❔ ✅ Pass A Function To Another Function?
Let's say I have 4 functions. The first function is an error handler that needs to have the parameters of a string and a function. The other 3 functions are functions that execute code and have unknown parameters and parameter types. How would I pass those 3 functions to the error handling function so that the error handling function can call them over again when needed?
415 Replies
what does that even mean?
unknown parameters and unknown parameter types?
why does the error handling function meed such a thing?
what is the error handling function supposed to DO with that?
not trying to come off rude or anything, but when ya'll ask questions like that, I feel like I have to defend my code and why I'm doing something instead of just getting helping doing what I'm trying to do. If you go back to this thread https://discordapp.com/channels/143867839282020352/1112175696547168377 you'll see where I took a giant if/else statement and broke it down into separate functions. I'm now taking those separated functions and instead of having to write the same functions in each file, I'm putting them into one file named
ErrorHandlers.cs
so that I can just call the necessary error handling function when needed. This function that I've shown above needs to be able to call the passed function again while tries is greater than zero. An example is here https://pastebin.com/teHhQSkR <- in this file, I have 3 helper functions: InputInvalid, CheckInputIsInteger, CheckCorrectIndex
. The 2 later functions use the the InputInvalid
function which calls ScreenSelection
over while tries is greater than 3 to allow the user to see the menu and input their selection again. I've moved these 3 functions to a separate file to prevent from having to write them at the top of every single file I create within my project. Write once, re-use 100 times. The only problem with having my InputInvalid
function in a separate file is that it doesn't know which function to call while tries is greater than 0, so I need to be able to pass a function to it.
unknown parameters and unknown parameter types?In response to this, it is what it states. Passing function a to function b without function b knowing what parameters function a has. This allows it to be used for any function I pass to it and the function gets executed properly. I just don't know how to do that in C#. In python, it would just be
function_a(*args, **kwargs)
but C# is nothing like python so I'm asking for help on how to pass a function with unknown parameters and parameter types to another function so that the function can be called again
like function A could have a string parameter. function B could have 2 string and 1 list parameter, and function C could have 3 intergers and a string parameter but the error handling function doesn't know that but it still needs to be able to call the function that is passed to it whether it's function a, b, or cI'm not asking you to justify your code
I'm asking you to clarify what you mean
because that affects the answer
what is the error handling function supposed to do with the function you pass it?
is it supposed to call it?
this is answered in my original message
how does it do that without being able to pass parameters?
is it not supposed to call it?
then what IS it supposed to do?
the mechanism you need depends on your requirements
long time since i've seem you talk in the general chat how are you my guy
same as ever, I suppose
@Mekasu Can you explain your intent and what is your purpose?
I'm not doing that. I just wrote a f'n book explaining what I'm trying to do
Receiving "functions" without knowing what they are or what they do is a bit weird, you usually don't want to do that
and yes, if I think your approach here doesn't make sense, I will absolutely suggest better alternatives. After answering the initial question as much as possible.
right, now take that book and trim it down to something useful
or just read the thing.
calm down mate
i edited my message. i cuss as general term usage. I'm not upset
but it's as simple as it sounds. the helper function is going to be passed various functions with various parameters, so the helper function needs to be able to accept any function and call it again
it makes it so that the error handling function can be used universally without strict parameter types
what is the error handler doing with the function you pass it? Presumably it's going to call it? How? Where do the arguments come from?
You just can't do things dynamically as you want to do, unless you have some sort of "ioc container" that would automatically solve dependencies for you. That's just how C# works. When calling the delegate you'd need to pass down parameters specifying what they are in order for the function to work out.
You will have to type at some point.
not necessarily true
Enlighten me on how would a person do that without a dictionary and a lot of spare time 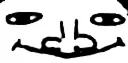
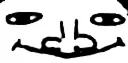
probably wouldn't RECOMMEND doing whatever dynamic or relfection solution is gonna be necessary for this, but it's most likely possible
ok lets try this
one moment
@Mekasu Can you just summarize for us what is your final goal?
In a shorter message you 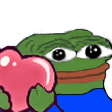
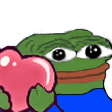
I've tried doing that and no one but me seems to understand what I'm saying so without being able to just share my screen and verbally explain, I'm going to use code. just a second
sounds good
this is my MainMenu
the functions ScreenSelection and LaunchGameScreen use helper functions
these are my helper functions
ScreenSelection passes information off to CheckInputIsInteger and CheckCorrectIndex which therein both use InputInvalid
The LaunchGameScreen function in MainMenu also uses InputInvalid
When the user inputs anything other than an integer, input invalid is called. When the input is invalid, it calls ScreenSelection over again to show the user the menu options and to allow them to try again
same with checking the index. it calls ScreenSelector over again when the number input doesn't match the list
yup
the LaunchGameScreen function also uses the InputInvalid function but in a slightly different manor. When it can't launch the desired screen, it tries 2 more times after that, but whilst trying it calls itself over again
by passing a function to the helper function InputInvalid, the function needs to know whether it needs to call ScreenSelection or LaunchGameScreen over again
however, ScreenSelection has no parameters where LaunchGameScreen has a string parameter
right
what do I mean by not knowing the parameters and types?
simple
More than just these 2 functions through my program is going to all use this InputInvalid helper function
but if you DO decide to call LaunchGameScreen.. how do you pass it a parameter
more specifically, what parameter do you pass to it?
those various functions are going to have various types of parameters, but I can't write the helper functions parameters to fit all those different functions as those functions needs their parameters to be called again
Is there any specific reason on why you're doing things like that instead of simply using a loop or controlling the flow of the app in a better way?
I understand what you want but I just don't understand why
in my original code, https://pastebin.com/WXJhPwBE, the functions were written for the file itself so I could just hardcode the parameters and function calls right there in them, but the helper functions are no longer being written in the files themselves as I found I was writing the same helper functions over and over again
why write them over and over again when I can just put them in one file and use them universally
we can DEAL with the problem of not knowing what the types and parameters are at compile time
but you still have to HAVE them
they have to come from SOMEWHERE if you want to call the function
so essentially you are trying to write an
IsInputValid
function that checks any and every input in your whole app because you are afraid that writing another method that validates certain input might be code repetition but it really isn't. Validating different inputs are different concerns and responsibilities. Seperate it and do oopI'm not even sure if that's the case
thats what I understand from wanting to take any params and calling any function based of that
I'll just put the input invalid function back in the files. I don't know anymore
yeah, let's stop overwhelming him
the question stands
just stop
where are the parameters coming from
without being able to get in voice and share my screena nd explain, I don't know anymore
I've tried to explain this the best way that I can and it's failing
I've tried showing code to help explain and thats failing to
so I just don't know anymore. maybe c# isn't a lagnuage for me
you've shown the code for the methods you want to wrap up and pass around dynamically
that's great
I haven't seen anything about the code where you intend to call whatever method was passed
when this function calls InputInvalid, InputInvalid needs to be able to call this function again
with the same parameters
so
there's the key
when these functions call InputInvalid, InputInvalid needs to be able to call the function over again from where the function called them to start with
InputInvalid does not need to call a function with any number or types of parameters
cause it doesn't have those parameters
it needs to call a function with NO parameters
and it's your job to give it one
InputInvalid needs an
Action
and the caller needs to do... whatever it wants
you can use an anonymous closure for that
This file contains 2 main methods. The first being SelectionScreen which prompts for user inputs and validates them. The validators at the top call correctly with decrementing tries for the user to attempt to get the input correct, or it defaults back to the previous screen. The second main method is at the bottom, LaunchGameScreen(string selectedAction). This function attempts to launch the desired screen and decrements tries if it can't until tries runs out and it defaults back to the previous screen. The problem with this file is that when LaunchGameScreen fails on attempt one, it recalls ScreenSelection instead of LaunchGameScreen again to give it another try. That's what I'm trying to fix. If it's not fixable to where both ScreenSelection and LaunchGameScreen can both use the InputInvalid function, then I'll put the countdown logic back into the LaunchGameScreens default case. I don't know anything about an action as the "basics tutorial" that I learned from didn't teach that so at this point, I'm lost and have no clue and just really do feel like giving up. This is just supposed to be a simple math game that The C# Academy has beginners doing. I have re-written this flipping game countless times over the last week and I can't figure this crap out and it's becoming too much.
it doesn't matter. I need a break. Thanks for what you guys have done. I'm going to bed. good night
I'm going back to the very basics and trying again. This time, it's a simple while loop
This loop continues for 3 tries giving the user 3 chances to get the right input. In python, I would write
so how do I do this basic while loop in c#?
I'm not going to give up just because it got tough
something like...
and @ReactiveVeina @Florian Voß and @snowy I'm sorry for my attitude earlier. I'm trying to get better at not getting so upset when I can't figure this stuff out. It's no ones fault but my own and I'm sorry
so I wouldn't use a while loop at all?
maybe
would it be more efficient to use a for loop?
if you want to put that upper limit in, a for ends up being simpler for tracking that
ok not a bad idea
the tricky part about using a while directly on the input is that the while evaluates at the beginning of the loop
so either you duplicate the code to retrieve user input, both before the loop and within it, or you put it only inside the loop with a custom break, and a condition that ensures the loop runs at least once
without the retry limit, it coupd maybe also be a do while
there's a bunch of ways you could organize it, semantically, most are equally valid
I went with this. thoughts?
I don't think that's right. From my point of view, this is giving the user 3 tries to input yes or no and when the loop ends, it's checking for yes but there is no check for no and there is no handle for what to do if they run out of chances
what about this?
so i moved getting the user input inside the loop, but its not recognizing when i insert no
or yes for that matter
you aren't actually repeating the user input retreival, on retry
and I honestly feel like this is too much code for what I'm trying to do
it's not
so how can I fix it in less code?
UI is hard
oh.
even text UI
so where do I need to put the user input capture for it to work correctly?
somewhere inside the loop, for starters
otherwise, it won't repeat
I did that
oh, missed that line
current code
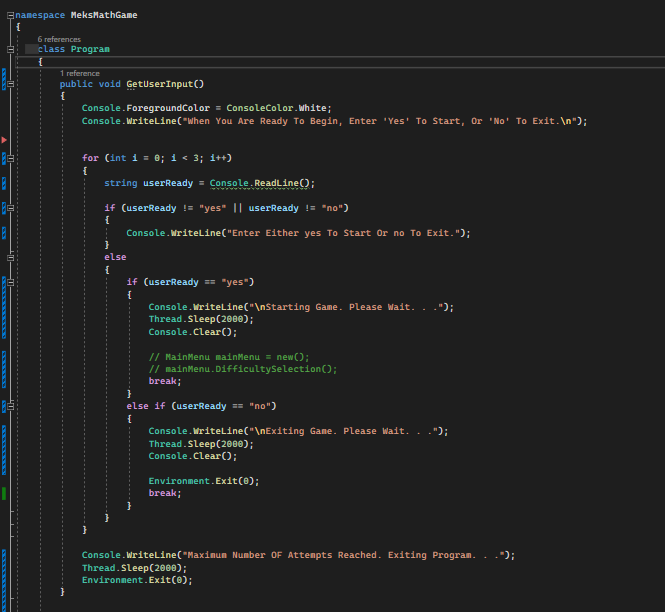
your retry condition is wrong
I'm not understanding how
I'm sorry
think it through
it's supposed to be if not yes or if not no
what happens when the input value is "yes"
it iterates again saying that it's wrong
why?
idk. fail safe against accidental key presses?
how does the condition evaluate?
right now, it doesn't matter if I enter yes or no, it fails
right
think through the condition that you wrote
how does it evaluate when the input is "yes"
if the user inputs yes or inputs no, it is supposed to trigger the else part of the if statement and then check if yes, then launch. If no, then exit
right, we know what it's supposed to do
I'm asking you to think about what it IS doing
^
^
same thing. It doesn't matter what I put in. RIght now it's just failing in general
the condition
how does the condition evaluate
apparently I'm just to stupid to get it because my answer isn't going to change. The condition is failing regardless as to what is entered.
yes
and you need to think it through to figure out why
youbhaven't told me anything about how the condition evaluates
evaluate this for me
with a value of "yes" for
userReady
that's an invalid expression due to the inner parenthesis
....no?
yes
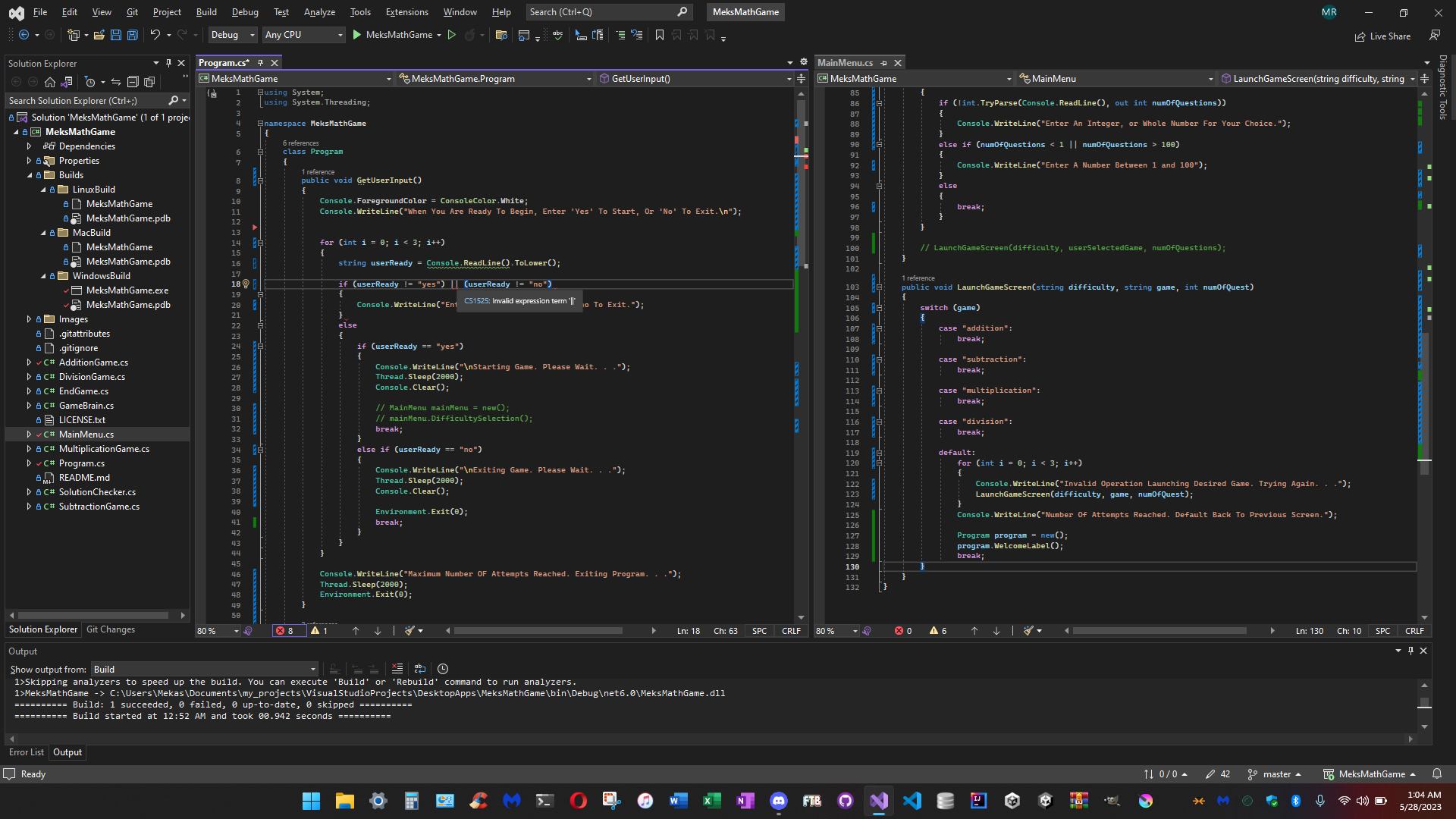
wait
I'm dumb
nevermind
that is invalid syntax for an if statement, yes
yea I'm dumb. forgot a parenthesis
in any case, I didn't ask you to write it in code
particularly as that is the code you already had
I asked you to evaluate it for me
ok at this point I'm just ignorant. I apparently can't
you don't know how boolean operators work?
||
I was told this was an or symbolindeed
which means what?
one or the other
to be colloquial, yes
and !=?
if (userReady != "yes" || userReady != "no")
if userReady does not equal yes, or userReady does not equal nothat is an accurate colloquial restatement of the condition, yes
what does that evaluate to when userInput is "yes"?
ok at this point I must be a great laugh or something because I seriously feel like I'm just going in circles. I've stated several times that it keeps failing. If I'm missing something then at this point just please tell me otherwise I'm going to trash the whole thing because this is becoming really redundant for me and I'm tired of feeling stupid
I'm not blaming you for my ignorance. I'm just expressing my current feelings
good
regarding expressing your feelings
I am asking you to evaluate something and you continue to tell me about your intentions for this code, and what you are experiencing with this code
you have yet to evaluate the experession I am asking you to evaluate
your question at this point should probably be "what do you mean by evaluate"
ok sure
what is the value of userReady that we're evaluating for?
yes or no
which one?
I'm checking to see if it's either of those
yes, that is the behavior you're trying to wrote code for
the code is not working
the behavior you are experiencing is that when the user enters "yes" your code does not behave as intended
so we are going to evaluate your code for a userReady value of "yes"
which is what I kept asking you to do
so, what is the value of userReady that we are evaluating for?
"yes"
the condition we're evaluating is
is "yes" equal to "yes"?
this shit literally has me crying like I feel so dumb
if the user enters "yes" then it's supposed to pass this check and go to the else statement
again
you are describing the behavior you want
we already know that the code is not giving you the behavior you want
we are trying to debug the code
which involves thinking about what the code is ACTUALLY doing, as opposed to what you WANTED it to do
stop derailing and answer the questions presented
is "yes" equal to "yes"?
yes
so, what does
userReady != "yes"
evaluate to?false if not yes
incorrect
i giv eup
whst is userReady?
a string and a variable
the value
that we're evaluating for
a string
the value
users input. jesus christs left toe my dude I don't know
yes
you do
the value of userReady that we are evaluating for is "yes"
because that is the value you put into your program that resulted in behavior you didn't want
is "yes" equal to "yes"?
and we're back to beginning of that circle
we are
"yes" is in fact equal to "yes"
so
(userReady != "yes")
evaluates to false
what does (userReady != "no")
evaluate to?all of my answers are continuously wrong so I'm just letting you explain. I'm not going to feel stupid anymore
your answers are much more "not answers" than wrong
if my statement is backwards, then just tell me that. If I need to put my true check at the top instead of the bottom then tell me that. Otherwise, this mulberry bush isn't going anywhere as I'm obviously not getting the point
you don't know what
(userReady != "no")
evaluates to when userReady
is "yes"
?i guess it would be false shrugs i don't know
is "yes" equal to "no"?
i guess not
then what does != return?
i guess false
!=
oh, hush, @MODiX
what does != return when the values being compared are not equal?
i guess false
don't guess
know
i don't know anything. I've been trying to get you to understand that and you won't. So therefore, I guess
if you legitimately don't know, go read the docs page
horseshit
you know everything you need to solve this problem or you would never have gotten this far
not to mention you proved you know EXACTLY how the != operator works when you answered effortlessly earlier
you are stressed and need to take a break or you are just being lazy and hoping I'll give up and give you the answer
it is neither. It's as simple as I'm obviously not getting it and unless some miracle happens, I'm not going to. It's literally as simple as
but it's obviously not going like that. I can't tell you why and this mulberry bush isn't helping.
so therefore
you call it a miracle, I call it learning
I'm not asking you small leading questions to try to annoy you
but it's obviously not doing that
I'm doing it because you WILL get it if you actually go through the effort to answer them
I know this. Hence me beating myself up instead of blaming you
neither is productive
I have been giving my best effort but 90% of my answers have been wrong so I just started guessing in hopes that I can get something right. This if statement should be just a simple as what I wrote above in both python and c# but I'm assuming that it's not. I don't know why it's not and I can't answer you on why it's not
which is why I'm helping you break down the problem into questions you CAN answer
what does
("yes" != "yes")
evaluate to?it evaluates to false, but that's what I'm trying to tell you. If the user inputs "yes" then it's supposed to trigger the else part of the if statement. I don't know why it's not
yes, I know
ok next question
you have told me many times in many different ways
what does
("yes" != "no")
evaluate to?f
f meaning false?
yes false
why?
well true because they're not equal
so, what does
false || true
evaluate to?falae
false
incorrect
omg
dones it evaluate to null?
"or" evaluates to
true
when either input is true
by definition
it only evaluates to false
when both inputs are false
so I need to check
if (userReady == "yes" || userReady == "no")
that would be one solution
and then invert the actual statement bodies
what would be another solution?
alternatively, switch || to &&
cause you don't want "either"
you want "neither"
wow......so simple
it usually is
understanding is ALWAYS more difficult and more valuable than just the end result
you're not wrong, but tbh I still don't fully grasp the difference other than the blatent statement that now
||
means either and &&
means neitherwell, that's a bit of a misnomer
&& means "and"
well I mean yea, but in the context of my check, its either neither
logically what you want is "user input is not yes AND not no"
which can be reworded as "neither yes nor no"
not yes or not no is a tautology
there is literally no input you could ever give that is equal to two different values at the same time
any input you give HAS to be not-equal to one of those two, because "yes" and "no" are not equal themselves
ergo, no matter what the user inputs, that if statement will always run
alternatively
it might be simpler to think ofbthe opposite
your valid values are either "yes" or "no"
so, in order to check for only valid values, you want NOT("yes" OR "no")
I.E.
which there is a law for in boolean algebra
I forget the name
I.E. if you want to invert an expression, you have to invert each term AND invert the operator
ok I got you. that makes sense
ok so help me understand what I'm doing here please. When I enter
addition
for my user response, it prints the else statement 3 times then goes to the next function asking me for the number of questions to answer
liek thatwell
I ought to ask you to walk through the code the same way
oh wait
I'm submitting a lower case response "addition" instead of "Addition" so it's causing the error?
well
yes and no
I would say so since I'm casting the check to lower and the list contains capitols
didn't even spot that
but that is indeed an issue
what did you spot?
when does your loop exit?
if the condition comes true, print the statement of difficulty and game selected then break
(based on the code written)
when does the condition evaluate to true?
when the user enters a game with a capitol first letter
which is in the list
right
so, it's based on the values of the user input and the list
where do those values come from?
but I don't know how to capitolize the first letter of the user's input incase they do it all lower case
the values are in the list
right, where does that come from?
it's the 4 games available to play for the user to pick from?
I'm not sure I'm understanding the question
where is
gameTypes
given a value?
and where does that value change?
in particular, relative to where the value is used, within the conditionin the list at the top of the function
those 4 values don't change
how about the user input?
userSelectedGame
it shouldn't be cast to all lower case, and that's my bad on that one, but I'm not sure how to cast an entire list to lower case, and I want them to have capitol letters for pretty printing
variable to hold users input
yes, where does it get its value?
in code
and where does that value change?
in code
particularly, relative to where it's used, within the condition
right here
string userSelectedGame = Console.ReadLine();
and changes?
it shouldn't. I removed the to lower cast that I had on the end
within the condition
but regardless, the users input needs to have a capitol first letter in order to correctly check against the list
that much I know
so, if the value never changes, and it controls exiting of the loop, how do you expect the loop to ever exit?
learn how to capitilize the first letter of the users response, or lowercase the entire list
there's a reason I continue to ignore that whole point
it's irrelevant, to this one
however you end up testing the input for validity
if it's not valid, what do you intend to do?
ok what about this?
no that's worse.
uhhh, if you say so
the issue I'm getting at is definitely still present
what do you intend to do when the game selection is not valid?
the else statement
that's the mechanism by which you will execute code to do what you intend to do
what do you intend to do?
I don't know. I guess I just need to lowercase the items in the list that way I can cast the users input to lower to be able to appropriately check against the list. Otherwise I have zero idea
this has nothing to do with uppercase or lowercase or the list
then please just tell me the issue. I don't have it in me to continuously guess
and it's not, like, a math problem
it's your program
you tell me what you want it to do
when the user enters an invalid game selection
assume you have figured out how to deal with the case issue
cause you will
print list to screen
enter loop
request user input
if input in list, break loop continue to next function
if not in list, continue loop
well, that's exactly the code you wrote
that doesn't REALLY describe what you want to happen when the user enters an invalid game selection
if not in list, continue loop
at least I'm assuming so
again, you're describing code more than function
what does the loop do?
what does the user see?
what does the user do?
this
is that what you want it to do?
(if "addition" was invalid)
loop and ask again
where is the code for "ask again"?
the else statement
where in the else statement?
there is no break statement in the else part of the if statement so therefore it will loop again
so it's not in the else statement?
it's at the beginning of the loop?
and you're relying on the else to take you back there?
past these comments, I don't know what you want from me. I'm trying my best here
what I want from you is for you to think more about the code you're writing, and its purpose
you said your goal is that when the user enters incorrect input, they should be asked for input again
yes the loop iterates itself up to 3 times without a correct answer
show me the line of code that does that
the line of code thst runs to ask the user for input after incorrect input was previously given
if "the loop iterates itself up to 3 times" isn't the answer, then I guess there isn't one. I don't know
is it because my user input line isn't inside the loop
"the loop iterates itself up to 3 times" is a description of how you want this code to work, it is not a line of code
yes
ok great. problem solved. Now onto actually checking against the list correctly
the most proper solution is to use case-insensitive comparison
so what's the breakdown for that line. Like how does that help me understand what it's doing?
when calling into code that isn't yours, the first answer to "how does that work" is pretty much always "docs"
then ill just go to the docs
Enumerable.Contains Method (System.Linq)
Determines whether a sequence contains a specified element.
in short, the .Contains() method does exactly what its name suggests
and what you were already using it for
I know what contains means. I moreso meant the StringComparer.OrginalIgnoreCase part
if you want to adjust the rules for what "equality" means when it is looking for an "equal" value, you give it an object that implements the
IEqualityComparer<T>
interface
I.E. an object that performs equality comparison for .Contains()
what you want is for string equality to be not case-sensitive
and conveniently, .NET provides a ready-made class that implements IEqualityComparer<T>
for exactly that rule
as well as a variety of other rulesStringComparer.OrdinalIgnoreCase Property (System)
Gets a StringComparer object that performs a case-insensitive ordinal string comparison.
ok I got you.
StringComparer.OrdinalIgnoreCase returns an instance of the StringComparer class, which implements
IEqualityComparer<string>
, for "ordinal and case-insensitive" comparison rules
"ordinal" basically meaning "binary ordering and equality"
sort of
that part is really irrelevant hereok so a different use case of a check system. Here I'm checking if the users input is an integer and if it is an integer, then check if it's between 1 and 100. The only reason I'm confused on this one as since we went through the other two is because of the boolean case with trying to parse the input.
would I do it similar to the others in the sense of
i feel like I got this one right
by my reckoning, both of those are equivalent
what do you mean?
all you did was rearrange the if/else blocks
but they're both functionally the same
you have three mutually-exclusive scenarios and are executing the same chunks of code for each one
and I found a problem here. When passing the selected game to the next function, it's not passing it as "addition" or the item in the list so since we used that
gameTypes.Contains(userSelectedGame, StringComparer.OrdinalIgnoreCase)
for the comparison check, how can I use that to pass the correct game from the list?
ok so how can I fix it to be correct?they're BOTH correct, that's my point
both lf those code snippets are functionally correct
they are doing functionally the same thing
well look at me doing something right on accident 😛
I mean, REALLY, the answer is to use an enum
but you could get away with using IndexOf() instead of Contains()
I THINK that has an overload for an IEqualityComparer
because right now the problem is that this function just jumps straight to the end thing of cancelling the application
or is it doing that because I don't have something right in another function?
no, your assessment is right
after messing around with the print statements of "Maximum Number Of Attempts Reached. Exiting Program. . ." I have determined that the issue is in this function
as in, that's the
Environment.Exit(0)
call that's being hit?right like I enter a number for the number of questions to be answered, it accepts it, triggers the appropriate print statement in the LaunchGameScreen function, but then continues the loop and errors out after the fact instead of breaking the loop
breaking what loop?
the one in GetDesiredQuestions or in LaunchGameScreen?
Launching Addition Game. Maximum Number OF Attempts Reached. Exiting Program. . . C:\Users\Mekas\Documents\my_projects\VisualStudioProjects\DesktopApps\MeksMathGame\bin\Debug\net6.0\MeksMathGame.exe (process 7572) exited with code 0. To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically close the console when debugging stops. Press any key to close this window . . .like here, it accepts my number for number of questions to be answered, goes to the next function of launching the corresponding game screen for the game being selected
Launching Addition Game
but instead of stopping after the print statement, it's going back into the GetDesiredQuestions function and triggering the environment exit partyes, exactly
why would it stop?
I would guess it's because the loop doesn't break when it triggers the next function, it pauses instead
with the way we've been setting this up, the loops don't technically break, but pause instead because of the next function call before the break statement
where is the line of code that is going to cause "it" to stop?
that's fair to say
ok so for example
when this condition renders true that "yes" has been answered, it's supposed to break the loop and go to the next function, but instead, it's pausing the loop and going to the next function so when that next function, in this case the LaunchGameScreen, reaches the end of it's code line, or error out, it goes back to that paused loop and then breaks and exits the environment when in reality it shouldn't be pausing the loop. It should be stopping/breaking the loop and continuing
the loop is only there to give the user another chance at entering the correct answer. Once the correct answer is given, the loop shouldn't exist anymore
yes, that is what's happening
ok so I've got my structure wrong for this whole thing
"wrong" is relative
if it's not what you wanted, it's not what you wanted
it certainly COULD be made to work
true. I'm just trying to think of how it needs to be written to give me what it's supposed to be doing instead of what it's currently doing
it could but then I'll have a program full of paused loops and I don't want that
well, it's rather straightforwards
that's not nearly as insane as you make it sound
and it may not be, but I just don't know if that would be efficient
that's... how call stacks work
if that's the correct term
I would say it's not well-orgaized
that's an opinion, but I think O can safely say most folks here would agree
ok so walk with me here. If I were to take where I call the next function before the break statement and put it outside the for loop, then where would I tell the for loop to exit the program after 3 tries?
you do as well, you just don't have the wisdom to put into words what's undesirable about it
like I said, it's rather straightforward
you already said it yourself
you want the loop to break before you call the next function
so you need to do literally that
cause right now you have
this will break and then call the next function, but now I have no where to tell the program to exit after 3 tries of incorrect answers
right, but either way, after 3 tries that NextFunction(); is going to be called once the loop finishes
this is indeed correct
so, you need to be able to detect HOW the loop exited
ok I'm listening
cause it can exit multiple ways
and what you do next depends on which one
or
stick with what you have, and maybe just clean up the semantics
well before I tired doing this with loops, I had this
you had callback delegates, yes?
that's not a terrible approach
if I were to really workshop this myself, I would probably do something with callbacks
this is what I had before, but I was trying to clean my code up and not make it so elongated
still looping, but recursive
essentially
in your honest opinion, should I go back to that?
not that, no
I'm ngl. I don't know how to do callbacks, or at least I don't think I do
but the whole deal that started this thread?
probably something like that
so helper functions
like that then?
fixed it
fixed it again lol wrong call at the end of the loop
going with that methodology, in this instance, userSelectedDiff at the bottom is not reachable as it's not in the same scope, so how would I get access to it?
🤯
like boy. that couldn't be more above my head than an airplane in the sky
honestly, mostly the same concept as the callback idea, but implemented with inheritance instead
ends up being cleaner, I think
yours or mine? lol
the callback is the OnExecuteTrying() method
mine is with inheritence, yea?
that TryExecute() calls
uhhhhh, no
there is no inheritance anywhere in your code
but, like, your original thought was to dedupe code
your peril was that you couldn't adequately identify whatnitbwas you were wanting to do, and what you wanted to dedupe
I mean yea, but what you wrote completely threw me out the ball game. I wouldn't have any idea what to write with that, where to put it, or how to use it
what you really WANT here
or at least, what you have the opportunity for, is to dedupe the logic you have repeated for retrieving user input
did you even see my attempt? lol I'm like a 1st grader and you're in the master's program lmao I can't even get access to my own variable
in particular, the logic for retrying the same user prompt until they get it right, or run out of tries
that can absolutely be deduped
if callbacks make more sense, we can go with that
idk if this is callbacks or not, but what I wrote here makes sense to me. I just can't get access to my own variable outside of the for loop
which one?
string userSelectDiff = Console.ReadLine();
it tells me that in line
Console.WriteLine("\nDifficulty: " + userSelectDiff);
doesn't exist within this contextindeed
I tried doing
public string userSelectDiff;
but it didn't like that at allyes, that would be invalid syntax
ok so learn me ObiWan
lol
you defined it inside the scope of the for loop, so it only exists inside the scope of the for loop
well doing
just cause a whole bunch of red lines so I'm not understanding
what red lines?
cause that is exactly what you want
uhh
yeah
what is userSelectDiff?
that doesn't exist in ANY context
I don't know. I tried to instantiate it outside of the for loop so that the bottom code could have access to it. I honestly don't know
well, you wrote it
what is userSelectDiff?
user input
in code
the variable that holds the console read line to prompt for user input
k
where is that variable defined?
inside the for loop for proper calling when the input is wrong
show me the line of code
what do you mean? it's right there a few messages up
it is, in fact, not
which line do you THINK is the definition for that variable?
right here is the code
yes, I am aware of the code you wrote
ok so the variable userSelectedDiff is in that code block right there, first line inside the for loop
I don't know what else you want me to say other than to copy that single line and repost it when it's already right there
yes, do that
also, pick which block of code we're talking about
because I thought we were talking about the one you posted with the errors notated
but that's not what you linked me to
this one is the same as https://discordapp.com/channels/143867839282020352/1112207639976345600/1112306053984550912 <- this one. The only difference is, this one (hyper link) is a small snippet of the replied to one. apologies
great
that's my bad. didn't realize we we're on two separate pages. sorry
which line do you think defines the userSelectDiff variable?
paste it
^
that line does not appear in the snippet
well no duh. In the snippet is where I tried to instantiate the variable outside of the for loop as stated here https://discordapp.com/channels/143867839282020352/1112207639976345600/1112306520814792798
yes
and that snippet has errors
errors telling you thst userSelectDiff is not defined
in that snippet
because it's not
yes. that's what I've been trying to solve
so, why, when I asked you which line from that snippet do you think defines the variable, are you giving me lines not in that snippet?
because as I stated here
so
are we trying to solve the errors in the snippet that contains errors, or not?
ok so as my friend stated, this a chess game for us. Neither of us know each other, and you don't know my experience with this language. I have only been doing C# for like maybe a week now. I'm used to working in higher level languages such as Python and JavaScript. This is all brand new to me working in a lower level language such as C#. I'm not trying to be an asshole and I'm sorry If I'm coming off that way, but none of this shit makes any sense to me when it should make all the sense in the world. I apologize for my part
I'm just trying to get the code outside of the loop to have access to the userSelectedDiff value
how this currently sits, I cannot pass the selected difficulty to the next function
alright
yes
we already determined this
you proposed a solution
and I told you it was the correct solution
move the variable definition outside the loop
you said it had errors
so we need to solve those errors
^ that brings us back to this https://discordapp.com/channels/143867839282020352/1112207639976345600/1112306053984550912
and did you read this?
yes
there is no need for you to apologize for things you don't know
the only thing you need is focus
which is irrelevant of what languages you do or don't know
the question stands
in that snippet
ok so moving the variables instantiation outside of the for loop causes a lot of undefined errors. How can I solve that
the snippet with errors
yes
the errors are telling you that userSelectDiff is not defined
correct
in that context
where is the line that you think should define it?
in that snippet
the one with the errors
this one
This is having it defined outside of the for loop with the errors so I don't have to keep scrolling up
k
I don't know unless it is in the class as a public variable
but that doesn't seem right to me
so
you wrote code that references a variable
named userSelectDiff
and you never considered that you need to define it
tbh I thought
string userSelectedDiff;
did define the variable.no, that defines
userSelectedDiff
also
when I asked "what line of code do you think defines it"
^
you in fact DID know the answer
as you should
you are the only one that can tell me what you thinkbecause when I said that, you said "references a variable" and that I "never considered that I needed to define it" so I didn't know that
string userSelectedDiff;
defined it
I thought it referenced it
or am I just lost againapparently you are, because just a moment ago, you said you thought that line defined it
now you're saying you thought that line referenced it
regardless
defines a local method variable named
userSelectedDiff
of type string
it tells the compiler that it exists, and what it's scope is
you then proceed to never use this variable
at the same time, you are repeatedly referencing something named userSelectDiff
that has not been defined in any scopeI've got about 15 more minutes for this to make sense or I'm just giving up with C# altogether. This language shouldn't be this hard
this has UTTERLY nothing to do with C#
if I defined it, but never used it, then what does
userSelectDiff = Console.ReadLine();
inside the for loop of the snippet mean thenthat is you reading user input from the console and attempting to store it into something named
userSelectDiff
which has not been definedthen how does it need to be defined?
how do you want to define it?
like
what do you want it to be?
and what type?
the code outside the for loop just needs access to it
that simple
right
so, you want it to be a local variable
outside of the loop
sure
what type?
whatever type gives the code outside that for loop access to it
type has nothing to do with accessibility
what value do you want to store in it?
what does Console.ReadLine() return?
my guy, how do I need to write that variable outside of that for loop so that the user prompt can get the user input and the code outside that for loop can access that input
what type do you want the variable to be?
whatever type gives the code outside that for loop access to it
type has nothing to do with accessibility
then don't ask me what type do I want it to be
I have to
you can't define a variable without knowing its type
a variable definition consists of three things
its name, its type, and its scope
we know what name you want
we know what scope you want
what type do you want?
what type does Console.ReadLine() return?
if before it was a string when defined inside the loop, what makes you think that's going to change? It's a string. It's obtaining a string. It is a variable to hold a string
so
string
that's all the pieces
you already knew that
I did
jesus take the wheel
what's the syntax for defining a variable?
you tell me
do you think it's changed since the last time you wrote a variable definiton?
Variables - C# language specification
This chapter covers variable categories, default values, definite assignment, and variable references.
so, what's the syntax for defining a variable named
userSelectDiff
of type string
?nope. it hasn't changed. Just tired of feeling stupid and like I'm going around circles because you continuously want me to answer questions that we both already know the answer to just because you call it learning. Like I'm good. If you won't show me a code example to visibly show me what I'm doing wrong with a simple explanation, then I'm no longer interested. I don't want the answer handed to me, but I'm not going to continue to feel like I'm going in circles of something simple or what could be simple. I know how to instantiate a variable. You already know I know how to do that so it's pointless to ask me again. You already know that the variable is holding a string but yet you asked me again. Like don't do that. Either show me an example with an explanation or I'm just done. I'm tired of feeling stupid and I'm tired of circles
the only reason we're going in circles is that you keep sending us there
yep my fault. thanks. good night
you say you know how to define a variable, and yet I told you a long time ago that your definition was wrong
ok
you say you don't want to be handed the answer, and yet when I break the problem down into small steps that you can use to get to the answer, you'd rather complain about it
again my fault. cool beans
there's a difference between being at fault for not knowing something (which is never valid) and being at fault for not being willing to think for yourself
the reason I ask you to answer small simple questions is that you A) can B) should eventually be able to ask them for yourself, on your own, and C) will ALWAYS remember things better by doing, rather than simply being shown
earlier, when the question was "how do I deal with uppercase in the user's input" I didn't go through this
I straight up gave you the answer and linked you to docs
well I no longer understand what you want from me. I have tried answering your questions only to be wrong 100 times. I've tried giving my best shot and yet here we are multiple hours and have yet to move off the first 2 files of the program. Apparently it is my fault if all I'm doing is complaining. So it's cool. I can just as easily go back to a language I know and achieve the same thing. I'm learning this language to simply have another language under my belt but I can so much more easily create the same game in the other languages I know so again, it's cool.
because it wasn't about taking knowledge you already have and learning to apply it, it was about the existence of library code you have no knowledge of
and again, THIS problem has nothing to do with the language
ok
variables exist in Python and JavaScript, and are defined the same way, save for types
yep
you are attempting to use a variable named
userSelectDiff
which you have never defined
you want it to be a string and have scope outside of the for loop
the syntax for that is
put that outside of your for loopif you will look at this god blessed code block, you will see that I did that! I've been done that! That's what is causing the errors for the rest of the variables usage inside and outside of the for loop!
you, in fact, have not
what you have is
oh this has to be a joke. Do explain what the difference's outcome is going to be before I lose it
userSelectDiff
and userSelectedDiff
are not the sameI'm not that slow
so again, please expaling what the differences outcome is going to be
uhm
well
what is it going to do with removing the
ed
from my variable outside the loop going to help with the variable inside the loopwhen you change userSelectedDiff to userSelectDiff, the variable userSelectDiff will be defined
and the code will compile
because the compiler will know what userSelectDiff is when you refer to it inside the loop
if you would have simply stated that my variables had different names AT THE START of this, we could have avoided every single bit of this. I'm not remedial and that would have made IMMEDIATE since.
and because it is defined outside the loop, your code later on after the loop will also be able to refer ot it
this is what I'm talking about. going in circles over something simple. It would have been a simple response to just say "it cant get access to it because the variables don't have the same name"
see how simple that is and so easy to understand instead of having to answer all those questions like a remedial toddler
that
simple
I'm done at this point. Like I'm done
the fact that you were willing to spend 2 hours arguing with a stranger without any effort to inspect your own code for yourself for trivial errors shows that you needed more than just me pointing out a typo
Mekasu, ReactiveVeina was trying to make you ask the questions yourself to see what the problem is. You were not open to help at all. Reactive has been VERY patient in this lengthy thread.
Sorry for intervening like this
I ask you small trivial questions because those are the questions you will ultimately be capable of asking for yourself
so it's my fault that they couldn't have just said the variable names didn't match
you're joking
because those are the questions I asked myself to spot the error
You dismissed every question and didnt take a second look, you would have seen the error if you asked the question yourself. Also, what IDE are you using? Something like this would show up
the fact that you were willing to entertain a 2 hour long arguement that could have easily been avoided with a simple "you have a typo" or something relative to it just shows immaturity. I do get that you have been patient with me, and for that I appreciate you, but to have all of that happen when it could have been avoided with a simple statement is just....wow..... and it's not that I "didn't take a second to look". It's the fact that it was a small oversight for me, but I guess that accounts for nothing because it doesn't matter what I say in my defense, it just going to continue to circle back to being my fault. This is why I stopped asking for help in the first place and never should have come here for help either because no matter what its always my fault so I'm good. I no longer care at this point and I won't be reading any further responses unless I'm forced to.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.