✅ The code only add root nodes into Queue instead of the whole pre-defined int arrays
I just got stuck with this puzzle https://leetcode.com/problems/binary-tree-level-order-traversal/solutions/3100951/easy-and-clean-solution/
My code is right here:
`
But the result is still not complete. The code only adds any root node into the queue/list while it was supposed to print out the binary tree with levels. (see the attached screenshot).
Could anyone kindly point me in the right direction?
LeetCode
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
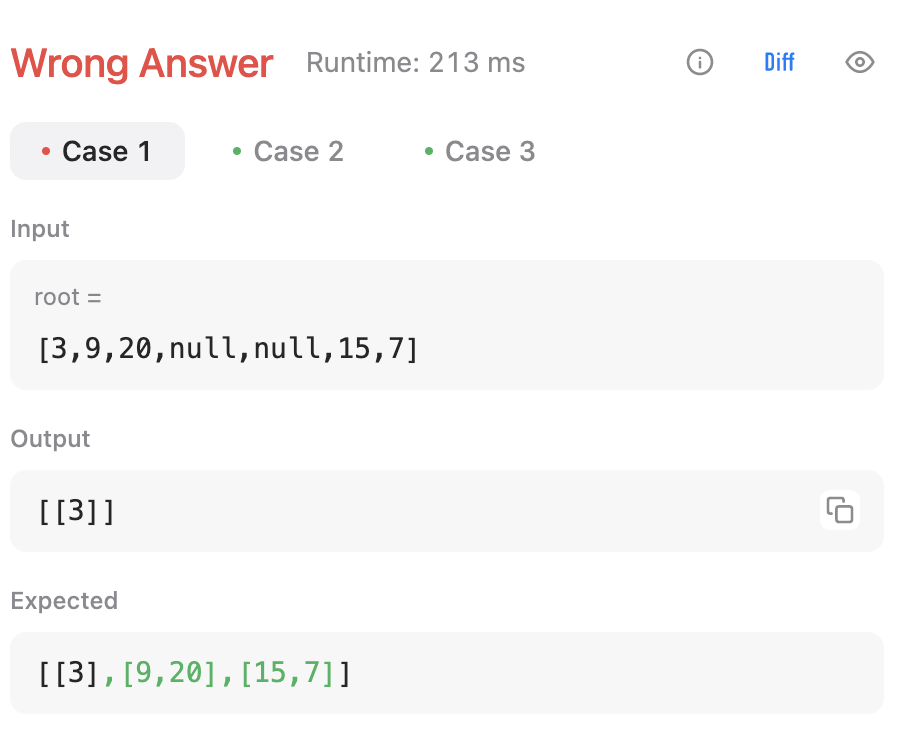
5 Replies
the issue is
curr.left
and curr.right
will always be null in your code
because your curr
node is not the root node but a new node that you created which doesn't have any children
your curr
node should be the one that you dequeue
from the queue, not a new node.
i suggest storing the Nodes in the qeueue with a Queue<TreeNode>
instead of their integer values. Then make curr
be the dequeued node and you can access its children
you only copied the integer value of the root node into your own node that you created, that doesn't make it hold the same child nodes
you understand @morry329 ?
you could ofc also copy over the child nodes to your newly created node but you better don't create new nodes at all hereWas this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.Thank you so much for your amazing explanation! One more small (trivial) question. So storing the nodes as a Queue<TreeNode> makes sense for this problem as we need to check if the child "node" has its own child elements? As I check other LC answers I have noticed List<int> as each level of child elements and in the end those answers return the List<IList<int>>, not List<IList<TreeNode>>. You see, I still have some gaps in understanding
you return List<IList<int>> because thats the method signature / return type LeetCode has given to you. however i personally think a
Queue<TreeNode>
is best suited to get to that result.
So storing the nodes as a Queue<TreeNode> makes sense for this problem as we need to check if the child "node" has its own child elementsexactly, we wanna check the
left
and right
fields of the type, so we gotta store something of that type reference. Storing an integer makes it so that we cant use it to access the other onesAhhhh I got it 🙂 I can see this puzzle very clearly now XD Thank you so much for answering my questions a couple of times, that was a massive help