How to pass parameters from ListRecords to CreateRecord in Resources?
I have the following relations Menus > Categories > Products. I have
ListProducts
where I do allow user to choose a Menu
from a dropdown and picked menu_id
is saved via a $queryString
. So now when I click on a Create action I would like to carry this menu_id
to the Create page and display related Categories to the user when creating + keeping him in the same Menu. Any best practices on of how to achieve this in Filament?
15 Replies
this sort of structure isnt really natively supported yet, but @dufjix might have a solution for you. he's working on a package
Thank you for getting back to me. I was wondering if we could expose methods for the actions on url generation that would allow to override and pass custom parameters for the action urls. I could PR if you think this could be a good idea. 🙂
you can just pass
->url()
to override the default with your new paramsThe problem is that getUrl is a static method. The only override possible now is to completely override the configureCreateAction.
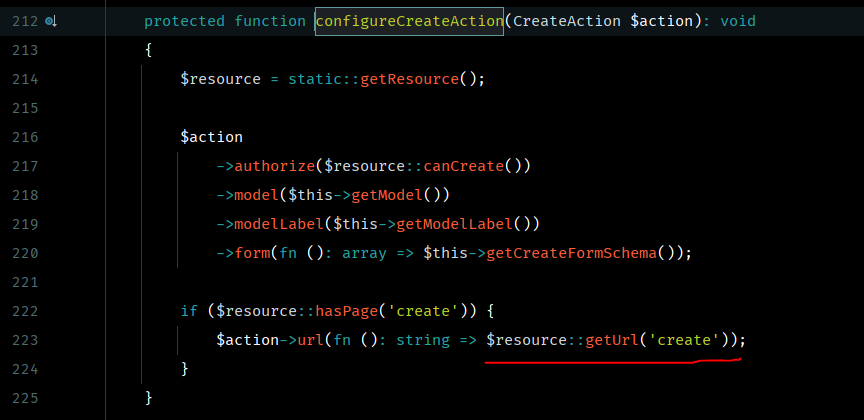
So I guess passing
->url()
to a custom action works but not as flexible when working within Resource default CRUD actions, unless I am not seeing a point. 🙂but if we could expose something like as a protected methods. This would allow to someone easily extend the default CRUD actions with custom parameters.
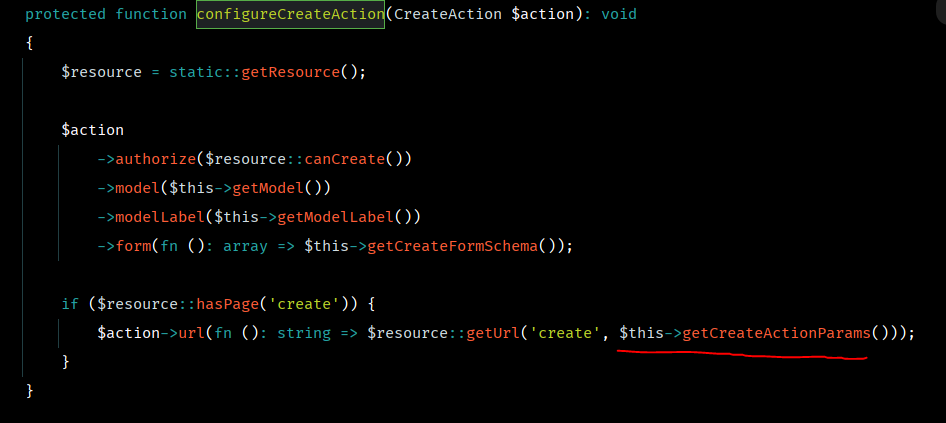
or just
$this->getCreateActionUrl
method and move the whole closure into it. So if someone needs custom URL he would not need to completely repeat the configureCreateAction
code.it doesnt matter if its static
CreateAction::make()->url(Resource::getUrl('create', ['params']))
Oh, I guess I got confused with
configureCreateAction
and thought I would lose some sort of the functionality. It looks like to work for what I need I guess.
One more question - if I wanted to override table edit action urls I would also need to include $record as a parameter to not break the Resources functionality, right?yes
EditAction::make()->url(fn ($record) => Resource::getUrl('edit', ['record' => $record]))
Nice! This is the way to even be able to build up nested routes too, just need a little bit of customisation but very possible. I have seen some questions in help that were looking for this.
Thank you for the great package you are building. I can't wait for v3. 👍 🤜
@.crylar check https://discord.com/channels/883083792112300104/970354547723730955/1093891100541980682
It may be what you need
wow this is very nice. I have actually started to build my own implementation some days ago but you got something going here! I do hope this ends up in the core eventually.
It does not need to be in there fully, just need some additonal helpers so we can reduce the package implementation footprint.
This was my process of thinking but I do see you are something heading in a similar direction.
I will check your package. 🙂