✅ Reading a yaml file that has multiple types
I'm having trouble finding examples that show how, given a key, to return a value which may be a string, int or list.
var value = configuration[key];
works on primitive types only;
var value = configuration.GetSection(key).GetChildren().Select(x => x.Value).ToList();
works(ish) on list but not on primitive types.
Whats the path of least complexity to get any value from any key, leaving up to the user to make sure correct methods are used on correct types?8 Replies
https://github.com/aaubry/YamlDotNet
Deserialize to
If something can be either a string, a list, or an int, then it's garbage data you're working with and may God have mercy on your soul
no that's pretty usual for yaml actually
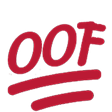
GitHub
GitHub - mcintyre321/OneOf: Easy to use F#-like ~discriminated~ uni...
Easy to use F#-like ~discriminated~ unions for C# with exhaustive compile time matching - GitHub - mcintyre321/OneOf: Easy to use F#-like ~discriminated~ unions for C# with exhaustive compile time ...
So if you can't change the data you're getting, this could be a way to get around it
You may need to add a converter for your yaml library so it knows how to handle it
that still requires defining types for all keys in yaml. I suppose I'm wondering (coming from python, so very new) is there a way to query for a value without first defining the types? i.e.
dynamic value = configuration[key];
whereas value could be anything - list, string - and leaving up to the user to interact with value correctly?I mean, you could use
object
worse comes to worst
But how do you reason about it?
If it happens to be a list, you can't do thing + 7
, but you can do that if it's an int
If it's a string you can't .Add()
to it, but you can if it's a list
EtcHere's the solution I came up with - intermediate deserialization to json, this way I avoid having to define a model. Would you say there are any immediate shortcomings, other than everything being string by default?
invoking