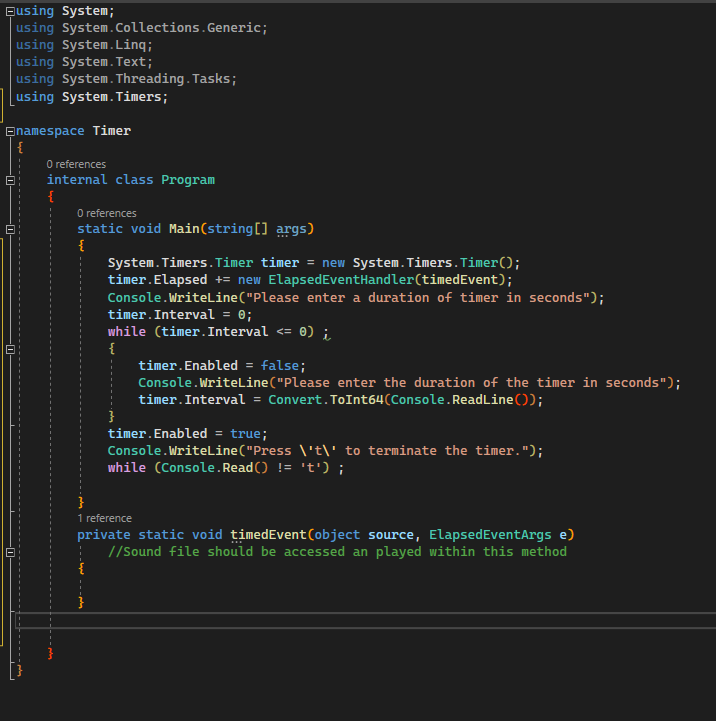
127 Replies
You'll most likely want to use NAudio
Would you mind elaborating please?
As in how to implement NAudio
Google "NAudio C#", find https://github.com/naudio/NAudio
scroll down on mainpage to "Playing an Audio File from a Console application"
see following code
Ok, cool - now where would I place the location of the actual audio file "C:\Users\Ace\Desktop\mixkit-retro-game-emergency-alarm-1000.wav"
I'd suggest you put it in your project folder, and set up a "copy to output directory" action for it
so its always available locally to your project
ok, makes sense
then rename it to "alarm.wav" or something nicer to work with, and you're off to the races 🙂
Do you know where I can find a tutorial to set up that action - very new to C# here
Sounds good, thanks :)
Easy. What are you using, visual studio?
as in, not code.
yh, I'm using Visual Studio
okay, so copy the file into your project root directory
then, in VS you should see the file in your solution explorer
click it, then check the "properties" window
there, under "Copy to Output" select" copy newer"
ok
For some reason the file isn't showing up even though I placed it in the project root directory
show
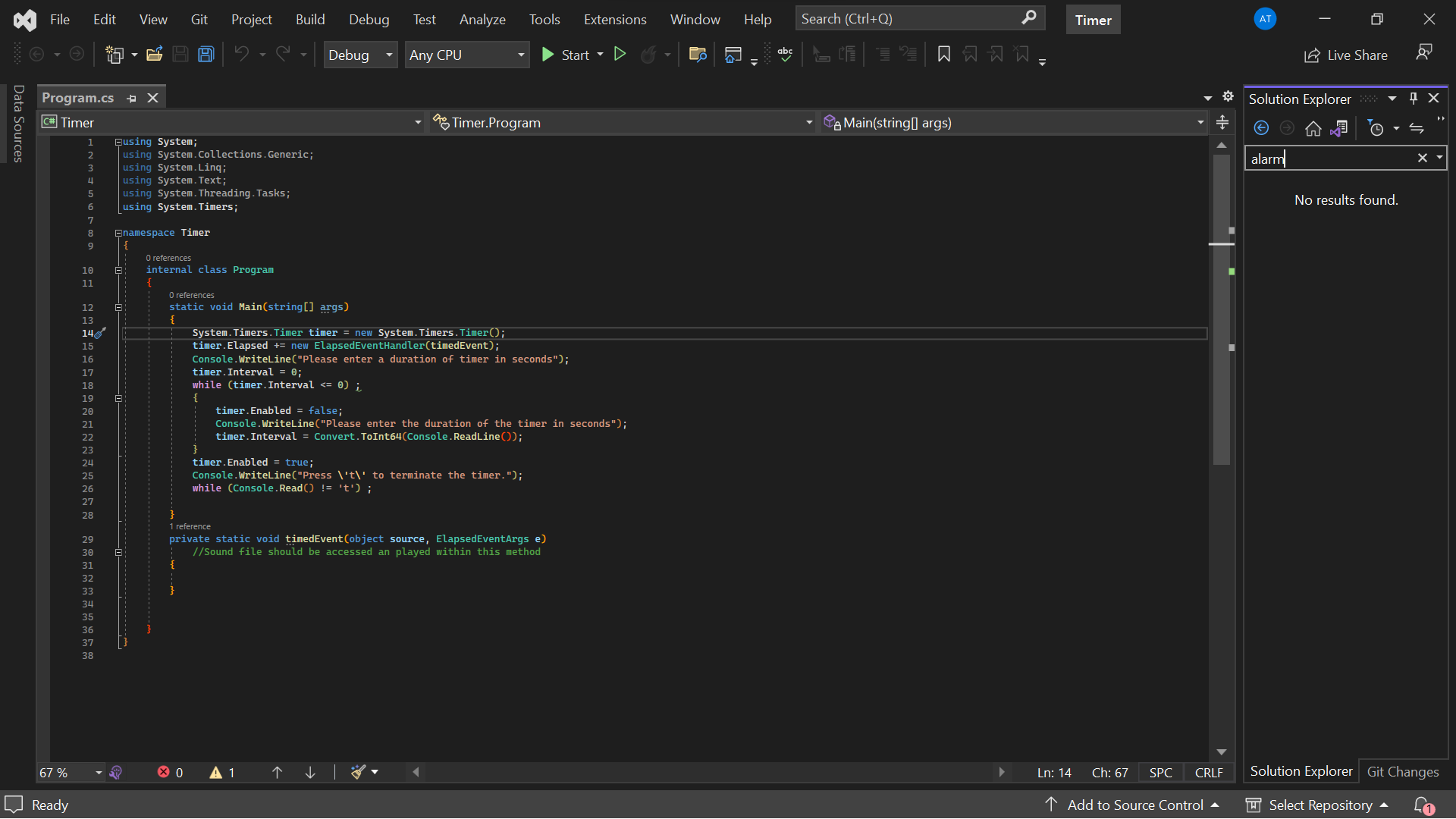
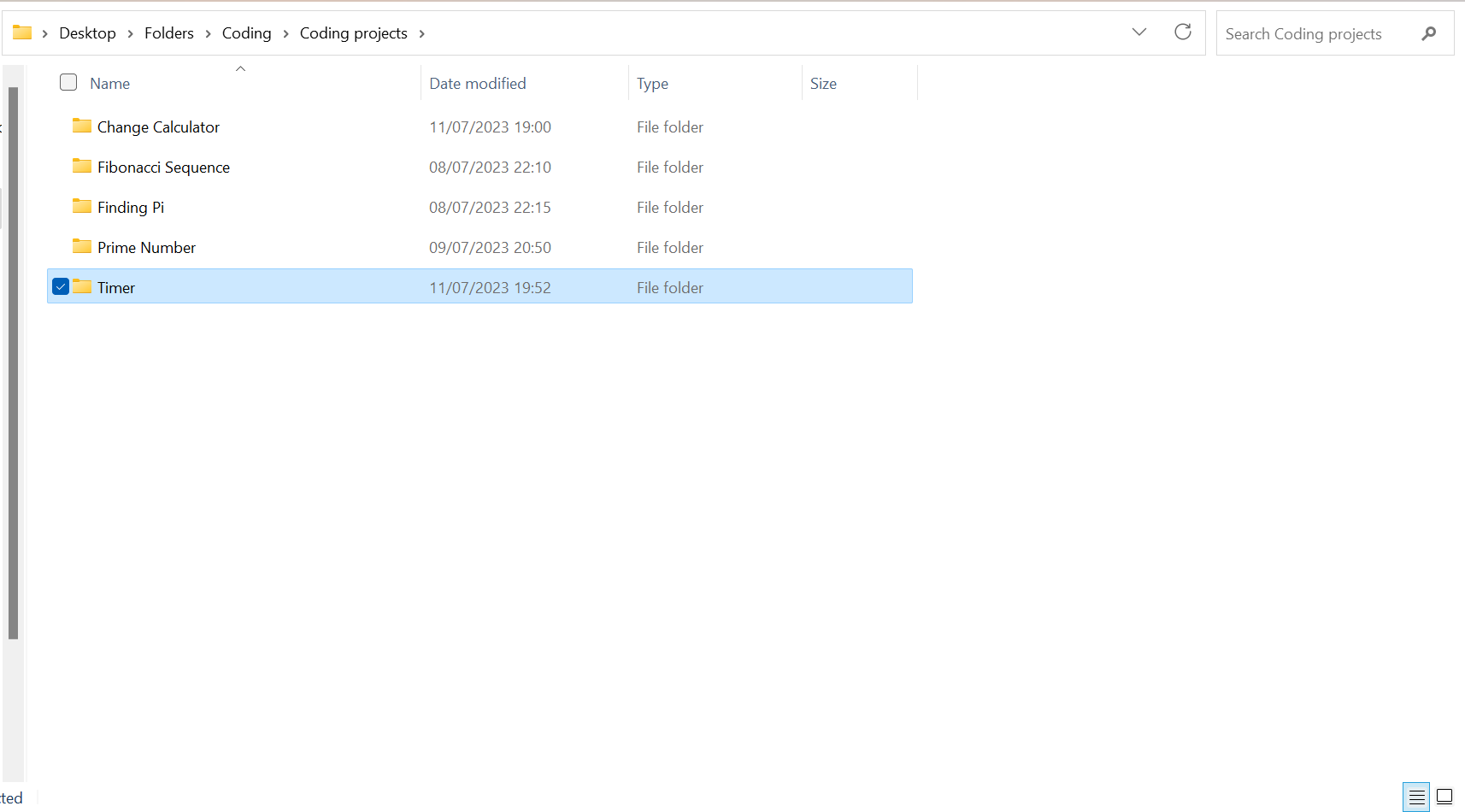
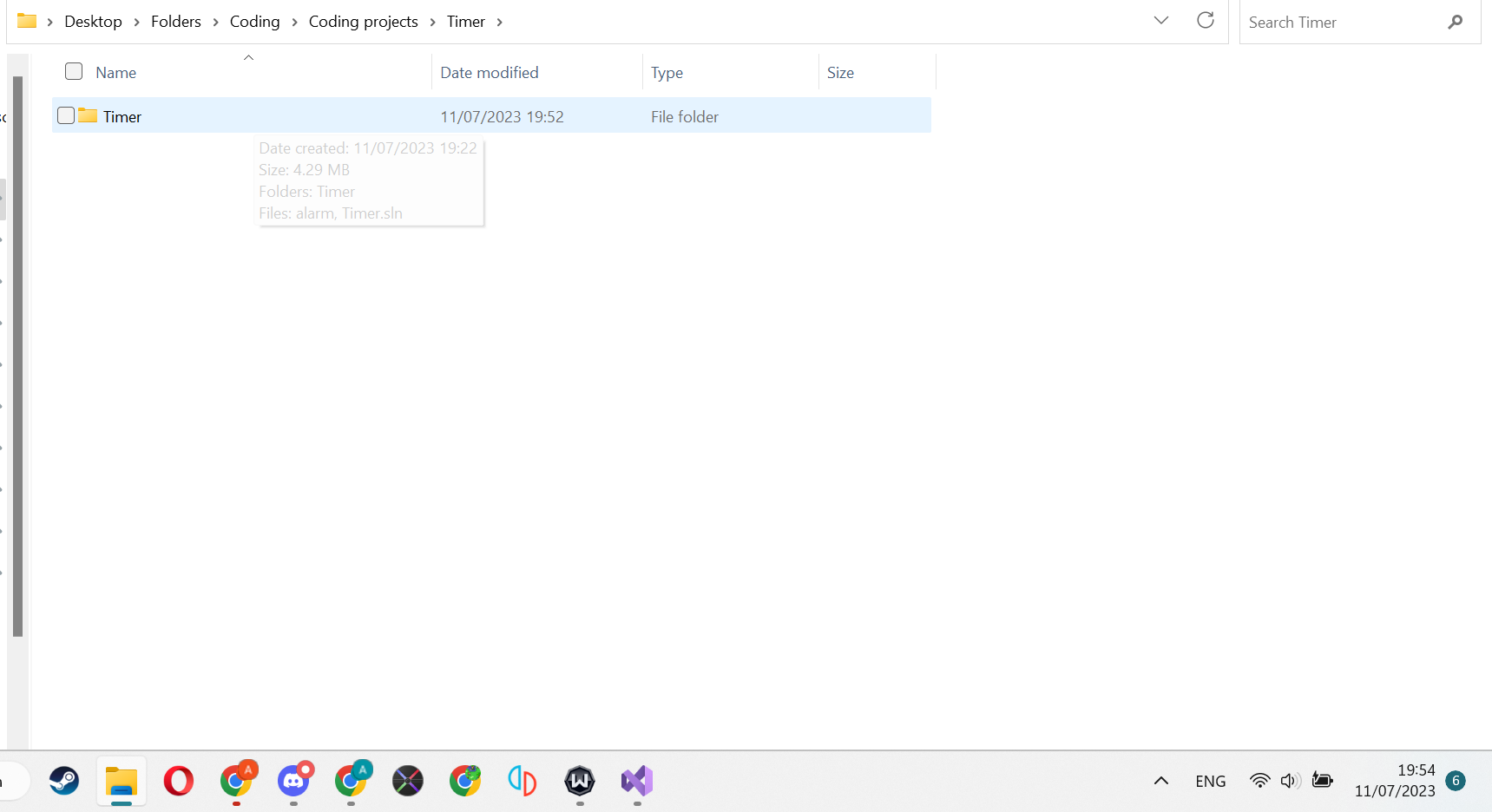
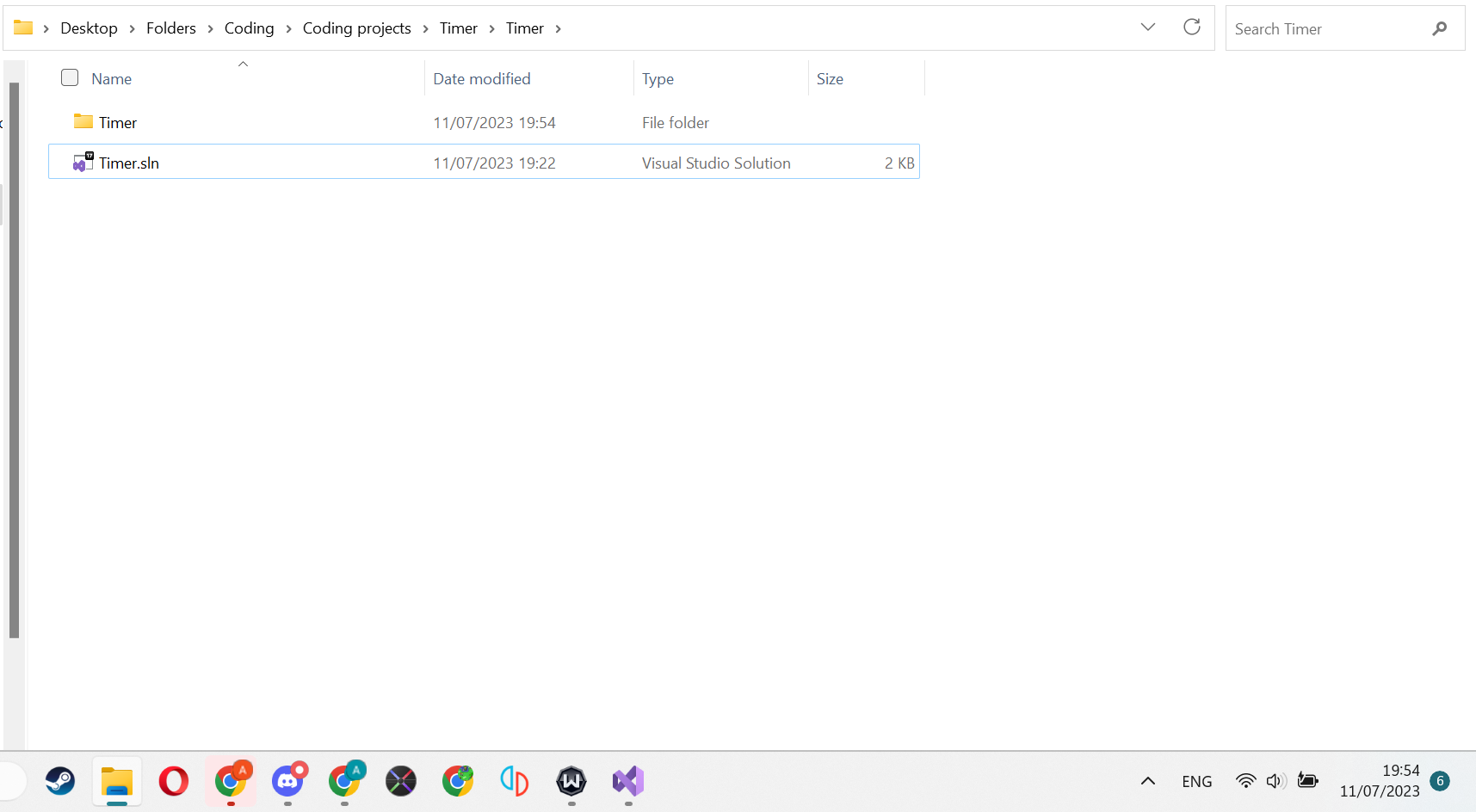
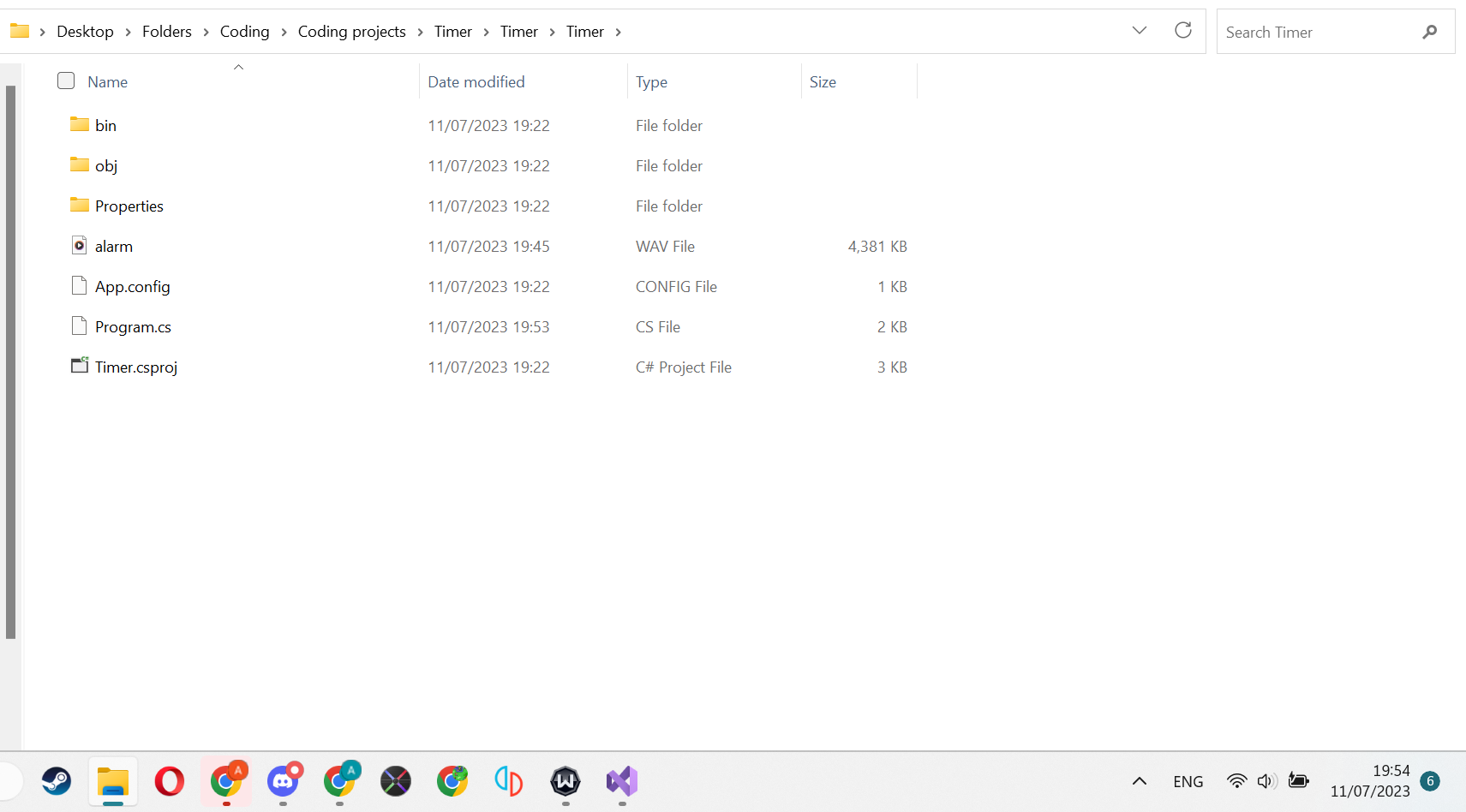
ok, remove the search parameter
ooooh
i see it
this is a .NET Framework project
not a .NET Core
meaning you are using a .NET version from... 2016-2017?
I think I am, It's using .Net Version 4.7.2
yep, thats indeed older than my children
Any particular reason you are using the old versions?
If not, I highly recommend upgrading
Honestly, I wacthed a tutorial from about a year or two ago when setting up VStudio so that's probably why
Alright, this is doable even on the old version, but you really really should upgrade
Will do!
Should I upgrade to .NET 7 or 6? I think there are some differences
7
I want to be using this when making projects from now on corrrect? • ASP.NET Core Runtime 7.0.9
Also are these settings correct?
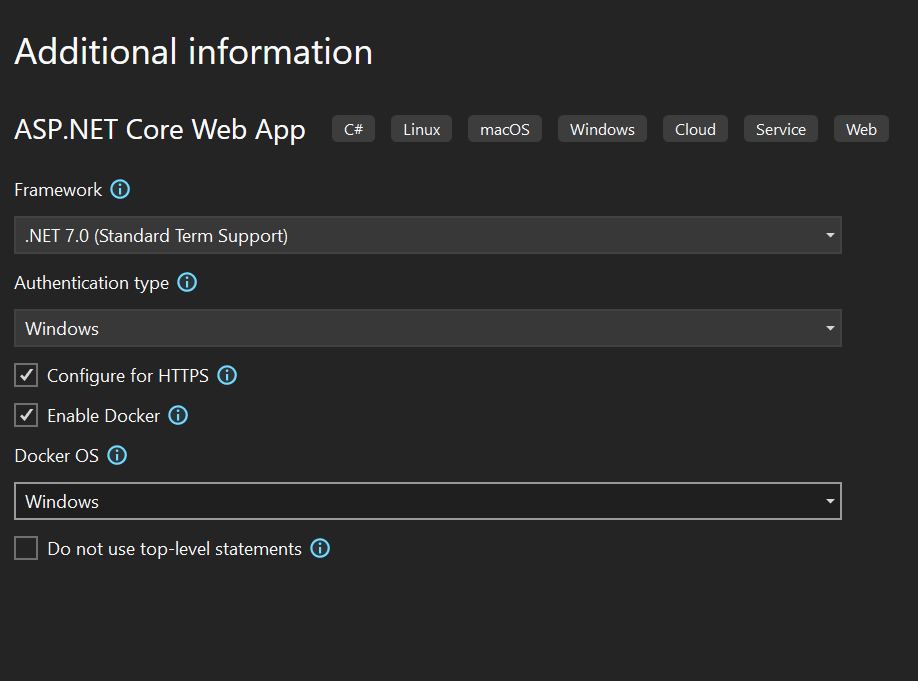
uh, where did it even ask that?
but yeah, thats the latest version of the runtime
but you need the SDK to actually make programs
the runtime is for running programs
yes, but remove "enable docker"
Ah ok, thanks for the heads up
and thats a webapp, not a console app
Better
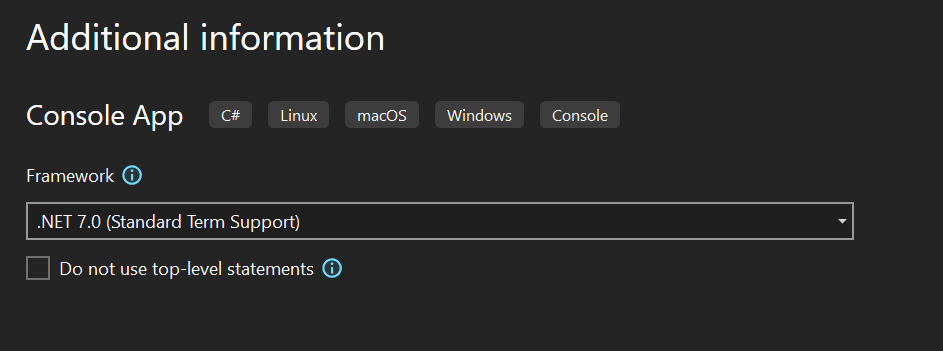
There we go
I've also gone ahead and downloaded the appropriate SDK
you might be more comfortable checking that checkbox 🙂
great
I hope you made sure it was the x64 versions?
a lot of people install x86 by mistake and then end up having issues
I made sure
excellent
Just need to transfer my code now
I'm getting some strange syntax error with my method at the bottom and something to do with nullability with the classes at the top - besides that all is good
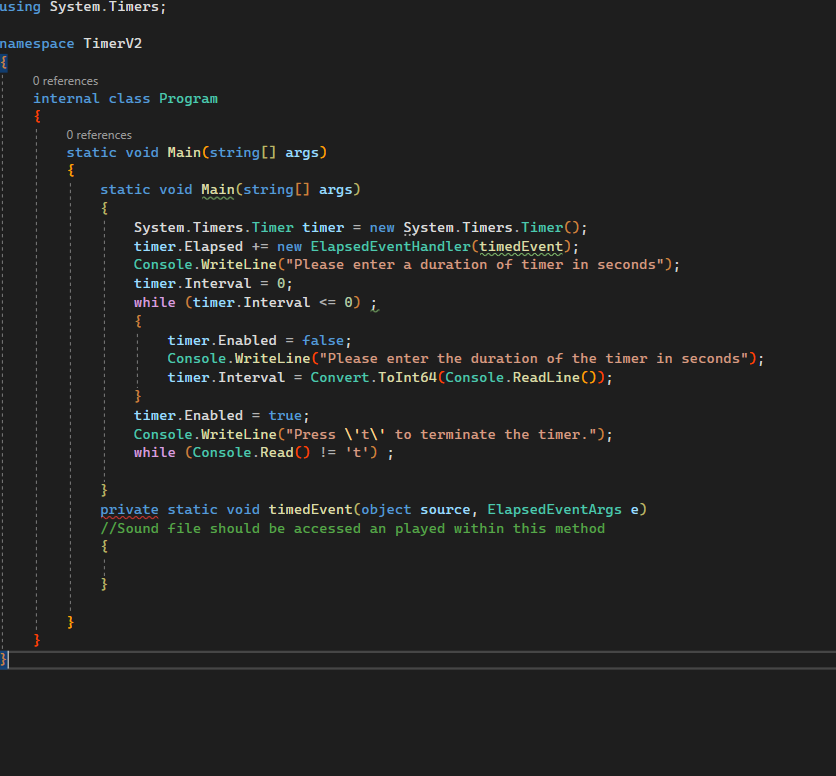
you have a nested main
two mains.
Ah
My mistake
You're referring to copy if newer correct?
Yup
Back to this
Where does the path for the music file go in the part of code you shared?
Have you added the nuget?
I'm not sure whcih Nuget to add
There are quite a few options
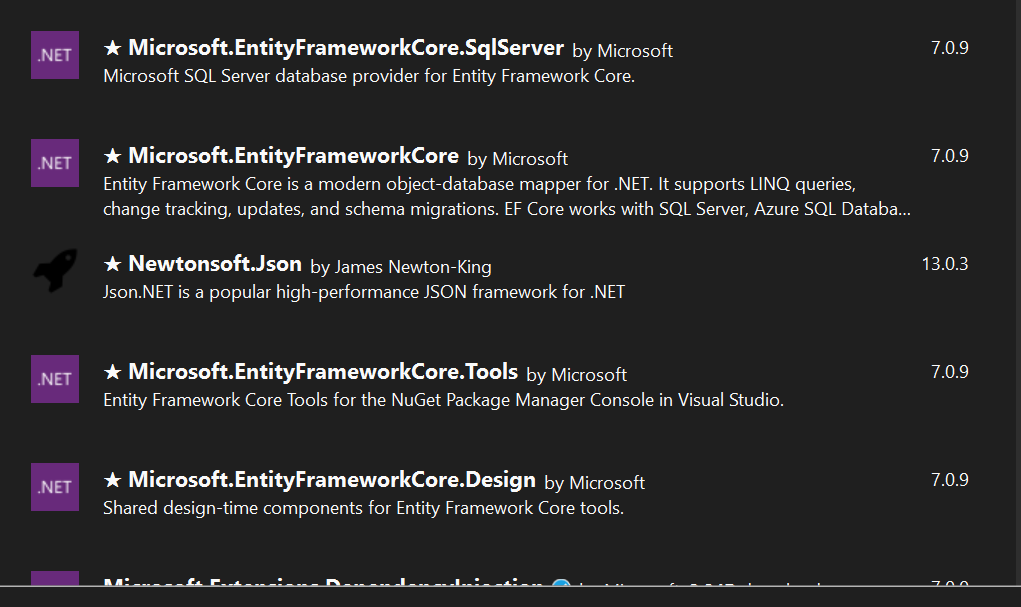
<PackageReference Include="NAudio" Version="2.1.0" />Look for one called NAudio or add it manually
I'm guessing I want .Core
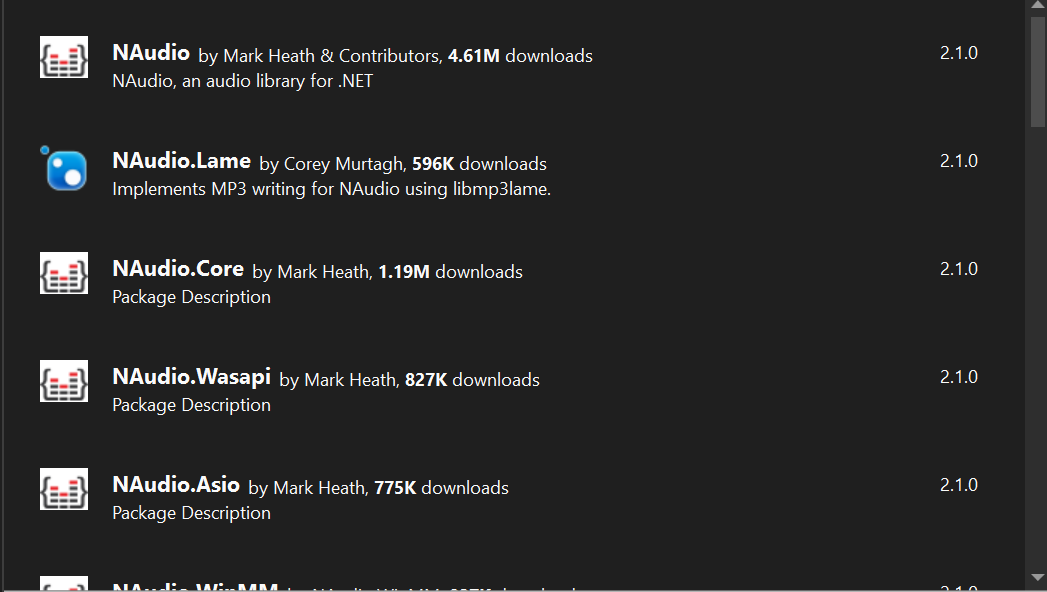
Use the first option, NAudio.Core is their well, core library, it should be implicitly installed along with other dependencies of itself
Aye, just instlal the top one
All installed
Now how to do I proceed?
How do you think? Legitimate question.
So, here's the code I need to use - my issue is not knowing where to place the path for the .wav file
Look at the code.
Try and figure it out
there is really only one place that makes even the slighest amount of sense
hell, the method parameter is actually called "fileName"
my initial guess was WaveOutEvent()
How so?
What do you think
WaveOutEvent
does?
and what do you think AudioFileReader
does?
Hint: one of these reads an audio file, the other plays the wave audioI'm guessing AudioFileReader, as the name suggests, reads the desired file
sounds about right to me
What Pobiega means is, if you don't try to figure it out yourself first it will be harder to learn. Since through trial and error you learn more than you usually would have learnt if you would have been given the answer verbatim, exploring what parameters you can feed it
^
giving you the answer verbatim would be what we call
(spoonfeeding)
its frowned upon generally
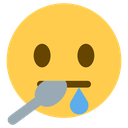
See that was my original guess but I thought for some reason that the WaveOutEvent makes more sense. Guess I should have looked at it a bit better and walked through the logic
I mean I appreciate not straight up being told answers so not qualms here
great.
Thanks for the patience and taking the time to walk me through and help out
no worries.
its worth noting that
Thread.Sleep()
is a little intrusive, it actually freezes your entire program for the duration given
its used here to prevent the program from finishing before the audio has finished playingAh ok, good to know
you can skip that, if your program isnt supposed to end directly after playing the audio
Ok, it should be fine for this particular program
But I am now struggling with this and I feel like I've missed something very obvious
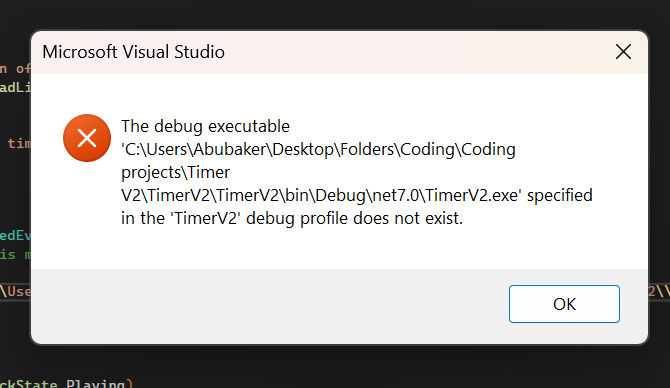
that path doesnt look right?
TimerV2\TimerV2\TimerV2
It should be
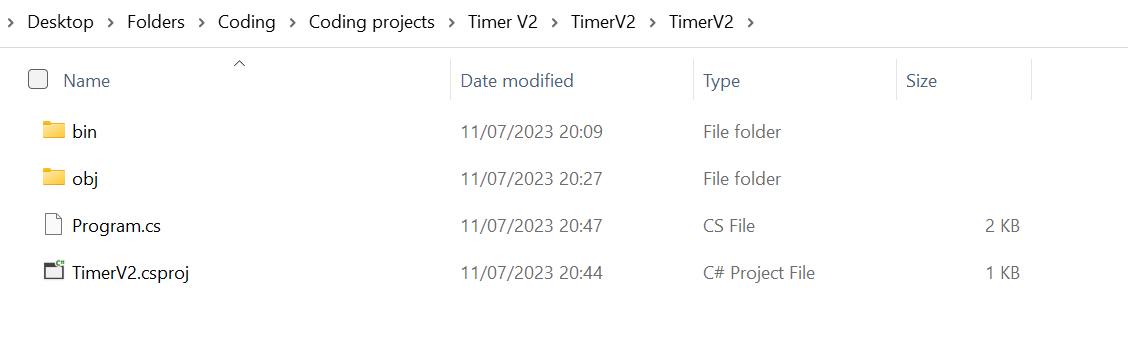
There doesn't seem to be an executable file thoigh
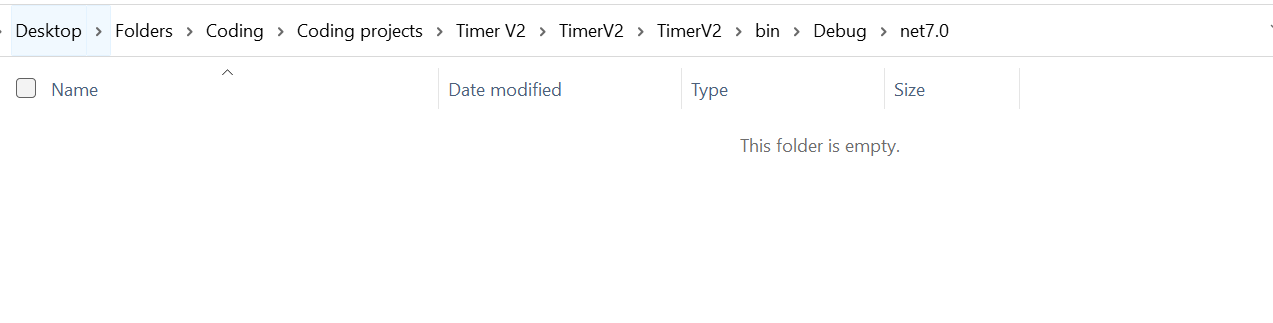
okay
do you have any compilation errors?
No
Right, after scanning through, the problem seems to be an additional } at the end of the program
that would indeed entirely prevent compilation
Right I think that's it
The interval is measured in seconds right or ms?
hm? what interval?
You can hover over the member to get more info about it
Im like 99% sure Visual Studio has that feature
it does indeed
Where I ask the user for the duration of the timer
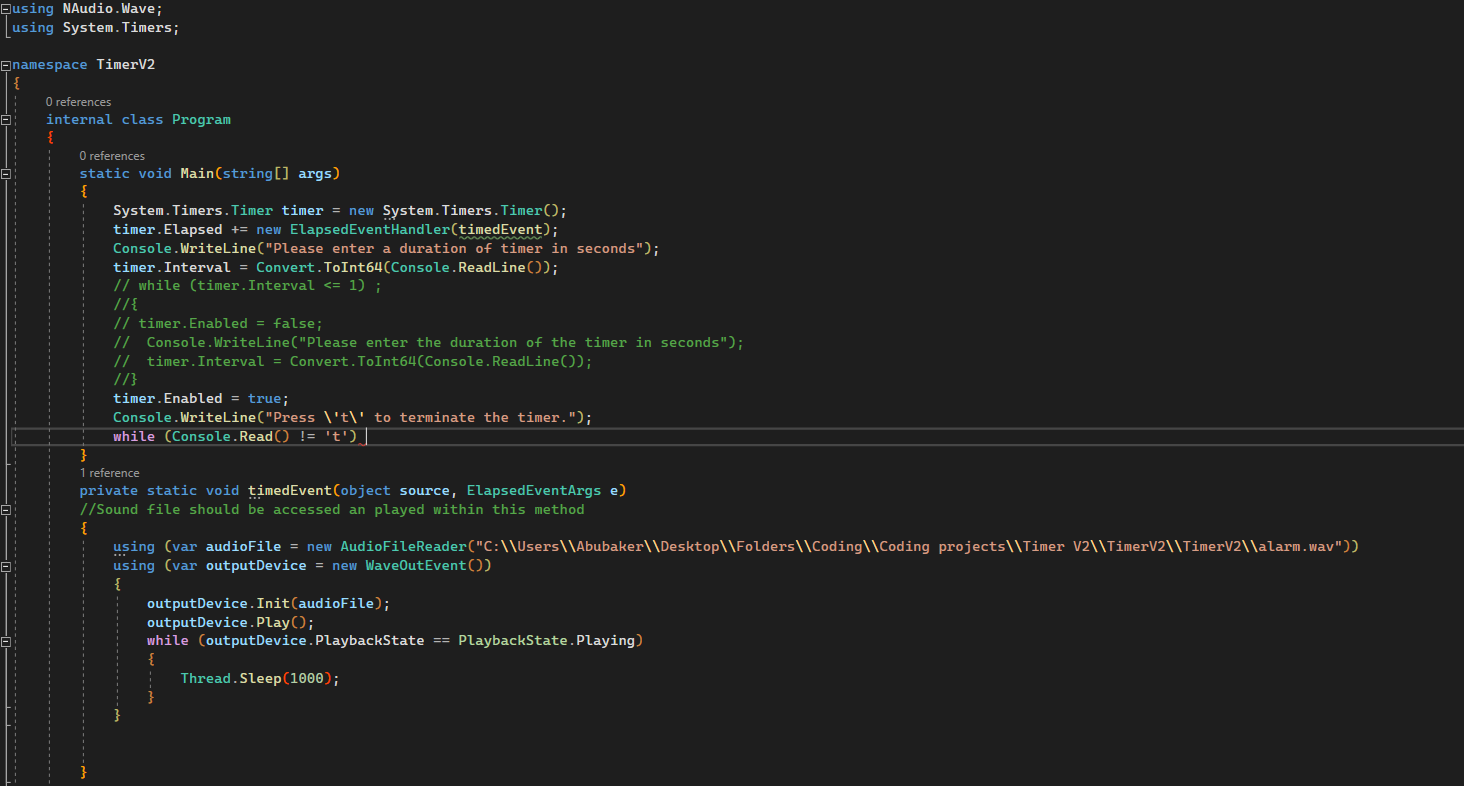
oh dear me
you hardcoded the absolute file path anyways?
after all the effort we went through to NOT have to do that?
😄
My bad, sorry
and no
Timer.Interval
is in milliseconds, as arion pointed out
also, be aware that Convert.ToInt
is... not recommended
hint: what does it do when I type "cheese" as my interval?TrySparse instead?
correct
I think this does the trick
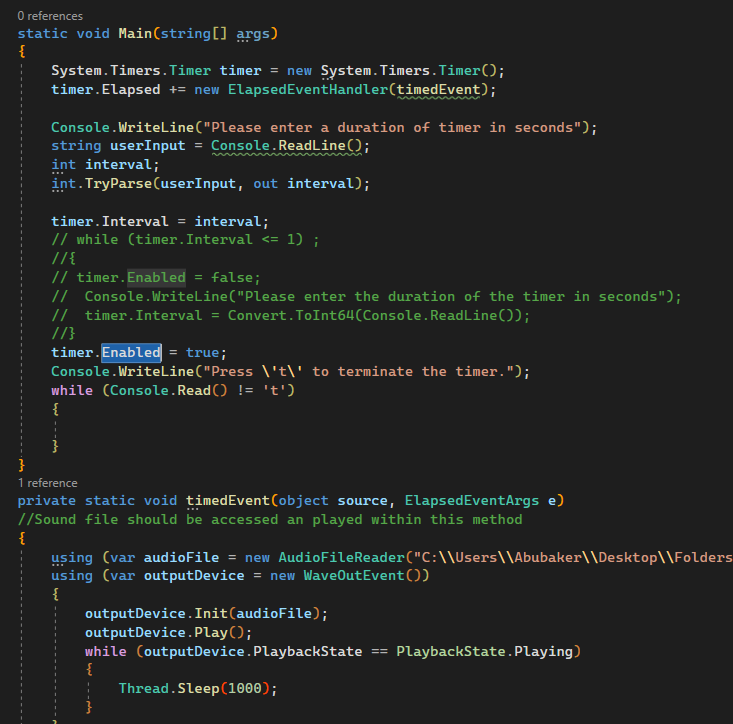
not really
you dont use the return value from
TryParse
so you dont actually know if it succeeded or not
Allow me to share one of my favorite static methods
Slap this badboy down and call it like int interval = GetFromConsole<int>("Enter your interval: ");
and you are doneI apparently need to express the type arguments explicitly
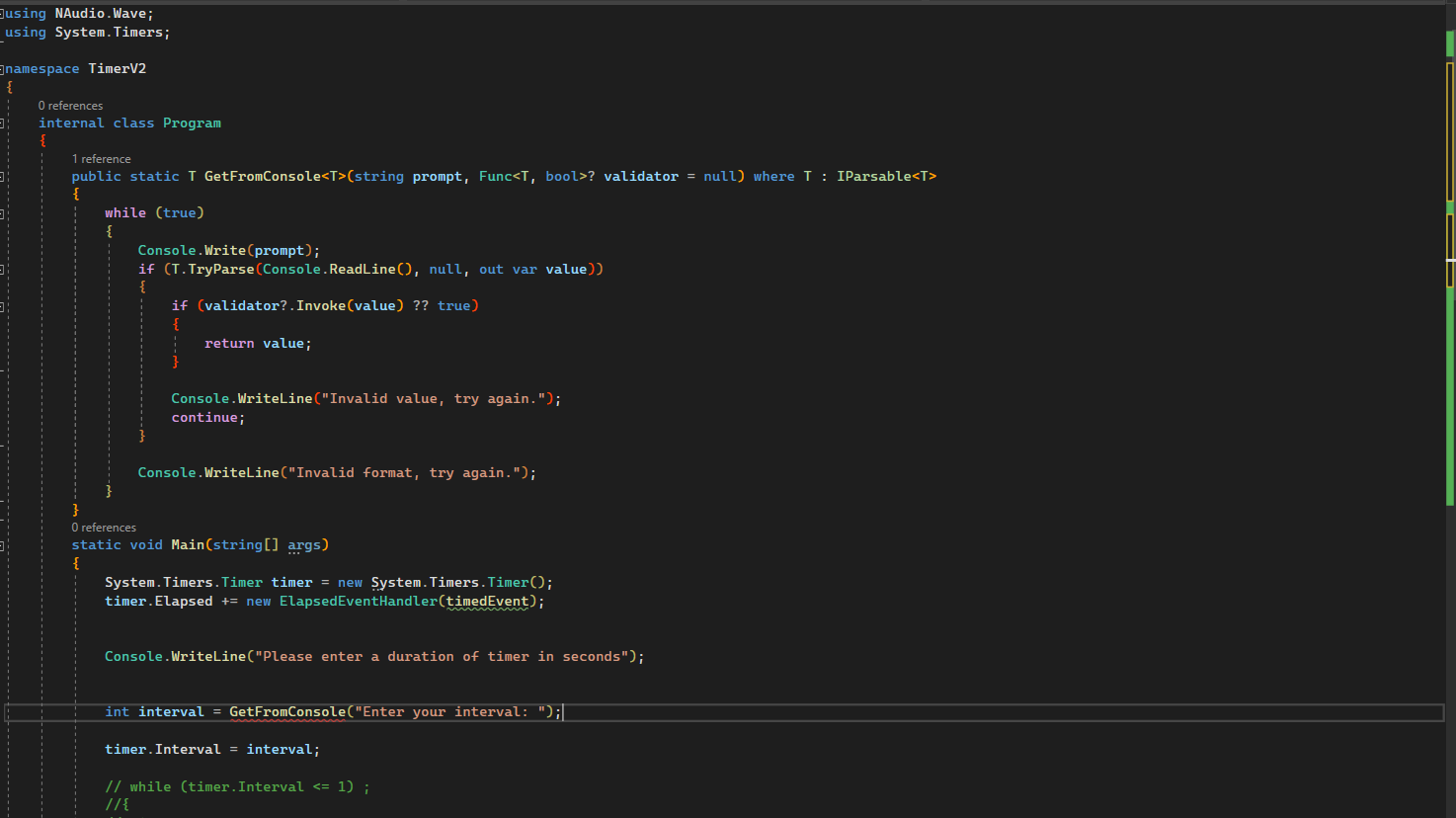
okay, just slap on a
<int>
then 🙂I honestly have no clue where to put the int
big brain 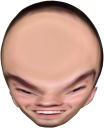
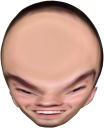
int interval = GetFromConsole<int>("Enter your interval: ");
generic type parameters always go after the method nameGot to note that one down
Multiple generic parameters are separated by an
,
so <int, bool>I wish it were that simple (for me), the audio isn't playing and I think it has something to do with the directory path maybe
generic methods is a bit advanced for your current level, so don't worry about it 😛
just change the filepath to "alarm.wav"
literally just that
Still no audio playing though
thats what I did here and it worked fine
are you sure your interval/timer stuff is working?
Number 1 good idea in programming is to test each part individually
I normally do, but I kind of jumped the gun here
I think I'm missing this (outputDevice.Volume = 0.1)
thats not relevant as such
I just like protecting my eardrums 😄
and I have in ear headphones
it works fine without it, its just really loud
I think that set of {} (yellow ones) are doing nothing here
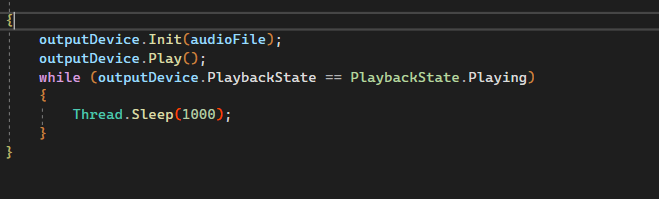
well not nothing, but they aren't supposed to be there anymore
hard to tell without knowing what goes above it
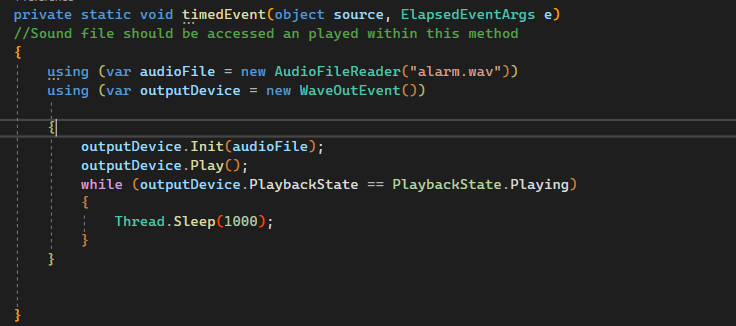
no they absolutely do something
you need to change up the syntax of your
using
s if you wanna get rid of that scope
I'm using modern C# using declarations
thats why my code didn't have them :p
just add some Console.WriteLine("Sound should be playing now");
or somnething to the top of your method
and see if its printingyup, your hunch was correct, nothing is printing
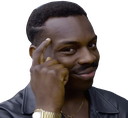
try doing
timer.Start();
oh dearie me
I never bothered to read the timer code, as it wasn't relevant to the actual topic :p
xD
Right arion, you were right that did indeed change things
tbh thou, wouldn't a
PeriodicTimer
be a lot easier?But the sound is still not playing
thats my entire main method
Enter interval in seconds: 5 Playing sound. Done playing sound. Playing sound. Done playing sound. Playing sound. Done playing sound.its really annoying 😄
Overcomplicating things (and then not getting them) is my middlename
Well... its not really fair to compare your code to mine
I write C# for 40 hours a week and have done so for the last 20 years or so
Yh, I'm aware
PeriodicTimer
came with .NET 6
so its fairly new, and didnt exist in .NET 4.7 that you were using an hour agoDoing 7 hours a week for 1 month isn't quite comparable. Though I have taken the long route with things a few times in the past
I'm still annoyed by the fact the sound itself isn't palying
Wanna jump on a screenshare and get it working?
pretty sure its that part ngl
Sure
try changing it into
timer.Elapsed += timedEvent;
#dev-vc-1
Thanks again for the taking the time to help
np
If you are done with this thread, please
/close
itLooks like nothing has happened here. I will mark this as stale and this post will be archived until there is new activity.