❔ Why is there a green squiggly line under the first Console.ReadLine(); ?
namespace CSharpPractice // had to check no top-level statements
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
string message = Console.ReadLine(); // <= green squiggly, CS8600
Console.WriteLine($"Echo: {message}");
Console.ReadLine(); // require user to press Enter to close program
}
}
}
40 Replies
check the returntype on
Console.ReadLine()
its string?
, not string
It's basically warning you that
Console.ReadLine()
might return null
.oh so i have to put the ? after the type? that mean message is allowed to be null?
and putting a
null
value in a string
variable would be misleading
since you've said "this variable never contains null"either that, or you can write
Console.ReadLine() ?? ""
is there a difference if message were to be null vs ""
yes
"" is a string, null is null
ok got it.thank you everyone 🙂
null means that there's nothing there, while "" is a string which just happens to be empty
got it, i will close now
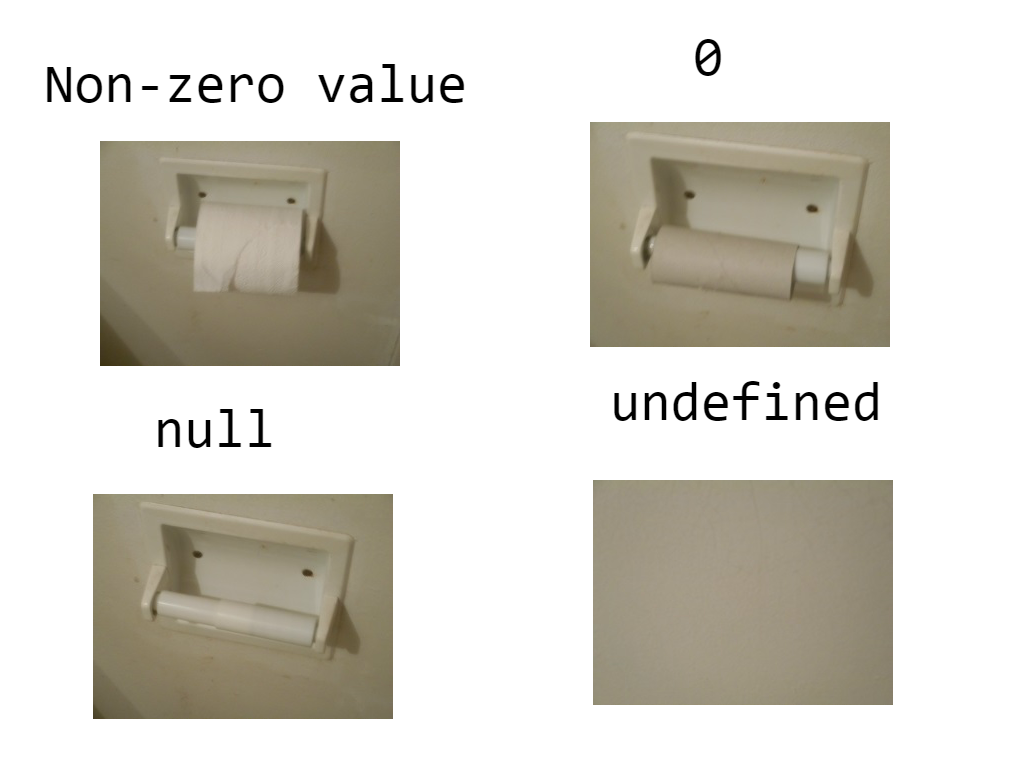
i still dont understand the null vs undefined based on that img
what is the difference in terms of memory?
null is absence of a value in memory, undefined means there's not even any memory address to look at
can also mean "uninitialized", at least that's where you would see that behaviour in C#
ok so with null there is still a memory location/address
yep
undefined is like trying to access a variable that does not exist anywhere in memory
yup
note that this does not compile
indeed
the compiler is smart enough to detect that
fairly trivial to detect
"trivial" 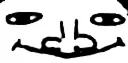
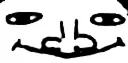
am i misunderstanding your code above?
string s;
is null by defaultit's not
at least local variables are not
If you do not assign a value to a variable then it's just uninitialized
i thought i read that declared variables are assigned their default values like 0, false, null. but now I'm reading that yeah its uninitialized
Well, local variables are uninitialized unless you give them a value. Fields are assigned their default value by default.
thinker227#5176
REPL Result: Success
Console Output
Compile: 596.746ms | Execution: 35.565ms | React with ❌ to remove this embed.
ahhh u cleared that up for me
i was so confused
so this code from ChaptGPt has an error:
string s;
if (s == null) {
Console.WriteLine("s is null");
} else {
Console.WriteLine("s is not null");
}
yes
because ChatGPT doesn't know anything
so i asked ChatGPT (the thing that knows nothing) more questions and it said local variables like string s; do have a memory location on the stack. So since it technically has a memory location, wouldn't it be more technically correct to say it's uninitialized than to say undefined?
Well, uninitialized variables aren't as much about memory as they're about semantics.
What happens if you try to access a property of something that's undefined?
Are two undefined things equal?
Would you get a runtime exception?
Uninitialized variables don't have a memory location, because you literally cannot compile and run a program containing an uninitialized variable.
Like, memory locations are only a thing at runtime, so if you can't compile the program and run it then it doesn't make sense to ask where the thing that doesn't compile is located in memory.
oh i never thought of it in that way
yeah memory is only at runtime
And going the Javascript and Python route and having a literal
undefined
value is just asking for trouble, and C# already has enough trouble considering the existence of null.in python and JS i can console.log something that's undefined?
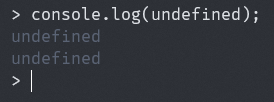
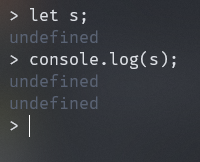
ahhh so C# really protects againsts errors that would have to do with something thats undefined
yep
All statically typed languages (at least that I know of) do that.
Since you have a compiler which has to analyze your code regardless, checking whether something is uninitialized is practically free-ish.
And languages like C++ and Rust which compile directly to machine code have to know memory locations at compile-time, so undefined would once again be an issue if you tried to get that to work. C# doesn't have to do that, but it still does certain optimizations which wouldn't work if undefined variables were a thing.
So tl;dr, variables cannot be undefined/uninitialized, because that's a compile error.
when creating class files, does the namespace reflect the folder hierarchy? I have a project call CSharpPractice and a folder called Classes and I attempted to create a class called BankAccount in the Classes folder
the namespace name was automatically set to "CSharpPractice.Classes"
is this a writing style in C#?
its a naming convention but not necessarily required
Generally speaking, yes, namespaces reflect the folder structure
I don't think this is too controversial, it makes sense
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.