Syntax Overview
Someone was asking for an overview of available syntax. I ended up coming this and figured it would be useful to post it here.
It doesn't include everything (notably most tuple expressions and scopes), but it's a good place to start:
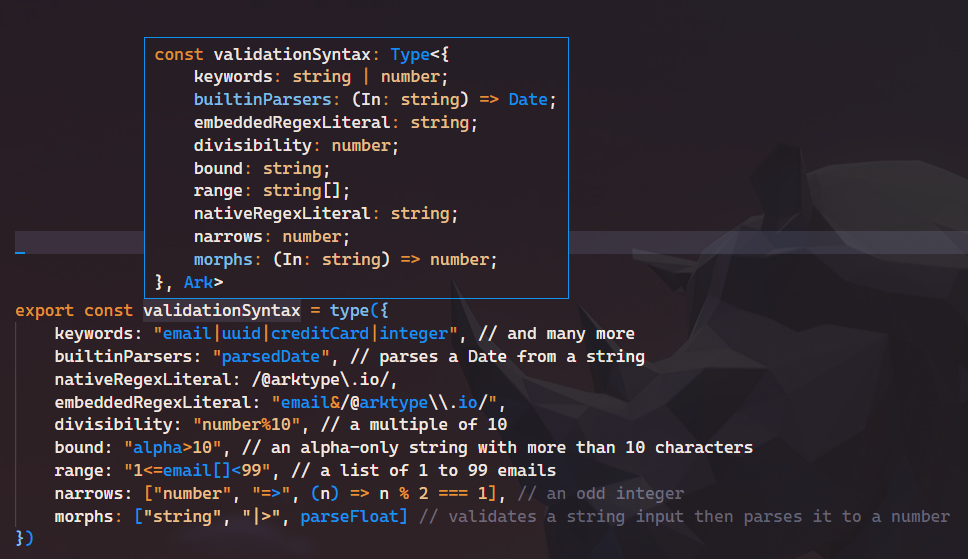
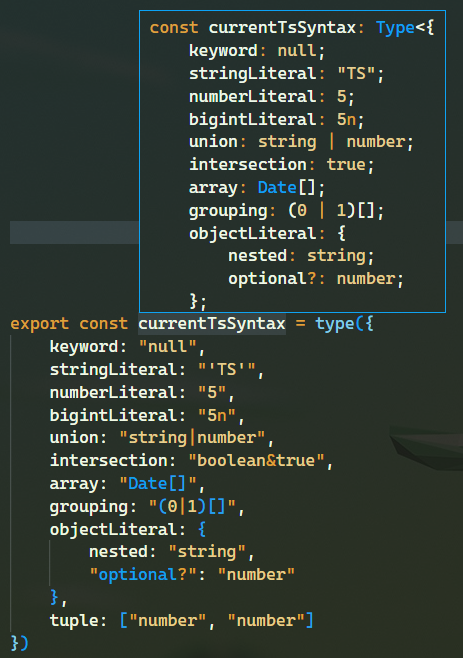
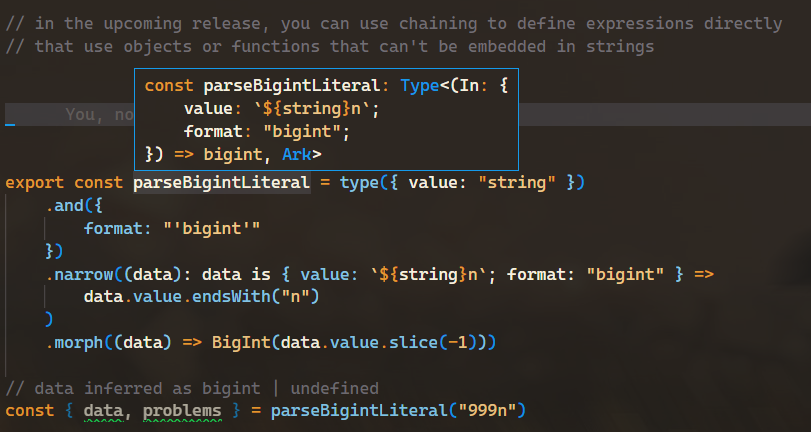
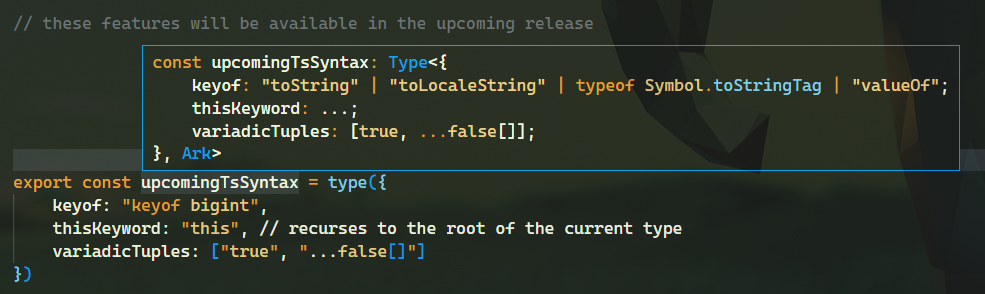
12 Replies
I'm now asking for overview of unawailable syntax
(i'm too sleepy to lol, so) huh
Sorry can't answer that without this https://github.com/arktypeio/arktype/issues/815
GitHub
Negated types · Issue #815 · arktypeio/arktype
This would allow some or all types to be negated (likely using a new ! operator). The challenge here would be integrating these checks with the type system so that intersections can still be reduce...
I mean more like not-in-0.14 / not-inplemented / not-planned
My response was a joke but the best thing to do is look at the unit tests. The organization isn't perfect but it's good. Anything that's commented out don't count on everything else is planned
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
@jakub yes (why didn't you just try it)
Unknown User•12mo ago
Message Not Public
Sign In & Join Server To View
(Recently someone asked (another) question with the why didn't you try reason being afk)
Yes you can include arbitrary spaces between operators/operands
Unknown User•8mo ago
Message Not Public
Sign In & Join Server To View
Yeah you can do
["instanceof", File]
Or instanceOf(File)
with the helper methodUnknown User•8mo ago
Message Not Public
Sign In & Join Server To View