❔ (Unsolved) writing from binary file to listbox
My teacher gave me code to implement for a highscore thing with binary files but in the code she gave us theres 2 undefined methods and ive no idea what they are supposed to even do. Anyone have any idea?
34 Replies
at the very botton its the
SetNextItem(highscoreArray, scoreNumber);
SetCurrentItem(highscoreArray, scoreNumber, username, score);
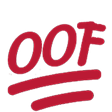
BinaryWriter
and Convert
, but I guess teachers be teachers and they're all stuck in 1999
Judging by the name of those methods, they're supposed to set an item to some value in the array
SetNextItem
is probably supposed to do something like highScoreArray[i + 1] = scoreNumber
Although, scoreNumber
seems to be an index in the array
So rather something like highScoreArray[scoreNumber + 1] = ???
SetCurrentItem
seems a bit more obvious, probably something akin to
Which is kinda atrocious in its own right
But, again, teachers being shit is kind of the normyeah idek she gave like me and 2 others this way of doing it as the 'harder' way and honestly i dont understand arrays much cause she skipped over that when teaching so i just try and avoid them as much as possible. Ill try putting something like those you gave in to see
Arrays are super simple tbh
An array is a collection of items
That's it
yeah like i understand what it is i just dk how to use them properly
To store multiple of the same kind of item
That's their one and only use
Yeah i mean as in like the actual code like what i write to use them, like its mainly the 2d arrays that idk
arr[0, 2]
will refer to k
in this case
That's all about a 2D arrayOhh k when i was trying to learn them in like the theory books she gave us it was so confusing
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Ok so i have a new error now
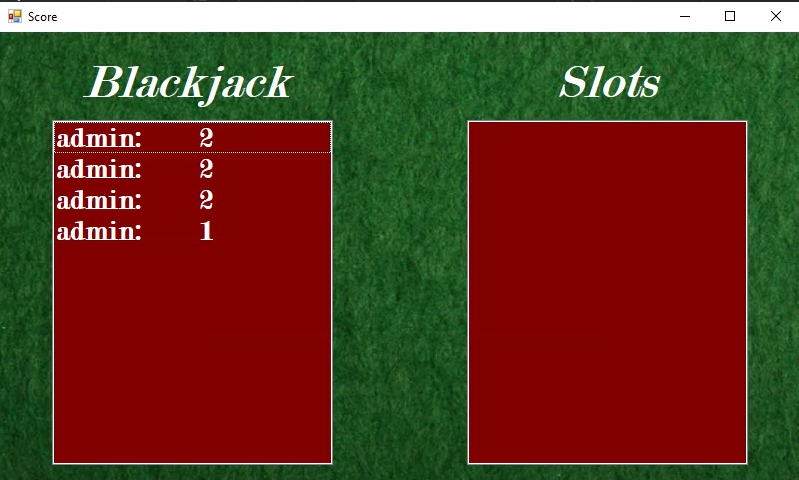
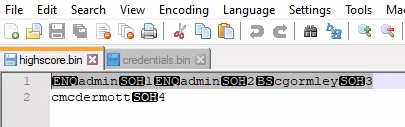
the scoreboard for some reason keeps repeating admin: 2 whn it should only be there once
and thats the code for putting it in the listbox
the other methods are up above
BlazeBin - wcsrmzqpvwba
A tool for sharing your source code with the world!
i cant get it to work no matter what i do ive been trying the past like 2 hours nearly
hello
Use the debugger, see what
blackjackScores
areby like putting in something like this? MessageBox.Show(Convert.ToString(blackjackScores));
$debug
Tutorial: Debug C# code - Visual Studio (Windows)
Learn features of the Visual Studio debugger and how to start the debugger, step through code, and inspect data in a C# application.
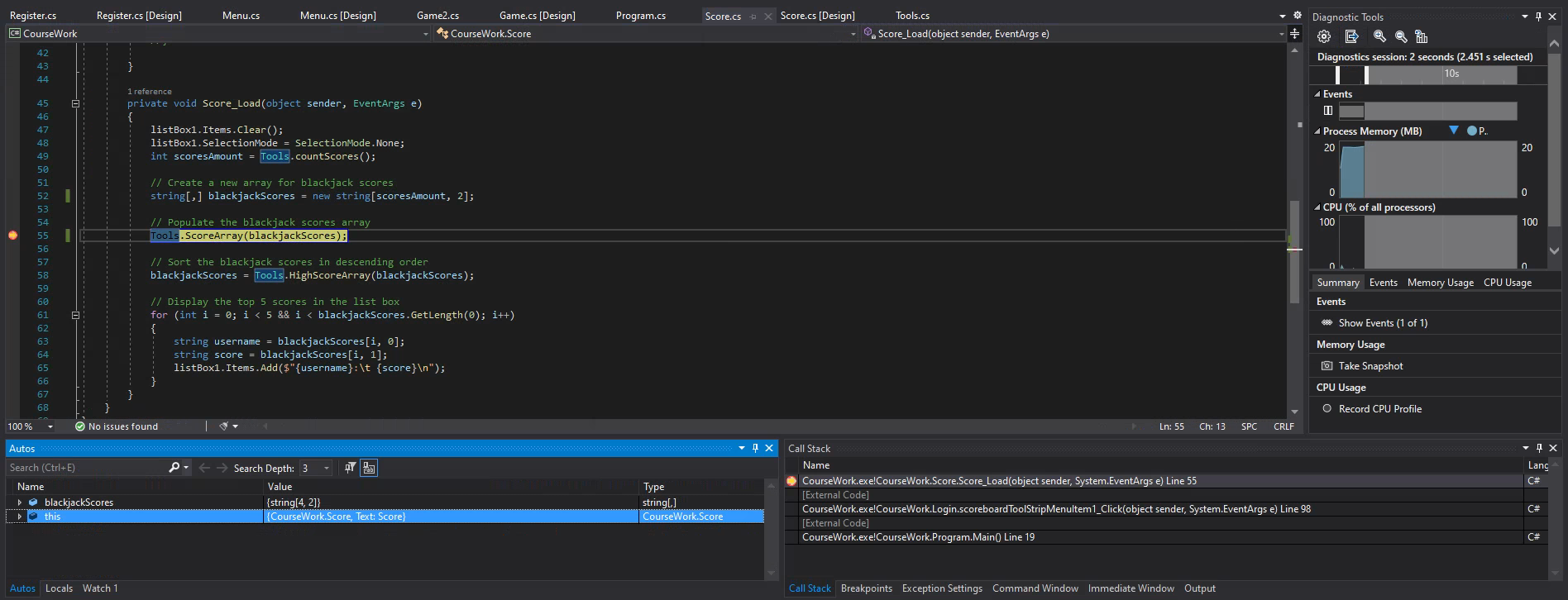
Expand it
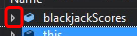
They all say null
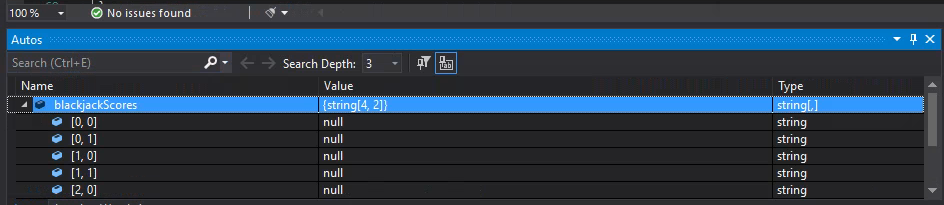
Step forward
Does
Tools.ScoreArray()
fill the array with values?this is the ScoreArray method
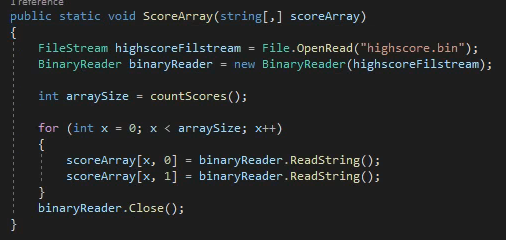
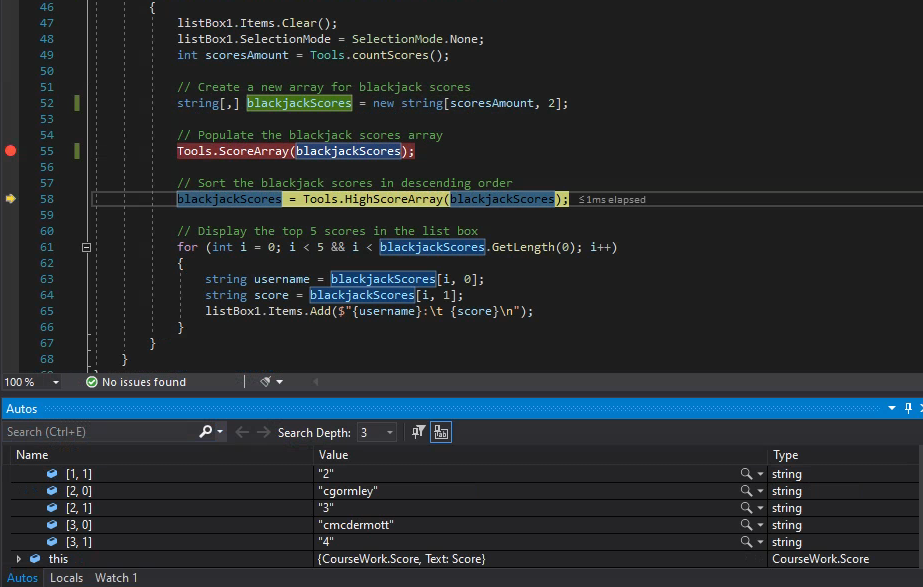
its filled with the correct values but it displays it wrong just
it must be something to do with the sorting then cause after that line it goes to this
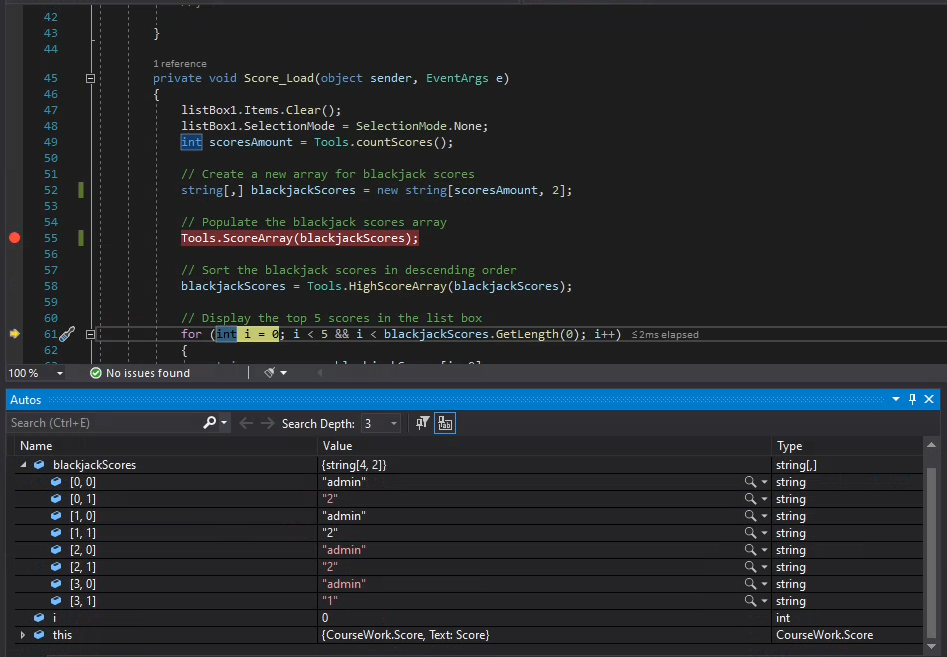
Probably, yes
its this method is where it goes wrong
¯\_(ツ)_/¯
I'll be honest, using a 2D array here instead of good and proper array of classes/records/structs muddies the waters a little too much for my liking
Use the debugger and see ig
alright thanks for trying though
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.