❔ Any reasons to use System.Memory<T> instead Array<T>
Just read docs about System.Memory<T>, tried it... I there any use cases where array can't do same work?
42 Replies
Also. Is there any way to get memory from MemoryPool.Shared.Rent of exact same size as minimal size in method parameters?
Memory<T>, similarly to Span<T>, is just a view of memory, it's not an array in itself.
Anyway, is there any use cases showing difference between their usage?
The same idea is applied to array. I can use AsSpan() and modify array's data, or send it somewhere if there's no async/await/threading
but with
Memory<T>
u can do it, as its just a struct
and not a ref struct
like Span<T>
Same for array, except that it's class
also u can throw a
Memory<T>
on native allocated memory (eg. from P/Invokes) without copying anything. with an array u would have to make a copyWell, this is useful case
which means a heap allocation for sure, and if u just want to provide access to the first X elements with an array u would have to create a new array, so new allocations, more stuff for the GC to care about and u have to copy data.
not to mention the
ReadOnly
variants ;pOk. I got the idea
And what about memory size from MemoryPool.Shared.Rent?
if its too big for what ya want, just slice it shorter ;p
eg
Thanks a lot
didnt use the memory pool yet, but i guess its similar to the array pool stuff
There's no way to slice memoryPool owner... And no Return of rented, only Dispose 🥲
Ok. but I understood all
yeah it's slightly different than how they showed
well, if i need to use a pool, i usually just use an array pool.
MemoryPool returns a IMemoryOwner
not an array/Memory directly
good to know, i should probably rewrite some of my stuff ;p
ArrayPool just returns a normal array though
yeah, i assume the memory pool variant is better cuz less instancing
oh, btw a real life example would probably be text parsing and alike
Why Pools designed to return a bit more... awkward behaviour that forces to save size
Already using it while learning OpenGL
well, for array pools its probably, if they have a "free" 40 sized array and u request a 32 sized array, they wouldnt need to allocate one
If memory is just start and size... They could just give slice of free memory
maybe its because of memory alignment
(i dunno too much about it, but in the end there must be a reason for it)
especially if it can be moved by GC that size offset could be also moved and used as free in pool...
Will try to find something about it
maybe its also, that they simply work with "constant" chunk sizes and simply get x chunks back, so they dont have to deal with too much fragmentation
Magic 4095 🙂
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
It was Unsafe.SizeOf<T> before
That this 4095 not commented any way or not set to variable/const with human-written name )
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
That makes sense. But it works only minSize set to -1
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Yeah, also found code
and here i am using mainly
stackalloc
;pI think i'll just use array 🤣
and somehow convert it to memory
new double[1024].AsMemory()
and u r done ;por simply use https://learn.microsoft.com/en-us/dotnet/communitytoolkit/high-performance/memoryowner
MemoryOwner - .NET Community Toolkit
A buffer type implementing
IMemoryOwner
that rents memory from a shared poolWow, will try
All I needed 🤣
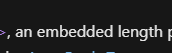
Thank you very much ✌️
dont forget to $close the thread if ur questions are answered
Use the
/close
command to mark a forum thread as answeredWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.