Create GIN index in Postgres
I need to create this index in postgres:
and this is the code I have:
Reading the docs on GitHub I was able to create "normal" index, but it's not clear how to specify index configuration options (like the type of index etc).
7 Replies
what I tried (not working):
Maybe it's something related with this limitation?
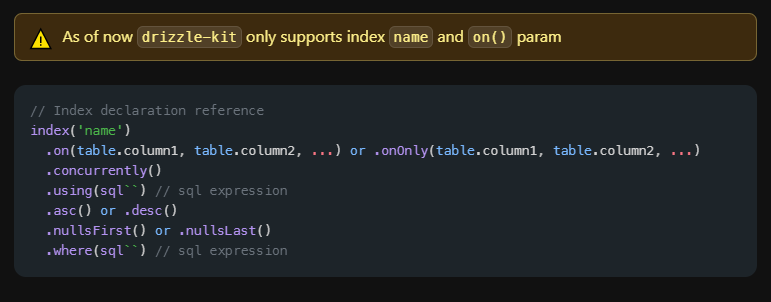
Did you figure anything out for this?
Did you just manually add the index line to the migration .sql file?
@pdina
anyone else figured it out?
GitHub
Add Full-Text Search capability · Issue #247 · drizzle-team/drizzle...
This is a feature request for Full-Text Search functionality to perform a fulltext search on specific fields. Ideally with support for using GIN/GIST indexes to speed up full text search.
does anyone have idea how to escape those
" ... "
around the column definiton for ts_vector
so it's a valid SQL
?I wrote a little script to remove the quotes and add a GIN index