❔ ✅ can anyone tell me what my problem is?
is the code(i got the first part for the random letter off of the internet) and it just dos not work and i do not know why, i input a number and nothing happens
no errors and i dont know what that warning means
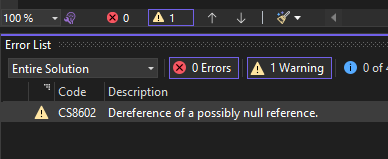
27 Replies
there is a lot wrong with that code to be honest
probably
it might help if you comment it and explain what each thing is supposed to be doing
is supposed to give me a random letter from a-z(lowercase) when i call for
that code is fine
yeah i took it off of google
the most obvious issue is that there is no way for your program to stop
the second is the part that seems like it should make it stop would never make it stop anyway because it checks things that don't change in the loop
is supposed to ask me how long i want the string to be
length asks the user
whentostop is used later to make i can
break;
on the exact length of length
why are you turning your numbers into strings?
well i need to turn them both into the same thing
when i think of it i can turn only one of them into a string since length is already a string
strings have a
.Length
property that gives you a number saying how many characters it has
is so i have an empty variable that i can add onto later
go through this code line by line and explain what it's supposed to do
well i didnt know that considering ive been trying to learn C# for like 3 days i barely know anything
you may also want to avoid
var
until you're more familiar with what types different things return
it makes it more obvious there's a redundant line there
second thing is you can convert a string to an integer with int.Parse
or int.TryParse
like 1:
outputs
"enter wanted length"
so the user knows they can and should input
like 2:
asks user for input and names it length
like 3:
makes a variable that is later used to stop a while (true)
when it reaches the same amount as length
like 4:
converts length
input into a string named slength
like 5:
oh...
yeah i think a problem is trying to add 1 to a string
gimme a minuteas for 3, you never actually use that variable to try to stop the loop
generally for while loops you want to have a condition for it to stop instead of just
while(true)
yeah i guess that would work too
gimme a minute to clean up some things
this is also a problem - you're adding 1 to the integer variable but that won't automatically update the one where you converted it to a string
aha it works now
one other thing you should consider changing is avoiding the
while (true)
because it makes it less obvious what you're trying to do
you could do something like while (whentoStop < ilength)
then put the writeline after the loop instead of inside iti want to get 1 line
thats why it prints once and then breaks
the change i suggested would have the exact same behavior
just structured in a more readable way
i can yeah
you don't have to, but it's what i would do
ok
ill keep that in mind next time i think of something
at least i learnt how to get user input
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.