❔ ✅ Creating a console application in C# that utilizes async/await functions.
hi so i have to create a console application and the porpuse is to etrieve information on file sizes and the number of files in a specified directory. Your application should be able to handle multiple directory paths as input.
there were asyinc functions i had to use and want to see anyway i can change it for the better or make it simpler
`
21 Replies
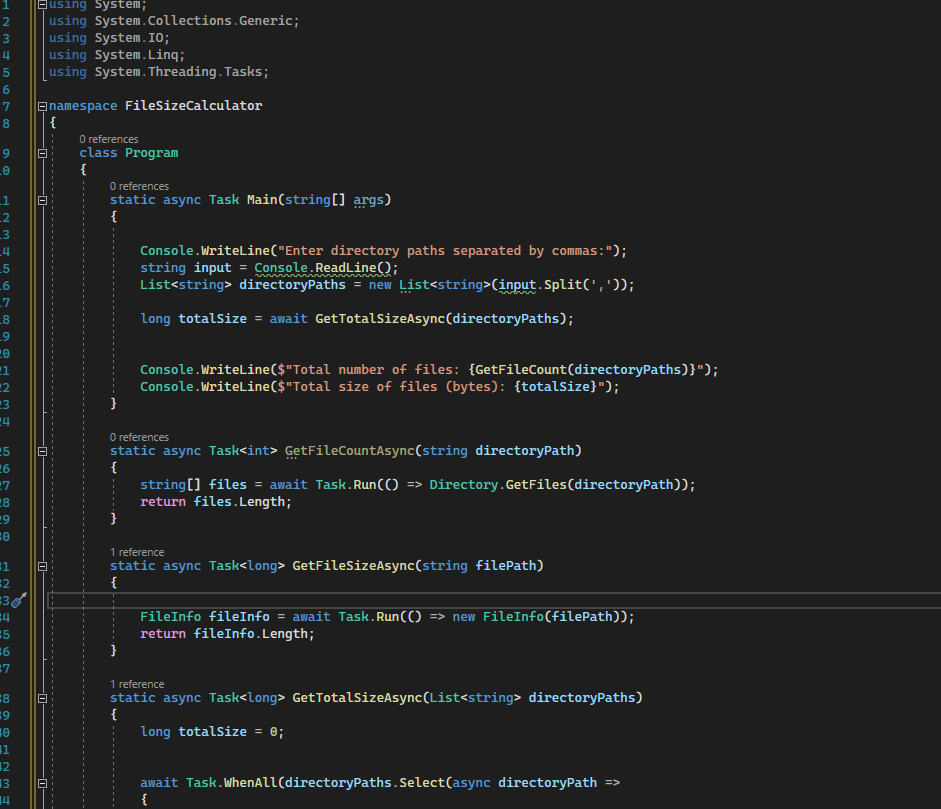
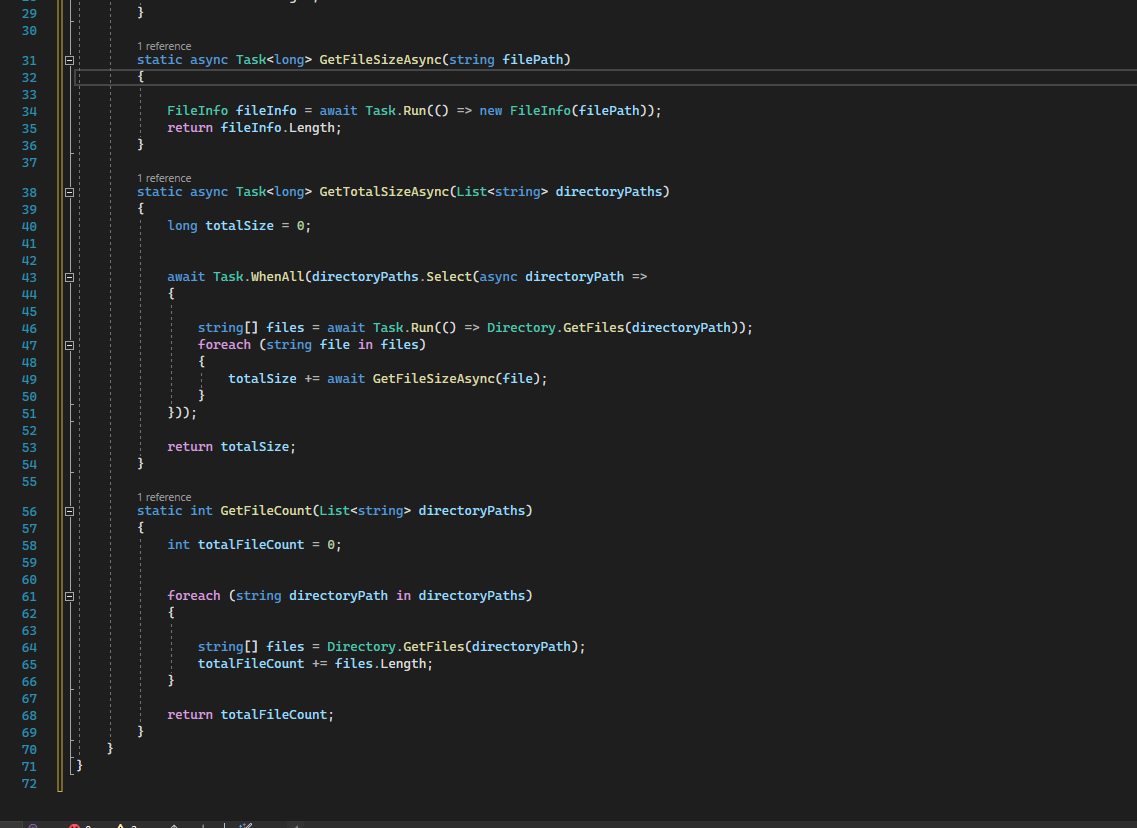
the main thing you need to learn about is the difference between io-bound and cpu-bound work
there's not much gain to taking io-bound work like this and wrapping it up in task.run
more specifically, this is very quick io-bound work - which is why the methods don't natively have async versions
(whereas, say,
File.ReadAllTextAsync
does exist)
generally, you would use Task.Run to push cpu-bound work onto another thread
i get the impulse to see anything to do with files, directories etc and to make it async - done it myself
but if the .NET core library doesn't get you an ...Async
method it's probably because it's not necessary or it's not possible (such as due to missing APIs from the underlying operating system)
for what it's worth your use of await Task.WhenAll
looks good 👍
though you could probably await everything into a long[]
and then use LINQ's .Sum()
to make it cleaner and avoid having a lambda capture the local variable totalSize
the
Task.WhenAll
?yeah
conceptually you're taking a string, the directoryPath, and turning it into longs
you could do that for all of them to get a big long[]
then do:
i cant use that yet
oh
is it one of those things where your teacher doesn't let you use certain language features until they say so
my condolences
i am just scared cuz staff is gonna check it with there own developer
wanted to see is there a way to make it better
wanted to check all the boxes
depends on what 'better' means
improve it like not making it dry
do u think its good enough?
there are things i would change, like using
var
everywhere, and ditching the Task.Run stuff as i mentioned
but it's fine
it looks like it would work and you have split up the code into methods of appropriate sizeoh ok
thank you for the help
i was worrying alot
ty so muchh and have a nice day
no prob. if you're a beginner then this is way better than most beginner code
ty man
and one more question do i need to close the post?
i don't think you have to but you can
oh i guess i can do it too
lmao
lol
ty again
I have a question regarding this too. Does it matter if we do
GetFiles().Length
or EnumerateFiles().Count()
? which is better?
I guess EnumerateFiles should be better because it doesnt load all of the filenames into memory at once but evaluates lazily?if you mean the snippet i posted, when
.ToArray()
is called it will load everything into memory at once anyway
the purpose of using EnumerateFiles is that, as an IEnumerable, it lets you chain together operations like .Select
which are executed as a single operation when you enumerate the collection
which makes simpler code than writing manual foreach loops, etc
i don't think there would be much of a performance differencedidnt even see your snippt tbh
but GetFiles() would also allow you to chain other linq operations
oh, right
probably no real difference in that case
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.