re-formatting duplicated data
so i have a db with the schema shown below
a
userPost
object consists of the following:
So:
- The user has created a posts
which has an id
, description
, createdAt
, expiresAt
- Within this, they have chosen to include some of their own user_items
, which are linked to this post via post_user_items
- Each user_items
has a corresponding items
based on the item_id
, and this contains the specific information about the item like its name
- They also choose generic items
from the database, which are linked to this post via post_items
I need to be able to retrieve a list of userPosts
where one of the items
linked via post_items
has an item_id = {something}
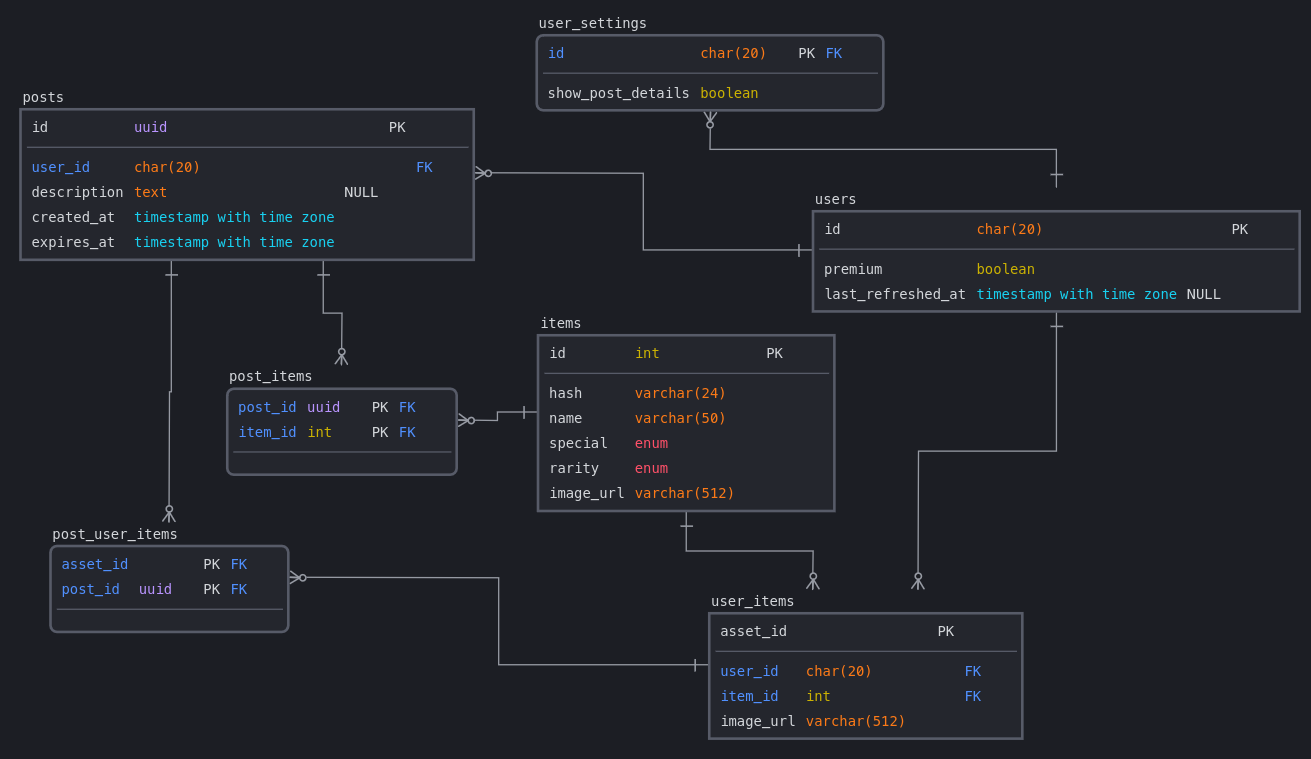
3 Replies
So far I have tried
but this returns one result for each
postItems
in the post, where everything is duplicated except the postItems
and itemsWants
are different
i have been banging my head against the wall for forever now
would really appreciate some help ❤️i guess i need this perhaps?
https://orm.drizzle.team/docs/joins#aggregating-results
Joins [SQL] – DrizzleORM
Drizzle ORM | %s
veryy confused tho
okay so this feels very close
however, how can i add a
where
that only returns userPosts
where one of the items.item.id = 1
almost?
just confusing
seems inefficient?
plz help
i wanna do something like
what am i doing wrong?
@Andrew Sherman any chance you could help when you have a sec please?
i don’t really understand what ${post.items}
actually is here
in terms of type, attributes etc