Problem running a migration
Hello there,
I'm currently facing an issue with a database migration. I have two simple tables defined in my code (shown below) that I'm attempting to migrate to a MariaDB server.
I generated the migration SQL from my code, and it looks good to me. When I run the SQL directly in my MariaDB server using a SQL editor, it runs without any issues.
However, when I run the migration in my code, I receive an error message:
I'm not sure why this error is occurring. Can you help me figure out what's wrong with my migration?
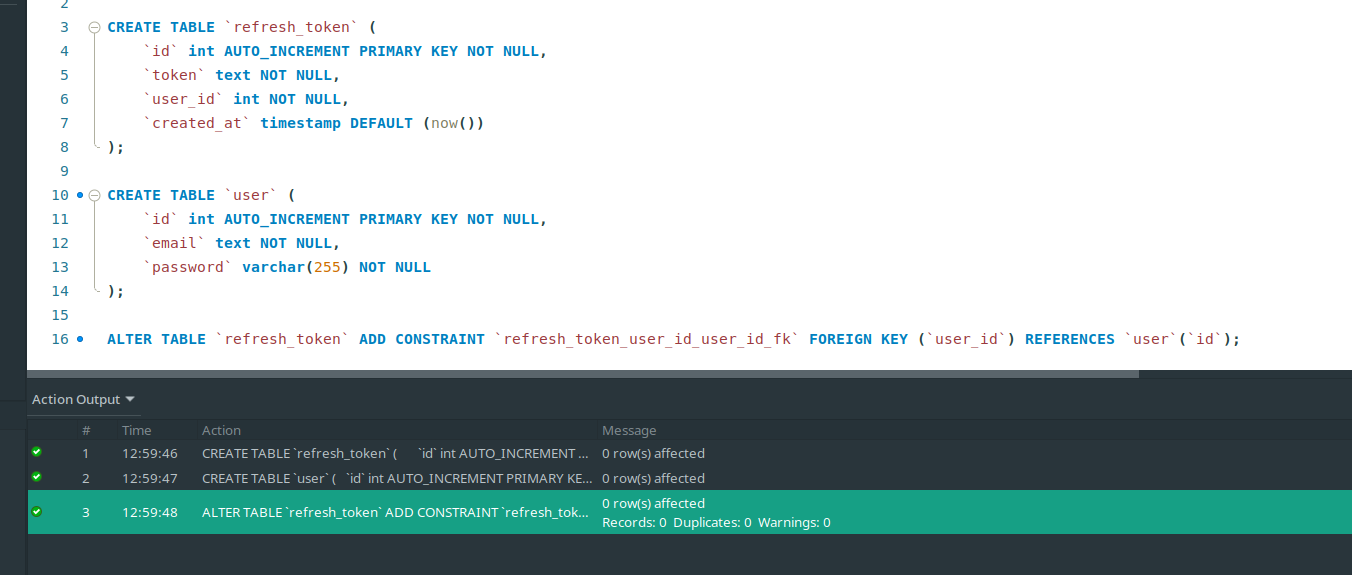
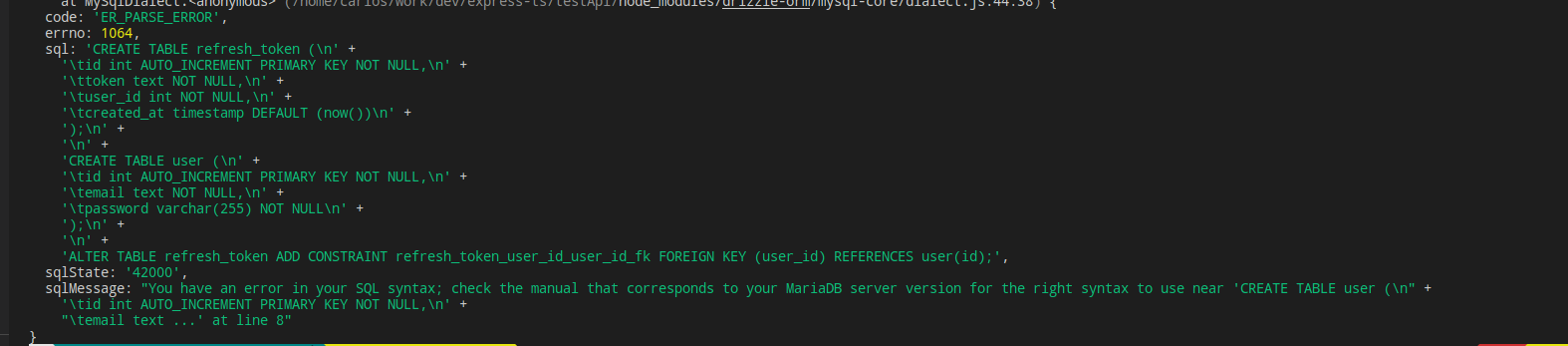
2 Replies
Add
breakpoints: true
to your Drizzle config
and regenerate the migrationsthanks u so much, that works