❔ [EF Core] Ignore properties from constructor of base class
Hey, I am currently trying to implement a new model in EF Core which looks like this:
In the current implementation, I only want to save the
LoggedIn
property to the database, because I don't need the other ones from the base class. They're just needed for the business logic in the code. So I tried to ignore them with the ModelBuilder
:
But I am still getting the error message:
That kinda makes sense to me. But I really need to find a way how just to ignore the properties from the base class, I don't want an additional database wrapper class or something. Is that possible?16 Replies
the issue is the constructor
how is EF supposed to construct your model when it doesn't have values for any of the parameters?
the properties aren't the issue
EF models should, ideally, not have a custom constructor
though, having a model like that (nearly all the props ignored) is really smelly
And instead of
.Ignore()
ing a bunch of properties... just make a model that doesn't have them
Exactlyyour db models should be db models and nothing else
also, even if what you currently had worked, that table has no primary key
okay, thanks for the tips guys.
so the better approach would be to create an extra e.g.
PlayerDB
class, which can be constructed from PlayerV
which just handles the database stuff?yes
and it also needs a primary key
some sort of unique ID
Unless the
Player
class you inherit from has italready prepared that for the
sadly, the
PlayerDB
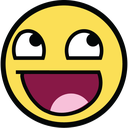
Player
class isn't even meant to be a database model. i just hoped i could make it fit for me, you knowLet the db generate the PK
Far as what is made to be in the db and what isn't meant to... EF models model the database
don't try to be clever with your db models
it just ends in pain
They should contain all that you need stored there
And only what you need stored there
.Select()
into other classes if need be
Or use an automapper
Or something elseugh please no
But EF models should just describe the database
okay i see, i'll keep that in mind. thank you guys so far
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.