"FOR"
I have this code:
I somehow need to make it so that I was shown both the price of pizza and its amount. I ran into a problem: the whole array is shown to me, and not the value determined from it. How can I extract this particular value for each pizza?
34 Replies
well you have a nested loop
so the outer loop
i
will run 6 times, and the inner loop will run 6*6
timesIt turns out I need to make them not nested?
in fact, you just need one loop
how exactly can i do this in one loop?
Well, both
foodPrices
and foodAmount
have the exact same number of items
and i
is just a number, it has no direct association to either array
(I'm not going to hand you the answer, but you should be able to figure it out from here on)I'll think about it
you can literally just remove the inner loop, but keep the two writelines.
I came to a working way.
Is it must be like
yep!
Thank you for your help!
yw.
btw, a more idiomatic C# solution to this would be to not have two arrays of prices and amounts
but rather have a single array of "Pizza" class/struct that represents both values
Something like that?
something like this
we can make our own types
and you should be taking advantage of that 🙂
I'm just a beginner in C#, and I didn't even think about a solution like that.
Anyways, thank you!
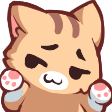
Thats fine! Im just telling you the "idiomatic" solution
Here another problem come. I have the same code, I just added a new
string
parameter called name
. Since I have a pizzeria, you have to buy pizza in a pizzeria, so I wanted to make such a mechanic. But just by calling food
in the condition, I will not be able to get "Name" from it. How can i do this?can you show your full code?
or did you base this on my code above?
Yeah
well,
food
is not just one pizza, its all of them
so you need to see if one of them match the provided name
you could do this with a loop, like before, but there are some very helpful methods for this kind of operation alreadyI need to make sure that I can't buy pizza that doesn't exist.
sure
I hate the translator, lol. I mean I want to make that I can't but pizza that doesn't exist
But I'll think you understood me
I understand what you are trying to say, np
I changed
Pizza
a bit, since we'll likely want to change the value of Amount
in the future
and record struct
would make that... awkward. Better to use a normal class here
the real magic is these lines:
I have not met var. I understand that it can store data of any type?
no, it just means "figure out the type for me"
in this case, that type is
Pizza?
Oh well, I take it that's pretty effective.
its entirely optional, I personally use it a lot
but I can see why a beginner would prefer to type out their types 🙂
Could you explain how these lines work?
the important part is understanding that there is no difference in type safety here - they are both the exact same thing being compiled
sure
FirstOrDefault
will return the first item in the collection that matches the predicate
function
the predicate being x => x.Name == userPizzaInput
here
thats a "lambda function", here its a function that takes in a Pizza x
and returns true
or false
the first item to return true
will be returned by FirstOrDefault
if the end of the collection is reached and no item has been returned, it will return default
, in this case null
(because Pizza is a class)That's really interesting. Thank you!
There are TONS of similar functions!
First
, Any
, All
, Single
, Where
, OrderBy
etcBy the way, I didn’t understand how Pizza turned into x, and why it’s x at all.
is, essentially, a shorthand for
got it! thanks!