Is it possible to update state of a single row in the records table?
I have the following toggle button on a table, and update Eloquent model based on the $state. The problem I am facing is that all of Toggle buttons on the table re-render right now and create some unpleasant experience. How can I limit this to only a single row?
30 Replies
By the way, I am noticing this side-effect only on a RecordsList without any edit page. Could this be related somehow?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
The problem I am facing is that all of Toggle buttons on the table re-render right now and create some unpleasant experienceAre you on the latest version? What does that look like? The one you changed should be disabled until there is a response from Livewire
Yeh I am on the latest version. I have two identical tables with the same code for toggle but one refreshes the whole table on toggle. The only difference is that I have just a single table page that has problems. 🙂
Here is what I got on the resource. 🙂
and here is the ListRecord. I have computed Eloquent model here, that I am update'ing. Could this be an issue?
On the other table I am updating a given $record.
Do you have a unique key on the table?
How do I set one?
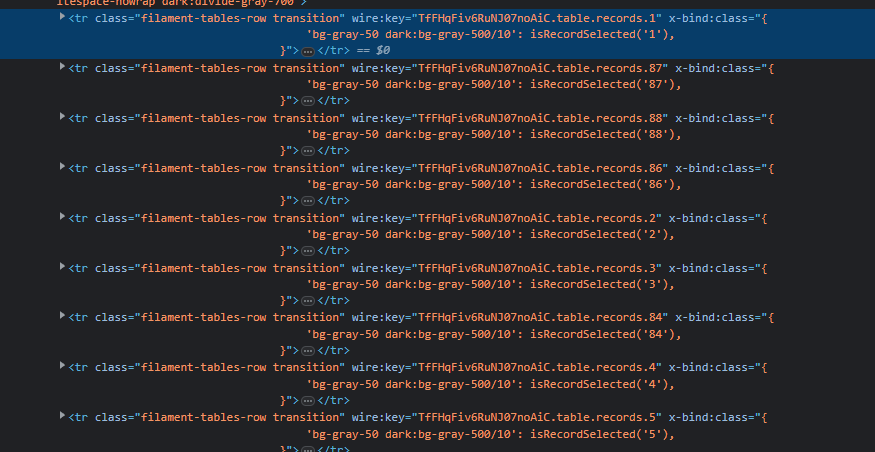
I see there is wire:key set but all of them are regenerated once I toggle
That seems fine 🤔
This is the table with keys where a single row is updated only.
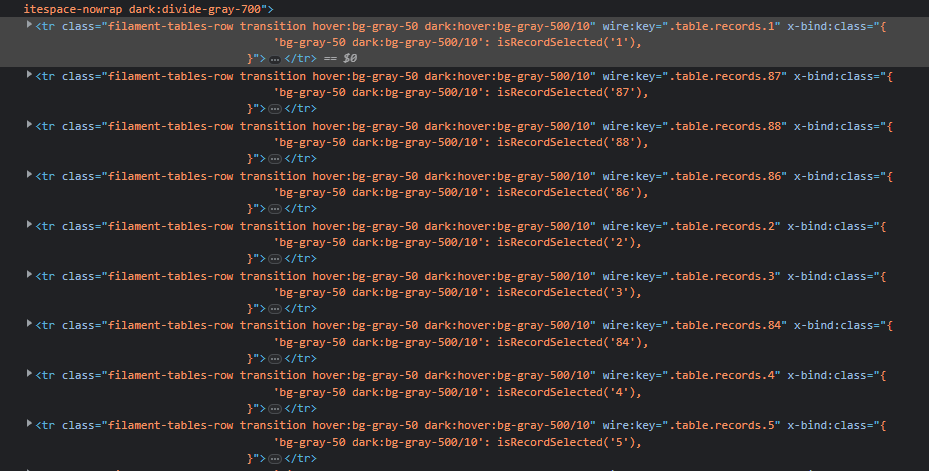
There is no this random prefix on a key though.
Check the toggle itself. Does it have a unique key?
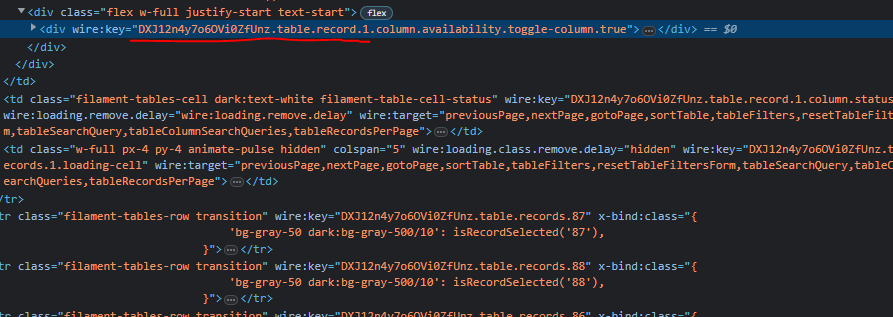
yep
Actually this should even be managed on the individual Alpine data. Can you show a video of the behaviour?
Just checking we're talking about the same thing
I am showing both - the table that updates fully for some reason and the working one. 🙂
Okay, that's something different than I thought 😅
Currently it's not updating the selected record at all. Maybe that's your issue?
I am messing up with some other parts now so that's why but when it did the same effect too.
I wonder what might cause the full refresh, and how can I control this.
Could it be the computed property where I just get Eloquent model out of the ID might be a problem?
I wonder what might cause the full refresh, and how can I control this.It's Livewire. It's always a full refresh with Livewire
On form we have something called afterStateUpdate or so, where you can then also tell what has changed but nothing on the table.
I don't really understand what you are doing with your
mount()
I have this dropdown, that just sets queryString, and then I filter by the ID in my table query to show relevant results for the selection.
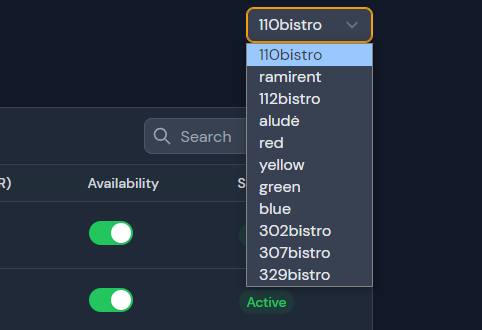
but these do not change during the toggle
Can I see the query/filter?
so through the action I set the podId, that later is computed to eloquent model when needed.
I am not a fan of moving Eloquent model through livewire state. 😄
Any ideas @Dennis Koch, maybe you would put me on track where to start debugging to find cause. 🙂
Hm, I find that when I define what to select from a multi-join query then I see a full table refresh.
Not really. I am sorry.
@Dennis Koch I found out this parent::__ in the ListRecord was causing a full page refresh. Do you know a better method maybe on how to extended a queryString?
Not really. Is there a difference if you move the call to the bottom?
Nah, if parent::__construct(); is added anywhere it results into a full refresh.
In the end, I do not need this contructor except I want to augment queryString when extending from ListRecords
I mean I want to add an additional item to this.
I thought constructor was a proper way but not sure now. 🙂