How do i properly set up nextjs external pages/api/* routes using trpc?
I have a working application, now for testing I want to create a few pages/api/* routes to see the raw data i get before pushing into a component.
I created pages/api/lessons with this content:
and I am getting an error:
I have trpc working on the index page (basic t3 app) but when i try to place it inside an nextjs route it never renders. If i remove trpc logic from the api route and just put some dummy data, it works fine. Where is my issue?
13 Replies
Server Side Calls | tRPC
You may need to call your procedure(s) directly from the same server they're hosted in, router.createCaller() can be used to achieve this.
Christopher Ehrlich
YouTube
Advanced tRPC - Callers, functions, and gSSP
🚨 createSSGHelpers has been renamed to createServerSideHelpers 🚨
Repo for this video: https://github.com/c-ehrlich/you-dont-need-callers
If you want to use schema in the frontend, they cannot be imported from the same file as things that run specifically in the backend. One solution would be to put them into a
procedureName.schema.ts
or simi...you really dont want to be calling your own backend from your backend
just extract what you need into a function and call it where needed
i am struggling to grasp this. That video didnt show how to use it in an api route. Could you elaborate a little more?
I also want the api route to be protected by clerk auth so that you can only get a response if you are authed, but I can work on that after maybe. I am using t3 turbo with clerk so I may have to account for this to make this work. I am currently getting a type error with a reference to clerk auth (see errors below)
here is my current file
I am getting type errors:
const caller = ***
post_title: data.post_title I know post_title does exist on that type because its working on pages/index
@whatplan could you take a look? I have tried about 10 different ways today and i still cant figure it out
Just got off work I will tonight
Thank you!
ok so last night I didnt really read your question too deeply (sorry)
so first: if you want to check the output of your api just use it in a component and JSON.stringify or console.log it
second, as I mentioned earlier and one of the main points of the video is almost always
createCaller
is not needed
what you want is to extract your backend logic into a function you can call in different places
(image is from the trpc docs link)
so in this case you have a function that does all the database calling logic and then you can call that from different places
in your case you could call it from within a trpc procedure, or from a nextjs api route
but tbh I dont understand exactly what your looking to do
if you could explain more I can help further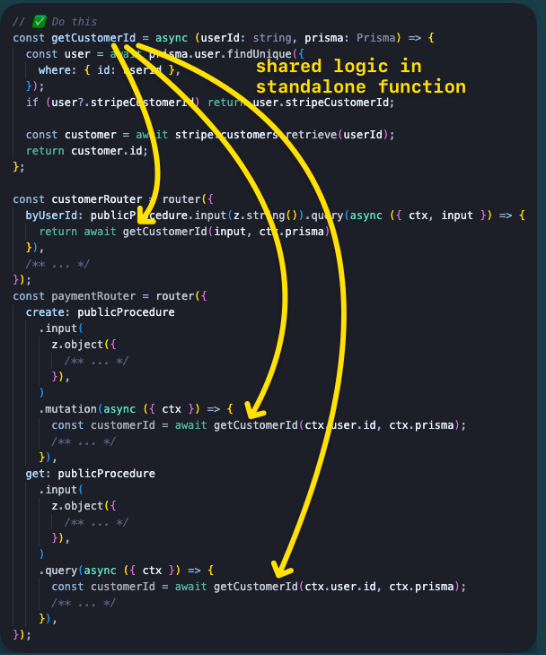
@Trader Launchpad look at this https://create.t3.gg/en/usage/trpc#expose-a-single-procedure-externally
"almost always createCaller is not needed
what you want is to extract your backend logic into a function you can call in different places" how would i handle protected routes in cases like this? Wouldnt i have to duplicate the code unnecessarily to check for clerk auth within the api route? I got it mostly working using createCaller, data is atleast printing to console, just having an issue displaying the json
thank you for all your help!
This helped alot, thank you!
Didn’t know this was a page on the docs site would’ve sent earlier sorry
And i think external api doesn’t work on protected routes. i have clerk user event webhook set up and it only works on public procedure
Yea if you make a request to a procedure that needs a session cookie, and you’re not providing a session cookie, it’s not gonna work
lucky for the webhook secret key