How to I write an equation to raise a variable to another variable using a for loop?
I can't use Math.Pow() here, it has to be a loop. If there's an easier loop to use here, I would definitely like some help, lmao
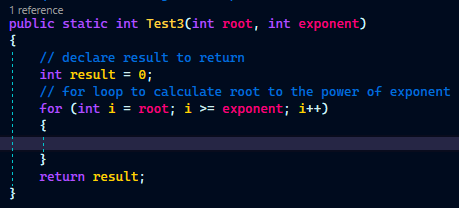
92 Replies
a for loop works for this
you just have to put all the other stuff in the right place
think about what you need to put inside the loop and how many times the loop needs to run
It needs to run 5 times
And I know the equation has to go in there (number^number) but I don't know how to write it in a way that actually works
what does it mean to raise a number to the power of another number?
multiply it by itself however many times the exponent says
sounds like something a loop might be good at
but I don't know how to write it like that
what, like root * root?
yep
(i'm assuming you aren't expected to handle negative exponents)
no
this is wrong, isn't it
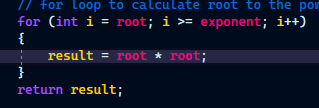
it's closer, but you're not including the result of any previous multiplications
you'll also have to change the first line to loop the correct number of times
so result++?
not really, break down the steps of something short like 2^3
?
2 * 2 * 2
right, then what steps are left to solve it
uhhh
just order of operations
you start with a 2, multiply that by 2, then multiply the result of that (4) by 2
root = root*root
?don't give answers please
pardon ?
aren't you asking for one ?
no
i'm helping someone learn to solve a problem
giving away the answer undermines that
maybe but if you know how to teach then not
let me try
if you don't mind which is
excuse me?
lol, you do
nvm then
but how would root = root*root work
good question
it doesn't
which is why people should only butt in if there's a good reason to
please don't use 3rd grade language
jimmacle#0000
you start with a 2, multiply that by 2, then multiply the result of that (4) by 2
Quoted by
<@!901546879530172498> from #How to I write an equation to raise a variable to another variable using a for loop? (click here)
React with ❌ to remove this embed.
see if you can translate this to code
not necessarily in a loop, but just write out that process
2*2=4*2
i mean code that will run in C#
I'm,,,, very new to this
sorry
that's why i'm breaking it down
so if you want to start with a 2, you could consider doing
result = root
now you getting it
then how would you make result have the value of for example, root^3
dude, i'm not the one with the problem. please don't interrupt unless you have something to contribute
i said
root = root*root
which was call to think for it
but you was rudebut that's wrong
so that's what i said
and not helpful to the person trying to learn
well as long as you can connect with him then sure...
the ^ operator doesn't work in c#
At least, my version of it
right, which is why i asked you to break down calculating the power to a more basic operation
which is multiplication
exponents are just repeated multiplication, that's what the assignment is trying to get you to implement
@CallMeSpriggy8033 let's fix your loop first
if that's fine with you
if you keep trying to derail this conversation i'm going to ask a mod to step in
why you so rude, learning from 2 won't make it lesser, but better also being rude is not going to do good for him
he can't fix the loop without understanding what he's actually trying to accomplish
don't call me, if you don't want to be my audience.
@CallMeSpriggy8033
Remember you wrote
i=root
at first step ?
in the loopso the for line is okay?
yup
...what?
but not the parts in it
no, it's totally wrong
the syntax was ok
which is what i was building to until you decided to be rude and try to take over the conversation
now read carefully
for(initialization, up-until, do-operation) {}
these are the 3 parts of the loop
initialization means, start from (From where the loop starts)
you are starting it from the root value but that is not right is it ?
you want to go up-until exponent, but that is also not written good
so let me break down what's wrong
first
initialization, From where the loop starts.
i
here should start from 0 (not 1, just remember that for now because of the language we use it's where the practices applies to) also it is based on other factors, but just remember that for now until you grown mature in it
up-until
the second part. Here you want your loop to go to certain number. Like the exponent. For example if you want to have exponent 5 then this will be
initialziation
= 0
up-until
= 4
so it will run as 0,1,2,3,4 (5 times starting from 0)
now 3rd part operation is correct how you handled. Which is increment by one or (++)
any questions until now @CallMeSpriggy8033 ?
let me know or we can move forward
So for example
or
consider the 5 or 4 in above loop as your exponent
makes sense ?@vitaljeevanjot I appreciate your attempt to help, but Jimmacle has this under control. When you jump in like you did while someone's trying to teach something, it gets to be really confusing for new devs.
Thank you, for the clarity however it thought it as public discussion so i was just filling the blanks but the reply from Jimmacle was a bit hurtful
From Jimmacle's perspective, he was trying to explain/teach a lesson and then you jumped into the conversation and took it over leading to much more confusion for the OP
I see, Yes Jimmacle did cleared this to me in the DM he just did
ok
i discotinue from here
thanks
I just want to figure out the equation for this loop head in hands
or whatever it is I need to write to ge this right
okay, so back to where we left off exponents are just repeated multiplication like you said at the beginning
consider the case
root ^ 1
, it's just root
since multiplying 0 by anything is 0, it's actually better to assume the exponent is at least 1 and put this case outside the loop so we have something to multiply with that isn't 0
like int result = root;
then in the loop we only have to think about the repeated multiplications
does that make sense??
so is the loop itself right or wrong
as it is now it's wrong, i'm trying to help you work out what the loop needs to do
so we've put the value of
root
in result
, now if we actually wanted the 2nd power we could add another line like result = result * root
right?
and if you wanted the 3rd power you could just copy and paste that line again, and so on until you get to the power you wantbut I don't know the power
right, that's where the loop will come in
instead of copying and pasting the line you can use the loop to make it run a specific number of times
so not like this?
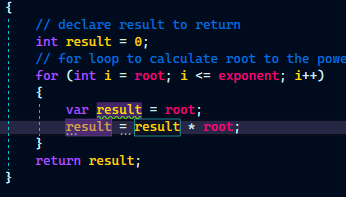
it's close, but you don't want to reset
result
to root
every time you loop
you want to keep building up the answer from an initial value
in this case you'd want to change int result = 0
to int result = root
and remove var result = root;
from inside the loopbut now it just returns the root number
which is expected, because it's not looping the correct number of times - we still have to fix this line

like vital was explaining, we basically want the for loop to count up to however much the exponent is
the first section initializes
i
to a starting value, the second part checks if the loop should stop, and the third part does something to i
each loop
so if you wanted to count from 0 up to exponent
what would that look like? (this isn't exactly correct but it will be closer)+1?
right, so that's what the third part is doing - every time the loop runs it will increment
i
by 1
but do we want to start from root
or something else?...something else?
right, so what do you think the
int i = root
part should be changed toI... don't know
1?
that's right (nearly), but do you understand why?
no
so when the code starts running the for loop, it will set
i
to 1 and then check your condition i <= exponent
to see if it should run at all
if that condition is true, it will run the code inside the loop, then run i++
to add 1 to i
then it checks the condition again and so on until i <= exponent
isn't true anymore
then the loop ends
does that make sense?yea
so the loop is basically counting up by 1 until
i
is more than exponent
but now it gives me this
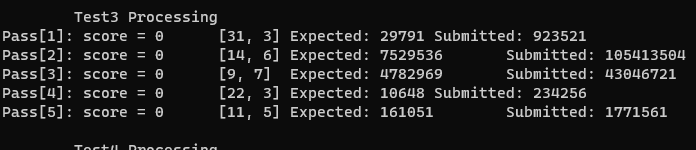
yeah, we have to account for the fact we set
result = root
at the beginning
for example, to calculate root ^ 2
we actually only multiply once
so the loop needs to run 1 less than the exponent< instead of <=
that's one way to do it, give it a try
yes!
It wokrs!
now this will depend on what your teacher expects, but if you try to calculate
root ^ 0
do you get the right answer?
i don't know if you're expected to handle that so it might not matterI don't think it does
might be a fun exercise to try and solve anyway 😛
but if not then it looks like you're all set
thanks!
would,,,,, you like to help me with a couple more?
there's one dealing with characters and strings that I'm not sure about
unfortunately i'm already behind on going to bed, but there are others who should be able to jump in and help if you make a new post
aight!
Night :)
o7