62 Replies
73
Or 45
again?
Idk, could be 37
It's not easy to provide an answer without a question
oh my bad
would it be passivle to go VC?
Not right now, no
after?
ok so
In the unspecified future, maybe
im trying to find the minimum of key strokes
when you can only use left right up down and enter
i had it working
but
is the problem clear?
Have you implemented a pathfinder for this?
A* is quite simple to implement and essentially solves this problem
you mean DFS or BFS
i havent used A* in my life so nope
It's basically like Dijkstra's but with a heuristic
it's actually quite simple to implement, and there are tons of implementations available online if you want something to reference
then you can just ask it to generate paths for you from wherever you are to every index of whatever letter you're looking for, and pick the shortest one
obviously if you wanted to minimise the total number of keystrokes for the entire operation you're approaching a travelling salesman problem
but with something this small you could bruteforce it
bruteforce it with A* you mean
yep
but even with multithreading, it's going to get quite expensive quite fast when size/string length increase
where can i paste my whole
code
$paste
If your code is too long, you can post to https://paste.mod.gg/ and copy the link into chat for others to see your shared code!
BlazeBin - uonabmvvdhnc
A tool for sharing your source code with the world!
my entire A* implementation for my unity project I'm working on at the moment, including a bunch of debug garbage and tons of helpers is ~300 lines
the actual code for building and solving the graph is ~100 max
so its working for 2 test cases but other 4 its
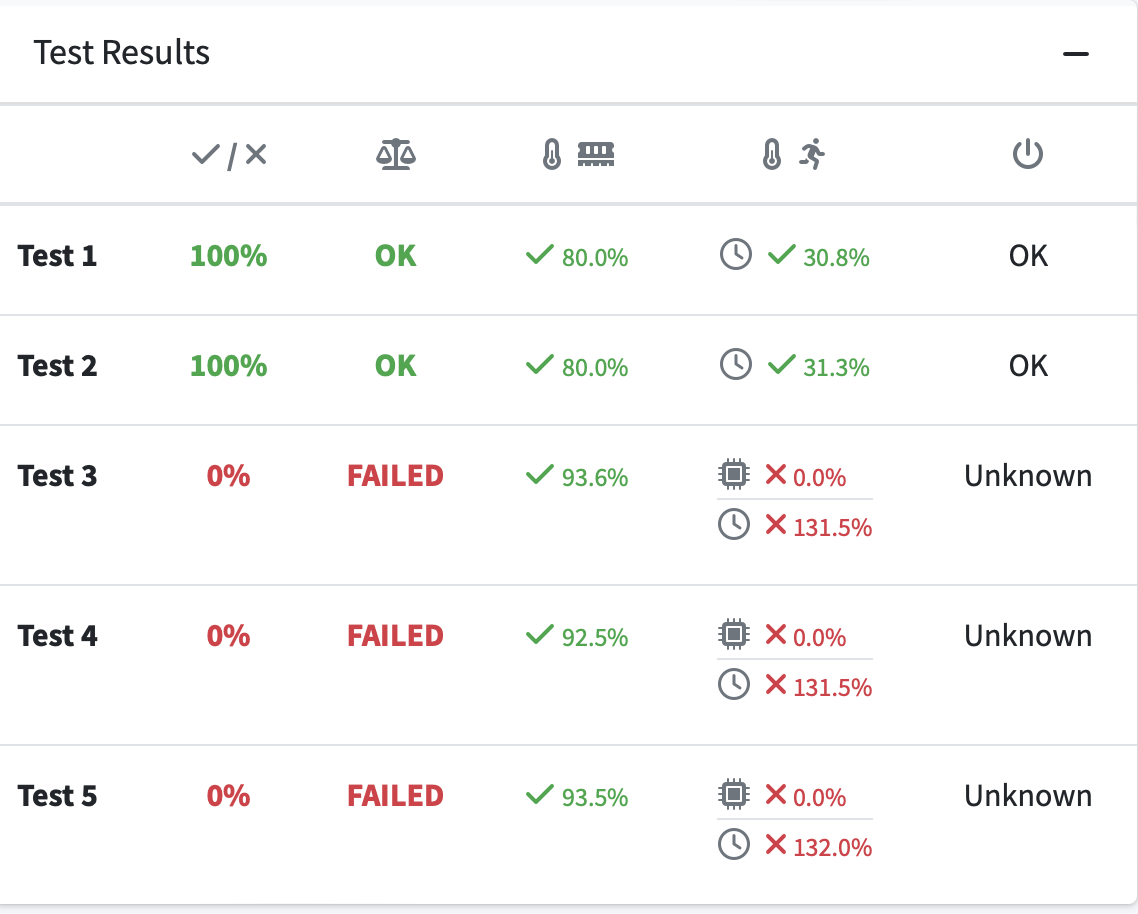
crashing because of execution time
you could almost certainly use an array here to hold the information
also you don't need
Tuple<int,int>
you can just do (int, int)
no need for new Tuple...
then, just (1, 10)
those won't help your execution time though
ah you're just calculating manhattan distance, that's entirely reasonable as a solution for this
shouldn't really be that slow then...well
dont know
implementing a solution myself as we speak, curious
with A* you mean
nah no need for it in this case, manhattan distance is fine
so how would you do it will you show me then
I think I'm in the right area
should really add some comments 

@Task.WhenAll wait if the letter is not in the grid we just omit it
oh, well then you can just add a
continue;
clause in the for loop in that case
I'll add it now actuallywait if i want to test it i need to make it general
there just modified it slightly to skip letters that aren't in the array
i mean i need to make it general for the n m word we finding and the grid
will look at it
give me a sec
no worries, was a fun little problem to solve, a good distraction from what I'm actually working on
shame I have no test cases locally to try it out
i will rewrite your code
and test it
but need to make it general so it can take input
@Task.WhenAll im sorry but how is your program working
when i paste it into my ide its not working
what .NET version are you on?
dont know where to check
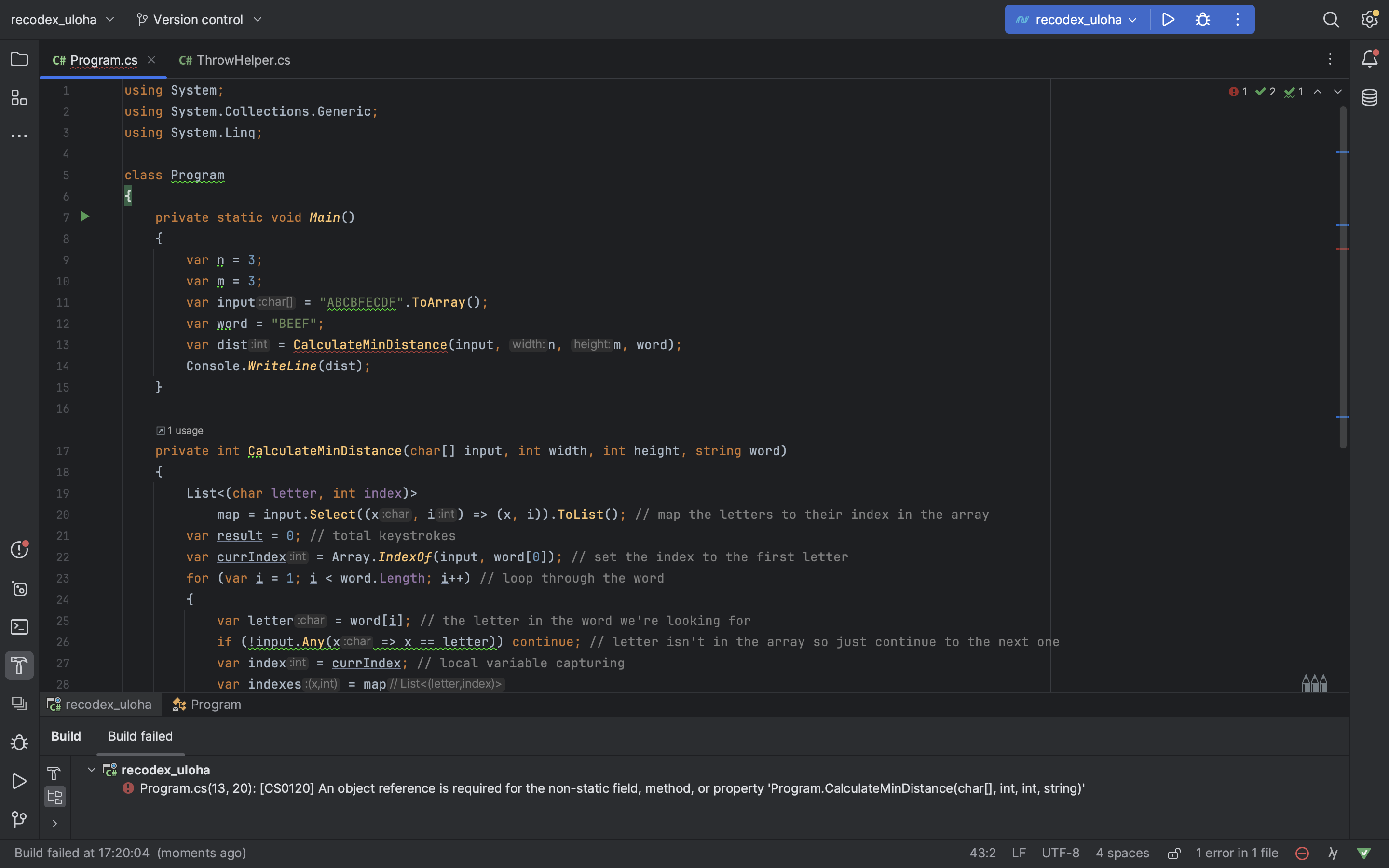
oh, that's my bad
you should make
CalculateMinDistance
static
didn't think about thateven tho 3 is not the right ans
is it not? B -> E is 2 steps, E -> E is 0 steps, E -> F is 1 step
3 steps
8
ah, fair enough, I can adjust that slightly
okey we can jsut do result +=1 if letter in grid
so its general for different inputs
yeah, all you have to change is
var currIndex = 0;
so it starts at a
in the top left and result += indexes.Item2 + 1;
add one extra keystroke for the enter
keystroke input
that's about it
oh and for(var i = 0;
so it actually starts at the start of the word now
instead of skipping ahead to the 2nd letteryep
same problem occured
as i was having
even that isn't fast enough?
even multithread this because each operation is reliant on the previous one
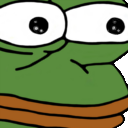
look
if it goes to the right its 17 if it goes downwards its 15
thats why i had list of pointers in my code so it checks different routs
ah in that case you'll need to implement A* for the heuristics, in A* you calculate manhattan distance as part of each decision you make
though even still that isn't guaranteed to work, this is nearly a travelling salesman problem
because you need the shortest path overall, not just the shortest path to each letter
ye but the grid will be max 256 char
bruteforcing it with breadthfirst is going to be quite slow for big grids
probably will have to implement the BFS
BFS might be overly slow for big grids with big words
though starting from the top left every time does limit the problem
but the shortest route could theoretically be to go all the way to the bottom right first, there's no actual way to know without brute forcing every path, which is a bit crappy
If i wanted that
how would i do that even with the going up so i have pointer bottom
@Task.WhenAll i found a code that does it
BlazeBin - isvmvwzmvalv
A tool for sharing your source code with the world!
interesting, not sure how that even works, currently adapting my pathfinder from my other project to see if I can get it to work with that
curious
thinking about it, probably not, it relies on manhattan distance as the heuristic and only looks at the next node, I'd have to implement another heuristic for the distance of the next node from the eventual goal too
breadth first is a solid solution as long as it's fast enough for you
well
i tried implementing it but failed
not the tests but i failed at implementation
BlazeBin - ojucgnoasydo
A tool for sharing your source code with the world!
@Task.WhenAll just checking if you found somehting beucase i didnt come up with anything
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.