Maximum execution time of 60 seconds exceeded
I have this Observer where I want to get the total whenever I create or update on my OrderResource
It's working but taking a long time to process and gets error at the end of the process "maximum execution time error of 60 seconds"
30 Replies
OrderResource
OrderObserver
What's the question?
The process of getting the total whenever I create/update was working but only after 60seconds and i get the 60seconds timeout
Again: What is your question?
Why does that simple summarization code take so long?
yes
That's something you need to figure out. It's hard to debug performance issues via chat.
Start by checking the execution time of the updateTotal method.
Is my method correct or there's more suitable solution to do this?
I guess you are running into a loop: When you call
$order->save()
your Observer::saved() method is called againCan't I just get the total directly from the textInput something like this
You can just use saveQuietly() instead save() to prevent this endless loop in your observer.
yes, problem already solved but created another problem.
the mthod only works when I updated but when I tried to create my total value is the same of $shippingFee which means $subTotal doesnt have value.
So maybe can resolve both if I can just directly get the total using the TextInput and disregard the Observer
My suggestion would be to make this whole thing reactive and calculate the total on the fly
https://filamentphp.com/docs/2.x/forms/advanced#reloading-the-form-when-a-field-is-updated
So basically you make orderItems and shippingFee reactive and on the total field you listen for changes and calculate everything
so i'll need do use afterStateUpdated multiple times?
Why multiple times? If I got you correct you just need it for your total field
ohh, I forgot to mention that im using the total outside the repeater
This is what I got when im using Placeholder but its only Placeholder, I need the TextInput
but its not working when I used TextInput instead of Placeholder
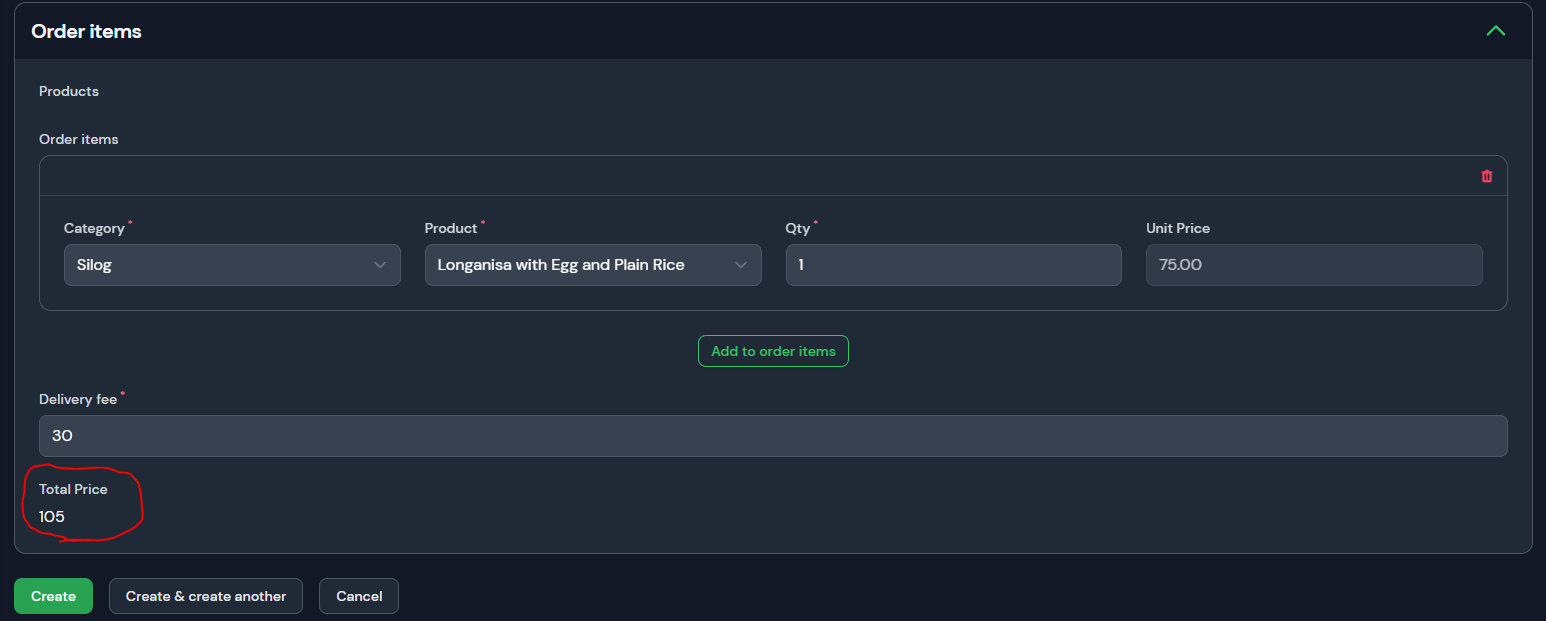
Can you show me the repeater code?
this is what I have so far, I was able to get the total for now but only if I set/updated the shipping_fee
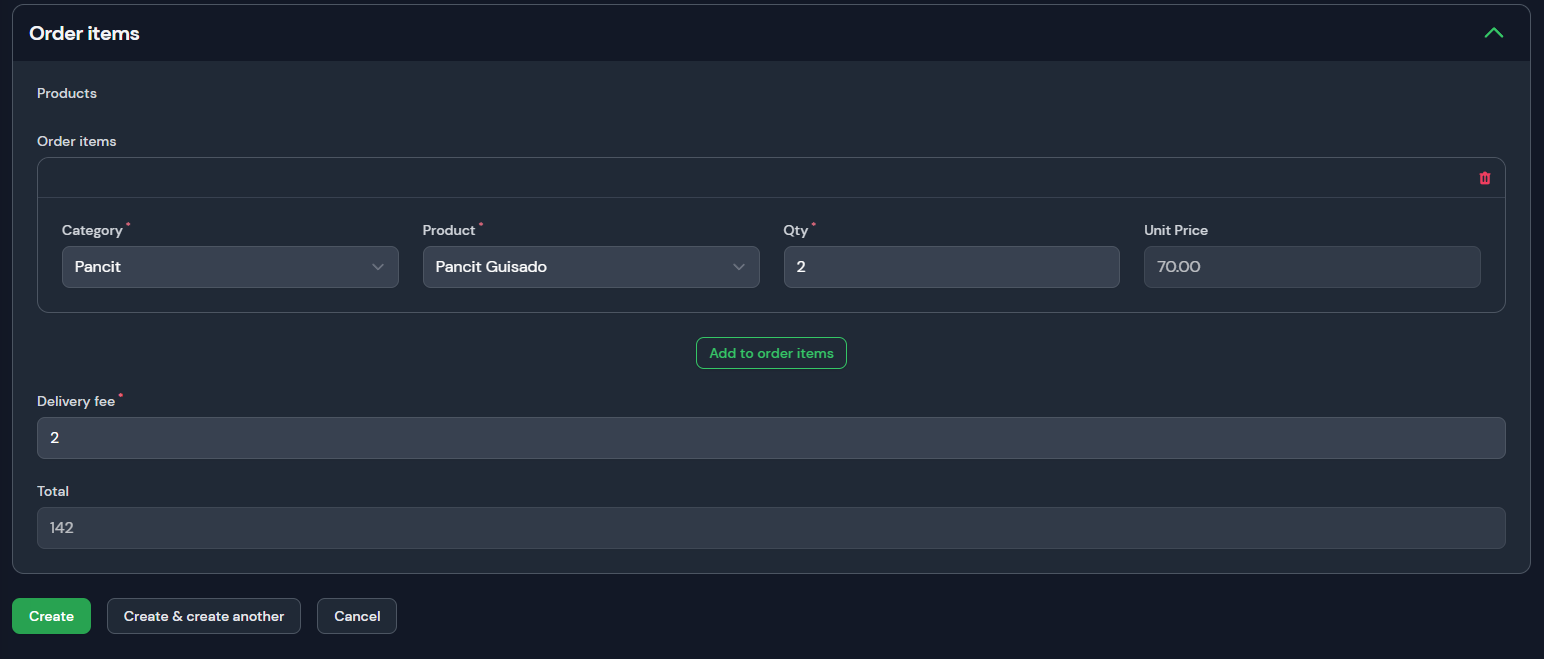
Yes because you only have afterStateUpdated on the shipping_fee field, you need it for product_quantity and price too
but when I tried it didnt work I think because total is outside the repeater.
That should work from inside the repeater
Make those fields reactive and use a placeholder field instead of `afterStateUpdated
He don't want a placeholder field, he want it as a TextInput
should I still use the orderItem collection? inside repeater?
Sorry, skipped that part
You probably have to
collection returns null but its working now,
I just need to used $get then do the '../../TextInput_outside_repeater', to get other the necessary values.
now I wont have to use Observer, idk which one is better but atleast for now its working.
Thankyou!
I didnt notice that It has an issue if I have 2 values on my repeater it will only get the total of the recent value.
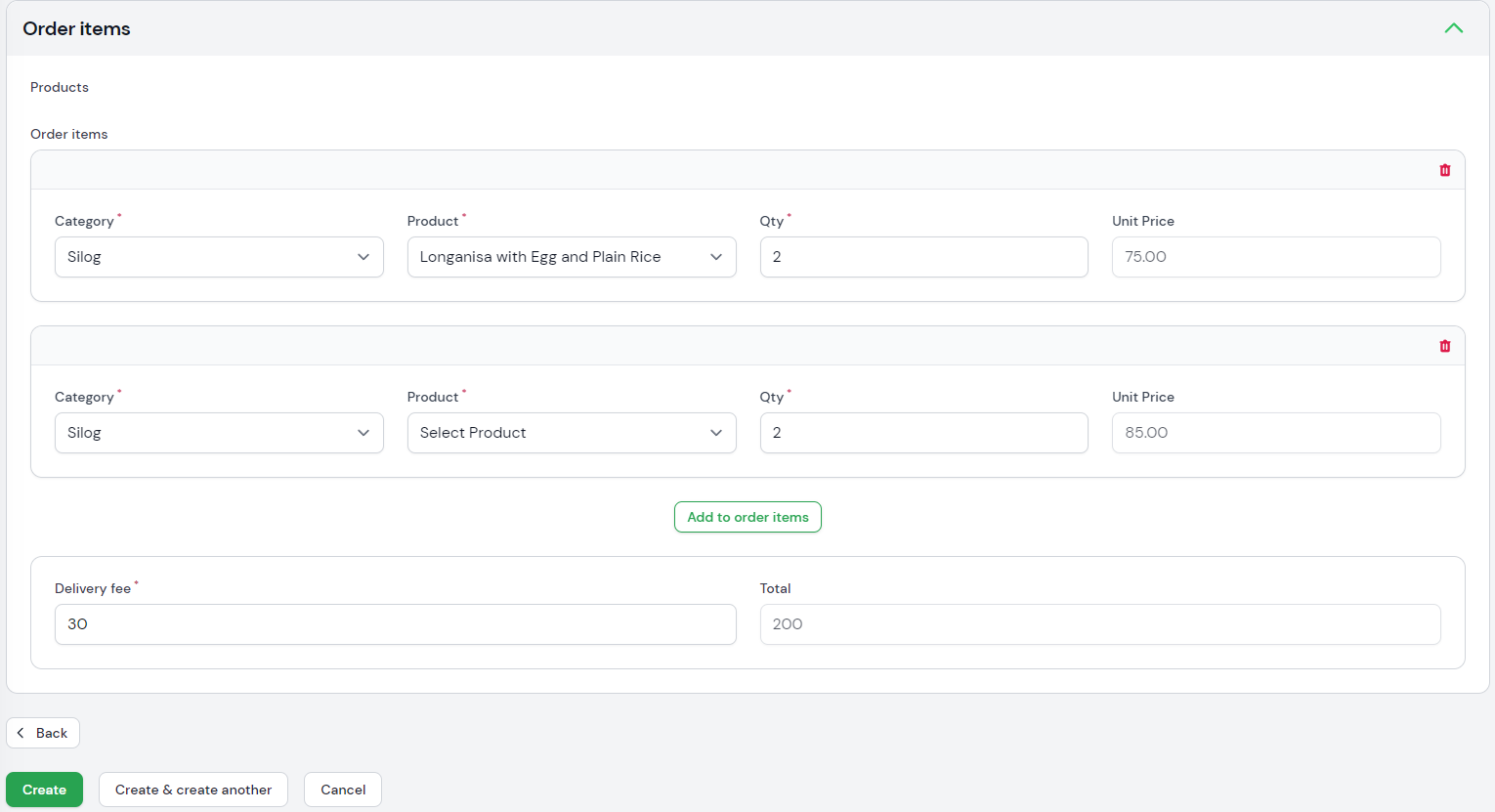