System.NullReferenceException in an if statement
I keep getting
System.NullReferenceException
directing me to the last if statement in the following code:
The problem is in _nodeContainer.TryGetNode...
. After I moved TryGetNode
from one place to another and added a new parameter, that error started appearing. Why does it always appear if all TryGetNode
's parameters are nullable:
26 Replies
_nodeContainer
could be null?Unsure what you mean, since nodeContainer is a method and it returns bool value
_nodeContainer
is a variable
Likely a fieldoh,
I see what you mean
lemme explain
here is what it looks like in code

I'm not that good at C# to explain it better
So it's null
The default value for all reference types is null
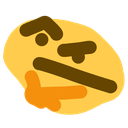
Because variables for reference types are essentially a pointer.
Null means they aren't pointing to anything yet.
That
!
is a hint, it tells the compiler "shut up about null, I know what I'm doing"I just don't know why it doesn't work only with my system if that way of getting a system is used everywhere in the solution
I might wanna do another way
that also works
What is that
[Dependency]
attribute?
Is it some field-based dependency injection framework at work?Fixing this might be as simple as:
But yeah I have no idea what that Dependency attribute is
I shift+clicked it and here is the code
Yeah, it does seem to be some weird DI framework
It’s RobustToolbox
a game engine for SS14
In that case, is the class you're injecting the dependency into registered in the IoC container?
can’t answer since I don’t know much about IoC and stuff sorry
Time to hit the docs, then
And see how IoC works in this toolbox thing
yeah, was going to visit a IoC docs page after I finish the thing Im working currently on lol
You could try not using dependency injection just to get it working
Currently trying another thing of getting a system for my class
the main reason I asked it here is cause the error didn’t seem clear to me
like uh
I didn’t get what exactly is null
also I just think that Dependency thing doesn’t work in classes that inherit Node.
Cause I can surely say it works fine for _EntitySystem_s
it finally works!
Appreciate your help @mtreit @ZZZZZZZZZZZZZZZZZZZZZZZZZ
Nice
Just had to use so called entitymanager to get the system
though now I’m really curious how IoC works
thanks once again!
IoC/DI generally works by creating instances of what you think you'll need at some point, storing them in a container, and then injecting those instances where they're needed
Basically, there's only one place where the things get created, so it's easy to swap them later if need be
For example, instead of having to swap every
var uploader = new ImgurUploader()
for var uploader = new CatboxUploader()
, you only swap services.AddSingleton<IUploader, ImgurUploader>()
for services.AddSingleton<IUploader, CatboxUploader>()
in one place
And everything that requests an IUploader
as a dependency will now get the new one