❔ Is this good practice with public accessors?
I come from C++ which used get/sets to access private members. In C#, there seems to be several ways of doing this. I learned the latest one. It seems like less time accessing these probbaly due to less function call overhead.
I'm creating a CustomDateTime class that's specific toward a game I'm working on. Is below good practice of using these public accessors? I assume the rule is if it fits in one line (member variable or expression), it's good practice?
21 Replies
$getsetdevolve
can be shortened to
can be shortened to
can be shortened to
can be shortened to
Generally speaking, there's no need for backing fields most of the time
And I see plenty of fiends in your code
Prefixed with
m_
for some reasoni think that's a C++-ism
i prefix private fields with _ personally
Same
iirc you can't/shouldn't do that in C++ because _ and __ prefixes are special
The question isn't on how to declare variables, as we all have our preferences and hopefully stay consistent with them, but am curious on these expression-bodied members. To me, it seems kind of confusing with what's a method and what's a variable with these accessors.
And there's no way to error check them when they're used like variables but is doing a single-lined expression internally.
a method has ()
technically property getters and setters are also methods, but abstracted away to have normal field access semantics
Equivalents to
respectively
I think I might stick to Get() and Set().
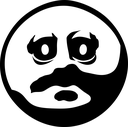
At work, we used
Get*() and Set*()
for VB.Net back in the day. I'm just used to it. But for games, which is what I'm doing, I think it would be good to see which is faster. I know some developers said to stick to Get() and Set() for performance.Properties are compiled to methods
There's zero performance gain
You just waste space writing 4 lines of code instead of 1
But what if you want to add error checking and other checks later?
It's rewriting it to those 4+ lines.
So now you have properties and Get/Set methods.
It's not consistent to me.
No, just use refactoring tools and turn that get-only prop into a full prop
And add the code you want
I see.
The refactoring tools will easily turn the property into a property with a backing field
And then you just need to add the code, say
or some such
Yeah, that is helpful.
Still, is this all good practice with the code above using these to access variables and single-lined expressions?
Yeah, that's pretty much the reason to use get-only properties
Cool, I'll try it out for this project.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.