ā Struct vs Class
When do i use a struct vs a class, There seems to be pretty much no difference between the two except for really small things, what i want is composition, so idk if that helps. i think a struct is waht i should use for that right?
71 Replies
reference vs value type is a big difference
why
is it really that big of a deal
having a reference to something vs the thing itself changes a lot of things
like
i dont rly understand what it would change
like how assignment works
when you pass a class instance to a method, your actually passing a reference to the actual instance
well i know that
so if you change it it changes everywhere
yes
and i imagine that doesnt happenw ith a struct then, but even then that doesnt sound like a big deal
structs on the other hand are copied
it is
structs have some other limitations too
as a general rule of thumb, default to classes
so
https://paste.ofcode.org/37PNwYAx6seZZWQt6MVij65 is this the way to do it? or should i do it a diff way
oh
ok i guess ill use a class then
Unity's
Vector2
and Vector3
are structs, because they essentially act as numbers. You wouldn't want to be able to change the x component of a vector and have that be reflected everywhere else that you've passed that vector to. Imagine for instance that you set an object's position to a vector, then you also pass that same vector to a method which saves it somewhere else. Now if something completely unrelated comes along and changes that vector, you really wouldn't want that to also change the position of the object.oh ye i should use a class because i want to be able to change this variable
unless what you're writing represents a single logical value (and its size is relatively small), e.g. a date, a vector, etc
ah ye
so this is wrongly used
yes
What if you did
Choosing Between Class and Struct - Framework Design Guidelines
Learn how to decide whether to design a type as a class, or to design a type as a struct. Understand how reference types and value types differ in .NET.
i changed it to a class
now is there a way to do what i did smalelr
well
do what
that part
unrelated, but im not a fan of nesting types
wher am i nesting types
Movement is inside CharMovement
oh
which should probably be called CharacterController
well ye but otherwise i have to create a new script
and idk what to do with the script
that arleady exists
oh
well id think of a different name because this one is confusing
anyway
you don't
you can just create a normal file
in unity?
yes
wait what
š®
unity c# is still c#
just out of date
and the unity api is weird
but other than that
well
if there are good alternatives for unity i could use that but the others seem way harder and more annoying
like ofc there is unreal but no way that im gonna code in C++
bruh the C# naming conventions suck, public fields have the same conventions as public classes, im just gonna get duplicates now
you can used to it
unreal c++ is a lot different from regular c++ from what ive heard
but im not telling you not to use unity
unity's fine
just not having garbage collections scares me
you can name fields the same as classes
no you cant it doesnt work
but unity's naming convention is different
in regular apps we dont use public fields
wdym
you use all getters and setters?
unity names all fields in camelCase anyway
yes
properties
they dumb tho like if im gonan have a getter and a setter, then why do i not just make it public
tf
Cyberrex#8052
REPL Result: Success
Compile: 408.569ms | Execution: 39.195ms | React with ā to remove this embed.
you can compile code
see it works
in discord????
oh
its a bot
its nested š
what is
FOR GOOD REASONS THO
the class
because if at some point you decided to change the default getter and setter, you could
fair
but the main reason is to be consistent
its not nested
its defined below it
mine is nested though
oh i thought you were talking about the repl
Because you may want to have logic in the getter/setter
getter and setters are a lot easier in c#
and u r done with having a getter and a setter, u can also have getters and initializers only
{ get; init; }
, etcoohhhh
ohhhhhhh thats cooool
intializer?
just =?
But Unity doesn't let you use them 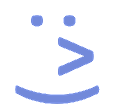
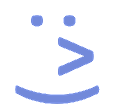
a setter that u can use only once
no init in unity? D:
oof
outdated language version
idk
my ide says i can do it
and its like synched with unity
but in the end if u would have to add functionality to the getter/setter it becomes a bit more bloaty, but still not as much as java:
but this way u dont have breaking changes in the API itself
also regarding nested classes: they work differently in c# than nested inner classes in java btw., as it wouldnt have automatically a reference to the outer instance.
lol
yo
question
how can i detect when the console app is minimized
open separate thread for that question. its totally unrelated to this
bruh does C# also not have enhanced enums?
nvm it does
idk what an enhanced enum is
to have it sorta like java u could use extension methods, but i think its sorta ugly
which u could then use via
MovementKind.Sprinting.GetMovementSpeed();
but something like this would be more c# like i would say:
If you didn't notice, this is a post for a completely different question. Please open a new post in #help.
Stuff like
init
is allowed but won't compile unless you add a sort of workaround, such as this:
Unity supports latest .NET Standard but stuff like init is not part of that, only .NET 6, 7 and 8Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.