How to reproduce a Prisma `include` statement for arrays of related entities without SQL?
Hello,
I'm trying to translate this SQL query to DrizzleORM;
My issue is that I don't know how to use / when to use the
array_agg
- do I have to use raw SQL ?
In Prisma, I could just do a findMany
for channels w/ include
the channel_performances.
In my case the objective is to retrieve the channels
with inside, the channel_performances
as array for each channels.
Do you know how to do that in Drizzle ORM ?
Thank you !14 Replies
You can use few approaches for that
1. Just use simple select with join and then aggregate results in your code. Great example with users+cities, that is actually the same you want
https://github.com/drizzle-team/drizzle-orm/blob/main/docs/joins.md#aggregating-results
GitHub
drizzle-orm/joins.md at main · drizzle-team/drizzle-orm
TypeScript ORM for SQL. Contribute to drizzle-team/drizzle-orm development by creating an account on GitHub.
Second approach would be to use json_agg
I'm afraid I can't find it so fast, but I believe @rphlmr has an example for you
And we are going to prepare first class support for json_agg. So you won't need to even write it. Syntax will be close as Prisma, but will be definitely in another layer on top of drizzle core. As a helper
@rphlmr Sorry to mention you twice 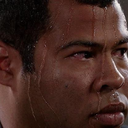
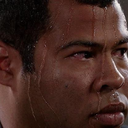
you're the best, thanks!
Thanks, I'll go with the first implementation for the sake of simplicity.
However, I got an issue in the code;
Where is defined
cityId
in the if
scoped statement ? My TS gives me an error of an undefined const.oh, maybe a typo
will update docs, thanks!
I guess you need to use
city.id
I figured it out ! :))
Thanks again @Andrii Sherman you're the G
do you have any suggestions on making this work with a nested relation?
Allowing for usage like this:
However, I cant really figure out how to stop typescript from complaining here and infer the correct type for
tasks
Some builtin feature to support such aggregation would be a great addition to the library, I really like what you guys built!
@Raphaël Moreau🧐 even if TypeScript complains, does it works?
I’ll try to see but right now I have no idea 😅
Yeah, it works
but i have no idea on how to make it work for typescript
I have to build a repro to test and debug
any chance you have something public I can pull ?
I know the issue but I don't know how to solve it.
jsonAggBuildObject
should take Record<string, AnyColumn>
or the return type of jsonBuildObject
as arg.
But jsonBuildObject
type relies on T and T can be of type ReturnType of jsonBuildObject
. So their is a loop for TS :/I'm very interested in this topic, I understand why Drizzle doesn't have this functionality but I'm really looking to replicate it as soon as possible (especially nested includes).
My use-case schema is this:
Basically, a whole bunch of nested stuff. Ideally, I could like this to come out to be
I was able to get it so that each menu item has a
modifierGroups
list, but I'm not sure where to go from there to get modifiers
in each modifierGroups
item.
Currently, I have
Which gives me
@Noahh My usual approach for these complicated things is to first write it out in raw SQL and then translate that to Drizzle. Its much easier to reason with
I think this would be best implemented by getting the
modifierGroups
in a subquery