Dropdown access depending on role
In this case, I want the dropdown "Status" to be locked to the Option Pending, and only users with the role super-admin can change it.
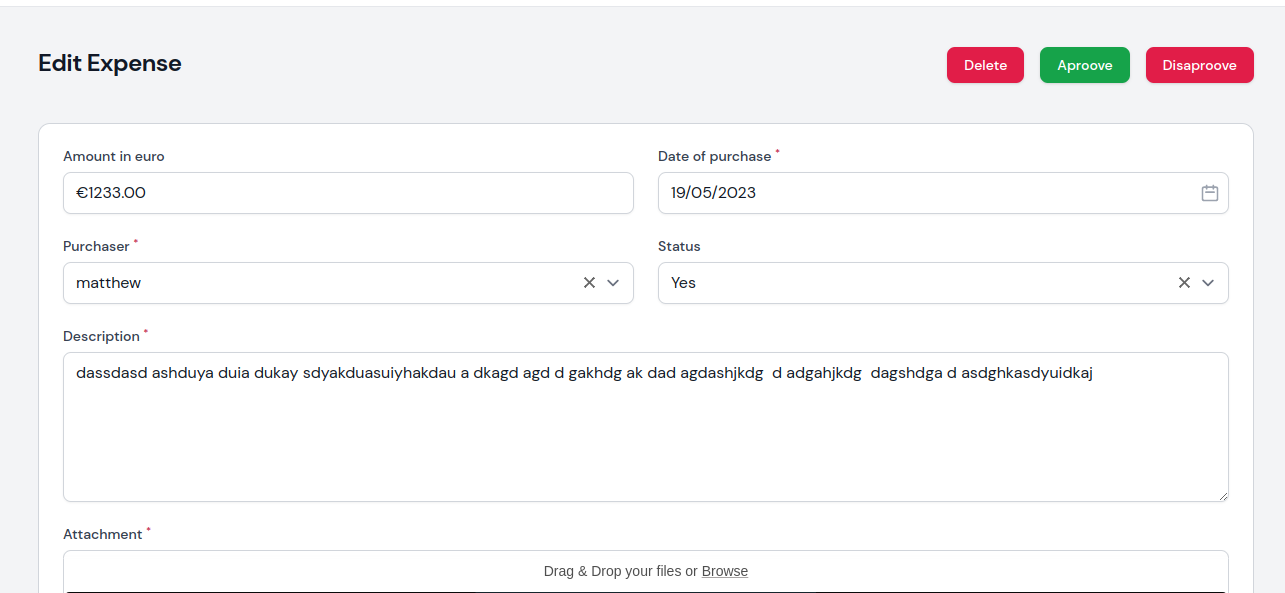
43 Replies
->disabled()
->dehydrated()
?
Yeah but how can I lock the dropdown, based on the role that is logged in?
are you using spatie permissions?
Im pretty sure, yes
->disabled(auth()->user()->cannot('super-admin')) ?
Im afraid not
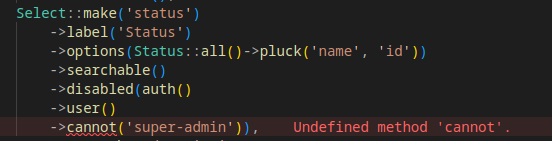
try !can, or !hasRole
They dont exist as options:/
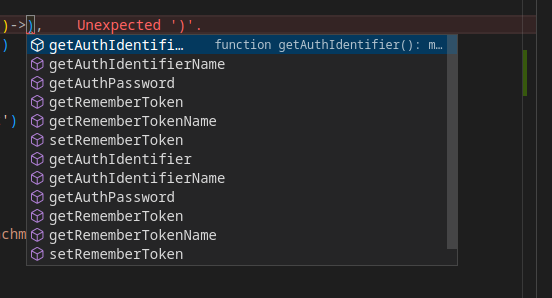
try and check on the browser
->disabled(!auth()->user()->hasRole('super-admin'))
hasRoles doesnt exist for me:/
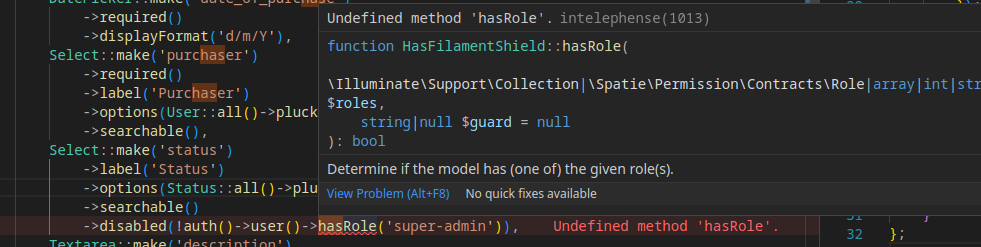
Does it for you?
should work. Any laravel error on the browser?
nope, no errors whatsoever
It just doesnt do anything. Its locked for all users
But the hasRoles doesnt exist for some reason
intelephense issue, maybe
can/cannot to permissions. Sorry, my bad
But check the spatie setup because hasRole should work: https://spatie.be/docs/laravel-permission/v5/basic-usage/role-permissions#content-checking-roles
You can also check by id: ->hasRole(1)
Using Permissions via Roles | laravel-permission
laravel-permission
the hasRole should work in the form in the resource file?
Maybe @Dan Harrin knows?
Yes, hasRole should work anywhere. It’s not a form thing. Are you sure you have a super-admin role set up? It’s not by default. Is that the name? It is case sensitive.
The fact that you’re not getting errors and that it is disabling means that it’s a role/permission configuration problem.
How can I make sure that I do have super-admin?\
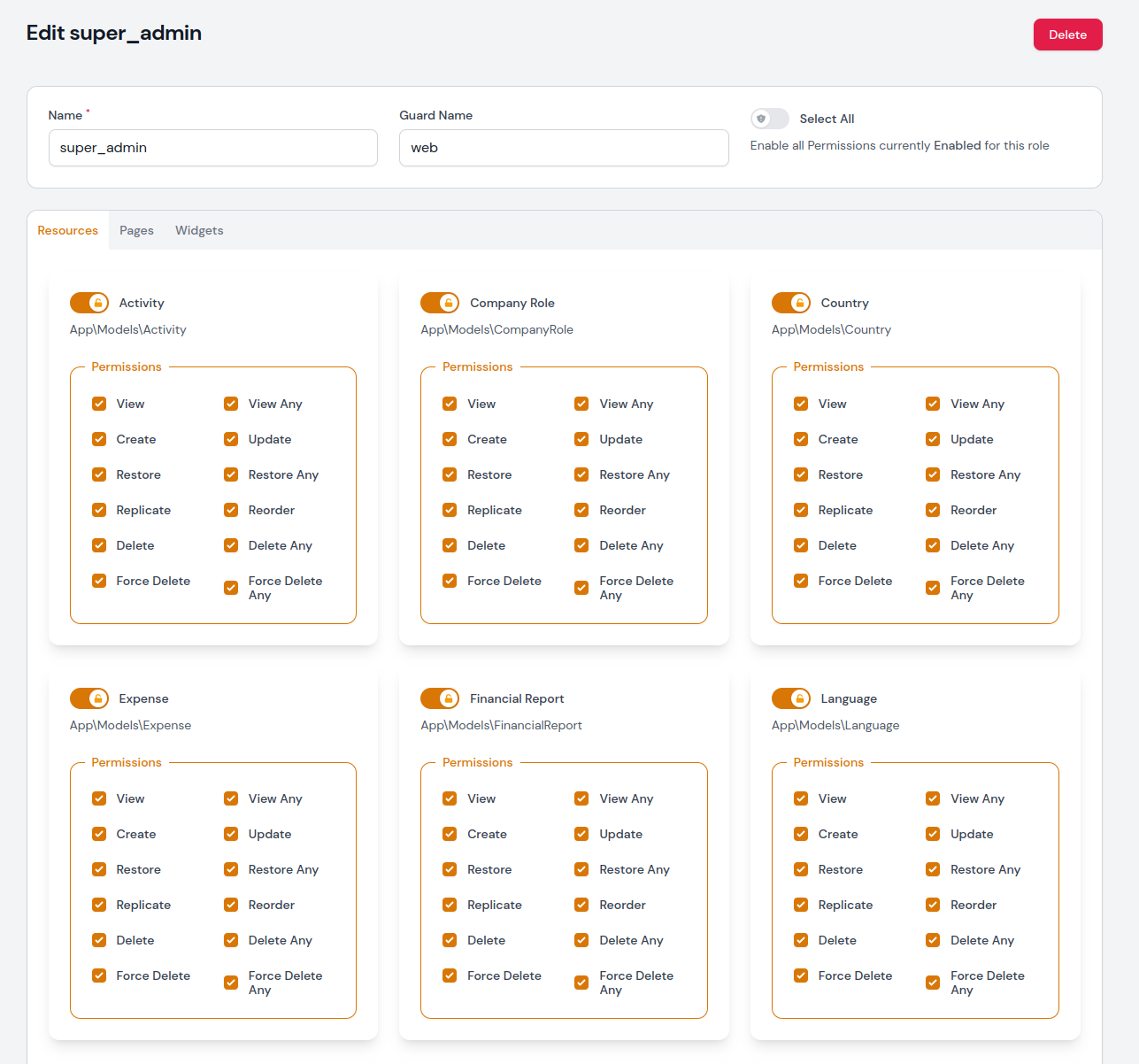
I have this
I tried this command in my previous project as well, and it also doesnt work
It seems like you do BUT your super admin name is with an underscore, not hyphen. Try that
hasRole('super_admin')
And then of course make sure whatever user you are logged in as is actually assigned the super admin role.
nice! That works! Even though it still shows up as an error
Hello, how to disable a field in a form while another field is empty/null?
@thethunderturner you might need to reindex the LSP
What I dont like, is that its hard coded. Is there a way to specify here that this user cannot change the status of the Select in the Expense model?
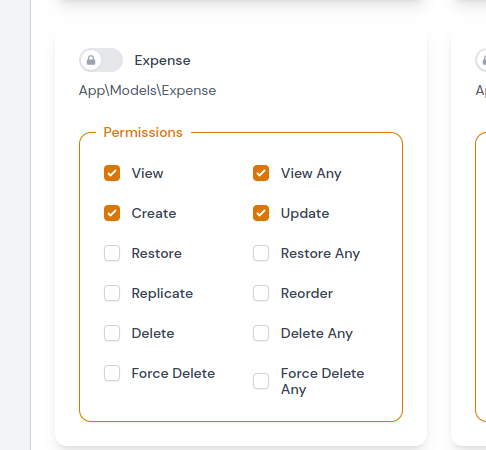
What is the LSP?
also you don't need both HasRoles and HasFilamentShield on your User model.
HasFilamentShield
includes the HasRoles
trait.I was thinking maybe tweaking the policies. but that hasnt done anything so far
LSP is the language service provider. In you case it's Intelephense. Bring up the command pallet and search for the command in the picture. That should help.

yep the error disappeared now haha
You would need a custom permission to achieve that and still check it on the field. That's not really a Policy level autorization.
Im honestly quite confused 😦
How would I create a custom permission just for the model Expense?
The resource should have HasShieldPermissions
I can create a custom permission, but how do I assign it to a function, to... do something
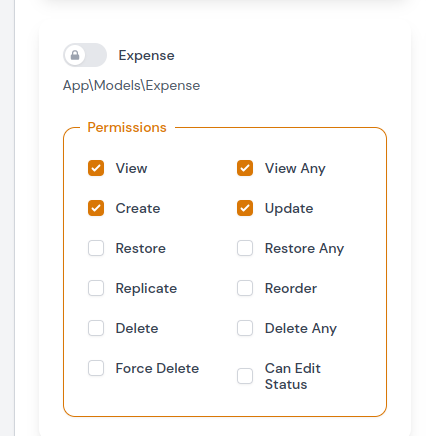
yeah. ive already read it, but it seems im at a roadblock
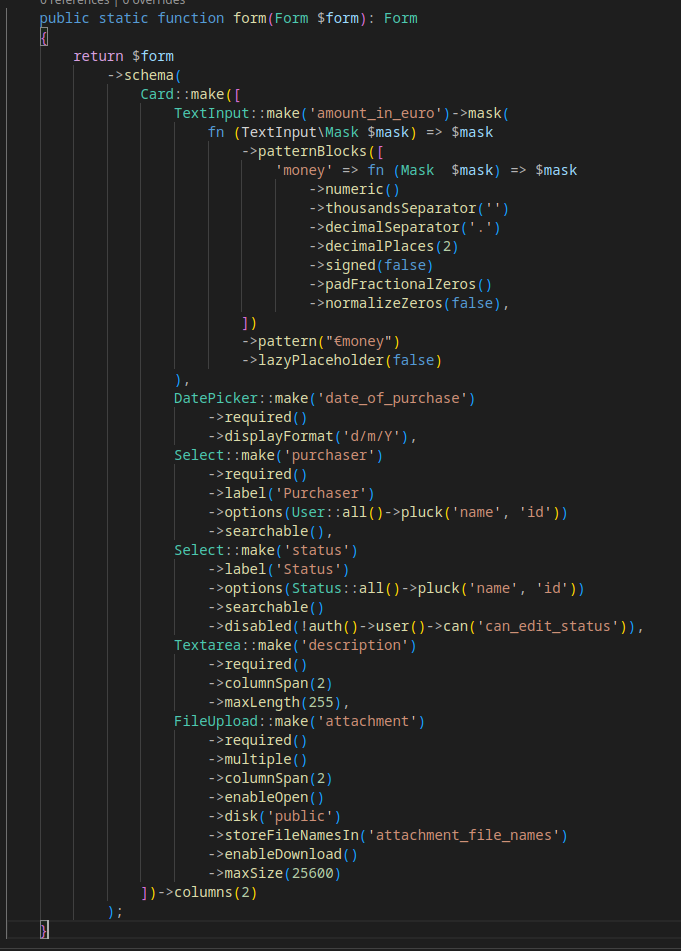
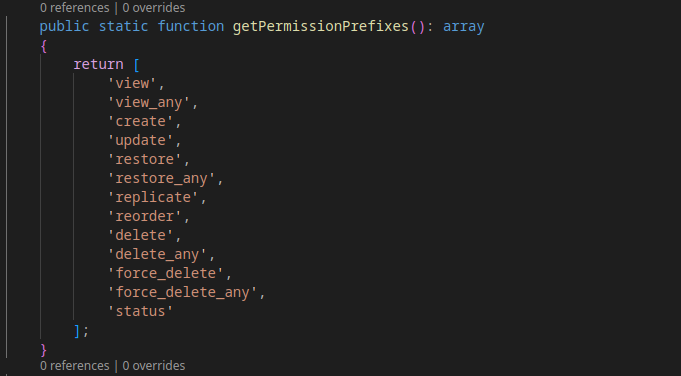
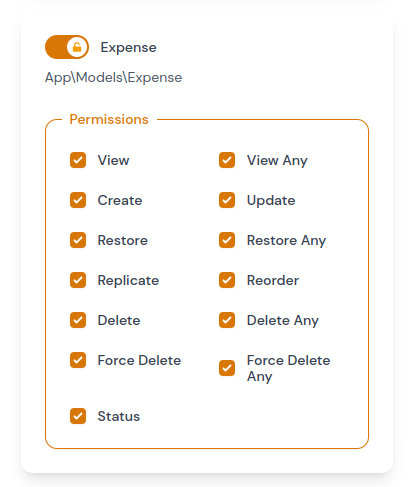
But I cant edit the dropdown
Hope you have some clue as to what I should do
Your permission is the wrong name. Based on how you se it up it would be
->can(‘status_expense’)
oh my god...
It works!
Thanks a million! :)