Action that changes value in Form
When the action Accept is pressed, the dropdown in the form should change to "Yes"
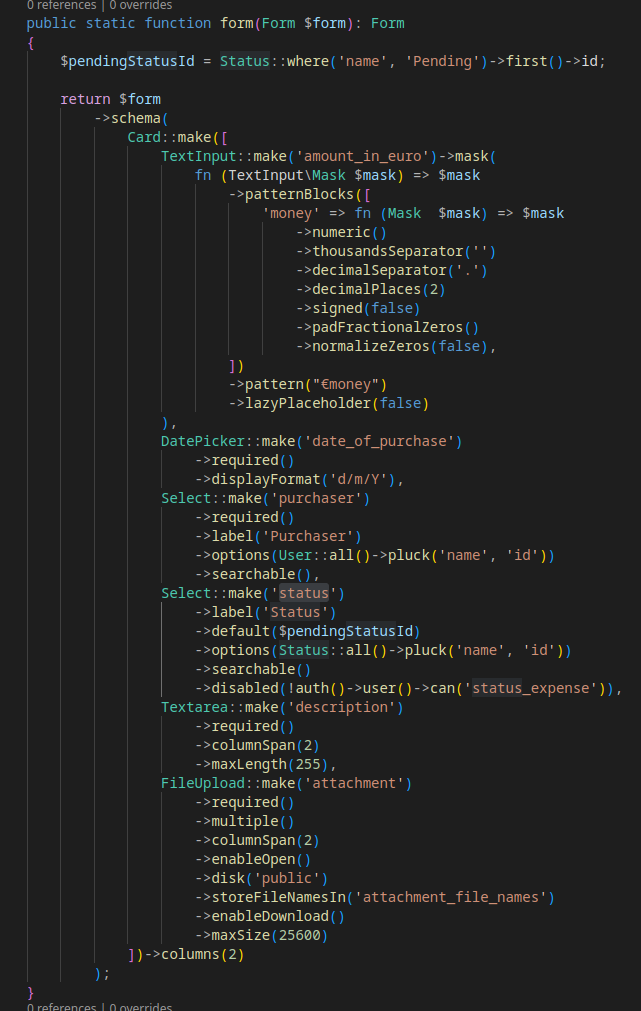
12 Replies
So far, when I click accept nothing happens
If i use this
Then I get this error
App\Filament\Resources\ExpenseResource\Pages\EditExpense::App\Filament\Resources\ExpenseResource\Pages{closure}(): Argument #1 ($record) must be of type App\Models\Status, null given,
you need to get the status from $data
$record doesnt exist yet
But, im on the edit page. Doesnt that mean that a record exist?
you can use $this->record
like this?
Its still giving an error

using phpmyadmin/tinker I can verify that the "Yes", exists
please read my code carefully
you did not copy it
you're right. I still had the previous copy
Could you please explain why we use $data in this case and not $record?
we only pass $record to the action in some places. mainly table actions. we should probably pass it in all places, but it will always be identical to $this->record on edit/view pages
But now, how do we know that we are changing the value of a Select in the form?
figured it out:)
Sorry for asking so many questions Dan but I dont have a lot of experience with Laravel/Filament or PHP