❔ making something wait in unity script
trying to make something wait 0.2 seconds but i just dont know how
51 Replies
tried StartCoroutine but i get
Argument 1: cannot convert from 'method group' to 'string'
don't really know unity script. In standard c# you could have something like Task.Delay()
https://gamedevbeginner.com/how-to-delay-a-function-in-unity/
does this article help any?
John French
Game Dev Beginner
How to delay a function in Unity - Game Dev Beginner
Learn how to delay a function, pause logic and trigger code at fixed intervals in Unity.
You can also do this in Unity
Unity supports async-await fine
Please share the script. Looks like your syntax is not what it's supposed to be
Or, you know, look up how Coroutines work since there's plenty of documentation
The unity script document didnt work
Share the script
We don't know what you did
StartCoroutine(colorChangeDelay);
is not how you call a Coroutineis it not?
how do you call it?
You need to invoke the method and internally
IEnumerator
-returning methods will invoke your code until a Yield instruction is met. In your case that's new WaitForSeconds(0.2f);
Unity handles these when you use StartCoroutine
So if you change that to StartCoroutine(colorChangeDelay());
it worksso what should i do
oh
Alternative:
why is unity docs so overly complex
well do you know why the color isnt changing?
its static red for some reason
They basically did the same thing as me
You were probably confused by the extra parameter
The docs are kinda shit here, yeah
i cant even do that
i cant do coroutine = WaitAndPrint
or in my case colorChangeDelay =
It's WaitAndPrint()
WaitAndPrint
equals a delegatewdym
You would be saving a reference to the method
Called a delegate
well do you know why the color isnt changing?
its static on red
Because you probably misunderstand the point of Coroutines
im using them for delay
Do you expect this to delay the length that
colorChangeDelay
is waiting for?no i just want it to wait 0.2 seconds every times it runs through so its not too fast
A Coroutine sort of runs in the background, so there is no context that simulates a delay here
ah
It's hard to explain, but your Coroutine does have a delay
This code in
Update
simply does not have thatim confused now
What you can do, it put this code in a seperate Coroutine
Give me a second
Something like this
Notice you can use the delay routine in another Coroutine to simulate the delay
You just can't do it where you did. These are methods that run on the main thread and they should never have a delay (because if they do, your program locks up), because this main thread does everything in your program (like rendering the screen).
wut
Either way, try this
im beyond confused
Your old code would run the code every single frame because it's in
Update
, and the delay didn't work either
Unity is not going to wait for it to runline 1: 7 errors
6
You should put a Debug.Log message in the code to see when it runs, then you can see what it does.
Like:
Debug.Log("I finished running");
at the end of the method, below the StartCoroutine()
What is the error?
just put in line 1
Can you screenshot your editor?
wdym
Screenshot your whole editor
I want to see how it looks like
the code?
Yes
You probably did not configure it properly
do you mean like this or something?
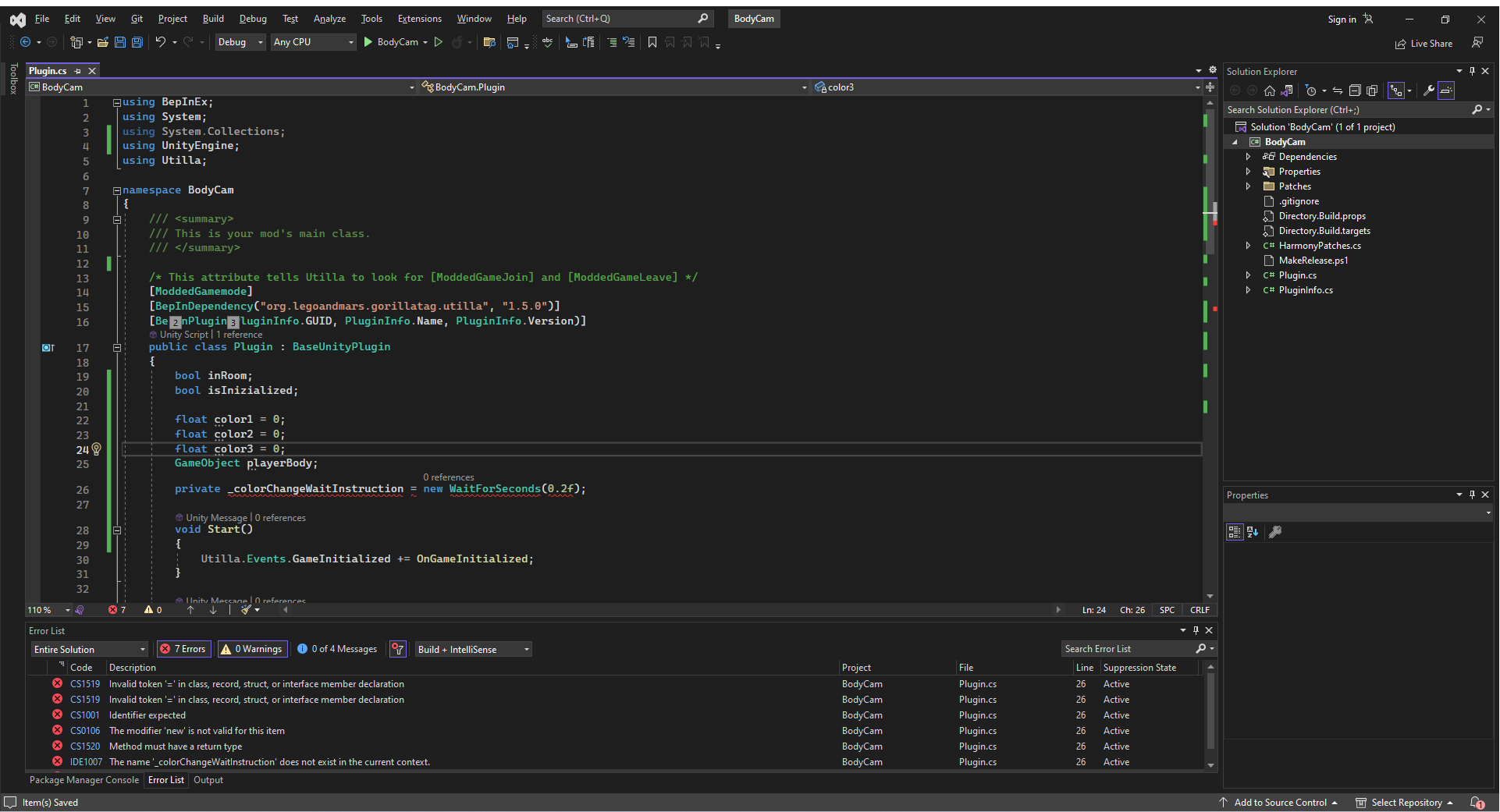
Small mistake in the code, change it to
private WaitForSeconds _colorChangeWaitInstruction = new WaitForSeconds(0.2f);
You also need to change yield return this._colorChangeWaitInstruction();
to yield return this._colorChangeWaitInstruction;
its still just static red
Then that has to do with your code
Try the Debug.Log and see if it delays properly
And you can also put your color values in it to see what it becomes
It's most likely not what you expect it to be
its stuck at 283.3333, 0, 0
which i dont know why
i know why
wait actually i dont know why
no idea
color1 reaches 255 and goes to 283.3333 and stays there
it for some reason doesnt stop at 255
well it changed colors now
it went from red->yellow->white->stuck
which is still not correct
Hmmmm
Well it increments one more time, so I'm guessing your if-statement is wrong
Perhaps your color is not exactly 255, and it's just below it, causing it to increment once more?
You're comparing floating points, which can be inaccurate
You can always use a
Clamp
on the colors to clamp them between 0-255Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.