❔ Weird Record compilation error: "must be a readable instance property or field"
I'd post it in the title, but it's too long 😭
Here's the records responsible for this error:
What's going on here? I have several other record types defined that have no issue having other records as positional parameters/properties. Example:
17 Replies
I'm gonna guess that inheritance isn't supported with constructor-definition syntax
Records - C# reference
Learn about the record type in C#
I'm not seeing any examples of inheritance used together with constructor-definition syntax
Here's the most confusing part: Renaming the property makes the error go away.
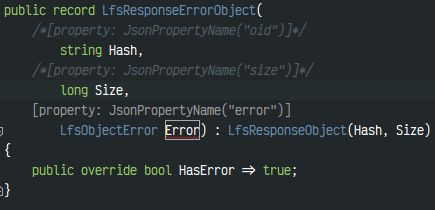
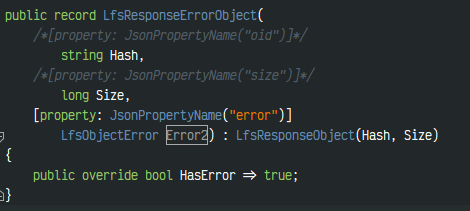
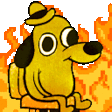
Are you sure the base clause is not binding to another definition of
LfsResponseObject
from somewhere which has an Error
property on it
fwiw, plugging your stuff into sharplab does not show me an error. https://sharplab.io/#v2:EYLgtghglgdgPgAQAwAIEEYB0A5ApgFwG4BYAKGTSwBVcAPfTAKQGcB7GTAZVwCcoIANlABeEfFHYlSZBAGYUEYM3w8IAY3woeuNax4ATFABkAZswBKuZgAd2zXAHlgAKx34AFGRTeUAbWs8rNa8+ACeICgs7AAKgcE8YdgQYLjuAESsUPppAJQAuihePsUYqAASEMwAFgA0Rd7+cSHhkWwwsUHNSSnpzCK4uQX1xSgC7ADmKJz9OVIy8tq6BsZmljZ2uACiPIE8Tq4anqQjPqUoFdV1xyejE1P9V8WNnQktUe1Nr92paby7g4VridTMx9m5trsUBC9DkUBEQWtbDB7GDDhdavdhLhZigAPS4vwAYU4AA4SQA2ckFSxLQwpMDAXgoADkDmCMAAYpV8AARIyYACC1igmCMHM4otWViRKJcbkw0J4zJQYAArsoUIyFFpcBB9IoBLgULBlBAYGojQEXmEUHoUCYoLgBIZWCYUGFgiy2bhOdy+YLhaLxZLQXKNIrlfhWCqxGoqihbH1xOxBAmIKoUvgmcyI5gyPMdbSVqGDvhFUcnlb4mEIu8OtXQt90rp9AN8oCbud8PhrJx8GJ1YTWK2UEPW48fM8G7W2vWuskfilmMwIOM23lhiV0KgALJWFdr2ZAA===I actually thought you were on to something for a moment. I'm actually writing my own wrapper for the git-lfs API, and I was previously using
Estranged.Lfs
which was an existing lib that partially wrapped it. It does not have an LfsResponseObject
type at all, and when I hover over it in Rider, it's showing me my own type and base type i'm deriving it from
But I think I just realized what it is
I defined some convenience methods on the LfsResponseObject
type, one of which is a static method called, you guessed it, Error
did not know that was gonna be an issueI feel like if we had used different symbol display options in the diagnostic, this would have been pretty easy to identify.
Does the error go away if you explicitly shadow this method (e.g. explicitly add an Error property to the type where the error is being reported.)
yep
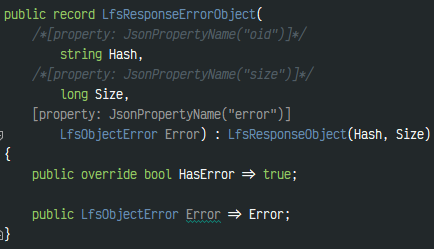
It's not an issue really, I'll just rename the helper methods to FromError instead of Error
glad to know what the cause was, finally. The whole "renaming fixes it" took awhile to click as to the issue
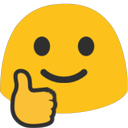
It would be handy if you could file a bug on us, asking us to use a symbol display format which makes it more clear what the offending member is. e.g. if it was
Record member 'LfsResponseObject.Error(string, HttpStatusCode)' must be a readable instance property or fieldI think it would be clear
Would an appropriate issue be a suggestion for a better error message for CS8866 specifically?
Yeah, just describe your scenario, if someone tries to fix it we can consider if any other related diagnostics should also be changed.
I wouldn't include the diagnostic ID in the issue title, just paraphrase the general diagnostic message, e.g. "'Record member does not match positional parameter' diagnostic message is unclear" or something like that.
I submitted a simplified version since the repro I provided seemed valid enough of a real use case (and my records in my actual project are a mess at the moment 😅 https://github.com/dotnet/roslyn/issues/67869
But thanks for the help!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.