Pass value to custom field
I am trying to create a custom field. It's a chart where I can select a date by clicking on the x-axis. When I click on zoomIn or zoomOut, the time span should change from, for example, 30 to 60 days. How can I pass the days to the field?
This is my code:
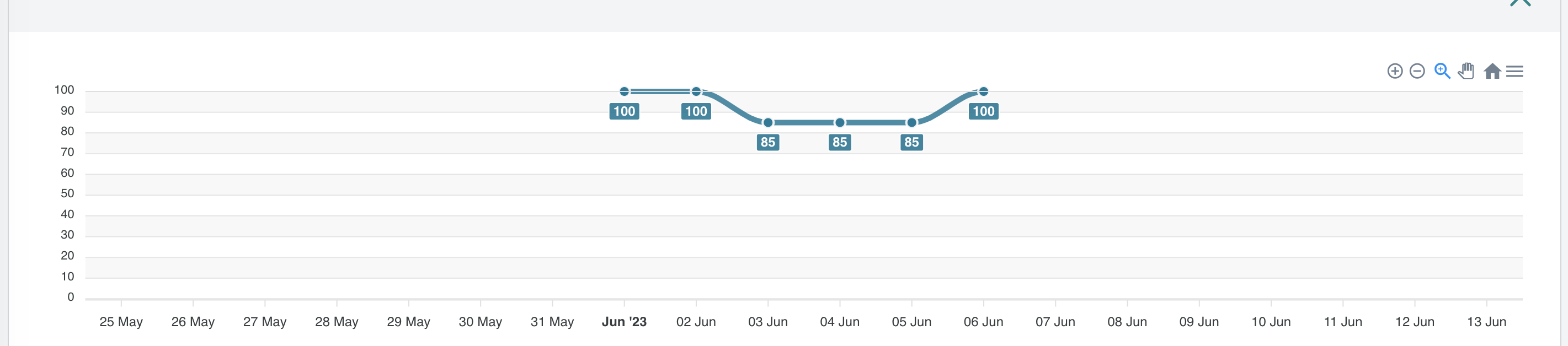
6 Replies
Have a look at some of the built-in fields like
TextInput
. There are a lots of protected properties with getter and setter methods (e.g. $type
, type()
and getType()
):
https://github.com/filamentphp/filament/blob/2.x/packages/forms/src/Components/TextInput.php#LL146C5-L146C5GitHub
filament/packages/forms/src/Components/TextInput.php at 2.x · filam...
Admin panel, form builder and table builder for Laravel. Built with the TALL stack. Designed for humans. - filament/packages/forms/src/Components/TextInput.php at 2.x · filamentphp/filament
You can use the same approach for the properties of your custom fields.
Thank you very much for your quick support. My problem is that I get the error "Unable to set component data. Public property [$days] not found on component" when I try to set the value with "@this.days = days".
I see! Your form field is not a Livewire component... I think
@this
only applies in a Livewire component template.Oh ok, then how can I use javascript to pass data to my field? Do I need to do this with "@js()"?
I don't understand the full context of your page but maybe you could refactor your code to use form events instead :
https://filamentphp.com/docs/2.x/forms/advanced#using-form-events