❔ Implement New Class to JSON with Default Values
Hello,
I have a class as followed:
Which returns:
Now, let's say I add a new class to 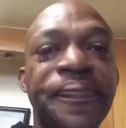
GuildModulesConfiguration
which would looks like this:
Whenever I try to deserialize and serialize the JSON file, it adds the new class as null without any values.
What I want to achieve is this:
Any ideas what I could possibly do? 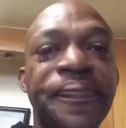
27 Replies
It seems you never instantiate that class
And a
default
value of a reference type is null
How would I go about instantiating it?
Like any other class
My code would be this:
Ah, well, if the JSON you're deserializing doesn't have the data you want... it won't have that data
I check if the modules.json file exists, and if not, it creates this result:
However, since json file depends on each guild, I don't wanna override their values back to default.
I actually hoped that adding default values to each key would serialize it but I assume that I have to define those values before serializing them? I just don't know whether that will create the same instance over and over again.
Yes, adding default values will deserialize them
So, this?
but neither property in this class has a default value
ah
I see, I know where you're heading with this.
Just initialize them with
= new();
I have to define those values within
GuildModulesConfiguration
rather than within their own class.Assuming of course your C# version is high enough to support shorthand constructors
Both
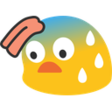
Angius#1586
REPL Result: Success
Result: string
Compile: 605.717ms | Execution: 59.915ms | React with ❌ to remove this embed.
ah
I see
(as a side note, as you can see,
Newtonsoft.Json
isn't really needed nowadays)I do know about that Json.NET exists but I never go into it
For some reason, i went with newtonsoft
Unless you're stuck with an outdated version of .NET, like working with Unity or some legacy projects, Newtonsoft won't give you anything that STJ won't
Well, besides being slow
Newtonsoft excels at being slow
Since I pretty much used Newtonsoft majorly throughout this project, I'm not sure if switching to STJ will break most code assuming that the method names are different
Method names are different, yes
I see, I tested your code above and defined the values and it seems like everything is working now. :D
Search-replace should handle most of it though
Nice
Thank you very much!
will consider using STJ on new projects, I see that literally every code on Stackoverflow uses STJ
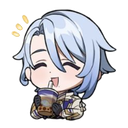
Yeah, as I said, no reason to use Newtonsoft in any new projects nowadays
Hell, the creator of Newtonsoft, James Newton-King was hired by Microsoft to work on STJ lol
oh lol
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.