❔ Multithreading an async function
I have an async function that I want to multithread with
n
number of threads, but I get convert error if I initialize the thread with new Thread(methodName)
From my understanding, I need to use the .Wait()
method every time I call the request
method, because it has a Task
object.
The method request()
:
73 Replies
This is the error I get from initializing thread with the method

If I change the return type from
Task
to void
, then I can initialize it, but the method doesn't complete at the await
keydon't manually create new threads, use the threadpool with Task.Run or the Parallel class
dont create a new httpclient on each request, you should only use one across your application
but you shouldn't run this method on a new thread, because it isn't cpu bound work
you could do something like this:
But this isn't making threads
Doesn't
WhenAll
wait until all the code is executed, but doesn't it do it synchronously, rather than creating multiple threadsWhy do you want to create multiple threads?
If you want to run the requests in parallel, then this works
An if you really want multiple threads then just use the methods i mentioned before and NOT new Thread()
your
request
method should work just fine in a single thread
its just waiting for IO
thats literally the ideal usecase for async
:pI'm trying to send multiple http requests at once, and some requests would get stuck in a timeout, so I think using multiple thread is necessary
unless I'm missing something
the new HttpClient() in each request 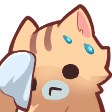
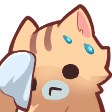
absolutely not.
I'm setting a new proxy address for each request. dang it, i forgot that i can just override those address lol
you can make multiple requests at once without having multiple threads.
as long as you don't use any blocking code, you're fine
Thank! The Task.Run works perfectly fine with this. but for educational purpose, is there a way to do it with multi threads?
Task.Run will use multiple threads.
Do you have an example?
don't use Task.Run for this!!!!
you don't need multiple threads to send the request at the same time
.
oh this is fascinating
I need to learn this separately
thank you guys
!close
Closed!
new problem
When I execute
Test()
, it seems like the code doesn't wait for the await part in the function
but if i replace await client.getasync(url)
to something that doesnt require async, the code runsif just call
Test();
instead of await Test();
its basically fire and forgetawait Task.WhenAll doesn't seem correct there
it should be outside the lambda
instead of thinking about multi threading u should first think about how async works
🤦 totally forgot
also u should not use
async void
but async Task
just for my understanding, doesn't your solution also use multiple threads?
this one
no
only one thread there.
arent the tasks executed on the thread pool and thus its simply not defined on which threads its running?
I highly recommend reading $nothread
There Is No Thread
This is an essential truth of async in its purest form: There is no thread.
the tl;dr is that no, not every task/await results in work being done on the threadpool
basically each task gets scheduled, runs on a non busy thread til its execution is paused, once it can resume it will continue execution on one of the threads again?
eg, the requests will be built on one or more threads of the thread pool, etc. then the task execution will be suspended until the request is sent and response comes in, then the task execution continues on a non busy thread again
With this code, how would I add dynamic handler (Proxy address changes per request) to HttpClient?
I don't see a way to add different handler values unless I initialize
HttpClient
in the method, because you can't add handlers after HttpClient is initializedUse IHttpClientFactory, imho
Great article, thx
well, this works fine.
granted its only three urls and all three of those are usually quick to respond, but I'm 100% sure it started all three before the first one replied.
what makes you think this?
yeah the whole "proxy addr changes per request" thing has me thrown off
I've never ever had to do anything like that before.
what repo?
its @Knuceles thread
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
yeah actually, in all the samples they shown so far the URL seemed to be the same
only the proxy changed.. so its hittiing the same url with multiple proxies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Agreed
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
What’s this about?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
What repository?
The code related to proxies?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Which thread
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
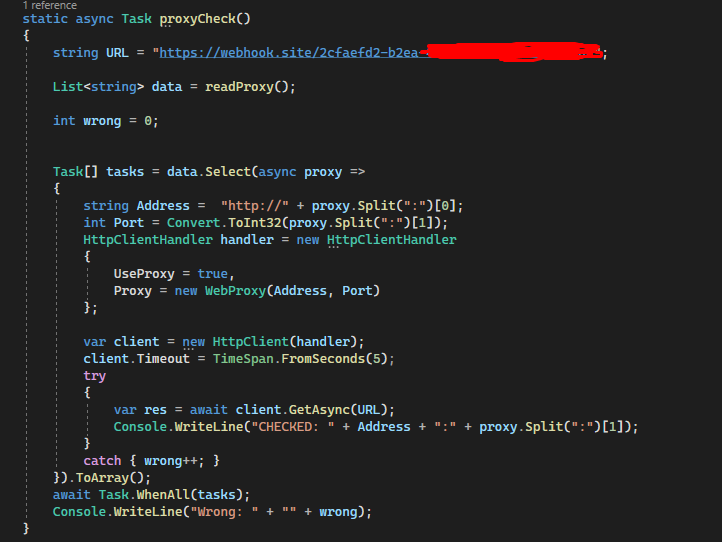
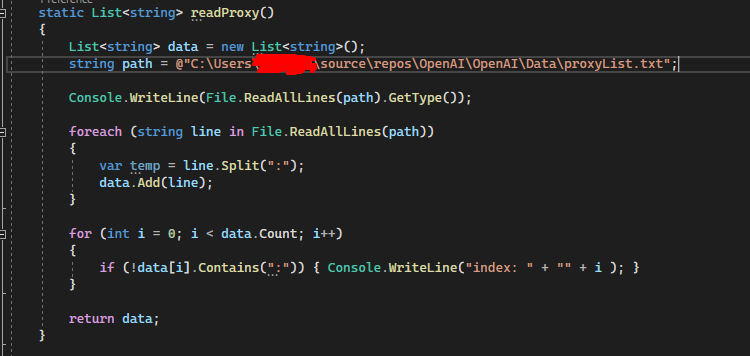
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
This is the thread related code
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
I'm just making a proxy checker
no i dont know what that means
Regarding the google analytics cookies, i know that those cookies are generated from client-side javascript file, so I wanted to see how I can retrieve them with C#
the intent is to see how I can get client-side js generated cookies
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Wdym?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Yes, but there must be some way for me to mimic the browser behavior in C#, right?
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Obtaining the cookie somehow
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
seems more like they want to bypass the openai rate limits, im out here
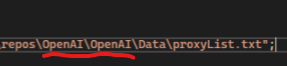
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
Too meticulous
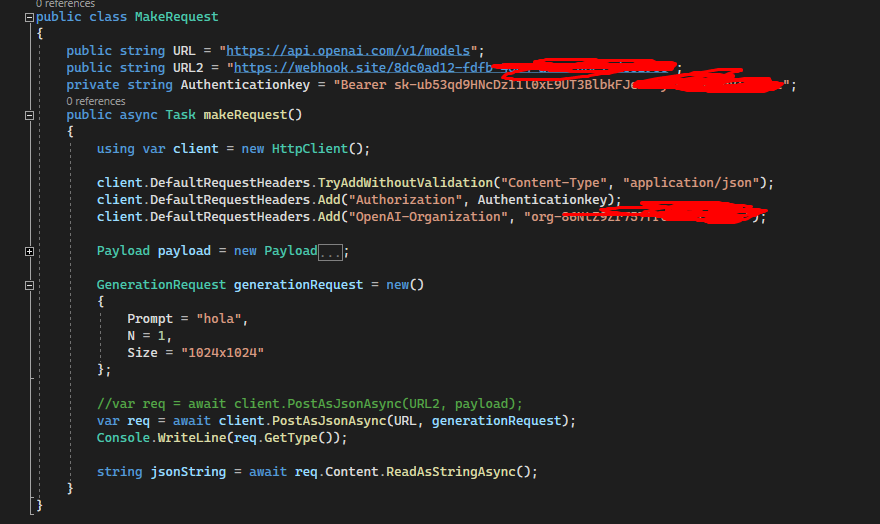
I'm just playing around with the OpenAI API
if it's not too obvious, im clearly new to C#
I created bunch of C# projects, and it's becoming too hard to manage, so I'm just putting everything in this one project
Unknown User•2y ago
Message Not Public
Sign In & Join Server To View
which includes this proxy project
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.