Accidently closed last post. Need help with data structure(Noob).
Hello this is my function
Model:
The focus here is on the parameters of a stepmodel. Current return value of merged steps is something like this(example):
Previous help consists of changing my Model to a Record. Unfortunately i cant do this because of constraints from PO.
Thanks in advance!
35 Replies
Why are you using 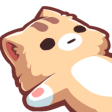
dynamic
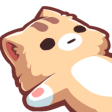
I used a tim correy tutorial that helped me set up a minimal API. https://www.youtube.com/watch?v=dwMFg6uxQ0I minute 58
What does
LoadData
do that'd require the use of dynamic
?
As a generic parameter no lessThis is LoadData
Ah, so it's some homebrew ORM thing
I can see
U
being dynamic
, although it should probably just be whatever it is in QueryAsync()
T
should never be dynamic
or object
, thoughI see, so what would you advise doing ?
Making a class that represents your data
And using that as
T
aah yes okay, but what i dont understand is how this helps the initial problem that i had. I feel like changing the structure from what I have now to what I want isnt related to changing dynamic to a type of class ... but then again im a total noob in C# and maybe im just thinking too much in a JS way?
Well, the SQL query will return the data in a specific shape
Right now, it returns data in the former shape
So you'll need to rewrite the query to return the latter
Or you'll need to change the shape of data after fetching it
For example, some
Yea rewriting the sql query isnt an option to my knowledge. This is because it is fetching data from 2 tables and combining them. A step table that contains steps and a parameters table that contains the parameters belonging to a step. the SQL result will contain the steps information but duplicate because it can have multiple parameters. I then try to combine the data where the steps are unique(so the duplicated info is just 1 step) with the parameters as an property of that object. This all works fine btw, its just the structure that im trying to change.
That's why I don't do SQL lol
yea fml
So i am changing the data after the query sucesfully. I just dont want it as an Array but i want it as:
angius#0000
For example, some
Quoted by
<@!85903769203642368> from #Accidently closed last post. Need help with data structure(Noob). (click here)
React with ❌ to remove this embed.
Okay so with dictionary I almost have what i want, next problem is this
This works, but I want to remove the name inside of the parametersModel(because its already used as the property key, exactly what i want) but if i remove it from the model it obviously doesnt know name anymore in the above code. Any idea how i could resolve that issue ?
Instead of
use
where
Thing
has no Name
...?Yea atm thing doesnt exists, i need to make a new model for that aswell?
Yes
but then i would need to edit the parameters model
to something like this right ?
Or just remove
Name
from ParametersModel
And use thatThis i dont follow, you mean like this?
No you cant mean that, that doesnt make sense
Just remove
Thing
yea i tried that, but that gives me this error.

@ZZZZZZZZZZZZZZZZZZZZZZZZZ I feel like we just made full circle to the Dynamic issue
This is the issue, yes

So i need to make apart from the model also a class that has the same structure and pass that instead of dynamic ?
Yes
Instead of the first
dynamic
, you'll probably have something like List<SomeClass>
SomeClass
being a class that describes the shape of data you get from the SQL queryRight, but that is precisely what the Model is for right ?
It feels duplicate to have a model aswell as the class that basicly represent the same data
The database model and the model for the outward-facing data can — and somethimes should — be different
yea that makes sense
Okay i created the class:
With this code we talked about before
But it gives me an error on Key and on Value.
You might now why this is ?
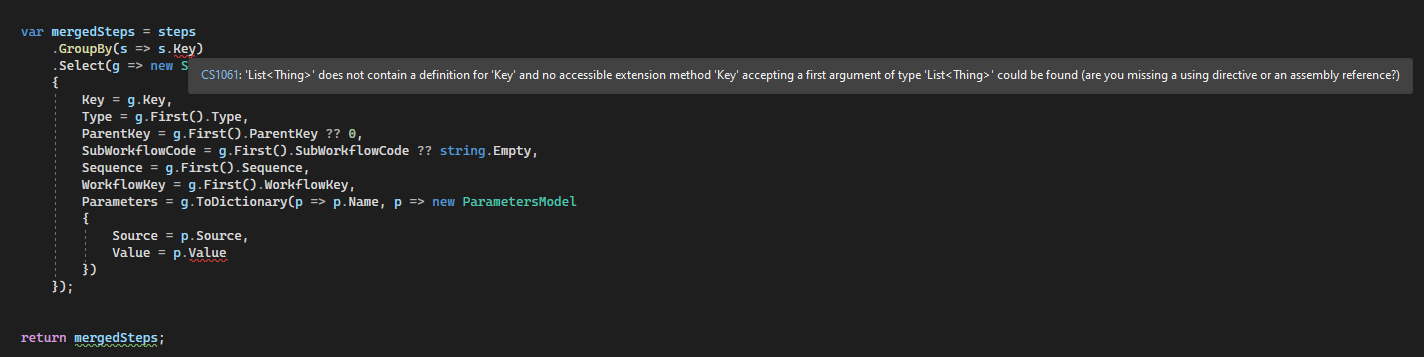
Ah, maybe
LoadData
retuens a collection of T
s by default?
Try just Thing
instead of List<Thing>
That is it! thank you so much
Anytime
Ill close the post, happy coding!