Array to string conversion:ErrorException when trying to store multiple files with FileUpload
23 Replies
This is the table:
Also, there is no array option in the table, so I was even more confused
You need to cast attachements to array in the model.
You mean, in the model?
That's what I said 🙂
oh oops 😂
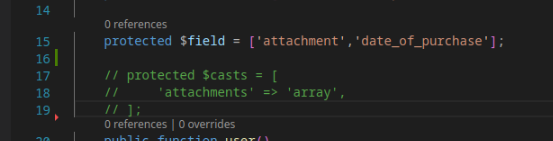
I thought i did, but it was commented
😉 that'll be why it's coming into an array / string mix match
I still get the error 😅
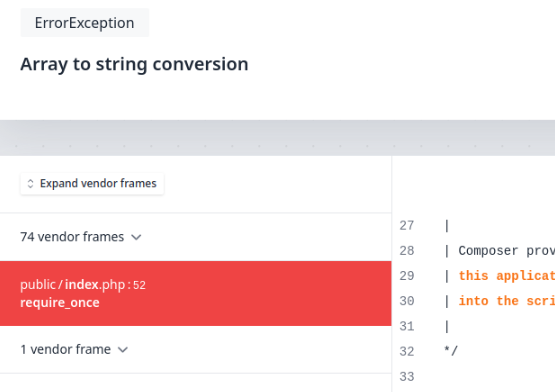
Is this on edit?
on create
What happens when you create a new one
I changed the attachment from a string, to a json
COuld that be the issue?
Possibly, you should nave a designated design path. So if you are using an select/multi-select you store in array usually. if you are using json then you need to cast to json, but I don't belive the editor natively supports date from a json dataset which would be why you are getting an array to string conversion
I changed to string again, and I still get the error
I get the error
Ok no so you want Json, sorry I've jsut read the docs for multi-select on file upload
and I need to case to json as well?
Yep, I also read it but I just thought it was the wrong choice in this case since I get an error
OHH
wait
There is also an issue with the file names. Since you have multiple files, you also store multiple file names
Ok cool, but you shouldn't get string to array issue on that. You store as json as it's passed as json. There isn't a castor for JSON last time I checked
I still get the error.
Hmm
What are my next moves
please dd() the $data in mutateFormDataBeforeCreate
I still get the same error
and nothing in the terminal
Done!
I fixed it
All i had to do is make a cast for attachment_file_names as well
yeah I missed storeFileNamesIn, that's good
APP_URL